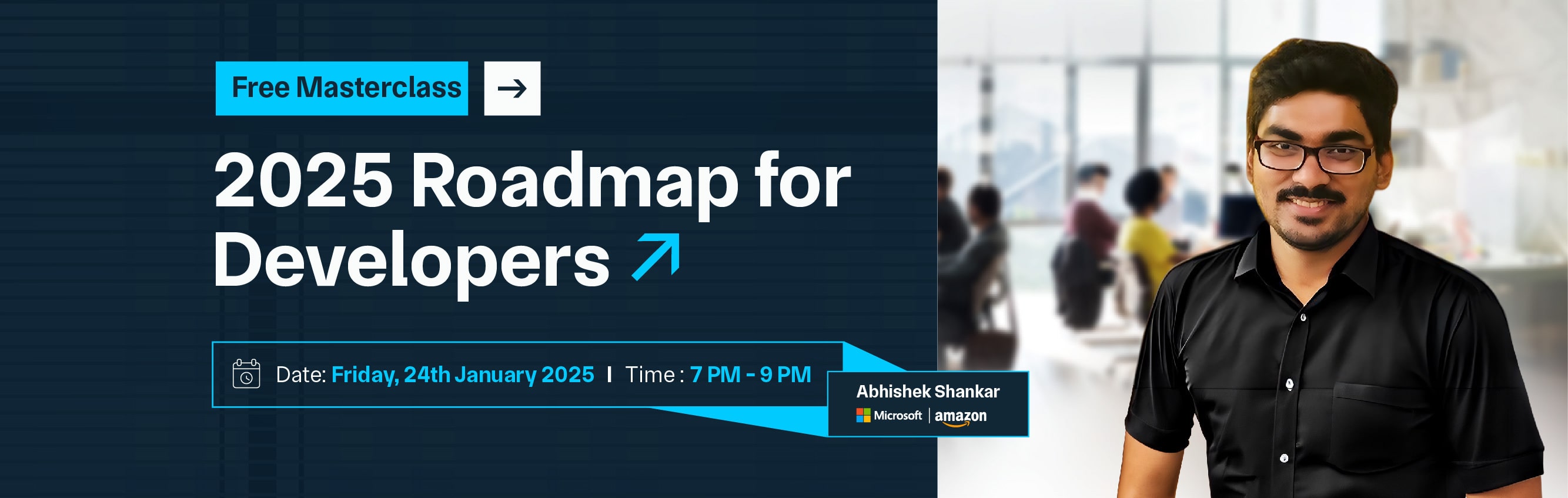

Understanding React architecture is crucial for developing efficient and scalable web applications. At its core, React is a JavaScript library designed for building user interfaces through a component-based architecture. This allows developers to create reusable and modular components, enhancing maintainability and organization within the codebase. Key concepts include props and state, which manage data flow and enable dynamic rendering of components.
The virtual DOM improves performance by minimizing direct manipulation of the actual DOM, leading to faster updates and a smoother user experience. React’s lifecycle methods and hooks, such as useEffect and useState, facilitate managing component behavior during different phases of their lifecycle. State management is also vital, with tools like Redux and the Context API allowing for centralized data handling, especially in larger applications.
Furthermore, routing is efficiently managed with libraries like React Router, enabling dynamic navigation between views. Styling options vary, including traditional CSS and modern CSS-in-JS solutions. Testing frameworks, such as Jest and React Testing Library, ensure the reliability of components. By mastering these aspects of React architecture, developers can create robust applications that are both performant and easy to scale, ultimately enhancing user satisfaction and engagement.
React is an open-source JavaScript library developed by Facebook for building user interfaces, particularly for single-page applications. It allows developers to create reusable UI components that manage their state, making it easier to build complex user interfaces efficiently. One of React's key features is the virtual DOM, which optimizes rendering by updating only the parts of the UI that have changed rather than reloading the entire page.
This results in faster and smoother user experiences. React uses a declarative approach, meaning developers describe what the UI should look like for different states, and React takes care of updating the view as data changes. It supports component-based architecture, which promotes reusability and modular design, allowing teams to work on different parts of an application simultaneously.
