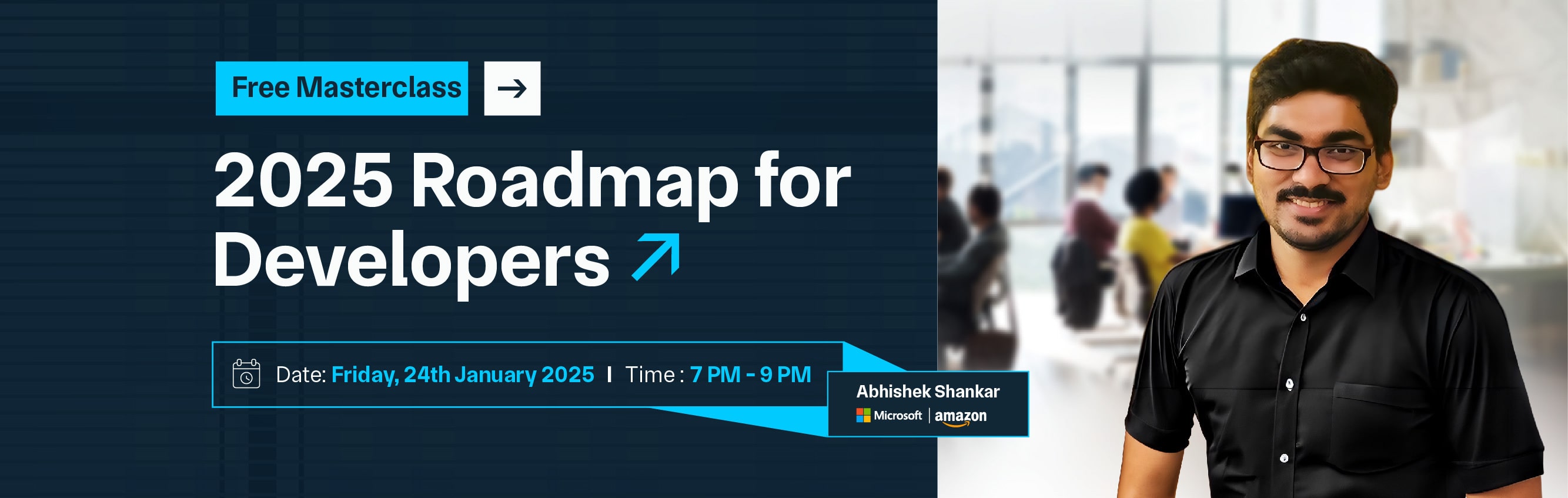

A SQL project typically involves designing and implementing a database system to efficiently manage specific types of data. It begins with defining the database schema, which outlines tables' structure, relationships, and attributes each table will store. For instance, in an Employee Management System, tables may include employee details such as ID, name, department, and salary, each with defined data types and constraints. Once the schema is established, the focus shifts to populating the database with relevant data and writing SQL queries to perform various operations.
These operations include inserting new records, updating existing ones, retrieving information based on specific criteria, and deleting data as needed. SQL queries are also used for aggregating data, generating reports, and conducting analyses to derive insights from the stored information. Throughout the project, attention is given to ensuring data integrity, optimising query performance, and adhering to best practices in database management.
SQL projects provide hands-on experience with database design and management and enhance skills in writing efficient queries and understanding relational database concepts, which are essential in database administration, data analysis, and software development. By focusing on database design principles, SQL syntax, and practical application, beginners can build a solid foundation in SQL while developing valuable skills for future database management endeavours.
SQL (Structured Query Language) is a standard language for managing and manipulating relational databases. It was developed in the early 1970s and has become the de facto language for database interaction. SQL allows users to define, manipulate, and control data stored in a relational database management system (RDBMS).
SQL is widely used across various industries for tasks ranging from simple data retrieval to complex database administration and management. It is supported by all major database management systems such as MySQL, PostgreSQL, Oracle Database, Microsoft SQL Server, and SQLite, making it a versatile and essential skill for database professionals, data analysts, software developers, and anyone involved in working with data.
Embark on a SQL project journey to master database management and query skills. From designing database schemas to writing complex SQL queries, this project offers hands-on experience in data manipulation, analysis, and optimisation. Ideal for beginners looking to build foundational SQL expertise in a practical, real-world context.
By following these steps, you can effectively plan, design, implement, and optimise your SQL project, gaining valuable experience and proficiency in SQL database management and query execution along the way.
Choosing the right SQL project depends on several factors, including your learning goals, interests, and the skills you want to develop. Here are some considerations to help you choose the right SQL project:
1. Interest and Relevance: Select a project that aligns with your interests or is relevant to your career goals. For example, if you're interested in finance, a project involving financial data analysis or portfolio management might be suitable.
