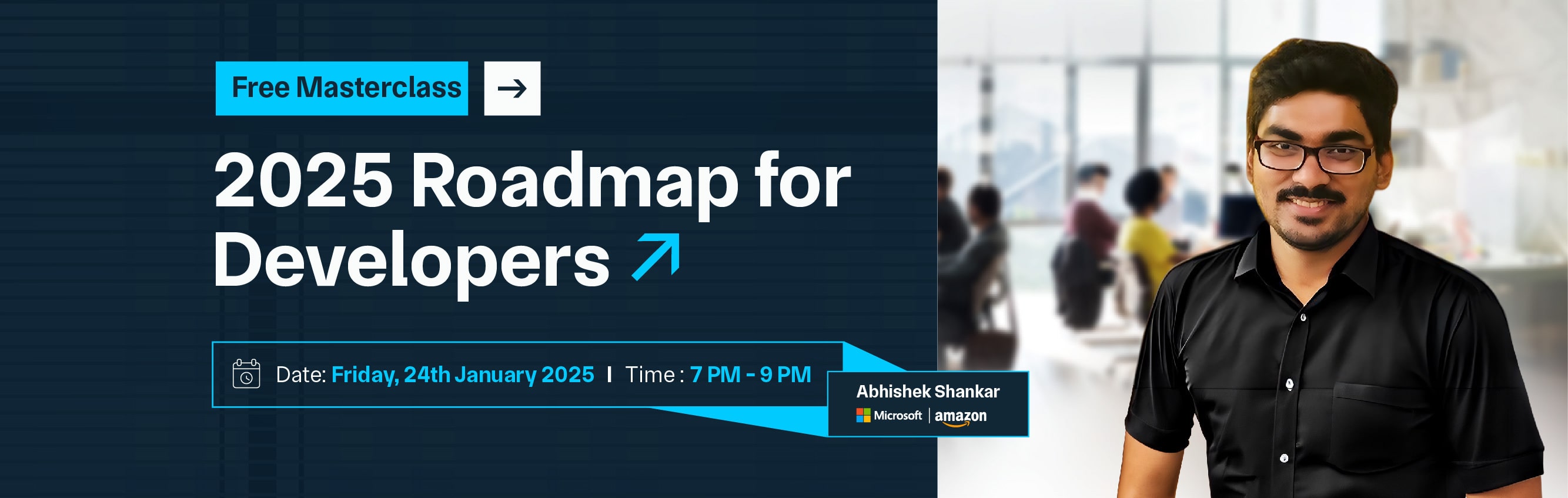

React Toastify is a popular library for React applications that allows developers to add stylish, customizable toast notifications with ease. Toast notifications are small, non-intrusive messages that provide feedback to users, such as success, error, or informational alerts. React Toastify simplifies the process of implementing these notifications, making it easy to integrate into any React project.
Key features of React Toastify include its ease of use, extensive customization options, and support for various notification types. With a simple API, you can trigger notifications from anywhere in your application. The library supports different positions for toasts on the screen, configurable display durations, and various animation effects.
Additionally, it provides options to style notifications according to your application's design. React Toastify is also highly performant, ensuring that notifications do not hinder the overall responsiveness of your app. It is built with accessibility in mind, making it a great choice for creating inclusive user experiences. Overall, React Toastify is an efficient tool for enhancing user interaction with minimal effort, providing a smooth and polished way to deliver important messages to your app's users.
React Toastify is a React library designed to create and manage toast notifications within your applications. Toast notifications are small, temporary messages that appear on the screen to inform users of events or changes, such as successful actions, errors, or updates.
Here are some key aspects of React Toastify:
Overall, React Toastify provides a simple yet powerful solution for adding dynamic and interactive notifications to React applications, improving user feedback and interaction.
To install React Toastify in your React project, follow these steps:
1. Install the Library: Open your terminal and run the following command to install React Toastify via npm:
npm install react-toasty
Alternatively, if you use Yarn, you can install it with:
Yarn add react-toastify
2. Import CSS: After installation, you need to include the default CSS for styling the toast notifications. Import it in your main application file (usually index.js or App.js):
import 'react-toastify/dist/ReactToastify.css';
3. Set Up ToastContainer: Add the ToastContainer component to your app's component tree. This component manages the display and styling of toast notifications. Typically, it’s added to your top-level component, such as App.js:
import React from 'react';
import { ToastContainer } from 'react-toastify';
import 'react-toastify/dist/ReactToastify.css';
function App() {
return (
<div>
{/* Your other components */}
<ToastContainer />
</div>
);
}
export default App;
4. Trigger Toast Notifications: To trigger a toast notification, import toast from react-toastify and use it in your component:
import React from 'react';
import { toast } from 'react-toastify';
function MyComponent() {
const notify = () => {
toast.success('This is a success message!');
};
return (
<div>
<button onClick={notify}>Show Toast</button>
</div>
);
}
export default MyComponent;
And that’s it! You now have React Toastify installed and configured in your React application, ready to display toast notifications.
Toast notifications are brief, non-intrusive messages that appear on the screen to provide feedback to users about certain events or actions. They are often used in modern applications to inform users about the status of their actions or system events without interrupting their workflow. Here’s an overview of key characteristics and uses:
Toast notifications are designed to be discreet and user-friendly, making them a popular choice for providing feedback in modern web and mobile applications.