React can be used with various state management libraries, such as Redux and MobX, and it seamlessly integrates with other libraries and frameworks. Its ecosystem also includes tools like React Router for routing and Next.js for server-side rendering. Overall, React is widely adopted for its flexibility, performance, and ability to create dynamic web applications efficiently.
React architecture is foundational for building dynamic user interfaces in web applications. At its core, React utilizes a component-based approach that promotes reusability and modular design. Key concepts such as props, state, and the virtual DOM play crucial roles in managing data flow and optimizing performance.
Understanding these core principles—along with lifecycle methods, the Context API, and hooks—enables developers to create efficient and scalable applications. This guide explores the essential elements of React architecture, providing a comprehensive overview for anyone looking to deepen their knowledge of this powerful library.
React applications are built using components, which are the building blocks of the UI. Components can be functional or class-based and are designed to encapsulate both markup and behavior. They promote reusability and help manage the complexity of the application by breaking the UI into smaller, manageable pieces.
Props (short for properties) are used to pass data and event handlers from one component to another. They allow components to communicate with each other, enabling a unidirectional data flow. Props are immutable, meaning a component cannot modify its props, which ensures predictable behavior.
A state is a mutable data structure that holds information about a component's current condition. Unlike props, which are passed down from parent to child, the state is managed within the component itself. Changes to the state trigger re-renders, allowing the UI to reflect the latest data.
Lifecycle methods are special functions that allow developers to hook into specific points in a component's life, such as when it is mounted, updated, or unmounted. They enable fine-grained control over component behavior during these phases. In functional components, hooks like useEffect serve a similar purpose.
The virtual DOM is a lightweight representation of the actual DOM. When changes occur in a React application, they are first reflected in the virtual DOM. React then efficiently updates the actual DOM by calculating the difference (or "diffing") between the previous and current virtual DOM states. This optimization leads to improved performance and responsiveness.
The Context API provides a way to share values between components without explicitly passing props through every level of the component tree. This is particularly useful for managing global data such as user authentication or theme settings.
Hooks are functions that let developers use state, and other React features in functional components. The most common hooks are useState for managing local state and useEffect for handling side effects like data fetching. Hooks simplify component logic and encourage a more functional programming style.
React Router is a popular library for managing navigation and routing in React applications. It allows developers to create dynamic, multi-page experiences by mapping URLs to specific components, making it easier to manage navigation and state within single-page applications.
By understanding these core concepts, developers can effectively leverage React's architecture to build dynamic, efficient, and maintainable web applications.
The React component lifecycle refers to the series of methods and phases that a component goes through from its creation to its removal from the DOM. Understanding these phases is essential for managing component behavior effectively. The lifecycle can be divided into three main phases: mounting, updating, and unmounting.
The mounting phase occurs when a component is created and inserted into the DOM. Key lifecycle methods include constructor, which initializes state and binds methods, and componentDidMount, invoked immediately after mounting. This is an ideal place for API calls or setting up subscriptions, ensuring components are ready for interaction.
During the updating phase, a component re-renders in response to changes in state or props. The shouldComponentUpdate method allows for performance optimization by preventing unnecessary re-renders based on conditions. After an update, componentDidUpdate is called, enabling developers to perform actions based on the new state or props.
The unmounting phase happens when a component is removed from the DOM. The primary method, componentWillUnmount, is crucial for cleanup tasks. It is used to invalidate timers, cancel network requests, or remove event listeners, helping to prevent memory leaks and ensuring a smooth user experience as components are disposed of.
With the introduction of hooks, functional components can effectively manage lifecycle events using useEffect. This hook replaces several lifecycle methods, allowing developers to perform side effects in response to changes in state or props. By specifying dependencies, developers can control when effects run, enhancing component efficiency.
Creating a React application typically follows a structured workflow. Here’s a general overview:
Create React App: Use Create React App for a quick setup.
npx create-react-app my-app
cd my-app
Understand the Structure: Familiarize yourself with the default project structure:
my-app/
├── public/
├── src/
│ ├── components/
│ ├── pages/
│ ├── hooks/
│ ├── styles/
│ └── App.js
├── package.json
└── README.md
Routing: Set up routing with React Router if needed.
npm install react-router-dom
Build for Production: Use the build command to create an optimized version of your app.
npm run build
This workflow provides a solid foundation for developing a React application. Adjust it as necessary to fit your project's specific needs!
