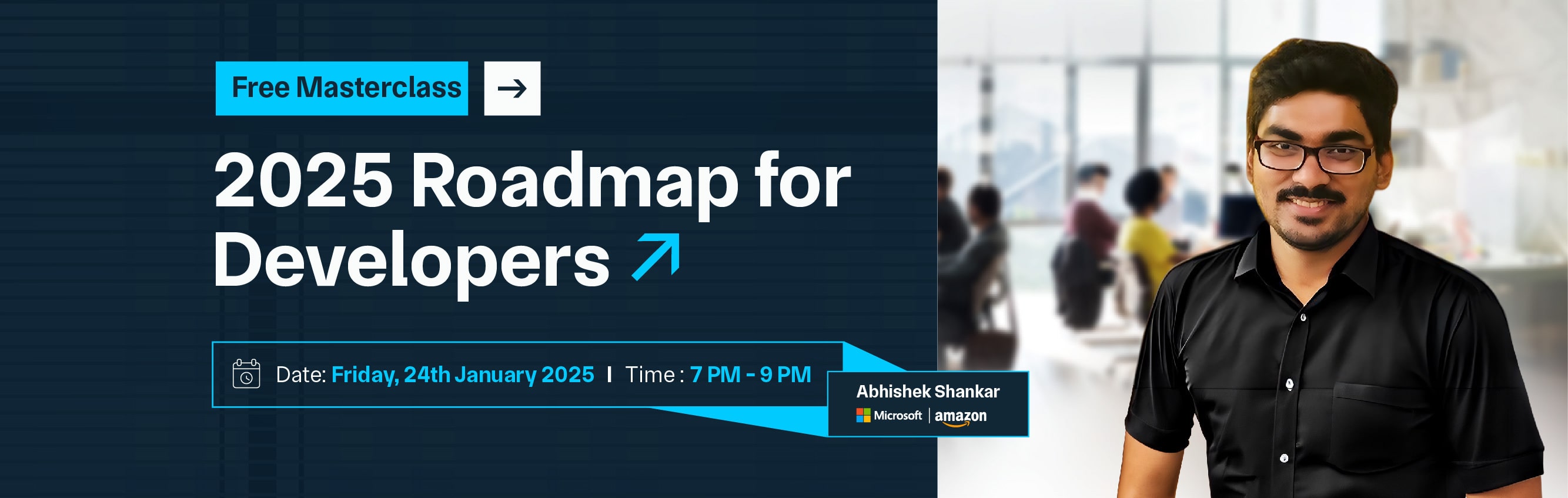

The Python round() function is a built-in utility that rounds a floating-point number to a specified number of decimal places. Its primary syntax is round(number[, ndigits]), where the number is the value to be rounded, and ndigits is an optional argument indicating the number of decimal places to round to. If ndigits is omitted, the function rounds to the nearest integer.
For example, round(3.14159) returns 3, while round(3.14159, 2) returns 3.14. One important aspect of the round() function is its handling of halfway cases, such as rounding 2.5. In Python, it follows the "round half to even" strategy, also known as banker's rounding, which means it rounds to the nearest even number. Thus, round(2.5) results in 2, while round(3.5) yields 4.
This approach minimizes cumulative rounding errors in large datasets. Additionally, round() can be useful in formatting outputs for financial applications or when precise decimal representation is necessary. Understanding the round() function is essential for any Python programmer looking to manage numerical data effectively.
The syntax of the round() function in Python is as follows:
round(number[, ndigits])
The round() function in Python is used to round a floating-point number to a specified number of decimal places. Here are some examples demonstrating its use:
round(number[, ndigits])
Rounding to the Nearest Integer:
result = round(5.7)
print(result) # Output: 6
Rounding to One Decimal Place:
result = round(3.14159, 1)
print(result) # Output: 3.1
Rounding to Two Decimal Places:
result = round(2.71828, 2)
print(result) # Output: 2.72
Rounding a Negative Number:
result = round(-2.5)
print(result) # Output: -2
Rounding Halfway Cases:
print(round(2.5)) # Output: 2 (round half to even)
print(round(3.5)) # Output: 4
print(round(4.5)) # Output: 4
print(round(5.5)) # Output: 6
Rounding Without Specifying ndigits:
result = round(7.8)
print(result) # Output: 8
Rounding with Zero Decimal Places:
result = round(9.999, 0)
print(result) # Output: 10.0 (returns a float)
The round() function in Python has two parameters:
1. Number:
2. ndigits (optional):
Rounding to Nearest Integer:
round(4.7) # Returns: 5
Rounding to One Decimal Place:
round(3.14159, 1) # Returns: 3.1
Rounding to Two Decimal Places:
round(2.678, 2) # Returns: 2.68
Omitting ndigits:
round(5.5) # Returns: 6 (rounds to the nearest even number)
Negative Numbers:
round(-3.5) # Returns: -4
Understanding these parameters allows you to effectively use the round() function for various rounding needs in your Python programs.