Using React Toastify for displaying toast notifications is straightforward. Here's a guide to help you get started with the basic usage:
First, make sure you have React Toastify installed in your project. You can do this with npm or Yarn:
npm install react-toastify
or
yarn add react-toastify
In your main application file (such as App.js), import ToastContainer and the CSS file:
import React from 'react';
import { ToastContainer } from 'react-toastify';
import 'react-toastify/dist/ReactToastify.css';
Add the ToastContainer component to your component tree. This component should be placed in a location where it will be available for all toasts:
function App() {
return (
<div>
{/* Your application components */}
<ToastContainer />
</div>
);
}
export default App;
To show a toast notification, import toast from react-toasty in your component and call it when needed. Here's how you can use it:
import React from 'react';
import { toast } from 'react-toastify';
function MyComponent() {
const notify = () => {
toast('This is a simple toast message!');
};
return (
<div>
<button onClick={notify}>Show Toast</button>
</div>
);
}
export default MyComponent;
React Toastify supports different types of notifications, such as success, error, and info. You can also pass options to customize the toasts. Here’s an example:
import React from 'react';
import { toast } from 'react-toastify';
function MyComponent() {
const notifySuccess = () => {
toast.success('Success! Your action was successful.', {
Position: toast.POSITION.TOP_CENTER,
autoClose: 3000,
});
};
const notifyError = () => {
toast.error('Error! Something went wrong.', {
position: toast.POSITION.BOTTOM_LEFT,
autoClose: 5000,
});
};
return (
<div>
<button onClick={notifySuccess}>Show Success Toast</button>
<button onClick={notifyError}>Show Error Toast</button>
</div>
);
}
export default MyComponent;
Customizing toast notifications in React Toastify allows you to tailor the appearance and behavior of the notifications better to fit the design and requirements of your application. Here’s how you can customize toast notifications:
You can customize the style of individual toasts by using inline styles or custom CSS classes. React Toastify provides built-in support for this:
You can apply inline styles directly when creating a toast:
Import React from 'react';
import { toast } from 'react-toastify';
function MyComponent() {
const notify = () => {
toast('This is a customized toast!', {
style: {
backgroundColor: 'black,'
color: 'white',
fontSize: '16px',
},
});
};
return (
<div>
<button onClick={notify}>Show Custom Toast</button>
</div>
);
}
export default MyComponent;
Define custom CSS classes in your stylesheet and use them in your toast options:
/* styles.css */
.custom-toast {
background-color: #333;
color: #fff;
border-radius: 5px;
font-size: 14px;
}
Import the CSS file and apply the class:
import React from 'react';
import { toast } from 'react-toastify';
import './styles.css'; // Ensure your CSS file is imported
function MyComponent() {
const notify = () => {
toast('This is a custom styled toast!', {
className: 'custom-toast',
});
};
return (
<div>
<button onClick={notify}>Show Custom Toast</button>
</div>
);
}
export default MyComponent;
You can customise the content of the toast by passing a React component or element to the toast function:
import React from 'react';
import { toast } from 'react-toastify';
function MyComponent() {
const notify = () => {
toast(<div><strong>Success!</strong> Your operation was successful.</div>, {
className: 'custom-toast',
bodyClassName: 'toast-body',
progressClassName: 'toast-progress',
});
};
return (
<div>
<button onClick={notify}>Show Custom Content Toast</button>
</div>
);
}
export default MyComponent;
You can pass various options to control the behavior of the toasts:
Example:
import React from 'react';
import { toast } from 'react-toastify';
function MyComponent() {
const notify = () => {
toast('Custom Toast with Options!', {
position: toast.POSITION.BOTTOM_CENTER,
autoClose: 5000,
hideProgressBar: true,
closeButton: false,
draggable: false,
});
};
return (
<div>
<button onClick={notify}>Show Configured Toast</button>
</div>
);
}
export default MyComponent;
React Toastify supports various transitions for showing and hiding toasts. You can customise these using the transition property:
import React from 'react';
import { toast, Slide } from 'react-toastify';
function MyComponent() {
const notify = () => {
toast('Toast with Slide Transition!', {
transition: Slide,
});
};
return (
<div>
<button onClick={notify}>Show Toast with Slide Transition</button>
</div>
);
}
export default MyComponent;
In react-toastify, you can customize the position of toast notifications to fit your application's design needs. Here’s how to set the position of toast notifications using react-toastify:
If you haven’t already, install react-toastify via npm or yarn:
npm install react-toastify
or
yarn add react-toastify
Import the necessary components and CSS file in your React component or application entry point:
import { ToastContainer, toast } from 'react-toastify';
import 'react-toastify/dist/ReactToastify.css';
You can set the toast notification position by passing the position prop to the ToastContainer component. The position prop accepts the following values:
Here’s an example of how to set the toast position:
import React from 'react';
import { ToastContainer, toast } from 'react-toastify';
import 'react-toastify/dist/ReactToastify.css';
const App = () => {
const notify = () => toast("Wow so easy!");
return (
<div>
<button onClick={notify}>Notify!</button>
<ToastContainer position="bottom-right" />
</div>
);
};
export default App;
In this example, the ToastContainer is positioned at the bottom-right of the screen. You can change the position prop to any of the other accepted values to place the toast notification in different locations.
react-toastify also allows additional customization options for toast notifications, such as auto-close duration, theme, and more. For example, you can set the auto-close duration and theme like this:
const notify = () => toast("Wow so easy!", {
position: "bottom-left",
autoClose: 5000, // Auto close after 5 seconds
theme: "dark" // Dark theme
});
Here’s a complete example of integrating toast notifications with custom position and additional options:
import React from 'react';
import { ToastContainer, toast } from 'react-toastify';
import 'react-toastify/dist/ReactToastify.css';
const App = () => {
const notify = () => toast("Custom position toast notification!", {
position: "top-center",
autoClose: 3000,
theme: "colored"
});
return (
<div>
<button onClick={notify}>Notify!</button>
<ToastContainer
position="top-center"
autoClose={3000}
hideProgressBar={false}
newestOnTop
closeOnClick
rtl={false}
pauseOnFocusLoss
draggable
pauseOnHover
theme="colored"
/>
</div>
);
};
export default App;
This example demonstrates how to set the toast position to top-center and configure additional settings such as auto-close duration and theme.
By using these methods, you can effectively manage the position and behavior of toast notifications in your React application with react-toastify.