2. Complexity Level: Consider your current skill level in SQL. Beginners may start with simpler projects like creating a basic database for employee management, while more experienced learners may tackle complex projects involving data integration, optimisation, or advanced querying.
3. Learning Objectives: Define what you want to learn from the project. Do you want to improve your SQL querying skills, understand database design principles, or learn about specific SQL features like joins or subqueries?
4. Availability of Data: Ensure you have access to appropriate data for your project. You can use sample datasets available online or create your dataset based on your project requirements.
5. Practical Application: Choose a project that has practical applications and simulates real-world scenarios. Projects that mimic industry-standard applications (e.g., e-commerce platforms, inventory management systems) can provide valuable experience.
6. Challenge and Growth: Select a project that challenges you and encourages growth in your SQL skills. This could involve tackling a new aspect of SQL you have yet to explore before or integrating SQL with other technologies (e.g., Python for data analysis).
7. Resources and Support: Consider the resources and support available to you. Are there tutorials, online courses, or forums where you can seek guidance and learn from others working on similar projects?
8. Portfolio Building: If you're building a portfolio for job applications or further education, choose a project that demonstrates your skills and showcases your ability to solve real-world problems using SQL.
By considering these factors, you can identify a SQL project that not only meets your learning objectives but also provides a rewarding experience in developing and applying SQL skills effectively.
1. Employee Management System
2. Library Catalog System
3. Student Information System
4. Inventory Tracking System
5. Hotel Reservation System
6. Customer Orders Database
7. Online Store Database
8. Bank Account Management System
9. Flight Booking System
10. Music Playlist Database
11. Blog Post Management System
12. Recipe Database
13. Event Registration System
14. Pet Adoption Database
15. Movie Rental Database
16. Job Application Tracking System
17. Gym Membership Database
18. Online Survey Database
19. Restaurant Menu Management System
20. Customer Support Ticket System
An Employee Management System database stores employee details such as ID, name, department, and salary. It facilitates basic CRUD operations (Create, Read, Update, Delete) to manage employee records efficiently.
This system helps HR departments track personnel information, monitor employee performance, and manage payroll. SQL queries can be implemented to add new employees, update existing records, retrieve employee information based on various criteria, and generate reports for management review.
Source code: Click Here
Example: Manages employee details such as ID, name, department, salary, and benefits. Facilitates CRUD operations for adding, updating, and deleting employee records. Useful for HR departments to track personnel information, manage payroll, and generate reports.
Functions:
A Library Catalog System database organises book information, including titles, authors, genres, and availability status. It supports functionalities for borrowing and returning books, managing overdue fines, and cataloguing new acquisitions.
SQL queries enable librarians to search for books by title, author, or genre, check availability, update inventory records, and generate reports on popular titles or borrowed volumes.
Source Code: Click Here
Example: Organizes book titles, authors, genres, and availability. Supports borrowing and returning books, manage overdue fines, and catalogs new acquisitions. Helps librarians efficiently manage library inventory and assist patrons in finding books.
Functions:
A Student Information System database stores student details like ID, name, courses enrolled, grades, and attendance records. It facilitates queries to retrieve student transcripts, calculate GPA, track academic progress, and manage course schedules.
This system is essential for educational institutions to maintain accurate student records, monitor academic performance, and generate reports for parents and faculty members.
Source Code: Click Here
Example: Stores student details like ID, name, courses enrolled, grades, and attendance. Calculates GPA, manages course schedules, and tracks academic progress. Essential for educational institutions to maintain accurate student records and facilitate academic administration.
Functions:
An Inventory Tracking System database manages product inventory with details such as product ID, name, quantity in stock, and unit price.
It supports queries for updating stock levels, checking product availability, and generating inventory reports. This system helps businesses streamline inventory management, track sales trends, and optimise stock levels to prevent overstocking or shortages.
Source Code: Click Here
Example: Manages product inventory with details such as ID, name, quantity, and price. Tracks stock levels, handle sales orders, and generates inventory reports. Helps businesses optimise inventory management and ensure product availability.
Functions:
A Hotel Reservation System database handles hotel room bookings, guest details, reservation dates, and availability. It supports queries to manage room reservations, check-in/check-out processes, and calculate booking durations. This system is crucial for hotels to efficiently manage room occupancy, handle guest reservations, and provide personalised services based on guest preferences.
Source Code: Click Here
Example: Handles hotel room bookings, guest details, reservation dates, and room availability. Manages check-ins, check-outs, and booking cancellations. Essential for hotels to efficiently manage room occupancy and provide personalised guest services.
Functions:
A Customer Orders Database tracks customer orders, including order details, products purchased, order status, and transaction history. It facilitates queries for managing order processing, updating order statuses, and generating sales reports. This system helps businesses monitor sales performance, track customer preferences, and streamline order fulfilment processes.
