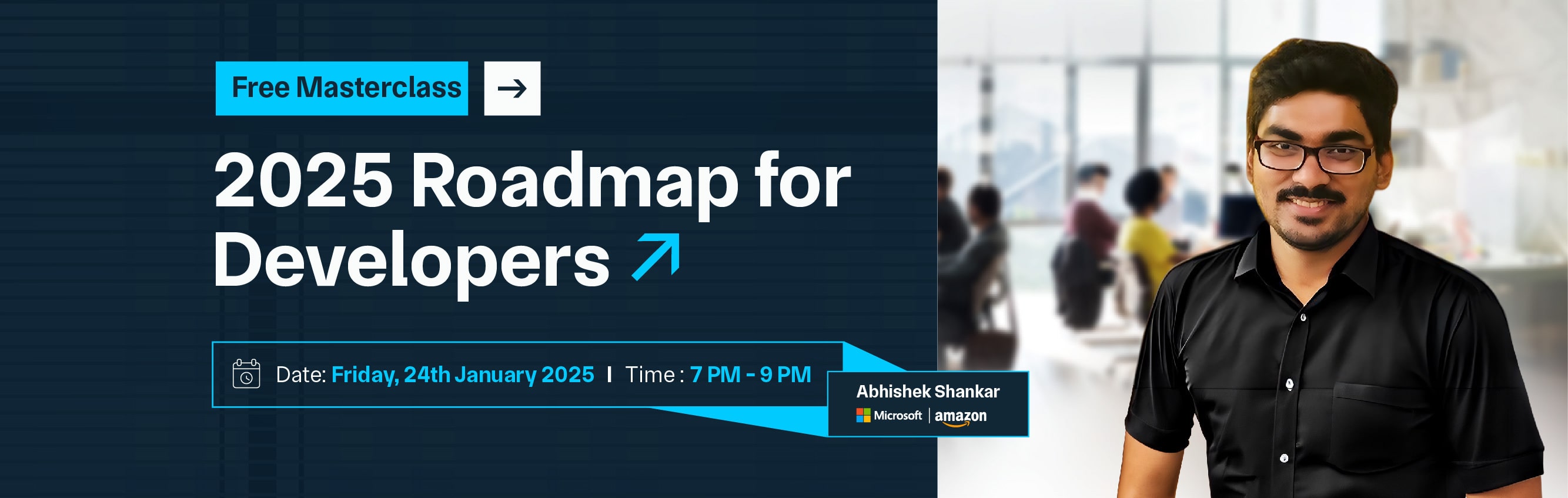

A React Functional Component is a JavaScript function that returns JSX, which defines what the UI should look like. Unlike class components, which are based on ES6 classes and have lifecycle methods and internal state management, functional components are simpler and focus solely on rendering. They receive props as arguments and use these to display data. Initially, functional components were stateless and did not support lifecycle methods, but this changed with the introduction of React Hooks in version 16.8.
Hooks allow functional components to use state (state), handle side effects (useEffect), and access context (useContext), bringing them on par with class components in terms of functionality. This makes functional components more versatile and easier to work with, as they offer a cleaner and more concise syntax. They are preferred for their simplicity and improved performance in many cases.
For example, a basic functional component might simply display a greeting message based on props. In contrast, a component using Hooks can manage local state and perform side effects, such as updating the document title, all within a single function. This modern approach aligns with the trend towards functional programming in JavaScript.
A React Functional Component is a type of component in React, a popular JavaScript library for building user interfaces.
import React from 'react';
function MyComponent(props) {
return <div>Hello, {props.name}!</div>;
}
export default MyComponent;
import React, { useState, useEffect } from 'react';
function Counter() {
const [count, setCount] = useState(0);
useEffect(() => {
document.title = `You clicked ${count} times`;
}, [count]);
return (
<div>
<p>You clicked {count} times</p>
<button onClick={() => setCount(count + 1)}>
Click me
</button>
</div>
);
}
export default Counter;
In this example:
Functional components are often preferred for their simplicity and performance benefits, especially with the power of Hooks to manage state and side effects.
Here's a practical example of a React Functional Component, including one that uses React Hooks for state management and side effects.