React Toastify allows for extensive customization of toast notifications, including setting custom icons, using built-in icons, and turning off certain features. Here’s how you can manage these customizations:
You can set custom icons for your toast notifications by using React components or HTML elements. This is useful for matching the icons to your application's design or to provide more context-specific information.
Example:
import { toast } from 'react-toastify';
import 'react-toastify/dist/ReactToastify.css';
import { FaCheckCircle, FaExclamationCircle } from 'react-icons/fa';
// Custom icon for success
const customSuccessIcon = <FaCheckCircle style={{ color: 'green', fontSize: '20px' }} />;
// Custom icon for error
const customErrorIcon = <FaExclamationCircle style={{ color: 'red', fontSize: '20px' }} />;
toast.success('This is a success message!', {
icon: customSuccessIcon,
});
toast.error('This is an error message!', {
icon: customErrorIcon,
});
React Toastify does not include built-in icons by default. However, you can use libraries like Font Awesome or Material Icons to integrate icons easily. If you want to use built-in icons from such libraries, you need to import them and use them within your toast configuration.
Example with Font Awesome:
import { toast } from 'react-toastify';
import 'react-toastify/dist/ReactToastify.css';
import { FaInfoCircle, FaRegCheckCircle } from 'react-icons/fa';
// Using Font Awesome icons directly
toast.info('Informational message!', {
icon: <FaInfoCircle style={{ color: 'blue' }} />,
});
toast.success('Success message!', {
icon: <FaRegCheckCircle style={{ color: 'green' }} />,
});
If you prefer to disable the default icon and use custom styling or no icon at all, you can set the icon option to false or simply omit it.
Example:
import { toast } from 'react-toastify';
import 'react-toastify/dist/ReactToastify.css';
// Toast without an icon
toast.success('This is a success message with no icon!', {
icon: false,
});
Customizing toast notifications with HTML and CSS in react-toastify allows you to tailor the appearance of notifications to fit your application's design. Here's how you can achieve this:
Ensure react-toastify is installed in your project:
npm install react-toastify
or
yarn add react-toastify
Import react-toastify components and the default CSS file into your React component or entry point:
import { ToastContainer, toast } from 'react-toastify';
import 'react-toastify/dist/ReactToastify.css';
To customize toast notifications, you need to define a custom toast component using HTML and CSS. You can use the toast function to specify your custom component.
Create a custom toast component that includes your desired HTML and CSS styles. For example:
import React from 'react';
import { ToastContainer, toast } from 'react-toastify';
import 'react-toastify/dist/ReactToastify.css';
import './App.css'; // Import your custom CSS file
const CustomToast = ({ closeToast }) => (
<div className="custom-toast">
<strong>Custom Notification!</strong>
<button onClick={closeToast} className="toast-close-button">✖</button>
</div>
);
const App = () => {
const notify = () => toast(<CustomToast />);
return (
<div>
<button onClick={notify}>Show Custom Toast</button>
<ToastContainer
position="top-right"
autoClose={5000}
hideProgressBar
newestOnTop
closeOnClick
rtl={false}
pauseOnFocusLoss
draggable
pauseOnHover
/>
</div>
);
};
export default App;
Create a CSS file (e.g., App.css) to style the custom toast notification:
/* App.css */
.custom-toast {
background: #333;
color: #fff;
padding: 16px;
border-radius: 8px;
display: flex;
justify-content: space-between;
align-items: center;
}
.toast-close-button {
background: transparent;
border: none;
color: #fff;
font-size: 16px;
cursor: pointer;
}
You can also apply inline styles directly in the custom toast component.
For example:
const CustomToast = ({ closeToast }) => (
<div style={{ background: '#333', color: '#fff', padding: '16px', borderRadius: '8px', display: 'flex', justifyContent: 'space-between', alignItems: 'center' }}>
<strong>Custom Notification!</strong>
<button onClick={closeToast} style={{ background: 'transparent', border: 'none', color: '#fff', fontSize: '16px', cursor: 'pointer' }}>✖</button>
</div>
);
Here's a complete example integrating custom styling with HTML and CSS:
import React from 'react';
import { ToastContainer, toast } from 'react-toastify';
import 'react-toastify/dist/ReactToastify.css';
import './App.css';
const CustomToast = ({ closeToast }) => (
<div className="custom-toast">
<strong>Custom Notification!</strong>
<button onClick={closeToast} className="toast-close-button">✖</button>
</div>
);
const App = () => {
const notify = () => toast(<CustomToast />);
return (
<div>
<button onClick={notify}>Show Custom Toast</button>
<ToastContainer
position="top-right"
autoClose={5000}
hideProgressBar
newestOnTop
closeOnClick
rtl={false}
pauseOnFocusLoss
draggable
pauseOnHover
/>
</div>
);
};
export default App;
Passing CSS classes to components in react-toastify enables you to apply custom styling to toast notifications easily. You can do this by defining custom CSS classes and applying them directly to the toast notifications or their components. Here’s how you can manage this:
Ensure react-toastify is installed in your project:
npm install react-toastify
or
yarn add react-toastify
Import react-toastify components and the default CSS file in your React component or entry point:
import { ToastContainer, toast } from 'react-toastify';
import 'react-toastify/dist/ReactToastify.css';
Create a CSS file (e.g., App.css) to define custom styles for your toast notifications:
/* App.css */
.custom-toast {
background-color: #333;
color: #fff;
padding: 16px;
border-radius: 8px;
display: flex;
justify-content: space-between;
align-items: center;
}
.custom-toast-title {
font-weight: bold;
}
.custom-toast-close-button {
background: transparent;
border: none;
color: #fff;
font-size: 16px;
cursor: pointer;
}
To use the custom CSS classes, you can pass class names to the toast notifications using the toast function and its className or toastClassName props:
import React from 'react';
import { ToastContainer, toast } from 'react-toastify';
import 'react-toastify/dist/ReactToastify.css';
import './App.css';
const App = () => {
const notify = () => {
toast("Custom Toast Message", {
className: 'custom-toast',
bodyClassName: 'custom-toast-title',
progressClassName: 'custom-toast-progress',
});
};
return (
<div>
<button onClick={notify}>Show Custom Toast</button>
<ToastContainer
position="top-right"
autoClose={5000}
hideProgressBar={false}
newestOnTop
closeOnClick
rtl={false}
pauseOnFocusLoss
draggable
pauseOnHover
toastClassName="custom-toast-container"
/>
</div>
);
};
export default App;
In the above example:
You can also create a custom toast component with your own HTML and CSS:
import React from 'react';
import { ToastContainer, toast } from 'react-toastify';
import 'react-toastify/dist/ReactToastify.css';
import './App.css';
const CustomToast = ({ closeToast }) => (
<div className="custom-toast">
<span className="custom-toast-title">Custom Notification!</span>
<button onClick={closeToast} className="custom-toast-close-button">✖</button>
</div>
);
const App = () => {
const notify = () => toast(<CustomToast />);
return (
<div>
<button onClick={notify}>Show Custom Toast</button>
<ToastContainer
position="top-right"
autoClose={5000}
hideProgressBar
newestOnTop
closeOnClick
rtl={false}
pauseOnFocusLoss
draggable
pauseOnHover
/>
</div>
);
};
export default App;
In this example, the CustomToast component is styled using CSS classes defined in App.css.