Source Code: Click Here
Example: Tracks customer orders, including order details, products purchased, and order status. Manages order processing, updates inventory levels, and generates sales reports. Crucial for businesses to manage sales operations and track customer purchases.
Functions:
An Online Store Database manages product catalogues, customer orders, payments, and inventory levels. It supports queries for managing product listings, processing online orders, and analysing sales data.
This system enables e-commerce businesses to optimise product offerings, track customer purchases, and ensure efficient order fulfilment and inventory management.
Source Code: Click Here
Example: Manages product catalogues, customer orders, payments, and inventory levels for an e-commerce platform. Processes online orders, manages product listings, and analyses sales data. Helps businesses operate and optimise their online sales channels.
Functions:
A Bank Account Management System database stores account details, transactions, customer information, and account balances. It supports queries for managing deposits, withdrawals, fund transfers, and generating account statements.
This system helps banks and financial institutions manage customer accounts securely, track financial transactions, and provide accurate financial reports and statements.
Source Code: Click Here
Example: Stores account details, transactions, and customer information. Manages deposits, withdrawals, fund transfers, and account balances. Essential for banks to provide secure account management and financial services to customers.
Function:
A Flight Booking System database manages flight schedules, passenger bookings, seat availability, and airline ticketing information. It supports queries for handling flight reservations, checking seat availability, and processing booking cancellations.
This system enables airlines and travel agencies to efficiently manage flight operations, monitor passenger bookings, and optimise seat allocation based on demand.
Source Code: Click Here
Example: Manages flight schedules, passenger bookings, seat availability, and airline ticketing information. Handles flight reservations, seat assignments, and booking cancellations. Crucial for airlines and travel agencies to manage flight operations and passenger bookings.
Functions:
A Music Playlist Database organises song titles, artists, genres, and user-created playlists. It supports queries for managing playlists, adding/removing songs, and generating playlist recommendations.
This system allows music streaming platforms to personalise user experiences, track user preferences, and recommend music based on listening history and genre preference.
Source Code: Click Here
Example: Stores song titles, artists, genres, and user-created playlists. Manages playlist creation, song additions/removals, and user interactions. Helps music streaming platforms personalise user experiences and recommend music based on preferences.
Functions:
A Blog Post Management System database stores blog posts, authors, categories, publication dates, and reader comments. It supports queries for managing blog content, searching posts by category or author, and displaying recent blog entries.
This system helps bloggers and content creators publish, organise, and promote blog content effectively, engage with readers, and analyse reader engagement through comments and views.
Source Code: Click Here
Example: Stores blog posts, authors, categories, publication dates, and reader comments. Manages blog content, facilitates post creation/editing, and displays blog entries. Crucial for bloggers and content creators to manage and publish blog content effectively.
Functions:
A Recipe Database stores recipes, ingredients, cooking instructions, and user ratings. It supports queries for searching recipes by ingredients, displaying top-rated recipes, and managing user-generated recipe collections. This system helps cooking enthusiasts and culinary professionals organise recipes, discover new dishes, and share cooking experiences with others.
Source Code: Click Here
Example: Stores recipes, ingredients, cooking instructions, and user ratings. Manages recipe creation, ingredient lists, and recipe collections. Helps cooking enthusiasts organise recipes, discover new dishes, and share culinary experiences.
Functions:
An Event Registration System database manages event details, attendee registrations, payment information, and event schedules. It supports queries for handling attendee registrations, checking event capacities, and generating attendee lists.
This system enables event organizers to streamline event planning, manage participant registrations efficiently, and ensure a seamless attendee experience.
Source Code: Click Here
Example: Manages event details, attendee registrations, payment information, and event schedules. Handles attendee management, event capacity tracking, and registration processing. Essential for event organizers to manage and coordinate events efficiently.
Functions:
A Pet Adoption Database tracks pet details, adoption status, shelter information, and adoption records. It supports queries for managing pet adoptions, searching for available pets, and tracking adoption histories.
This system helps animal shelters and rescue organisations manage pet intake, promote pet adoption events, and connect pets with potential adopters.
Source Code: Click Here
Example: Tracks pet details, adoption status, shelter information, and adoption records. Manages pet intake, adoption applications, and adoption histories. Helps animal shelters and rescue organisations match pets with potential adopters.
Functions:
A Movie Rental Database manages movie titles, genres, rental histories, customer details, and rental transactions. It supports queries for managing movie rentals, tracking overdue rentals, and generating rental reports. This system helps movie rental stores and streaming services track movie availability, manage customer accounts, and analyze rental trends.
Source Code: Click Here
Example: Manages movie titles, genres, rental histories, customer details, and rental transactions. Handles movie rentals, late fees, and customer accounts. Helps movie rental stores and streaming services manage movie inventory and customer rentals.