This example demonstrates a simple, functional component that takes props and displays a greeting:
import React from 'react';
function Greeting({ name }) {
return <h1>Hello, {name}!</h1>;
}
export default Greeting;
In this example, the functional component uses the useState and useEffect hooks. It manages a counter state and updates the document title based on the counter value:
import React, { estate, useEffect } from 'react';
function Counter() {
// Declare a state variable named 'count' and a function to update it
const [count, setCount] = useState(0);
// useEffect hook to update the document title whenever 'count' changes
useEffect(() => {
document.title = `You clicked ${count} times`;
}, [count]); // Dependency array: effect runs when 'count' changes
// Return JSX to render the component
return (
<div>
<p>You clicked {count} times</p>
<button onClick={() => setCount(count + 1)}>
Click me
</button>
</div>
);
}
export default Counter;
In React, props (short for properties) are used to pass data from a parent component to a child component. A functional component receives props as an argument, which it can use to render dynamic content based on the values provided. Here’s how you can use props in a React functional component:
Here's a simple, functional component that uses props to display a greeting message:
import React from 'react';
// Functional component that accepts props
function Greeting(props) {
return <h1>Hello, {props.name}!</h1>;
}
export default Greeting;
In this example, Greeting is a functional component that takes props as its parameter. It accesses props. name to display a personalized greeting message.
For cleaner code, you can use destructuring to directly extract the properties you need:
import React from 'react';
// Functional component with destructured props
function Greeting({ name, age }) {
return (
<div>
<h1>Hello, {name}!</h1>
<p>You are {age} years old.</p>
</div>
);
}
export default Greeting;
Here, { name, age } is used to extract name and age directly from props. This makes the code more readable and concise.
To use the Greeting component and pass props to it, you would do something like this:
import React from 'react';
import ReactDOM from 'react-dom';
import Greeting from './Greeting'; // Assuming Greeting is in the same directory
function App() {
return (
<div>
<Greeting name="Alice" age={30} />
<Greeting name="Bob" age={25} />
</div>
);
}
ReactDOM.render(<App />, document.getElementById('root'));
In the App component, Greeting is used twice with different name and age props, demonstrating how data can be passed and used in functional components.
In React, an arrow function component is a shorthand syntax for creating functional components using ES6 arrow functions. Arrow functions provide a concise way to write components and are often preferred for their brevity and clarity.
Here’s a basic example of a React arrow function component:
import React from 'react';
// Arrow function component
const Greeting = ({ name }) => {
return <h1>Hello, {name}!</h1>;
};
export default Greeting;
In this example:
Arrow function components can also use React Hooks for state and side effects:
import React, { useState, useEffect } from 'react';
// Arrow function component with Hooks
const Counter = () => {
// Declare a state variable named 'count' and a function to update it
const [count, setCount] = useState(0);
// useEffect hook to perform side effects
useEffect(() => {
document.title = `You clicked ${count} times`;
}, [count]); // Dependency array: effect runs when 'count' changes
// Return JSX to render the component
return (
<div>
<p>You clicked {count} times</p>
<button onClick={() => setCount(count + 1)}>
Click me
</button>
</div>
);
};
export default Counter;
A React Stateless Function Component, also known simply as a "functional component," is a type of component that does not manage its own state or handle lifecycle methods. It is designed primarily for presenting UI based on the data it receives via props. Stateless components are simpler and more efficient than class components for rendering UI.
Here’s an example of a stateless function component:
import React from 'react';
// Stateless function component
function Greeting(props) {
return <h1>Hello, {props.name}!</h1>;
}
export default Greeting;
In this example:
Using destructuring can make the code cleaner:
import React from 'react';
// Stateless function component with destructuring
const Greeting = ({ name }) => {
return <h1>Hello, {name}!</h1>;
};
export default Greeting;
Here’s how you might use the Greeting component within a parent component:
import React from 'react';
import ReactDOM from 'react-dom';
import Greeting from './Greeting'; // Assuming Greeting is in the same directory
function App() {
return (
<div>
<Greeting name="Alice" />
<Greeting name="Bob" />
</div>
);
}
ReactDOM.render(<App />, document.getElementById('root'));
In this example:
Stateless function components are ideal for rendering UI that is purely based on the props received, making them simpler and easier to understand. They are a foundational concept in React and are often used in combination with other features like Hooks to build more complex functionality.