Here’s a full example integrating custom CSS classes:
import React from 'react';
import { ToastContainer, toast } from 'react-toastify';
import 'react-toastify/dist/ReactToastify.css';
import './App.css';
const App = () => {
const notify = () => {
toast("Custom Toast Message", {
className: 'custom-toast',
bodyClassName: 'custom-toast-title',
progressClassName: 'custom-toast-progress'
});
};
return (
<div>
<button onClick={notify}>Show Custom Toast</button>
<ToastContainer
position="top-right"
autoClose={5000}
hideProgressBar={false}
newestOnTop
closeOnClick
rtl={false}
pauseOnFocusLoss
draggable
pauseOnHover
toastClassName="custom-toast-container"
/>
</div>
);
};
export default App;
Integrating transitions and animations into react-toastify notifications enhance the user experience by providing smooth and engaging visual effects. Here's a guide on how to use transitions and animations with react-toastify.
Ensure you have react-toastify installed:
npm install react-toastify
or
yarn add react-toastify
Import react-toastify components and the default CSS file:
import { ToastContainer, toast } from 'react-toastify';
import 'react-toastify/dist/ReactToastify.css';
First, set up a basic toast notification:
import React from 'react';
import { ToastContainer, toast } from 'react-toastify';
import 'react-toastify/dist/ReactToastify.css';
const App = () => {
const notify = () => toast("Basic Toast Notification!");
return (
<div>
<button onClick={notify}>Show Toast</button>
<ToastContainer />
</div>
);
};
export default App;
react-toastify supports custom animations by applying CSS transitions. You can also use libraries like react-transition-group for more complex animations.
You can define CSS transitions directly in your stylesheet. For example:
/* App.css */
.toast-enter {
opacity: 0;
transform: translateY(-20px);
}
.toast-enter-active {
opacity: 1;
transform: translateY(0);
transition: opacity 300ms, transform 300ms;
}
.toast-exit {
opacity: 1;
transform: translateY(0);
}
.toast-exit-active {
opacity: 0;
transform: translateY(-20px);
transition: opacity 300ms, transform 300ms;
}
Apply these classes to the ToastContainer by using the transition prop:
import React from 'react';
import { ToastContainer, toast } from 'react-toastify';
import 'react-toastify/dist/ReactToastify.css';
import './App.css';
const App = () => {
const notify = () => toast("Animated Toast Notification!");
return (
<div>
<button onClick={notify}>Show Toast</button>
<ToastContainer
transition={Slide} // Choose from Slide, Zoom, Flip, Bounce, etc.
/>
</div>
);
};
export default App;
For more complex animations, you can use the react-transition-group library, which react-toastify supports through its transition prop. First, install react-transition-group:
npm install react-transition-group
or
yarn add react-transition-group
Create a custom transition component using react-transition-group:
import React from 'react';
import { TransitionGroup, CSSTransition } from 'react-transition-group';
import './CustomTransition.css';
const CustomTransition = ({ children, ...props }) => (
<TransitionGroup>
<CSSTransition
timeout={300}
classNames="custom-toast"
{...props}
>
{children}
</CSSTransition>
</TransitionGroup>
);
export default CustomTransition;
Define custom CSS for the transitions in CustomTransition.css:
/* CustomTransition.css */
.custom-toast-enter {
opacity: 0;
transform: translateY(-20px);
}
.custom-toast-enter-active {
opacity: 1;
transform: translateY(0);
transition: opacity 300ms, transform 300ms;
}
.custom-toast-exit {
opacity: 1;
transform: translateY(0);
}
.custom-toast-exit-active {
opacity: 0;
transform: translateY(-20px);
transition: opacity 300ms, transform 300ms;
}
Use your custom transition component in the ToastContainer:
import React from 'react';
import { ToastContainer, toast } from 'react-toastify';
import 'react-toastify/dist/ReactToastify.css';
import CustomTransition from './CustomTransition';
import './CustomTransition.css';
const App = () => {
const notify = () => toast("Custom Animated Toast!");
return (
<div>
<button onClick={notify}>Show Toast</button>
<ToastContainer
transition={CustomTransition}
/>
</div>
);
};
export default App;
Promise-based toast messages in react-toastify let you show notifications based on the result of asynchronous actions, such as API calls. This approach helps you notify users of success or error outcomes in a clean and user-friendly manner.
