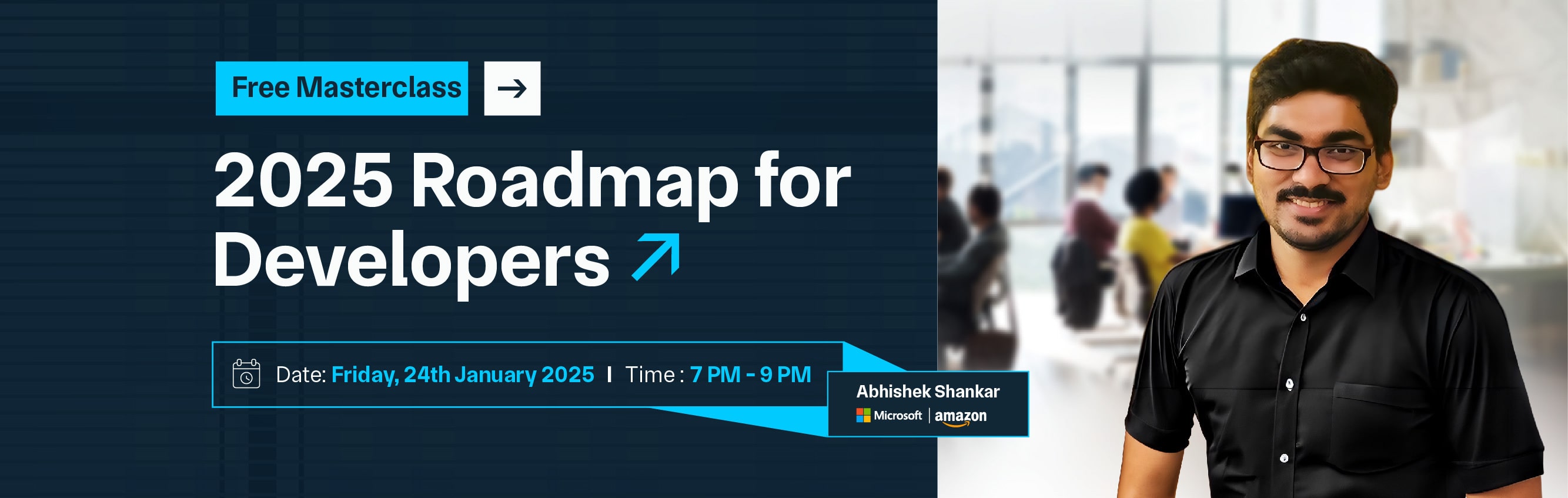

React Native Debugger is an essential tool for developers working with React Native, providing a robust environment for identifying and fixing issues in mobile applications. Integrated with the Chrome DevTools, it allows for powerful debugging capabilities, such as setting breakpoints, inspecting variables, and monitoring network requests in real-time. Developers can easily connect the debugger to their running application, enabling them to view the component hierarchy and analyze performance metrics efficiently.
With features like the network inspector, console log access, and elements inspector, React Native Debugger streamlines the debugging process, making it easier to pinpoint errors and optimize app performance. Additionally, it supports hot reloading, allowing developers to see changes instantly without losing the application state. As mobile applications become increasingly complex, mastering React Native Debugger is vital for ensuring a smooth development workflow and delivering high-quality user experiences.
Whether you are a beginner or an experienced developer, leveraging this powerful tool can significantly enhance your productivity and troubleshooting skills in React Native development. To get started, developers can easily install React Native Debugger and integrate it into their projects, paving the way for more efficient coding practices.
React Native Debugger is a standalone debugging tool specifically designed for React Native applications. It integrates with Chrome DevTools, providing developers with a comprehensive environment to identify and fix issues in their mobile apps. The debugger offers powerful features, including:
By providing these tools, React Native Debugger significantly enhances the development workflow, making it easier to create high-quality, bug-free mobile applications. It’s an essential resource for both beginner and experienced developers looking to improve their debugging efficiency.