In React, functional components can manage state using Hooks, a feature introduced in React 16.8. The most commonly used Hook for state management is useState. This allows functional components to have a local state, similar to class components but with a simpler syntax.
The useState Hook provides a way to add state to functional components. It returns a state variable and a function to update that state.
Here’s a basic example of a functional component with a state:
import React, { useState } from 'react';
function Counter() {
// Declare a state variable named 'count' and a function to update it
const [count, setCount] = useState(0);
return (
<div>
<p>You clicked {count} times</p>
<button onClick={() => setCount(count + 1)}>
Click me
</button>
</div>
);
}
export default Counter;
1. Importing useState: You need to import useState from React to use it in your component.
2. Declaring State: const [count, setCount] = useState(0);
3. Using State:
You can also manage multiple pieces of state within a single functional component:
import React, { useState } from 'react';
function Form() {
// Declare multiple state variables
const [name, setName] = useState('');
const [age, setAge] = useState('');
return (
<div>
<label>
Name:
<input
type="text"
value={name}
onChange={(e) => setName(e.target.value)}
/>
</label>
<br />
<label>
Age:
<input
type="number"
value={age}
onChange={(e) => setAge(e.target.value)}
/>
</label>
<br />
<p>Name: {name}</p>
<p>Age: {age}</p>
</div>
);
}
export default Form;
Multiple State Variables:
Updating State:
Functional components with state are powerful and flexible, allowing you to build interactive UIs without needing class components. The useState Hook simplifies state management and keeps the component code clean and concise.
In React, event handlers are used to respond to user interactions, such as clicks, form submissions, or keystrokes. When using functional components, event handlers are defined as functions within the component and are passed to JSX elements as props.
Here’s a simple example of a React functional component with an event handler:
import React, { useState } from 'react';
function ClickCounter() {
// Declare a state variable named 'count' and a function to update it
const [count, setCount] = useState(0);
// Event handler function
const handleClick = () => {
setCount(count + 1);
};
return (
<div>
<p>You clicked {count} times</p>
<button onClick={handleClick}>
Click me
</button>
</div>
);
}
export default ClickCounter;
1. State Declaration:
2. Event Handler Function:
3. Event Handling in JSX:
Event handler functions often receive an event object, which provides information about the event. For example, handling form submissions:
import React, { useState } from 'react';
function Form() {
const [name, setName] = useState('');
// Event handler function for form submission
const handleSubmit = (event) => {
event.preventDefault(); // Prevent the default form submission behavior
alert(`A name was submitted: ${name}`);
};
return (
<form onSubmit={handleSubmit}>
<label>
Name:
<input
type="text"
value={name}
onChange={(e) => setName(e.target.value)}
/>
</label>
<button type="submit">Submit</button>
</form>
);
}
export default Form;
1. Form Submission:
2. Form Element:
3. Input Handling:
You can also define event handlers inline in JSX:
import React, { useState } from 'react';
function Button() {
const [message, setMessage] = useState('Hello!');
return (
<button onClick={() => setMessage('Button Clicked!')}>
{message}
</button>
);
}
export default Button;
In this example, the event handler is defined directly within the onClick prop as an arrow function.
Event handlers in functional components provide a way to manage user interactions and respond to events, making them a key part of building interactive UIs in React.
In React, a callback function is a function passed as a prop from a parent component to a child component. It allows the child component to communicate with the parent component or handle events and actions that occur within the child. Callback functions are essential for managing state changes, handling user interactions, and coordinating between components.
Here's a simple example demonstrating a callback function in a React functional component:
import React, { useState } from 'react';
import Child from './Child'; // Assuming Child is in the same directory
function Parent() {
const [message, setMessage] = useState('');
// Callback function to update the message
const handleMessageChange = (newMessage) => {
setMessage(newMessage);
};
return (
<div>
<h1>Message from Child: {message}</h1>
<Child onMessageChange={handleMessageChange} />
</div>
);
}
export default Parent;
import React from 'react';
function Child({ onMessageChange }) {
// Handler function to call the callback with a new message
const sendMessage = () => {
onMessageChange('Hello from the Child component!');
};
return (
<button onClick={sendMessage}>
Send Message to Parent
</button>
);
}
export default Child;
Parent Component:
Child Component:
Callback functions can also be used to pass parameters. For example, if you want to send more complex data:
import React, { useState } from 'react';
import Child from './Child';
function Parent() {
const [data, setData] = useState({ name: '', age: 0 });
const handleDataChange = (name, age) => {
setData({ name, age });
};
return (
<div>
<h1>Name: {data.name}, Age: {data.age}</h1>
<Child onDataChange={handleDataChange} />
</div>
);
}
export default Parent;
import React from 'react';
function Child({ onDataChange }) {
const updateData = () => {
onDataChange('Alice', 30);
};
return (
<button onClick={updateData}>
Update Parent Data
</button>
);
}
export default Child;
Parent Component:
Child Component:
Callback functions facilitate communication between parent and child components in React, making them essential for managing data flow and interactions within your application.