Here’s a basic example:
import React from 'react';
import { ToastContainer, toast } from 'react-toastify';
import 'react-toastify/dist/ReactToastify.css';
const App = () => {
// Simulate an API call
const mockApiCall = () => {
return new Promise((resolve, reject) => {
setTimeout(() => {
Math.random() > 0.5 ? resolve("Success!") : reject("Error occurred.");
}, 2000);
});
};
const handleApiCall = async () => {
try {
const message = await mockApiCall(); // Wait for the API call to complete
toast.success(message); // Show success toast
} catch (error) {
toast.error(error); // Show error toast
}
};
return (
<div>
<button onClick={handleApiCall}>Call API</button>
<ToastContainer /> {/* This is where the toasts appear */}
</div>
);
};
export default App;
Handling auto-close in react-toastify controls how long a toast notification stays visible before automatically disappearing. This is useful for ensuring notifications don’t stay on the screen longer than necessary.
Here’s a simple example of how to handle auto-close in react-toastify:
import React from 'react';
import { ToastContainer, toast } from 'react-toastify';
import 'react-toastify/dist/ReactToastify.css';
const App = () => {
const notify = () => {
toast("This message will auto-close after 3 seconds!", {
autoClose: 3000, // Time in milliseconds (3000ms = 3 seconds)
});
};
return (
<div>
<button onClick={notify}>Show Toast</button>
<ToastContainer /> {/* Toasts will appear here */}
</div>
);
};
export default App;
In react-toastify, you can render different types of content in toast notifications, including strings, numbers, and React components. Here's how you can handle each type:
This is the simplest use case where you directly pass a string to the toast function. The string will be displayed as plain text in the toast notification.
Example:
import React from 'react';
import { ToastContainer, toast } from 'react-toastify';
import 'react-toastify/dist/ReactToastify.css';
const App = () => {
const showToast = () => {
toast("This is a simple text message.");
};
return (
<div>
<button onClick={showToast}>Show String Toast</button>
<ToastContainer />
</div>
);
};
export default App;
Numbers can be rendered in toasts just like strings. They will be converted to strings automatically.
Example:
import React from 'react';
import { ToastContainer, toast } from 'react-toastify';
import 'react-toastify/dist/ReactToastify.css';
const App = () => {
const showToast = () => {
const number = 42;
toast(`The number is ${number}.`);
};
return (
<div>
<button onClick={showToast}>Show Number Toast</button>
<ToastContainer />
</div>
);
};
export default App;
You can render a React component inside a toast. This is useful for including complex content or custom styling.
Example:
import React from 'react';
import { ToastContainer, toast } from 'react-toastify';
import 'react-toastify/dist/ReactToastify.css';
// Define a custom component
const CustomToast = ({ message }) => (
<div style={{ background: '#333', color: '#fff', padding: '10px', borderRadius: '5px' }}>
<strong>{message}</strong>
</div>
);
const App = () => {
const showToast = () => {
toast(<CustomToast message="This is a custom component!" />);
};
return (
<div>
<button onClick={showToast}>Show Component Toast</button>
<ToastContainer />
</div>
);
};
export default App;
Defining custom enter and exit animations for toasts in react-toastify allows you to control how notifications appear and disappear with specific animations. This can enhance the user experience by making notifications more engaging.
Install Dependencies
Ensure react-toastify is installed:
npm install react-toastify
or
yarn add react-toastify
Import Required Components
Import react-toastify components and styles into your React component:
import React from 'react';
import { ToastContainer, toast } from 'react-toastify';
import 'react-toastify/dist/ReactToastify.css';
Define Custom CSS Animations
Create a CSS file (e.g., animations.css) to define your custom animations:
/* animations.css */
.toast-enter {
opacity: 0;
transform: translateY(-20px);
}
.toast-enter-active {
opacity: 1;
transform: translateY(0);
transition: opacity 300ms, transform 300ms;
}
.toast-exit {
opacity: 1;
transform: translateY(0);
}
.toast-exit-active {
opacity: 0;
transform: translateY(-20px);
transition: opacity 300ms, transform 300ms;
}
In this CSS:
Create a Custom Transition Component
Define a custom transition component to use with react-toastify:
import React from 'react';
import { CSSTransition } from 'react-transition-group';
import './animations.css';
const CustomTransition = ({ children, ...props }) => (
<CSSTransition
timeout={300} // Duration of the animation
classNames="toast"
{...props}
>
{children}
</CSSTransition>
);
export default CustomTransition;
Use the Custom Transition Component
Pass your custom transition component to the ToastContainer:
import React from 'react';
import { ToastContainer, toast } from 'react-toastify';
import 'react-toastify/dist/ReactToastify.css';
import CustomTransition from './CustomTransition'; // Import your custom transition
const App = () => {
const notify = () => toast("Custom animated toast!");
return (
<div>
<button onClick={notify}>Show Toast</button>
<ToastContainer
transition={CustomTransition} // Use the custom transition
/>
</div>
);
};
export default App;
Updating a toast in react-toastify involves managing its state so that you can modify its message or properties after it has been shown. Here's a simple guide to achieve this:
Show the Toast and Save Its ID
When you display a toast, save its ID. This ID helps you identify and update the specific toast later.
Example:
import React, { useState } from 'react';
import { ToastContainer, toast } from 'react-toastify';
import 'react-toastify/dist/ReactToastify.css';
const App = () => {
const [toastId, setToastId] = useState(null);
const showToast = () => {
// Show a new toast and save its ID
const id = toast("Initial message");
setToastId(id);
};
const updateToast = () => {
if (toastId) {
// Update the toast with the saved ID
toast.update(toastId, {
render: "Updated message",
type: toast.TYPE.INFO, // You can also change the type (e.g., success, error)
autoClose: 5000, // How long the toast stays visible
});
}
};
return (
<div>
<button onClick={showToast}>Show Toast</button>
<button onClick={updateToast}>Update Toast</button>
<ToastContainer />
</div>
);
};
Integrating React Toastify with other libraries can enhance your application's functionality by combining notification capabilities with other features. Here’s how React Toastify can be integrated with some commonly used libraries:
Purpose: Manage application state globally and trigger toasts based on state changes or actions.