React DevTools is available as a Chrome and Firefox extension, and it can also be used in standalone mode for React Native applications. By integrating React DevTools into your workflow, you can significantly enhance your ability to develop and troubleshoot React applications efficiently.
When it comes to debugging in React Native, developers have access to both built-in tools and third-party solutions. Here’s a comparison of the two:
React Native Debugger is a powerful standalone tool that integrates seamlessly with Chrome DevTools, making it an invaluable resource for developers. It allows users to inspect the component hierarchy of their React Native applications, providing insights into how components interact and how state and props are passed down.
The debugger's ability to monitor network requests enables developers to analyze API calls, responses, and any errors that may arise during data fetching. Furthermore, it supports hot reloading, allowing developers to see real-time changes without losing the application state, which significantly speeds up the debugging process and improves overall productivity.
The console log is one of the simplest yet most effective debugging tools available in React Native. Developers can use it to output messages, warnings, or errors directly to the terminal or browser console, providing immediate feedback on the application’s behavior.
This tool is particularly useful for tracking variable values, monitoring flow control, and understanding how different parts of the application interact. Although it may not offer the advanced features of other debugging tools, its simplicity and accessibility make it a go-to solution for quick debugging tasks and initial troubleshooting.
Flipper is an official platform designed for debugging mobile applications, including those built with React Native. It provides a comprehensive suite of tools that enhance the debugging experience, such as network inspection, which allows developers to view and analyze requests made by their applications.
Additionally, Flipper features a layout inspector that helps visualize the component tree and its properties, aiding in UI debugging. Performance monitoring capabilities allow developers to track rendering times and identify bottlenecks. Its integration with various plugins further extends its functionality, making Flipper a versatile choice for debugging React Native applications.
Sentry is a robust error monitoring tool that captures errors in real-time, making it an essential asset for maintaining production-quality applications. It provides detailed reports that include stack traces, user context, and breadcrumbs, which help developers quickly identify the root causes of issues.
Sentry's ability to track errors across different environments allows teams to see which bugs affect users and prioritize fixes accordingly. By integrating Sentry into a React Native project, developers can gain valuable insights into application performance and user experience, ultimately leading to more reliable software.
Bugfender is a remote logging solution that enables developers to capture logs from users' devices in real-time. This is particularly beneficial for diagnosing issues that may occur in production but are difficult to replicate in development environments.
By collecting logs from various devices, developers can gain a comprehensive understanding of how their application behaves under different conditions and configurations. Bugfender's user-friendly interface allows for easy log retrieval and analysis, making it a useful tool for troubleshooting complex problems that arise in live applications.
For applications utilizing Redux for state management, Redux DevTools provides an invaluable suite of features designed to enhance the debugging process. This tool allows developers to track state changes and actions dispatched within the application, giving them insights into how the application's state evolves.
One of its standout features is time-travel debugging, which enables developers to revert to previous states and observe how changes impact the application. By visualizing the state history, developers can pinpoint issues related to state management and ensure that their Redux implementation is functioning correctly.
Here’s a detailed explanation of each React Native debugging tool:
React Native Debugger is a powerful standalone debugging tool that integrates seamlessly with Chrome DevTools, offering developers an efficient environment to inspect and debug their applications. It allows users to visualize the component hierarchy, monitor network requests, and analyze performance metrics.
With features like hot reloading, developers can see real-time changes without losing the application state, significantly speeding up the debugging process. This tool is particularly useful for identifying UI-related issues, tracking state and props, and ensuring that components behave as expected, making it essential for both beginners and experienced developers.
Flipper is an official debugging platform for mobile applications, including those built with React Native. It provides a user-friendly interface and a suite of tools that enhance the debugging experience. With Flipper, developers can inspect network requests, visualize the component hierarchy, and monitor app performance.
It supports various plugins, allowing developers to customize their debugging experience based on specific needs. By facilitating real-time interaction with the app, Flipper helps identify issues quickly and optimizes the development workflow, making it a valuable asset for any React Native project.
