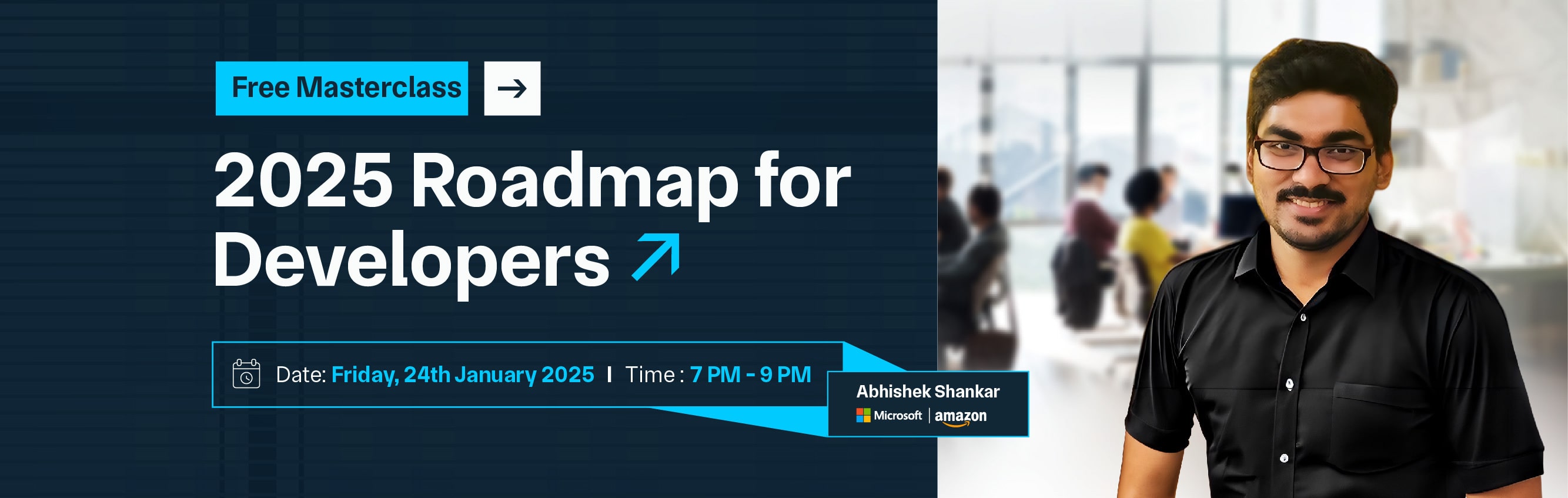

The componentDidUpdate() method in ReactJS is a crucial lifecycle method that gets invoked immediately after a component updates. This includes updates due to changes in props or state. It provides developers with an opportunity to perform side effects based on these changes, such as fetching new data or updating the DOM. The method receives two parameters: prevProps and prevState, which allow developers to compare the current props and state with their previous values.
This comparison is vital to avoid unnecessary updates or infinite loops, particularly when calling setState() within the method. For instance, if a component’s count state changes, componentDidUpdate() can be used to log the new count or trigger an API call based on the updated state. While it’s powerful, developers should use it judiciously, keeping performance in mind, as excessive or complex operations can lead to sluggishness.
As React evolves with hooks, the functionality of componentDidUpdate() can also be replicated using the useEffect hook in functional components, making it easier for developers to manage side effects without relying on class components. Overall, componentDidUpdate() is essential for building responsive and efficient React applications.
componentDidUpdate is a lifecycle method in React that is invoked immediately after a component updates. This can occur when the component's state or props change. It is a critical method for managing side effects in response to updates. It allows developers to perform actions like data fetching or DOM manipulation based on the new state or props.
1. Invocation Timing: It is called after the render method, meaning the updated component is now in the DOM.
2. Parameters: The method receives two arguments:
3. Common Use Cases:
4. Avoiding Infinite Loops: Care should be taken to compare the new props or state with the previous ones to avoid unnecessary updates and potential infinite loops when calling setState.
5. Transition to Hooks: In functional components, similar behavior can be achieved using the useEffect hook, which provides a more concise way to handle side effects.
Overall, componentDidUpdate is essential for maintaining the responsiveness and functionality of React components in dynamic applications.
The syntax for the componentDidUpdate() method in a React class component is straightforward.
Here’s how it looks:
componentDidUpdate(prevProps, prevState) {
// Your code here
}
Here’s a simple example demonstrating the syntax and usage of componentDidUpdate():
class ExampleComponent extends React.Component {
constructor(props) {
super(props);
this.state = { count: 0 };
}
incrementCount = () => {
this.setState((prevState) => ({ count: prevState.count + 1 }));
};
componentDidUpdate(prevProps, prevState) {
if (prevState.count !== this.state.count) {
console.log(`Count has changed to: ${this.state.count}`);
// Perform actions based on the count change
}
}
render() {
return (
<div>
<p>Current Count: {this.state.count}</p>
<button onClick={this.incrementCount}>Increment</button>
</div>
);
}
}
In this example, componentDidUpdate() checks if the count state has changed and logs the new value. This demonstrates how to use the method to respond to state changes effectively.