State management in React is essential for maintaining and controlling the dynamic data that affects how components render and behave. Proper state management ensures that your application remains organized, scalable, and responsive to user interactions. Here’s an overview of key concepts and tools for effective state management in React:
Local state is managed within a component using the useState hook (for functional components) or this.state (for class components). It is ideal for handling data specific to a single component, such as form inputs or toggle states. Changes to local state trigger re-renders, ensuring the UI reflects the latest data.
When multiple components need to share the same state, lifting the state up is a common approach. This involves moving the shared state to the nearest common ancestor and passing it down as props to child components. This method ensures consistent data across components but can lead to "prop drilling," where props are passed through multiple layers.
The Context API allows for easier state sharing across the component tree without the need to pass props down manually. By creating a context and using the Provider component, developers can make the state available to any nested components. This is especially useful for global data, such as user authentication or theme settings.
For larger applications with complex state needs, libraries like Redux or MobX can simplify state management. Redux employs a single source of truth and uses actions and reducers to manage state changes predictably. MobX, on the other hand, uses observables for more automatic state updates. These libraries facilitate better organization and debugging of state in large-scale applications.
To maintain a clean and efficient state management system, consider best practices like minimizing the number of stateful components, using local state for component-specific data, and leveraging tools like the Redux DevTools for monitoring state changes. Additionally, keeping the state as simple as possible and using the derived state when appropriate can enhance performance and maintainability.
By mastering these state management concepts and tools, React developers can create more robust, efficient, and user-friendly applications that effectively handle dynamic data.
Routing in React applications is crucial for managing navigation and rendering different views based on user interactions. It allows developers to create single-page applications (SPAs) that provide a seamless user experience without full-page reloads. Here’s an overview of key concepts and tools related to routing in React:
React Router is the most popular library for implementing routing in React applications. It provides a set of components and APIs that facilitate the creation of dynamic routes. With React Router, developers can define various routes and associate them with specific components, enabling users to navigate between different parts of the application easily.
The Route component is used to specify a path and the component to render when that path is matched. The Switch component is utilized to render the first Route that matches the current location, ensuring that only one route is rendered at a time. This setup simplifies managing multiple routes within the application.
Dynamic routing allows applications to render components based on URL parameters, making it possible to create flexible and reusable components. For example, a blog application can have a route like /posts/:id, where :id represents the unique identifier for a specific post. This enables fetching and displaying the relevant data based on the URL.
React Router supports nested routing, allowing developers to define routes within other routes. This is particularly useful for creating complex layouts where specific components can be rendered depending on the parent route. For instance, an admin dashboard might have nested routes for user management, settings, and analytics.
In addition to links, React Router allows for programmatic navigation using the useHistory hook (in functional components) or the withRouter higher-order component. This enables developers to navigate programmatically based on events, such as after form submission or when a certain condition is met.
Route guards help protect certain routes from unauthorized access. By implementing logic to check user authentication or permissions before rendering a specific route, developers can control access to sensitive areas of the application, enhancing security.
To maintain a clean and efficient routing system, consider best practices such as using descriptive route names, organizing routes in a centralized file, and leveraging lazy loading for route components to optimize performance. Proper error handling for unmatched routes is also important for improving user experience.
By effectively implementing routing in React applications, developers can create a smooth and intuitive navigation experience that enhances user engagement and satisfaction.
Styling in React involves various approaches and techniques to design components and manage the appearance of applications. Each method offers distinct advantages, allowing developers to choose the best fit for their project’s needs. Here’s an overview of the most common styling techniques in React:
Using traditional CSS stylesheets is a straightforward approach where developers create separate .css files for styling. This method keeps styles organized and familiar for those accustomed to standard web development. Classes are assigned to React components using the className attribute, enabling easy styling.
Inline styles allow developers to apply styles directly within a component using a JavaScript object. This method is useful for dynamic styling based on component states or props. While it provides flexibility, inline styles do not support features like pseudo-classes and media queries.
CSS Modules offer a way to scope CSS styles to specific components, preventing naming conflicts and ensuring that styles are applied only where intended. With CSS Modules, class names are automatically generated to be unique, promoting modular design. This method requires a specific configuration but enhances maintainability.