Sentry is a robust error-tracking tool that provides real-time insights into application errors and exceptions. By integrating Sentry into a React Native app, developers can capture detailed reports of crashes and bugs that occur in production. These reports include stack traces, user context, and breadcrumbs, helping teams pinpoint the root cause of issues quickly.
Sentry’s ability to track errors across different environments enables developers to prioritize fixes based on user impact, ultimately improving application stability and user experience. Its proactive monitoring ensures that developers can address problems before they escalate.
Bugfender is a remote logging solution that allows developers to capture logs from users' devices in real-time. This capability is particularly valuable for diagnosing issues that occur in production but are difficult to replicate in a development environment.
By collecting logs across various devices, Bugfender provides a comprehensive view of application behavior, enabling developers to understand how the app performs under different conditions. Its user-friendly interface facilitates easy log retrieval and analysis, making it an effective tool for troubleshooting complex problems and enhancing overall application reliability.
Redux DevTools is an essential tool for applications that use Redux for state management. It provides developers with powerful features to track state changes and dispatched actions within the app. One of its standout capabilities is time-travel debugging, which allows developers to revert to previous states and observe how changes impact the application.
This visual representation of state history helps identify issues related to state management and ensures that the Redux implementation is functioning correctly. By integrating Redux DevTools, developers can enhance their debugging efficiency and streamline the development process.
React DevTools is a browser extension that enables developers to inspect the React component hierarchy in their applications. This tool allows users to view the state and props of each component, making it easier to understand how data flows through the application.
Additionally, React DevTools offers performance profiling features that help identify performance bottlenecks and optimize rendering behavior. By providing insights into component interactions and state management, React DevTools enhances the debugging experience and allows developers to build more efficient and responsive applications.
The console.log function is one of the simplest yet most effective debugging tools available in React Native. It allows developers to output messages, warnings, or errors directly to the terminal or console, providing immediate feedback during development. This tool is invaluable for tracking variable values, monitoring flow control, and understanding how different parts of the application interact.
Although it lacks the advanced features of other debugging tools, its straightforward approach makes it a go-to solution for quick debugging tasks and initial troubleshooting, especially for new developers.
Jest is a powerful testing framework that works seamlessly with React Native, enabling developers to write unit tests and integration tests for their applications. By promoting test-driven development (TDD) practices, Jest helps ensure code quality and reliability by catching bugs early in the development process.
Its intuitive API and built-in assertion library make it easy to create and run tests, while features like snapshot testing allow developers to verify UI components' outputs. With Jest, teams can confidently refactor code, knowing that they have robust test coverage to catch regressions and maintain application stability.
Detox is an end-to-end testing framework specifically designed for React Native applications. It allows developers to write and execute automated tests that simulate user interactions, ensuring that the application behaves as expected under real-world conditions. Detox provides a rich set of APIs to interact with UI elements, perform gestures, and validate application responses.
By facilitating comprehensive testing across various devices and platforms, Detox helps catch bugs before they reach production, ultimately enhancing the user experience and increasing confidence in the application’s stability and performance.
Crashlytics is a powerful crash-reporting tool that helps developers track and manage application crashes in real-time. By integrating Crashlytics into a React Native application, developers can receive detailed crash reports, including stack traces, device information, and user activity leading up to the crash. This information is crucial for diagnosing issues and understanding their impact on users.
With its user-friendly dashboard, Crashlytics enables teams to prioritize fixes based on the severity and frequency of crashes, ultimately leading to more stable applications and improved user satisfaction.
Storybook is a UI component explorer that allows developers to build, test, and document components in isolation. By using Storybook, developers can create a library of components with various states and variations, making it easier to visualize and debug UI elements.
This tool facilitates collaboration between designers and developers by providing a clear representation of component behavior and appearance. Storybook also supports extensive add-ons for accessibility testing and performance monitoring, enhancing the overall development workflow and ensuring that UI components are both functional and user-friendly.