The componentDidUpdate() method in React is called immediately after a component updates. This can happen under several circumstances:
The componentDidUpdate() method is particularly useful in various scenarios where you need to respond to changes in a React component. Here are some common use cases for when to use componentDidUpdate():
When a component receives new props that require fetching data (e.g., when a user ID prop changes), you can use componentDidUpdate() to trigger a data fetch.
componentDidUpdate(prevProps) {
if (prevProps.userId !== this.props.userId) {
this.fetchUserData(this.props.userId);
}
}
If you need to perform side effects based on state changes—like updating external libraries or APIs—componentDidUpdate() is the right place to do it.
componentDidUpdate(prevState) {
if (prevState.count !== this.state.count) {
// Trigger an animation or external API call
}
}
If you need to perform direct DOM manipulations (like focusing an input field) based on prop or state changes, you can do this in componentDidUpdate().
componentDidUpdate(prevProps) {
if (prevProps.isEditing !== this.props.isEditing && this.props.isEditing) {
this.inputRef.focus();
}
}
If your component needs to derive a state based on prop changes, componentDidUpdate() can be useful for comparing previous and current props and updating the state accordingly.
You can use componentDidUpdate() to log changes for debugging or tracking user interactions, helping you gather insights about how users are interacting with your component.
The shouldComponentUpdate() lifecycle method in React is a powerful tool for optimizing performance by controlling when a component should re-render. By implementing this method, developers can minimize unnecessary updates, which is especially useful in components that render frequently or have complex rendering logic.
shouldComponentUpdate() is called before the rendering process when new props or states are received. It allows you to specify whether the component should be updated or not based on the changes in props and state.
shouldComponentUpdate(nextProps, nextState) {
// Return true to allow the update, false to prevent it
}
React.PureComponent is an optimized version of React.Component that implements a shallow comparison of props and state. It automatically handles updates, allowing you to avoid unnecessary re-renders when the data hasn't changed. Here's how to use React.PureComponent effectively for performance improvements in your React applications.
React.PureComponent is a class that extends React.Component. The main difference is that PureComponent performs a shallow comparison of props and state in its shouldComponentUpdate() method. If the previous props and state are the same as the next props and state, the component will not re-render.
Here's a step-by-step guide on how to implement React.PureComponent:
import React from 'react';
Define your component by extending React.PureComponent instead of React.Component.
class MyPureComponent extends React.PureComponent {
render() {
return <div>{this.props.text}</div>;
}
}
You can now use MyPureComponent just like any other React component:
class ParentComponent extends React.Component {
state = {
text: 'Hello, World!',
};
updateText = () => {
this.setState({ text: 'Hello, React!' });
};
render() {
return (
<div>
<MyPureComponent text={this.state.text} />
<button onClick={this.updateText}>Update Text</button>
</div>
);
}
}
Here are several examples demonstrating how to use the componentDidUpdate() lifecycle method in React. Each example showcases a different use case for handling updates effectively.
In this example, a component fetches user data whenever the userId prop changes.
class UserProfile extends React.Component {
state = {
userData: null,
};
componentDidMount() {
this.fetchUserData(this.props.userId);
}
componentDidUpdate(prevProps) {
// Fetch new data if userId changes
if (prevProps.userId !== this.props.userId) {
this.fetchUserData(this.props.userId);
}
}
fetchUserData(userId) {
// Simulating an API call
fetch(`https://api.example.com/users/${userId}`)
.then(response => response.json())
.then(data => this.setState({ userData: data }));
}
render() {
const { userData } = this.state;
return (
<div>
{userData ? (
<h1>{userData.name}</h1>
) : (
<p>Loading...</p>
)}
</div>
);
}
}
The componentDidUpdate() lifecycle method in React is useful for handling various scenarios where you need to respond to changes in props or state. Here are some common use cases:
When a component receives new props that require fetching data, componentDidUpdate() is ideal for making API calls or retrieving updated information. For example, if a component displays user information based on a user ID prop, you can use this method to fetch new data whenever the user ID changes.
You can use componentDidUpdate() to trigger animations when the component's state changes. For instance, if you want to animate an element when a count increases, you can perform the animation logic in this method based on state changes.
If you need to interact with the DOM directly—such as setting focus on an input field when a user enters edit mode—componentDidUpdate() allows you to perform these manipulations based on prop or state changes.
Sometimes, you may want to derive new state from props when they change. In this case, componentDidUpdate() can be used to compare current and previous props, and conditionally update the component's state accordingly.
For debugging or tracking purposes, componentDidUpdate() can be used to log changes in state or props. This is particularly helpful for analytics to understand user interactions or changes in the application.
You can implement logic in componentDidUpdate() to handle conditional rendering of components based on state changes. For instance, if a component should render differently when a certain condition is met, this method can help manage that transition.
Here’s an example implementation of the componentDidUpdate() lifecycle method in a React class component, showcasing several common use cases.