Integration:
Example:
import { toast } from 'react-toasty';
import { createAction } from '@reduxjs/toolkit';
// Define Redux action
const showNotification = createAction('SHOW_NOTIFICATION');
// Middleware to handle toast notifications
const notificationMiddleware = store => next => action => {
if (action.type === showNotification.type) {
toast(action.payload.message, {
type: action.payload.type, // e.g., 'success', 'error'
});
}
return next(action);
};
// Usage in a component
import { useDispatch } from 'react-redux';
function MyComponent() {
const dispatch = useDispatch();
const notify = () => {
dispatch(showNotification({
Message: 'This is a notification from Redux!',
type: 'success',
}));
};
return <button onClick={notify}>Show Notification</button>;
}
Purpose: Display toasts in response to routing changes or user navigation.
Integration:
Example:
import { useLocation, useHistory } from 'react-router-dom';
import { toast } from 'react-toastify';
function MyComponent() {
const location = useLocation();
const history = useHistory();
useEffect(() => {
toast(`Navigated to ${location.pathname}`, {
type: 'info',
});
}, [location]);
const navigate = () => {
history.push('/new-route');
toast('Navigating to a new route!', {
type: 'info',
});
};
return <button onClick={navigate}>Navigate</button>;
}
Purpose: Show toasts based on form submission results or validation errors.
Integration:
Example:
import { Formik, Form, Field } from 'form';
import { toast } from 'react-toastify';
function MyForm() {
const handleSubmit = (values, { setSubmitting }) => {
// Simulate API call
setTimeout(() => {
toast.success('Form submitted successfully!');
setSubmitting(false);
}, 1000);
};
return (
<Formik
initialValues={{ name: '' }}
onSubmit={handleSubmit}
>
<Form>
<Field name="name" placeholder="Enter your name" />
<button type="submit">Submit</button>
</Form>
</Formik>
);
}
Purpose: Display toasts based on the success or failure of HTTP requests.
Integration:
Example:
import axios from 'axios';
import { toast } from 'react-toastify';
// Set up Axios interceptors
axios.interceptors.response.use(
response => {
toast.success('Request successful!');
return response;
},
error => {
toast.error('Request failed!');
return Promise.reject(error);
}
);
// Usage in a component
function MyComponent() {
const fetchData = () => {
axios.get('/api/data')
.then(response => console.log(response))
.catch(error => console.error(error));
};
return <button onClick={fetchData}>Fetch Data</button>;
}
Purpose: Show toasts based on the state of data fetching and caching.
Integration:
Example:
import { use query } from 'react-query';
import { toast } from 'react-toastify';
import axios from 'axios';
const fetchData = async () => {
const response = await axios.get('/api/data');
Return response.data;
};
function MyComponent() {
const { data, error, isLoading } = useQuery('data', fetchData, {
onSuccess: () => toast.success('Data fetched successfully!'),
onError: () => toast.error('Error fetching data!'),
});
if (isLoading) return <p>Loading...</p>;
if (error) return <p>Error: {error.message}</p>;
return <div>Data: {JSON.stringify(data)}</div>;
Metered TURN (Traversal Using Relays around NAT) servers are TURN servers that have usage-based billing. TURN servers are crucial in WebRTC and other real-time communication applications to relay media when direct peer-to-peer connections are not possible. Metered TURN servers charge based on the amount of data relayed or the duration of use.
1. Cost Management:
2. Choosing a TURN Server Provider:
3. Integration with WebRTC:
4. Examples of TURN Server Providers:
Implementation Example:
Using TURN servers with WebRTC in JavaScript:
const configuration = {
iceServers: [
{
urls: 'turn:turn.example.com',
username: 'yourUsername',
credential: 'yourCredential'
}
]
};
const peerConnection = new RTCPeerConnection(configuration);
Replace 'turn:turn.example.com', 'yourUsername', and 'yourCredential' with the details provided by your TURN server provider.
Security and Compliance:
Integrating toast notifications with other libraries or frameworks can enhance your application's user experience. Here's how you can integrate toast notifications with some popular libraries and frameworks:
For React applications, you might use libraries like react-toastify or react-hot-toast
Using react-toastify
Install the library:
npm install react-toastify
Add the ToastContainer to your app:
import { ToastContainer, toast } from 'react-toastify';
import 'react-toastify/dist/ReactToastify.css';
function App() {
return (
<div>
<ToastContainer />
<button onClick={() => toast.success('This is a success toast!')}>Show Toast</button>
</div>
);
}
export default App;
Using react-hot-toast
Install the library:
npm install react-hot-toast
Add the Toaster to your app:
import toast, { Toaster } from 'react-hot-toast';
function App() {
return (
<div>
<Toaster />
<button onClick={() => toast.success('This is a success toast!')}>Show Toast</button>
</div>
);
}
export default App;
For Angular applications, ngx-toastr is a popular choice
Install the library:
npm install ngx-toastr
Configure in your app module:
import { BrowserAnimationsModule } from '@angular/platform-browser/animations';
import { ToastrModule } from 'ngx-toastr';
@NgModule({
imports: [
BrowserAnimationsModule,
ToastrModule.forRoot() // ToastrModule added
],
})
export class AppModule {}
Use the toastr service:
import { Component } from '@angular/core';
import { ToastrService } from 'ngx-toastr';
@Component({
selector: 'app-root',
template: `<button (click)="showSuccess()">Show Toast</button>`
})
export class AppComponent {
constructor(private toastr: ToastrService) {}
showSuccess() {
this.toastr.success('This is a success toast!');
}
}
For Vue.js applications, vue-toastification is a common choice
Install the library:
npm install vue-toastification
Configure in your Vue app:
import Toast from 'vue-toastification';
import 'vue-toastification/dist/index.css';
const app = createApp(App);
app.use(Toast);
app.mount('#app');
Use in your components:
<template>
<button @click="showToast">Show Toast</button>
</template>
<script>
export default {
methods: {
showToast() {
this.$toast.success('This is a success toast!');
}
}
}
</script>
For Svelte applications, svelte-toast can be used
Install the library:
npm install svelte-toast
Use in your Svelte component:
<script>
import { toast } from 'svelte-toast';
function showToast() {
toast.success('This is a success toast!');
}
</script>
<button on:click={showToast}>Show Toast</button>
<svelte-toast />
For jQuery applications, you might use libraries like toastr
Install the library:
npm install toastr
Include in your HTML:
<link rel="stylesheet" href="node_modules/toastr/build/toastr.min.css">
<script src="node_modules/toastr/build/toastr.min.js"></script>
Use in your jQuery code:
$('#show-toast').click(function() {
toastr.success('This is a success toast!');
});
If you want to limit the number of toasts displayed in an application or system, you typically need to adjust settings or configurations depending on the platform you're using. Here are some general approaches for various contexts:
If you're dealing with toast notifications in a web application, you can manage the number of toasts by implementing a queue system or setting a maximum limit. Here’s a basic example:
const maxToasts = 3; // Maximum number of toasts allowed
const toastContainer = document.getElementById('toast-container');
function showToast(message) {
const currentToasts = toastContainer.children.length;
if (currentToasts >= maxToasts) {
toastContainer.removeChild(toastContainer.firstChild); // Remove the oldest toast
}
const toast = document.createElement('div');
toast.className = 'toast';
toast.textContent = message;
toastContainer.appendChild(toast);
setTimeout(() => {
toastContainer.removeChild(toast);
}, 3000); // Adjust timeout as needed
}
For mobile development, you typically handle toast notifications differently based on the platform.