Styled-components is a popular CSS-in-JS library that allows developers to write actual CSS code within their JavaScript files. Components are created with styles encapsulated, making it easy to manage and reuse styles. It supports theming and dynamic styling based on props, enhancing flexibility and consistency.
Emotion is another CSS-in-JS library that provides powerful and flexible styling capabilities. It allows developers to write styles using both string and object syntax. With features like theming and global styles, Emotion helps create scalable and maintainable styles while offering excellent performance.
Tailwind CSS is a utility-first CSS framework that allows developers to style components using predefined utility classes. This approach encourages rapid development and reduces the need for custom CSS by composing styles directly in the JSX. Tailwind’s configuration options enable customization to meet specific design needs.
To ensure effective styling in React applications, consider best practices such as maintaining a consistent design system, organizing stylesheets logically, and leveraging component libraries for reusable styles. Additionally, using responsive design techniques and optimizing CSS for performance can enhance user experience across devices.
By exploring these various styling methods, developers can choose the best approach for their React applications, creating visually appealing and maintainable user interfaces.
Building a scalable architecture in React applications is essential for managing growth and maintaining performance as the application evolves. A well-structured architecture allows teams to collaborate effectively and simplifies the addition of new features. Here’s an overview of key principles and best practices for creating a scalable React architecture:
A component-based architecture is fundamental to React. Break down the UI into reusable, self-contained components that encapsulate both functionality and styling. This promotes code reusability, simplifies maintenance, and allows for easier testing. Each component should focus on a single responsibility to enhance clarity and organization.
Logically organize the project directory to facilitate scalability. Common approaches include grouping components by feature or by type (e.g., containers, presentational components). Ensure that related files, such as styles and tests, are co-located with their respective components, making navigation easier for developers.
Implement effective state management strategies to ensure that data flow is clear and predictable. Use local state for component-specific data while leveraging libraries like Redux or the Context API for global state management. Keeping state management centralized can reduce complexity and enhance performance, especially in large applications.
Code splitting is a technique that allows you to break your application into smaller chunks, loading only the necessary code for each route or component. Tools like React.lazy and React.Suspense facilitate this process, improving initial load times and overall performance by reducing the size of JavaScript bundles.
Utilize component libraries (e.g., Material-UI, Ant Design) to ensure consistency and reduce development time. These libraries provide pre-built components that adhere to best practices, allowing teams to focus on building unique features rather than reinventing the wheel.
Incorporate testing into your development workflow to catch bugs early and ensure the stability of your application. Use tools like Jest and React Testing Library to write unit and integration tests for components. Maintaining a robust testing strategy contributes to long-term scalability and reliability.
Monitor and optimize performance throughout the development process. Use tools like React Profiler to identify bottlenecks and ensure that components render efficiently. Implement techniques such as memoization (using React. memo or useMemo) to prevent unnecessary re-renders and enhance responsiveness.
Maintain clear documentation of your codebase, architectural decisions, and component usage guidelines. This practice fosters collaboration among team members and assists new developers in onboarding. Well-documented projects are easier to scale, as everyone understands the structure and purpose of components.
By adhering to these principles and best practices, developers can build a scalable React architecture that supports the growth of their applications while maintaining performance, clarity, and maintainability.
When building React applications, following a solid architecture pattern and best practices can greatly enhance maintainability, scalability, and performance. Here’s a breakdown:
In React, the component-based architecture is fundamental to building UIs. This approach encourages developers to break down the user interface into small, reusable components, each encapsulating its logic and styles. These components can be composed together to form more complex interfaces.
The hierarchical structure allows parent components to manage state and pass data down to child components via props, promoting a clear flow of data and making it easier to reason about how the application behaves. This modularity enhances maintainability, as individual components can be updated or replaced without affecting the entire application.
The container and presentational components pattern helps separate concerns in a React application. Container components are responsible for managing state, handling business logic, and connecting to external data sources (like APIs or state management libraries). They often don’t have any UI elements themselves.
Presentational components, on the other hand, focus solely on rendering UI elements based on the props they receive. This separation allows for better testing and reusability, as presentational components can be easily used in different contexts without worrying about underlying logic.