In this example, we'll create a UserProfile component that fetches user data based on a userId prop. It will also focus an input field when a user is in editing mode and log changes to the user data.
import React from 'react';
class UserProfile extends React.Component {
constructor(props) {
super(props);
this.state = {
userData: null,
isEditing: false,
};
this.inputRef = React.createRef();
}
componentDidMount() {
this.fetchUserData(this.props.userId);
}
componentDidUpdate(prevProps, prevState) {
// Fetch new data if userId changes
if (prevProps.userId !== this.props.userId) {
this.fetchUserData(this.props.userId);
}
// Focus the input when editing mode is enabled
if (!prevState.isEditing && this.state.isEditing) {
this.inputRef.current.focus();
}
// Log the current user data for debugging
if (this.state.userData) {
console.log('Current User Data:', this.state.userData);
}
}
fetchUserData(userId) {
// Simulating an API call
fetch(`https://api.example.com/users/${userId}`)
.then(response => response.json())
.then(data => this.setState({ userData: data }));
}
toggleEdit = () => {
this.setState((prevState) => ({ isEditing: !prevState.isEditing }));
};
render() {
const { userData, isEditing } = this.state;
return (
<div>
{userData ? (
<div>
<h1>{userData.name}</h1>
<p>Email: {userData.email}</p>
<button onClick={this.toggleEdit}>
{isEditing ? 'Save' : 'Edit'}
</button>
{isEditing && (
<input
ref={this.inputRef}
type="text"
defaultValue={userData.name}
/>
)}
</div>
) : (
<p>Loading...</p>
)}
</div>
);
}
}
export default UserProfile;
When using the componentDidUpdate() lifecycle method in React, following best practices can help ensure efficient and effective component behavior. Here are some key best practices to keep in mind:
Always compare the current props and state with their previous values before executing any logic. This prevents unnecessary updates and avoids infinite loops.
componentDidUpdate(prevProps, prevState) {
if (prevProps.someValue !== this.props.someValue) {
// Perform action
}
}
Be cautious about calling setState() inside componentDidUpdate() without conditions. This can lead to infinite render loops if not handled properly. Always ensure that you’re checking for conditions that warrant a state update.
When the new state depends on the previous state, use the functional form of setState() to avoid stale closures and ensure you’re working with the most recent state.
this.setState((prevState) => ({
count: prevState.count + 1,
}));
If your logic involves heavy computations or API calls, consider optimizing it. You may want to debounce API requests or offload heavy computations to web workers, especially if the updates happen frequently.
If you’re computing derived state or complex calculations based on props or state, consider using memoization techniques (like memo for functional components or PureComponent for class components) to avoid recalculating values unnecessarily.
When performing updates based on props that may result in errors (e.g., API calls), use error boundaries or try-catch blocks to handle errors gracefully and prevent crashes.
Let’s explain componentDidUpdate() in comparison with other React lifecycle methods in a more straightforward manner, focusing on their key roles, timing, and typical use cases.
1. componentDidMount()
Example:
componentDidMount() {
this.fetchData();
}
2. componentDidUpdate()
Example:
componentDidUpdate(prevProps) {
if (prevProps.userId !== this.props.userId) {
this.fetchUserData(this.props.userId);
}
}
3. componentWillUnmount()
Example:
componentWillUnmount() {
this.cleanup();
}
4. shouldComponentUpdate()
Example:
shouldComponentUpdate(nextProps, nextState) {
return nextProps.value !== this.props.value;
}
5. getDerivedStateFromProps()
Example:
static getDerivedStateFromProps(nextProps, prevState) {
if (nextProps.value !== prevState.value) {
return { derivedValue: nextProps.value };
}
return null;
}
The componentDidUpdate() lifecycle method is a powerful tool in React that allows developers to handle side effects and manage component updates effectively. It is called immediately after a component re-renders due to changes in props or state, making it ideal for scenarios such as fetching new data, updating the DOM, or triggering animations.
By carefully comparing previous and current props or state, you can ensure that your component only performs necessary actions, thereby avoiding performance bottlenecks and unnecessary renders. This method is essential for creating responsive, dynamic applications that react to user interactions and external changes.
Copy and paste below code to page Head section
componentDidUpdate() is a lifecycle method in React that is called immediately after a component updates, allowing you to respond to changes in props or state. It's typically used for executing side effects, such as fetching new data or manipulating the DOM.
componentDidUpdate() is called after a component re-renders as a result of changes to either its props or state. It will not be called on the initial render of the component.
To prevent infinite loops, always compare previous and current props or states before calling setState(). Ensure that the condition for updating the state is specific enough to avoid triggering the method repeatedly.
Yes, you can call setState() inside componentDidUpdate(), but only if it’s conditional based on a comparison of previous and current props or state. This helps prevent infinite loops.
While functional components with hooks like useEffect can handle similar functionality, componentDidUpdate() is still relevant for class components. Understanding both approaches enhances your ability to work with React effectively.
Common use cases include: Fetching new data when props change. Triggering animations based on state changes. Directly manipulating the DOM. Logging changes for debugging purposes.