Fastlane is an automation tool that streamlines the process of building, testing, and deploying React Native applications. It helps developers automate repetitive tasks, such as generating screenshots, managing app metadata, and deploying to app stores. By integrating Fastlane into the development workflow, teams can save time and reduce manual errors associated with releases.
Its customizable lanes allow developers to define specific workflows tailored to their needs, ultimately enhancing productivity and ensuring consistent deployment practices. Fastlane is particularly useful for teams looking to improve their continuous integration and continuous deployment (CI/CD) processes.
Lottie is a library that enables developers to render animations in React Native applications easily. It supports animations created in Adobe After Effects, allowing developers to use high-quality, vector-based animations without sacrificing performance. Lottie includes tools for previewing and debugging animations, ensuring that they function correctly within the application.
By incorporating Lottie into a project, developers can enhance the user interface with engaging animations that improve the overall user experience. Its lightweight nature and ease of use make it a popular choice for adding visual flair to mobile apps.
ESLint is a static code analysis tool designed to identify and fix code quality issues in JavaScript and JSX. By integrating ESLint into a React Native project, developers can enforce coding standards and best practices, reducing the likelihood of bugs and enhancing code maintainability.
ESLint allows for customizable rules and configurations, enabling teams to tailor it to their specific coding style. Its ability to catch errors early in the development process helps ensure that code is clean, consistent, and free of common pitfalls, ultimately leading to a more robust and reliable application.
Prettier is an opinionated code formatter that helps maintain a consistent code style across React Native projects. By automatically formatting code according to predefined rules, Prettier reduces the cognitive load on developers, allowing them to focus on writing code rather than worrying about style inconsistencies.
This tool integrates easily with most development environments and supports various languages and frameworks. By ensuring a uniform codebase, Prettier enhances readability and collaboration among team members, making it easier to debug and maintain the code over time.
Console errors and warnings are essential debugging indicators in React Native development. They provide developers with immediate feedback about potential issues in their applications, helping to ensure smoother performance and a better user experience.
Console errors are critical messages that indicate something has gone wrong in the application. These errors can arise from various issues, such as syntax errors, missing components, or incorrect prop types. When an error occurs, the console typically provides a stack trace, which helps developers pinpoint the location and nature of the problem.
By addressing these errors promptly, developers can prevent application crashes and improve overall stability. Errors are often displayed in red, making them easy to identify in the console output.
Warnings, while less severe than errors, are still significant indicators of potential problems in the application. They usually indicate deprecated practices, potential performance issues, or incorrect prop usage. Warnings serve as a prompt for developers to review their code and make necessary adjustments, even if the application continues to run.
For example, a warning might suggest that a certain component is not receiving the required props, which could lead to unexpected behavior. Warnings are generally displayed in yellow, making them noticeable but less urgent than errors.
Monitoring console errors and warnings is crucial for maintaining code quality and application performance. By regularly checking the console during development, developers can catch and resolve issues early, ultimately leading to a more robust and user-friendly application.
Tools like React Native Debugger and Flipper enhance this process by providing a more organized view of console messages, making it easier to identify and address problems efficiently.
A performance monitor is a vital tool for developers working with React Native applications, helping them identify and optimize performance bottlenecks to ensure smooth user experiences.
In React Native, performance can be influenced by various factors, including rendering times, memory usage, and network requests. By effectively monitoring these aspects, developers can make informed decisions to enhance their applications' responsiveness and efficiency.
Performance monitors typically provide real-time insights into various metrics. For instance, they can track frame rates, CPU usage, and memory consumption, allowing developers to understand how their app performs under different conditions.
Tools like Flipper and React Native Debugger come equipped with performance monitoring capabilities, enabling developers to visualize the rendering process and pinpoint components that may be causing slowdowns.