In React, lifecycle methods are traditionally associated with class components, allowing you to perform actions at specific points in a component's lifecycle (e.g., mounting, updating, unmounting). However, with functional components, lifecycle management is achieved using React Hooks, which provide similar capabilities more functionally and concisely.
The useEffect Hook is the primary tool for managing side effects and mimicking lifecycle behavior in functional components. It allows you to run code after the component renders and handle various lifecycle phases such as mounting, updating, and unmounting.
Here’s a simple example of using useEffect in a functional component:
import React, { useState, useEffect } from 'react';
function Example() {
const [count, setCount] = useState(0);
// useEffect to run code after every render
useEffect(() => {
console.log('Component rendered or updated');
// Cleanup function (optional)
return () => {
console.log('Cleanup before next effect or unmount');
};
}, [count]); // Dependency array: effect runs when `count` changes
return (
<div>
<p>You clicked {count} times</p>
<button onClick={() => setCount(count + 1)}>
Click me
</button>
</div>
);
}
export default Example;
Running Effect:
Cleanup Function:
Mounting: To run code once after the component mounts, pass an empty dependency array:
useEffect(() => {
console.log('Component mounted');
}, []); // Empty array: effect runs only once after mounting
Updating: To run code whenever specific dependencies change, include them in the dependency array:
useEffect(() => {
console.log('Count changed:', count);
}, [count]); // Effect runs whenever `count` changes
Unmounting: The cleanup function inside useEffect is called when the component unmounts:
useEffect(() => {
return () => {
console.log('Component unmounted');
};
}, []); // Cleanup runs when the component unmounts
You can use multiple useEffect calls to handle different aspects of lifecycle management:
import React, { useState, useEffect } from 'react';
function MultiEffectComponent() {
const [count, setCount] = useState(0);
const [name, setName] = useState('');
useEffect(() => {
console.log('Component mounted or name changed');
}, [name]); // Runs when `name` changes
useEffect(() => {
console.log('Component mounted or count changed');
}, [count]); // Runs when `count` changes
return (
<div>
<p>Count: {count}</p>
<p>Name: {name}</p>
<button onClick={() => setCount(count + 1)}>Increment Count</button>
<input
type="text"
value={name}
onChange={(e) => setName(e.target.value)}
/>
</div>
);
}
export default MultiEffectComponent;
By using useEffect, functional components in React can manage lifecycle events and side effects effectively, offering a clean and intuitive way to handle component behavior across its lifecycle.
A "pure" React function component is a functional component that renders the same output given the same input (props). It is a concept borrowed from functional programming, emphasizing predictability and immutability. Pure components do not have side effects and do not rely on internal state or lifecycle methods to affect their rendering.
1. Deterministic Output: Given the same props, a pure component will always render the same output. This makes the component predictable and easier to test.
2. No Side Effects: Pure components do not perform actions that affect other parts of the application or interact with external systems (like making HTTP requests or manipulating the DOM directly).
3. Simple and Stateless: Pure function components are often stateless, focusing solely on rendering based on props. They can be either function declarations or arrow functions.
Here’s a simple example of a pure function component:
import React from 'react';
// Pure function component
function Greeting({ name }) {
return <h1>Hello, {name}!</h1>;
}
export default Greeting;
In this example:
Using destructuring in the function parameter can make the code more concise:
import React from 'react';
// Pure function component with destructuring
const Greeting = ({ name, age }) => {
return (
<div>
<h1>Hello, {name}!</h1>
<p>You are {age} years old.</p>
</div>
);
};
export default Greeting;
In scenarios where performance is critical, you can use React.memo to optimize functional components that accept props and might otherwise cause unnecessary re-renders:
import React from 'react';
// Pure function component wrapped with React.memo for optimization
const Greeting = React.memo(({ name }) => {
console.log('Rendering Greeting component');
return <h1>Hello, {name}!</h1>;
});
export default Greeting;
In React, exporting and importing components is essential for organizing and reusing code across different parts of an application. Components can be exported and imported in various ways, depending on whether you want to use named exports or default exports.