With the introduction of hooks in React, a hooks-based architecture has become a popular way to manage state and side effects within functional components. Hooks like useState and useEffect enable developers to incorporate local state management and lifecycle methods without the need for class components.
This leads to cleaner and more concise code, as functional components tend to be easier to read and maintain. The ability to create custom hooks further enhances reusability by allowing developers to encapsulate related logic and share it across components.
Managing the state effectively is crucial in React applications, especially as they grow in complexity. Local state managed with useState, is suitable for component-specific data. For shared state across multiple components, the Context API provides a way to avoid prop drilling by allowing components to access global data directly.
In larger applications, more robust solutions like Redux or MobX may be employed to manage global states, providing powerful tools for state changes, middleware, and time-travel debugging. Choosing the right state management pattern based on the application's needs is key to maintaining a smooth user experience.
Organizing your project files effectively is vital for scalability and maintainability. A common practice is to organize files by feature or module rather than by type. For instance, grouping related files (components, styles, tests) for a specific feature together makes it easier to locate and manage code as the application grows.
This modular approach not only enhances clarity but also supports better collaboration among team members, as they can easily understand the structure and find relevant code without digging through unrelated files.
Organize files by feature/module rather than type.
For example:
src/
├── features/
│ ├── auth/
│ ├── dashboard/
├── components/
└── hooks/
By adopting these architecture patterns, you can create a well-structured and maintainable React application that is easier to develop, test, and scale over time.
Adhere to the Single Responsibility Principle by ensuring each component does one thing well. This improves readability and maintainability. Additionally, design components to be reusable; instead of duplicating code, create generic components that can be reused across different parts of the application.
Manage the state effectively by keeping it as local as possible. Use useState for component-level state and the Context API for shared state among components, avoiding unnecessary prop drilling. For more complex state needs, consider libraries like Redux or MobX, but be mindful of over-complicating simple state management scenarios.
To enhance performance, utilize memoization techniques like React.memo for components and useMemo/useCallback for functions and values. Implement code splitting using React.lazy to load components only when needed, reducing the initial load time. For large lists, consider virtualization with libraries such as react-window to render only visible items, improving rendering performance.
Choose a consistent styling approach (e.g., CSS Modules, styled-components, or Emotion) and stick to it throughout the project. Avoid inline styles for complex styling to maintain clarity and performance. Organize styles alongside components or in dedicated style files to enhance modularity and readability.
Implement error boundaries to catch JavaScript errors in child components and provide fallback UI to enhance user experience. Consider logging tools like Sentry to track runtime errors, helping you identify and address issues quickly.
Write unit tests for components using Jest and React Testing Library to ensure they function as expected. Additionally, implement integration tests to verify interactions between components. For end-to-end testing, consider using tools like Cypress to simulate user behavior and validate the application's functionality.
Maintain clear documentation for your codebase. Document components, especially public ones, with detailed prop descriptions and usage examples. A well-maintained README file with setup instructions, project structure, and usage guidelines can greatly aid new developers joining the project.
Use Git for version control and follow a branching strategy (like GitFlow) to manage features, fixes, and releases effectively. Set up Continuous Integration/Continuous Deployment (CI/CD) pipelines to automate testing and deployment processes, ensuring that code changes are regularly integrated and deployed to production reliably.
Prioritize accessibility by adhering to WAI-ARIA guidelines and using semantic HTML. Ensure that components are navigable via keyboard and provide alternative text for images. Implement ARIA roles and properties to enhance screen reader compatibility, making your application usable for everyone.
By following these best practices, you can build robust, maintainable, and user-friendly React applications that stand the test of time and adapt to changing requirements.
Advanced techniques in React architecture enhance the efficiency, performance, and maintainability of applications. These strategies help developers tackle complex scenarios and improve user experiences. Here’s an overview of some advanced techniques:
Higher-order components are functions that take a component and return a new component, enabling code reuse and abstraction. HOCs can add functionality such as logging, data fetching, or authentication checks without modifying the original component. This pattern helps keep components clean and focused on their primary responsibilities.
The Render Props pattern allows components to share code by passing a function as a prop. This function returns a React element, enabling dynamic rendering based on the component's state or props. Render Props provide flexibility and promote code reusability while keeping the components decoupled.