One of the critical areas of focus is rendering performance. In React Native, inefficient rendering can lead to sluggish interfaces and a poor user experience. Performance monitors help developers identify components that re-render unnecessarily or take too long to render, prompting them to optimize their code.
Techniques like memoization, PureComponents, and optimizing component hierarchies can be employed to improve performance.
In addition to rendering, monitoring network performance is crucial. Performance monitors can track API call times, response sizes, and network errors. This information is vital for diagnosing issues related to data fetching, which can significantly impact the user experience.
By optimizing network requests, such as implementing caching strategies or using efficient data formats, developers can enhance overall application performance.
Setting up React Native Debugger is a straightforward process that can significantly enhance your development experience by providing powerful debugging capabilities. Here’s a step-by-step guide to getting started:
To begin, download the React Native Debugger application from its GitHub releases page. Choose the appropriate version for your operating system (macOS, Windows, or Linux) and follow the installation instructions. For macOS, you can simply drag the application into your Applications folder.
Next, you need to configure your React Native project to connect with the debugger. If you're using React Native CLI, start your application by running:
react-native start
This command starts the Metro bundler. Once it's running, you can launch your app using:
react-native run-android
or
react-native run-ios
Once your application is running, open the React Native Debugger app. By default, it listens for connections on port 8081. If the debugger is running, it should automatically connect to the React Native app.
To enable debugging in your application, shake your device or press Cmd + D (iOS) or Cmd + M (Android emulator) to open the developer menu. Select “Debug JS Remotely.” This action will open a new tab in your default browser and connect your app to the debugger.
With the debugger connected, you can start inspecting your application. Use the Components tab to view the component hierarchy, inspect state and props, and make live edits. The Network tab allows you to monitor network requests, while the Console tab displays logs and errors from your application.
You can customize the React Native Debugger settings according to your preferences. Click on the gear icon to access the preferences menu, where you can configure various options, such as turning on or off certain features and changing the appearance of the debugger.
Launching React Native Debugger is a critical step in utilizing its powerful features for debugging your React Native applications. Here’s how to effectively launch and connect the debugger to your project:
First, locate the React Native Debugger application you installed previously. For macOS, you can find it in your Applications folder. Double-click the app icon to open it. For Windows or Linux, follow the respective instructions to launch the application.
Before connecting the debugger, you need to ensure your React Native application is running. Open a terminal and navigate to your project directory. Start the Metro bundler by executing the following command:
react-native start
This command initializes the bundler, which serves your JavaScript code. If you haven't already launched the app on a simulator or a physical device, you can do so with:
react-native run-android
or
react-native run-ios
Once your application is running, return to the React Native Debugger. By default, it listens for connections on port 8081, which is the same port used by the Metro bundler. If the debugger is already open when you start your app, it should automatically connect. If not, you can check the connection by looking for any logs in the debugger console.
To enable remote debugging, access the developer menu in your application. On a physical device, shake the device; on an emulator, you can press Cmd + D (iOS) or Cmd + M (Android). From the developer menu, select “Debug JS Remotely.” This will open a new browser tab that connects the app to the React Native Debugger.
Once connected, the React Native Debugger should display logs from your application in the console. You can verify the connection by inspecting the Components tab to view the hierarchy of your app's components and their current states and props.
With the debugger launched and connected, you can now explore its features. Utilize the Console to view logs and errors, the Network tab to monitor API calls, and the Elements tab to inspect and manipulate components in real time. This will greatly enhance your ability to troubleshoot and optimize your application.
Debugging tools are essential for identifying and resolving issues in React Native applications. They provide developers with various features that streamline the troubleshooting process and enhance code quality. Here’s an overview of the key tools and features available for debugging in React Native:
React Native Debugger is a standalone debugging tool that integrates with Chrome DevTools, offering a comprehensive suite of features. It allows developers to inspect the component hierarchy, view the state and props of components, and monitor network requests.
The debugger supports hot reloading, enabling real-time updates without losing the application state. This tool is invaluable for tracking down UI issues and optimizing performance.