A default export allows you to export a single value or component from a module. This is the most common way to export React components when you only have one component per file.
Greeting.js
import React from 'react';
// Default export of the Greeting component
const Greeting = ({ name }) => {
return <h1>Hello, {name}!</h1>;
};
export default Greeting;
App.js
import React from 'react';
import Greeting from './Greeting'; // Importing the default export
function App() {
return (
<div>
<Greeting name="Alice" />
</div>
);
}
export default App;
In this example:
Named exports allow you to export multiple values or components from a module. You use named exports when you want to export multiple components or other values from a single file.
Components.js
import React from 'react';
// Named exports of two components
export const Greeting = ({ name }) => {
return <h1>Hello, {name}!</h1>;
};
export const Farewell = ({ name }) => {
return <h1>Goodbye, {name}!</h1>;
};
App.js
import React from 'react';
import { Greeting, Farewell } from './Components'; // Importing named exports
function App() {
return (
<div>
<Greeting name="Alice" />
<Farewell name="Bob" />
</div>
);
}
export default App;
In this example:
You can also combine default and named exports in the same file:
Components.js
import React from 'react';
// Default export of the main component
const MainComponent = ({ name }) => {
return <h1>Main Component: {name}</h1>;
};
// Named export of an additional component
export const AdditionalComponent = ({ name }) => {
return <h2>Additional Component: {name}</h2>;
};
export default MainComponent;
App.js
import React from 'react';
import MainComponent, { AdditionalComponent } from './Components'; // Combining default and named imports
function App() {
return (
<div>
<MainComponent name="Alice" />
<AdditionalComponent name="Bob" />
</div>
);
}
export default App;
In this example:
In React, ref (short for "reference") is a feature that allows you to directly access and interact with DOM elements or React components created within a functional component. Using refs is useful for scenarios where you need to manage focus, measure dimensions, or perform animations directly on DOM elements. In functional components, you use the useRef Hook to work with refs.
The useRef Hook provides a way to create and manage refs in functional components. It returns a mutable object with a .current property that you can use to store a reference to a DOM element or a React component instance.
Here's a basic example of using useRef to manage a DOM element:
import React, { useRef } from 'react';
function FocusInput() {
const inputRef = useRef(null); // Create a ref using useRef
// Function to focus the input element
const focusInput = () => {
inputRef.current.focus(); // Access the DOM element via ref
};
return (
<div>
<input ref={inputRef} type="text" placeholder="Click button to focus" />
<button onClick={focusInput}>Focus Input</button>
</div>
);
}
export default FocusInput;
Creating the Ref:
Attaching the Ref:
Using the Ref:
You can also manage multiple refs by creating multiple useRef instances:
import React, { useRef } from 'react';
function MultipleRefs() {
const inputRef = useRef(null);
const buttonRef = useRef(null);
const focusInput = () => {
inputRef.current.focus();
};
const highlightButton = () => {
buttonRef.current.style.backgroundColor = 'yellow';
};
return (
<div>
<input ref={inputRef} type="text" placeholder="Focus me" />
<button ref={buttonRef} onClick={highlightButton}>
Highlight Me
</button>
<button onClick={focusInput}>Focus Input</button>
</div>
);
}
export default MultipleRefs;
While refs are commonly used with DOM elements, they can also be used with class components or functional components with forwardRef:
import React, { useRef, forwardRef, useImperativeHandle } from 'react';
// ForwardRef component
const CustomInput = forwardRef((props, ref) => {
const inputRef = useRef(null);
// Expose methods to parent
useImperativeHandle(ref, () => ({
focus: () => {
inputRef.current.focus();
}
}));
return <input ref={inputRef} {...props} />;
});
function Parent() {
const customInputRef = useRef(null);
const focusCustomInput = () => {
customInputRef.current.focus();
};
return (
<div>
<CustomInput ref={customInputRef} placeholder="Forwarded Ref Input" />
<button onClick={focusCustomInput}>Focus Custom Input</button>
</div>
);
}
export default Parent;
Forwarding Refs:
Imperative Handle:
Using the Ref:
Sure! Here’s an example of how to create a React functional component using TypeScript. This example includes basic usage of props and type definitions.