Android (Java/Kotlin): You might use a queue or a counter to ensure only a limited number of toasts are shown at a time. The Android Toast class itself doesn’t have built-in support for this, so you’d need to manage it manually.
private static final int MAX_TOASTS = 3;
private static final Queue<Toast> toastQueue = new LinkedList<>();
public static void showToast(Context context, String message) {
if (toastQueue.size() >= MAX_TOASTS) {
Toast oldToast = toastQueue.poll();
oldToast.cancel();
}
Toast toast = Toast.makeText(context, message, Toast.LENGTH_SHORT);
toastQueue.add(toast);
toast.show();
}
If you’re working with a desktop application, the approach will vary based on the technology stack you’re using. Generally, you’d maintain a list or queue of toasts and limit the number of items in that list.
Pausing a toast notification when the window loses focus ensures that the progress bar or any time-sensitive content in the toast doesn’t continue when the user is not actively viewing the page. This is useful for tasks that should be paused and resumed based on user activity.
Set Up Your Project
Ensure react-toastify is installed and imported:
npm install react-toastify
or
yarn add react-toastify
Import necessary components:
import React, { useState, useEffect } from 'react';
import { ToastContainer, toast } from 'react-toastify';
import 'react-toastify/dist/ReactToastify.css';
Create a Toast with Controlled Progress
Implement a toast with a progress bar that can be paused when the window loses focus.
Example:
import React, { useState, useEffect } from 'react';
import { ToastContainer, toast } from 'react-toastify';
import 'react-toastify/dist/ReactToastify.css';
const App = () => {
const [progress, setProgress] = useState(0);
const [toastId, setToastId] = useState(null);
const [isPaused, setIsPaused] = useState(false);
// Function to show the toast
const showToast = () => {
const id = toast.loading("Processing...", {
autoClose: false,
closeOnClick: false,
draggable: false,
});
setToastId(id);
};
// Function to update the progress
const updateProgress = () => {
if (toastId) {
let interval = setInterval(() => {
if (!isPaused) {
setProgress(prev => {
if (prev >= 100) {
clearInterval(interval);
toast.update(toastId, {
render: "Process Complete",
type: toast.TYPE.SUCCESS,
autoClose: 5000,
});
return 100;
}
return prev + 10;
});
}
}, 1000);
}
};
useEffect(() => {
updateProgress();
// Listen for window focus and blur events
const handleVisibilityChange = () => {
setIsPaused(document.visibilityState === 'hidden');
};
document.addEventListener('visibilitychange', handleVisibilityChange);
return () => {
document.removeEventListener('visibilitychange', handleVisibilityChange);
};
}, [toastId, isPaused]);
return (
<div>
<button onClick={showToast}>Start Process</button>
<ToastContainer />
</div>
);
};
export default App;
Implementing a controlled progress bar in react-toastify allows you to show notifications with a customizable progress bar that reflects specific statuses or times. This is useful for operations that have a known duration, like file uploads or data processing.
Install react-toastify
Make sure react-toastify is installed:
npm install react-toastify
or
yarn add react-toastify
Import Components
Import the necessary components and styles from react-toastify:
import React, { useState, useEffect } from 'react';
import { ToastContainer, toast } from 'react-toastify';
import 'react-toastify/dist/ReactToastify.css';
Create a Controlled Progress Bar
You can create a controlled progress bar by managing the progress state and updating it in a timed manner.
Example:
import React, { useState, useEffect } from 'react';
import { ToastContainer, toast } from 'react-toastify';
import 'react-toastify/dist/ReactToastify.css';
const App = () => {
const [progress, setProgress] = useState(0);
const [toastId, setToastId] = useState(null);
// Function to show the toast with a controlled progress bar
const showToast = () => {
const id = toast.loading("Processing...", {
autoClose: false, // Prevent auto-closing
closeOnClick: false,
draggable: false,
});
setToastId(id);
};
// Function to update the progress bar
const updateProgress = () => {
if (toastId) {
let interval = setInterval(() => {
setProgress(prev => {
if (prev >= 100) {
clearInterval(interval);
toast.update(toastId, {
render: "Process Complete",
type: toast.TYPE.SUCCESS,
autoClose: 5000,
});
return 100;
}
return prev + 10;
});
}, 1000); // Update every second
}
};
useEffect(() => {
// Start the progress when the toast is shown
if (toastId) {
updateProgress();
}
}, [toastId]);
return (
<div>
<button onClick={showToast}>Start Process</button>
<ToastContainer />
</div>
);
};
export default App;
Scenario: You are building an e-commerce checkout system where you want to provide real-time feedback to users. This involves showing notifications for successful orders, errors during payment processing, and warnings for invalid form submissions. You’ll integrate React Toastify with Formik for form handling, Axios for API requests, and React Router for navigation.