Flipper is an official debugging platform for mobile applications, including React Native. It provides a user-friendly interface with various plugins that enhance debugging capabilities.
Key features include network inspection, layout inspection, and performance monitoring. Flipper allows developers to interactively debug their applications and provides useful insights into network activity, helping to identify issues related to API calls.
The console.log function is a simple yet effective way to debug applications. By inserting log statements throughout the code, developers can track variable values and monitor application flow.
Console logs can help pinpoint where issues arise, making them a go-to tool for initial troubleshooting. While basic, they provide immediate feedback and are easy to implement.
React DevTools is a browser extension that allows developers to inspect the component tree of their React Native applications. It provides insights into component states and props, enabling developers to understand how data flows through the app.
Additionally, it offers performance profiling features, helping to identify components that may be causing performance bottlenecks.
Jest is a testing framework commonly used in React Native applications. It enables developers to write unit and integration tests, ensuring that individual components and the overall application function as expected.
Jest’s snapshot testing feature allows developers to capture the rendered output of components, making it easy to track changes and catch regressions during development.
Detox is an end-to-end testing framework designed for React Native applications. It allows developers to write tests that simulate user interactions, verifying that the application behaves correctly in real-world scenarios. Detox helps catch issues that may not be apparent through unit tests, ensuring a smooth user experience.
Error boundaries are React components that catch JavaScript errors in their child components, preventing crashes and allowing for graceful error handling. By implementing error boundaries, developers can display fallback UI or log errors to monitoring services, improving the application's resilience.
Performance monitoring tools, such as Sentry and Crashlytics, provide real-time insights into application performance and errors. They help track crashes, exceptions, and performance bottlenecks, allowing developers to prioritize fixes based on user impact. These tools are vital for maintaining application stability in production.
Debugging is an essential skill for developers, particularly when working with complex applications like those built with React Native. Here are some common debugging techniques that can help identify and resolve issues effectively:
One of the simplest and most effective debugging techniques is using console.log statements to output variable values, function calls, and application states at different points in the code. This allows developers to track the flow of execution and identify where things may be going wrong. While basic, this method is often the first step in diagnosing issues.
Breakpoints allow developers to pause code execution at specific lines. This feature is available in debugging tools like React Native Debugger and Chrome DevTools. By examining the current state, variables, and call stack at a breakpoint, developers can gain insights into how the application behaves at that moment, making it easier to identify bugs.
Implementing error boundaries in React components helps catch errors during rendering. When an error occurs, the boundary can display a fallback UI, preventing the entire application from crashing. This technique not only enhances user experience but also provides insights into where errors are happening, allowing developers to address them more effectively.
Many issues in React Native applications stem from network requests, such as API calls. Using tools like Flipper or the Network tab in React DevTools allows developers to monitor these requests, view response times, and check for errors. This can help identify issues related to data fetching and server communication.
Performance profiling helps identify performance bottlenecks in applications. Tools like React DevTools offer profiling features that show how long each component takes to render. By analyzing this data, developers can optimize components that may be causing slowdowns, improving the overall user experience.
Writing unit tests and integration tests is a proactive debugging technique. Tools like Jest enable developers to test individual components and their interactions, ensuring they function as expected. This approach helps catch errors early in the development process and increases confidence in code reliability.
Conducting code reviews or engaging in pair programming can provide fresh perspectives on problematic code. Collaborating with another developer can help identify issues that one person might overlook. This technique promotes knowledge sharing and often leads to better coding practices.
Linting tools like ESLint can catch potential errors and enforce coding standards before the code is even executed. By integrating linting into the development workflow, developers can prevent common mistakes, such as syntax errors or improper use of React components, thus reducing debugging time later on.
Effective debugging is crucial for developing robust React Native applications. By following best practices, developers can streamline the debugging process and enhance code quality. Here are some key best practices to consider:
When utilizing console.log, ensure that your messages are descriptive and contextually relevant. Instead of generic logs, include variable names and relevant state information. This clarity helps you quickly understand what is being logged, making it easier to track down issues.