1. Install TypeScript and React Type Definitions
If you haven't set up TypeScript in your React project yet, make sure you have the necessary packages:
npm install typescript @types/react @types/react-dom
2. Create the Component
Here's a simple functional component named Greeting that takes a name prop and renders a greeting message.
// Greeting.tsx
import React from 'react';
// Define the type for props
interface GreetingProps {
name: string;
}
// Define the functional component
const Greeting: React.FC<GreetingProps> = ({ name }) => {
return <h1>Hello, {name}!</h1>;
};
export default Greeting;
Type Definition (GreetingProps):
Functional Component:
Destructuring Props:
You can use this Greeting component in another part of your app like this:
// App.tsx
import React from 'react';
import Greeting from './Greeting';
const App: React.FC = () => {
return (
<div>
<Greeting name="Alice" />
<Greeting name="Bob" />
</div>
);
};
export default App;
In React, you can create components using either function components or class components. Both have their uses, but function components have become more popular with the introduction of Hooks in React 16.8. Here’s a comparison between the two:
Definition: Function components are simpler and easier to write. They are just JavaScript functions that return JSX.
Example:
import React from 'react';
interface Props {
name: string;
}
const Greeting: React.FC<Props> = ({ name }) => {
return <h1>Hello, {name}!</h1>;
};
export default Greeting;
Key Features:
When to Use:
Definition: Class components are created by extending React.Component. They offer a more traditional way to write React components and include lifecycle methods.
Example:
import React, { Component } from 'react';
interface Props {
name: string;
}
interface State {
count: number;
}
class Greeting extends Component<Props, State> {
constructor(props: Props) {
super(props);
this.state = { count: 0 };
}
componentDidMount() {
// Lifecycle method example
console.log('Component mounted');
}
handleClick = () => {
this.setState({ count: this.state.count + 1 });
}
render() {
return (
<div>
<h1>Hello, {this.props.name}!</h1>
<button onClick={this.handleClick}>Click me: {this.state.count}</button>
</div>
);
}
}
export default Greeting;
Key Features:
When to Use:
Function components have become the preferred choice in modern React development due to their simplicity, ease of use, and the powerful capabilities offered by Hooks, which allow for efficient state management and side effect handling. Their concise syntax and reduced boilerplate make them more readable and easier to maintain.
Conversely, class components, while more verbose and complex, are still important for understanding and maintaining older codebases and libraries that utilize lifecycle methods. Overall, function components align with current best practices and are generally recommended for new projects, but familiarity with class components remains valuable for comprehensive React development and legacy code integration.
Copy and paste below code to page Head section
Function components are simpler and use a straightforward syntax, while class components require more boilerplate and are built using ES6 classes. Function components benefit from React Hooks, which allow for state management and side effects handling without needing lifecycle methods. Class components use this and offer lifecycle methods like componentDidMount and componentDidUpdate.
React Hooks are functions that let you use state and other React features without writing a class. They are used exclusively within function components. Common Hooks include useState for managing state and useEffect for handling side effects. Hooks simplify the management of state and side effects in function components and offer a cleaner, more concise way to handle complex logic.
No, Hooks are only applicable to function components. Class components have their way of managing state and lifecycle methods. For class components, you'll use this.state and this.setState for state management and lifecycle methods like componentDidMount and componentDidUpdate.
For most new developments, function components with Hooks are recommended due to their simplicity and efficiency. They make the code more readable and easier to maintain. However, if you are working with legacy code or need to use specific lifecycle methods not yet replicated by Hooks, class components may still be relevant.
Function components often have better performance characteristics due to their simpler structure and the absence of this context, which can reduce overhead. With React’s optimization techniques, such as React.memo, function components can be highly performant. However, performance differences are typically minor, and both function and class components can be optimized effectively.
Class components may be preferred if you need to work within an existing codebase that extensively uses class components or if you require specific lifecycle methods that are not yet available with Hooks. In certain cases, class components might also be more familiar to teams or developers accustomed to older React patterns.