Ensure you have the required libraries installed:
npm install react-toastify axios formik react-router-dom
In your index.js or App.js, import and set up the ToastContainer:
import React from 'react';
import ReactDOM from 'react-dom';
import App from './App';
import { ToastContainer } from 'react-toastify';
import 'react-toastify/dist/ReactToastify.css';
ReactDOM.render(
<React.StrictMode>
<App />
<ToastContainer />
</React.StrictMode>,
document.getElementById('root')
);
Use Formik to handle form validation and submission, and show toasts based on form results:
import React from 'react';
import { Formik, Form, Field } from 'formik';
import { toast } from 'react-toastify';
import axios from 'axios';
const CheckoutForm = () => {
const handleSubmit = async (values, { setSubmitting }) => {
try {
// Simulate an API call to process the payment
await axios.post('/api/checkout', values);
toast.success('Order placed successfully!');
} catch (error) {
toast.error('Failed to place order. Please try again.');
} finally {
setSubmitting(false);
}
};
return (
<Formik
initialValues={{ name: '', email: '', address: '' }}
onSubmit={handleSubmit}
>
{({ isSubmitting }) => (
<Form>
<div>
<label>Name:</label>
<Field type="text" name="name" required />
</div>
<div>
<label>Email:</label>
<Field type="email" name="email" required />
</div>
<div>
<label>Address:</label>
<Field type="text" name="address" required />
</div>
<button type="submit" disabled={isSubmitting}>
Place Order
</button>
</Form>
)}
</Formik>
);
};
export default CheckoutForm;
Use React Router for navigation and show toasts when users navigate to different pages or perform actions:
import React from 'react';
import { BrowserRouter as Router, Route, Switch, useHistory } from 'react-router-dom';
import CheckoutForm from './CheckoutForm';
import { toast } from 'react-toastify';
const App = () => {
const history = useHistory();
const handleNavigate = () => {
toast.info('Navigating to the checkout page.');
history.push('/checkout');
};
return (
<Router>
<div>
<button onClick={handleNavigate}>Go to Checkout</button>
<Switch>
<Route path="/checkout">
<CheckoutForm />
</Route>
<Route path="/">
<h1>Welcome to the Store</h1>
</Route>
</Switch>
</div>
</Router>
);
};
export default App;
Configure Axios interceptors to handle global notifications for API requests:
import axios from 'axios';
import { toast } from 'react-toastify';
// Axios response interceptor for global notifications
axios.interceptors.response.use(
response => {
// Handle successful response
return response;
},
error => {
// Handle failed response
toast.error('An error occurred while communicating with the server.');
return Promise.reject(error);
}
);
To implement a controlled progress bar in a React Toastify notification, you need to customize the notification to display a progress bar that updates based on a timer or other conditions.
This involves using React hooks to manage the progress state and updating it periodically. You’ll set up a custom toast with a progress element and ensure it updates smoothly over time. This setup provides visual feedback on long-running processes, enhancing user experience by keeping users informed about ongoing tasks.
First, ensure you have all the necessary libraries installed. You’ll need react-toastify for notifications, axios for making HTTP requests, formik for form management, and react-router-dom for routing.
Set up ToastContainer in your application’s entry point to manage and display toast notifications. This component should be included in your main application file so that it can be accessed globally.
Use Formik to handle form state, validation, and submission. Integrate React Toastify to display notifications based on the success or failure of the form submission. You will trigger notifications after receiving responses from your backend.
Utilise React Router for navigation between different parts of your application. Display toasts when users navigate to new pages or perform significant actions, such as moving to the checkout page. This provides immediate feedback and enhances user experience.
Configure Axios to manage global notifications for HTTP requests and responses. Use Axios interceptors to automatically trigger toast notifications for successful or failed API requests, allowing you to handle these events consistently across your application.
Ensure that your API requests use the configured Axios instance to leverage global error handling and notifications. This integration ensures that any API-related events are consistently reported through toast notifications.
Incorporating React Toastify into your application greatly enhances the user experience by delivering timely, informative notifications that keep users engaged and informed. Its seamless integration with libraries such as Formik, Axios, and React Router ensures that feedback is consistently delivered across various aspects of your app, from form submissions and API interactions to navigation events.
The extensive customization options allow you to tailor notifications to fit your application's design and user needs, while global configuration ensures a unified approach to managing user feedback. Overall, React Toastify provides a powerful tool for improving usability and user satisfaction by offering clear, relevant, and well-timed notifications.
Copy and paste below code to page Head section
React Toastify is a popular library for displaying toast notifications in React applications. It provides a simple and customizable way to show notifications for various events and user interactions, such as form submissions or API responses.
To set up React Toastify, import ToastContainer and include it in your component tree, typically in your main application file (e.g., App.js). Then, use the toast function to trigger notifications throughout your application.
Yes, React Toastify offers extensive customization options. You can adjust the position, duration, and type of notifications, as well as apply custom CSS styles to match your application's design.
Integrate React Toastify with Formik by triggering notifications based on form submission results. Use Formik's onSubmit function to handle form submission and display notifications based on success or error.
Configure Axios interceptors to manage global notifications for API requests. Set up interceptors to show notifications for successful responses or errors. This ensures consistent feedback across all API interactions.
Yes, you can use React Toastify with React Router to display notifications related to navigation events. Trigger notifications when users navigate to different routes or perform significant actions.