Use error boundaries in your React components to gracefully handle errors during rendering. This technique allows you to catch JavaScript errors and prevent the entire application from crashing, displaying a user-friendly error message instead. It also provides insights into where errors occur.
If a component is particularly complex, consider breaking it down into smaller, more manageable parts. This modularity not only simplifies debugging but also enhances code readability and reusability. Smaller components are easier to test and debug in isolation.
Keep your debugging tools and libraries up to date. New versions often come with performance improvements, bug fixes, and additional features that can enhance your debugging experience. Regular updates ensure compatibility with the latest React Native features.
Maintain a document of known issues and their workarounds. This practice helps the development team remember previous problems and their solutions, making it easier to resolve similar issues in the future. It also facilitates onboarding for new team members.
Incorporate unit tests and integration tests using frameworks like Jest. Writing tests not only help identify bugs early but also encourage good coding practices. With a solid testing suite, you can confidently refactor code, knowing that tests will catch regressions.
Utilize performance monitoring tools to keep an eye on the app’s performance over time. Regular monitoring helps identify potential bottlenecks and areas for optimization, ensuring that your app remains responsive and efficient as it evolves.
Keep your code organized and maintain a clean file structure. A well-structured project makes it easier to locate files and components, streamlining the debugging process. Following consistent naming conventions also aids in understanding code functionality at a glance.
Choosing the right debugging tool is essential for effective debugging in React Native. Here are some factors to consider when selecting a tool:
Choose a debugging tool that integrates seamlessly with your existing development environment. Tools like React Native Debugger and Flipper offer easy integration with React Native projects, allowing you to monitor performance, inspect components, and analyze network requests without significant setup.
Evaluate the features offered by the debugging tools. Look for capabilities like component inspection, state and prop visualization, network request monitoring, and performance profiling. A comprehensive feature set will help you diagnose issues more efficiently.
A user-friendly interface can significantly enhance your debugging experience. Choose tools with intuitive layouts and clear navigation, enabling you to access the features you need quickly. A well-designed interface reduces the learning curve and improves productivity.
Consider the level of community support and documentation available for the tool. Tools with active communities often have more resources, tutorials, and troubleshooting guides, making it easier to get help when you encounter challenges.
If your application targets multiple platforms (iOS and Android), ensure that the debugging tool supports both. This compatibility will streamline your development process and reduce the need for switching between different tools for different platforms.
Evaluate the performance impact of the debugging tool on your application. Some tools can slow down the application during debugging, so it’s essential to choose one that provides robust features without significantly affecting performance.
Debugging React Native applications can be challenging, but understanding common issues and how to resolve them can streamline the development process. Below are common issues, along with detailed explanations and coding examples for effective troubleshooting.
When your app fails to start or crashes, the first step is to check the logs. Use the terminal or React Native Debugger to look for any error messages. These logs often include stack traces that point to the source of the problem. A common solution is to clear the Metro bundler cache:
React-native start --reset-cache
If the issue persists, ensure all dependencies are updated. Use npm outdated or yarn outdated to check for outdated packages. Update them with:
npm update
or
yarn upgrade
If your components are not rendering correctly, it’s essential to inspect the props being passed. Use React DevTools or React Native Debugger to view the current props and state of your components. Ensure they are defined correctly. For example, if you’re passing props to a component, check that they’re correctly spelled and formatted:
<MyComponent title={this.state.title} />
If the title is undefined, the component may fail to render as expected. You can also implement a fallback in your component:
const MyComponent = ({ title }) => {
return <Text>{title || 'Default Title'}</Text>;
};
Navigation problems often stem from misconfigured routes. Verify that your navigation stack is set up correctly. If using React Navigation, check your navigator setup:
import { NavigationContainer } from '@react-navigation/native';
import { createStackNavigator } from '@react-navigation/stack';
const Stack = createStackNavigator();
function App() {
return (
<NavigationContainer>
<Stack.Navigator>
<Stack.Screen name="Home" component={HomeScreen} />
<Stack.Screen name="Details" component={DetailsScreen} />
</Stack.Navigator>
</NavigationContainer>
);
}
If you’re still having trouble, debug the navigation state by logging it:
const navigation = useNavigation();
console.log(navigation.getState());
For lagging applications, profiling your components is crucial. Use the React DevTools performance profiler to identify slow components. Here’s how to use React. memo for optimizing rendering:
const MyComponent = React.memo(({ data }) => {
return <Text>{data}</Text>;
});
If the component does not change frequently, memoization can prevent unnecessary re-renders. You can also minimize state updates by restructuring your component hierarchy to limit re-renders of unaffected components.