Functions:
A Job Application Tracking System database manages job listings, applicant details, application statuses, and interview schedules. It supports queries for posting job openings, tracking applicant progress, scheduling interviews, and generating hiring reports.
This system helps HR departments and recruiters streamline the hiring process, manage candidate information efficiently, and make informed hiring decisions based on applicant qualifications and interview performance.
Source Code: Click Here
Example: Tracks job listings, applicant details, application statuses, and interview schedules. Manages job postings, applicant tracking, and interview scheduling. Essential for HR departments and recruiters to streamline the hiring process and manage candidate interactions.
Functions:
A Gym Membership Database stores member details, membership plans, attendance records, and fitness class schedules. It supports queries for managing membership registrations, tracking attendance, renewing memberships, and generating membership reports.
This system helps fitness centers and gyms manage member interactions, monitor facility usage, and optimize class schedules based on member preferences and attendance patterns.
Source Code: Click Here
Example: Stores member details, membership plans, attendance records, and fitness class schedules. Manages membership registrations, tracks member attendance, and processes membership renewals. Helps gyms and fitness centers manage member interactions and facility usage.
Functions:
An Online Survey Database stores survey questions, respondent details, responses, and survey results. It supports queries for creating surveys, distributing them to participants, collecting responses, and analyzing survey data.
This system helps organizations conduct market research, gather customer feedback, measure employee satisfaction, and make data-driven decisions based on survey insights.
Source Code: Click Here
Example: Stores survey questions, respondent details, responses, and survey results. Manages survey creation, distribution, response collection, and data analysis. Helps organizations gather feedback, conduct market research, and make data-driven decisions.
Functions:
A Restaurant Menu Management System database manages menu items, prices, categories, and customer orders. It supports queries for updating menu items, managing inventory levels, processing customer orders, and generating sales reports.
This system helps restaurants optimize menu offerings, track popular dishes, manage ingredient stocks, and provide efficient service based on customer preferences and ordering trends.
Source Code: Click Here
Example: Manages menu items, prices, categories, and customer orders for a restaurant. Handles menu updates, order processing, and inventory management. Essential for restaurants to manage menu offerings, track sales, and optimize food service operations.
Functions:
A Customer Support Ticket System database tracks customer support tickets, issue details, customer information, and ticket statuses. It supports queries for managing ticket assignments, tracking ticket resolutions, and generating support ticket metrics.
This system helps customer support teams prioritize and resolve customer issues efficiently, track service levels, and maintain customer satisfaction through the timely resolution of support requests.
Source Code: Click Here
Example: Tracks customer support tickets, issue details, customer information, and ticket statuses. Manages ticket assignments, tracks ticket resolutions, and generates support metrics. Crucial for customer support teams to handle and resolve customer issues efficiently.
Functions:
SQL projects find diverse applications across various industries and domains. Here are some common use cases where SQL projects are beneficial.
SQL projects for beginners offer several advantages that contribute to a solid foundation in database management and SQL skills.
When embarking on SQL projects for beginners, there are several limitations and challenges to consider.
SQL projects for beginners provide invaluable hands-on experience in database management and query execution. These projects offer opportunities to learn essential SQL skills such as creating databases, defining tables, querying data with SELECT statements, and performing CRUD operations (Create, Read, Update, Delete). By working on SQL projects, beginners can gain confidence in manipulating data, optimizing database performance, and generating meaningful insights through reports and analysis.
Moreover, these projects simulate real-world scenarios across various domains like HR, education, e-commerce, and more, preparing beginners for practical challenges in database management roles. Overall, SQL projects serve as a solid foundation for mastering SQL fundamentals and building a rewarding career in database development and administration.
Copy and paste below code to page Head section
SQL (Structured Query Language) is a standard language for managing and manipulating relational databases. It allows users to define, access, and manipulate data in databases.
SQL projects are important because they provide hands-on experience in managing databases, querying data, and designing database schemas. They help in developing practical skills that are highly valued in various industries, such as IT, finance, healthcare, and retail.
SQL projects help you learn skills such as database design, writing SQL queries (Select, Insert, Update, Delete), data manipulation, database normalization, and database management. These skills are essential for roles in database administration, data analysis, software development, and more.
SQL projects benefit businesses by improving data management practices, enabling better decision-making through data-driven insights, automating repetitive tasks, enhancing customer service through personalized interactions, and optimizing operational efficiency.
Beginner-friendly SQL projects include Employee Management Systems, Library Catalog Systems, Student Information Systems, Inventory Tracking Systems, and Hotel Reservation Systems. These projects help in understanding basic database operations and building foundational SQL skills.
For SQL projects, you typically need a relational database management system (RDBMS) such as MySQL, PostgreSQL, Microsoft SQL Server, or SQLite. Additionally, tools like SQL Server Management Studio (SSMS), MySQL Workbench, or pgAdmin can be used for database administration and development tasks.