The round() function in Python returns different types of values based on the parameters provided:
1. When ndigits is Omitted:
Example:
result = round(4.7)
print(result) # Output: 5
2. When ndigits is Specified:
Example:
result = round(3.14159, 2)
print(result) # Output: 3.14
3. Return Type:
Example:
result = round(5.0, 1)
print(result) # Output: 5.0 (Return type is float)
Floating-point numbers in programming, including Python, can exhibit several anomalies due to their representation and inherent limitations. Here are some common issues:
1. Precision Errors:
Floating-point numbers cannot represent all decimal values exactly. For example, 0.1 and 0.2 cannot be precisely represented in binary, leading to rounding errors. This often results in unexpected behavior during arithmetic operations.
print(0.1 + 0.2) # Output: 0.30000000000000004
2. Rounding Errors:
When performing calculations, results may be different from what you expect due to rounding errors. These occur because the binary representation of the numbers is truncated.
a = 0.1 + 0.2
print(a == 0.3) # Output: False
3. Overflow and Underflow:
Floating-point numbers have a maximum and minimum value. Exceeding these limits leads to overflow (resulting in inf) or underflow (resulting in 0).
print(1e+308 * 10) # Output: inf
4. Comparisons:
Due to precision issues, comparing floating-point numbers can yield misleading results. It’s often better to check if two numbers are "close enough" rather than exactly equal.
import math
print(math.isclose(0.1 + 0.2, 0.3)) # Output: True
5. Accumulated Errors:
In iterative calculations or large datasets, small errors can accumulate, leading to significant discrepancies. This is particularly relevant in algorithms like numerical integration.
The round() function is widely used in various applications where numerical precision is essential. Here are some practical applications:
1. Financial Calculations:
In financial applications, it's crucial to round monetary values to two decimal places to represent cents accurately.
For example:
price = 19.995
rounded_price = round(price, 2) # Output: 20.00
2. Scientific Data Analysis:
When analyzing scientific data, rounding can simplify results for reporting purposes. For instance, rounding measurement results to a reasonable number of significant figures can make data more interpretable.
measurement = 0.123456
rounded_measurement = round(measurement, 3) # Output: 0.123
3. User Interface Display:
In applications with graphical user interfaces, rounded numbers can enhance readability. Displaying rounded figures in dashboards or reports can make the data more user-friendly.
score = 87.6789
display_score = round(score, 1) # Output: 87.7
4. Statistical Analysis:
In statistics, rounding can be applied when presenting means, medians, or other calculated values to a specified number of decimal places for clarity.
average = 67.456
rounded_average = round(average, 2) # Output: 67.46
5. Game Development:
In game development, scores, health points, or resource counts need to be rounded to provide an engaging experience for players.
For instance:
player_health = 95.75
rounded_health = round(player_health) # Output: 96
6. Data Normalization:
Rounding is often used in data preprocessing to normalize values before analysis or modeling, ensuring consistency in input data.
values = [0.1, 0.2, 0.15, 0.25]
normalized_values = [round(v, 1) for v in values] # Output: [0.1, 0.2, 0.2, 0.3]
The round() function can also be applied to NumPy arrays for efficient rounding of multiple elements at once. Here’s how to do it:
import numpy as np
# Create a NumPy array
data = np.array([1.5, 2.3, 3.7, 4.2, 5.8])
# Round to the nearest integer
rounded_data = np.round(data)
print("Original Array:", data) # Output: Original Array: [1.5 2.3 3.7 4.2 5.8]
print("Rounded Array:", rounded_data) # Output: Rounded Array: [2. 2. 4. 4. 6.]
You can also specify the number of decimal places to round to by passing an additional argument to np.round().