Network issues can often be traced back to incorrect API endpoints. Test your APIs separately using tools like Postman. In your code, ensure you handle requests properly:
fetch('https://api.example.com/data')
.then(response => {
if (!response. ok) {
throw new Error('Network response was not ok');
}
return response.json();
})
.then(data => console.log(data))
.catch(error => console.error('There was a problem with the fetch operation:', error));
If you encounter CORS issues, verify that your server allows requests from your app's domain. Adjust server settings as necessary.
Build errors can be frustrating but are usually indicated in the terminal logs. Pay attention to error messages and check if you’ve correctly linked native modules. For React Native 0.60 and above, auto-linking should handle most cases, but you can manually link if needed:
react-native link <library-name>
For Android, if you encounter issues, cleaning the build folder may help:
cd android && ./gradlew clean
Then, try rebuilding the app.
Testing on multiple devices is essential since behavior can vary across different platforms. Make sure to check the permissions required for certain features. If you’re using the camera, for example, ensure that permissions are set correctly:
import { PermissionsAndroid } from 'react-native';
async function requestCameraPermission() {
try {
const granted = await PermissionsAndroid.request(
PermissionsAndroid.PERMISSIONS.CAMERA,
{
title: 'Camera Permission',
Message: 'This app needs access to your camera,'
buttonNeutral: 'Ask Me Later',
buttonNegative: 'Cancel',
buttonPositive: 'OK',
}
);
if (granted === PermissionsAndroid.RESULTS.GRANTED) {
console.log('You can use the camera');
} else {
console.log('Camera permission denied');
}
} catch (err) {
console.warn(err);
}
}
Make sure to check the behavior on both iOS and Android, as permission handling can differ between platforms.
React Native Debugger is an invaluable tool for developers working with React Native. Its comprehensive feature set simplifies the debugging process, making it easier to inspect components, monitor network requests, and analyze performance.
By incorporating this tool into your development workflow, you can enhance your productivity and improve the overall quality of your applications. Regular use of React Native Debugger will lead to a deeper understanding of your app's behavior, enabling you to deliver more robust and efficient React Native applications.
Copy and paste below code to page Head section
React Native Debugger is a standalone debugging tool designed for React Native applications. It integrates features from Chrome DevTools and React DevTools, allowing developers to inspect components, monitor network requests, and profile performance.
You can download React Native Debugger from its GitHub repository. Follow the installation instructions provided for your operating system (macOS, Windows, or Linux).
To connect your app, first start your React Native application using react-native start. Then, open React Native Debugger and ensure it’s listening on the default port (8081). Open the developer menu in your app (by shaking the device or using the emulator) and select "Debug JS Remotely."
The Network tab allows you to monitor all network requests made by your application. You can view request URLs, response statuses, and payloads, which helps in diagnosing issues related to API calls and data fetching.
Yes, React Native Debugger includes performance profiling tools that allow you to analyze the rendering performance of your components. This feature helps identify slow components and optimize them for a better user experience.
Yes, React Native Debugger can be used for both iOS and Android applications. It provides a consistent debugging experience across platforms.