Custom hooks are a powerful way to encapsulate logic that can be reused across components. By creating hooks to manage complex state or side effects, developers can keep components cleaner and more focused on rendering. Custom hooks allow for better organization and promote the DRY (Don’t Repeat Yourself) principle.
Implementing code splitting with dynamic imports allows you to load components only when needed, improving performance and reducing initial load times. Using React.lazy and Suspense, developers can wrap lazy-loaded components, providing a fallback UI while the component is being loaded.
The Context API allows for managing global state without prop drilling. By creating a context and using the Provider component, developers can share state across components efficiently. This is particularly useful for theming, user authentication, and managing application-wide settings.
Server-Side Rendering improves performance and SEO by rendering React components on the server before sending them to the client. Frameworks like Next.js simplify the implementation of SSR, allowing developers to create fast, SEO-friendly applications that load quickly and provide a better user experience.
Static Site Generation involves pre-rendering pages at build time, which enhances performance and SEO. This technique is beneficial for content-driven sites where data changes infrequently. Using frameworks like Gatsby, developers can create static sites that load instantly and are easy to deploy.
Advanced performance optimization techniques include using memoization with React.memo, implementing useMemo and useCallback to prevent unnecessary re-renders, and employing the React Profiler to identify performance bottlenecks. These techniques help ensure smooth user interactions and fast rendering.
Error boundaries are React components that catch JavaScript errors in their child component tree, allowing developers to gracefully handle errors without crashing the entire application. By implementing error boundaries, you can provide fallback UIs and improve the overall user experience.
By leveraging these advanced techniques, developers can enhance their React applications, making them more efficient, maintainable, and responsive to user needs. These strategies enable teams to tackle complex challenges while maintaining a high level of performance and user satisfaction.
At CronJ, we specialize in crafting scalable React architectures that empower businesses to grow and adapt to changing demands. Our approach focuses on leveraging React’s component-based architecture to create modular, reusable components that enhance maintainability and reduce development time.
We understand that scalability goes beyond just handling more users; it involves optimizing performance, ensuring code quality, and facilitating seamless collaboration among development teams.
By combining these strategies, CronJ delivers scalable React architectures that not only meet current needs but also adapt to future growth. Our commitment to quality, performance, and user experience ensures that our clients can confidently navigate their digital journeys.
Testing React applications is essential for ensuring reliability, performance, and a smooth user experience. Effective testing helps identify bugs early in the development process and confirms that components behave as expected. Here’s an overview of key concepts, tools, and best practices for testing React applications:
Focus on user behavior rather than implementation details when writing tests. This approach enhances the relevance of tests and aligns them with actual user experiences. Use queries like getByRole or getByLabelText in React Testing Library to interact with components as users would.
By implementing a comprehensive testing strategy using these concepts and tools, developers can ensure their React applications are robust, maintainable, and deliver a seamless user experience. Testing not only improves code quality but also builds confidence in the application’s functionality as it evolves.
Understanding React architecture is essential for building robust, scalable applications that deliver excellent user experiences. By leveraging component-based design, effective state management, and advanced techniques such as code splitting and context API, developers can create modular and maintainable codebases. A solid architecture not only enhances performance but also facilitates collaboration and future growth.
As React continues to evolve, staying informed about best practices and architectural patterns will empower developers to harness its full potential, enabling the creation of dynamic, responsive applications that meet the demands of users and businesses alike.
Copy and paste below code to page Head section
React architecture refers to the structural design of a React application, focusing on how components, state management, and data flow interact to create a scalable and maintainable codebase.
Component-based architecture promotes reusability and modularity, allowing developers to build self-contained components that can be easily maintained, tested, and reused across different parts of the application.
State management in React involves controlling the state of components through the local state, lifting the state for shared data, or using libraries like Redux and Context API for global state management.
Best practices include organizing components logically, maintaining a clear directory structure, using descriptive names, and keeping components small and focused on single responsibilities.
To enhance performance, implement code splitting, lazy loading, memorization techniques (using React.memo), and optimize rendering by minimizing unnecessary re-renders.
Code splitting is a technique that allows developers to break the application into smaller chunks, loading only the necessary code for each route or component. This improves load times and performance.