# Round to one decimal place
rounded_one_decimal = np.round(data, 1)
print("Rounded to One Decimal Place:", rounded_one_decimal) # Output: Rounded to One Decimal PPlace: [1.5 2.3 3.7 4.2 5.8]
The round() function in Python can handle negative numbers just as effectively as positive numbers. Here are some examples to illustrate how it works:
When rounding negative floating-point numbers, Python follows the same rules as for positive numbers. The function rounds to the nearest integer or specified decimal place.
# Rounding a negative float
negative_float = -3.7
rounded_value = round(negative_float)
print("Original Value:", negative_float) # Output: Original Value: -3.7
print("Rounded Value:", rounded_value) # Output: Rounded Value: -4
The round() function in Python can also be applied to integer values. When rounding integers, the function simply returns the integer as it is since integers are already whole numbers. However, you can still specify the ndigits parameter if needed.
When you pass an integer to the round() function without specifying ndigits, it returns the integer unchanged.
# Rounding an integer
integer_value = 7
rounded_value = round(integer_value)
print("Original Value:", integer_value) # Output: Original Value: 7
print("Rounded Value:", rounded_value) # Output: Rounded Value: 7
Even though integers don't have decimal places, you can specify ndigits. The function will return a float.
# Rounding an integer with ndigits specified
integer_value_2 = 15
rounded_value_2 = round(integer_value_2, 1)
print("Original Value:", integer_value_2) # Output: Original Value: 15
print("Rounded Value:", rounded_value_2) # Output: Rounded Value: 15.0
The round() function is commonly used to round floating-point numbers to the nearest integer or to a specified number of decimal places. Here are some examples:
When you pass a float to the round() function without specifying ndigits, it rounds the number to the nearest integer.
# Rounding a positive float
positive_float = 4.6
rounded_value = round(positive_float)
print("Original Value:", positive_float) # Output: Original Value: 4.6
print("Rounded Value:", rounded_value) # Output: Rounded Value: 5
You can round a float to a specific number of decimal places by providing the ndigits parameter.
# Rounding a float to two decimal places
float_value = 3.14159
rounded_value_2 = round(float_value, 2)
print("Original Value:", float_value) # Output: Original Value: 3.14159
print("Rounded Value:", rounded_value_2) # Output: Rounded Value: 3.14
The round() function is widely utilized across various domains. Here are some practical applications:
Financial Calculations:
Rounding is crucial in finance to ensure monetary values are represented accurately. For example, prices, taxes, and interest rates are often rounded to two decimal places to reflect cents.
price = 19.995
rounded_price = round(price, 2) # Output: 20.00
Scientific Measurements:
In scientific research, measurements are often rounded to a specific number of significant figures for clarity and consistency in reporting results.
measurement = 0.123456
rounded_measurement = round(measurement, 3) # Output: 0.123
User Interface Design:
In applications and dashboards, rounded numbers enhance readability. Displaying rounded values improves user experience by making data more digestible.
score = 87.6789
display_score = round(score, 1) # Output: 87.7
Statistical Analysis:
When presenting statistical results, such as averages or standard deviations, rounding can provide a clearer and more concise representation of data.
average = 67.456
rounded_average = round(average, 2) # Output: 67.46
Game Development:
In gaming, scores, health points, or resources may be rounded to provide an engaging experience for players. This can make the numbers easier to interpret during gameplay.
player_health = 95.75
rounded_health = round(player_health) # Output: 96
The round() function in Python is a powerful and versatile tool for managing numerical data. Its ability to round both floating-point and integer values makes it indispensable in various applications, from financial calculations and scientific measurements to user interface design and data analysis.
By ensuring clarity and precision, the round() function enhances the accuracy of data presentation and improves user experiences. Understanding how to utilize rounding in programming effectively can lead to better decision-making and more reliable outcomes across different domains.
Copy and paste below code to page Head section
The round() function is used to round a floating-point number to the nearest integer or to a specified number of decimal places.
The round() function takes two parameters: number (the value to round) and ndigits (optional, the number of decimal places to round to).
If you omit ndigits, the round() function will round the number to the nearest integer and return it as an integer.
Python uses "round half to even" (bankers' rounding) for halfway cases. For example, round(2.5) results in 2, while round(3.5) results in 4.
Yes, the round() function works with negative numbers in the same way it does with positive numbers, following the same rounding rules.
The return type of round() is int if ndigits are omitted and a result is a whole number. If ndigits is specified, it returns a float.