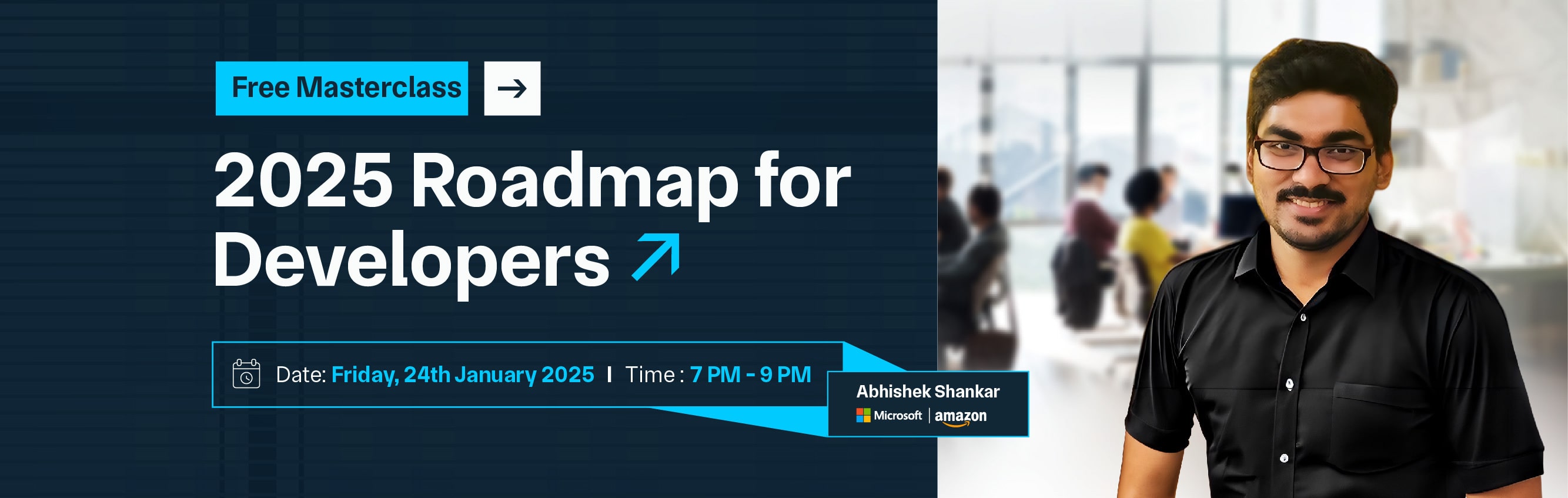

In Angular, property binding is a fundamental concept that allows you to set the properties of HTML elements dynamically based on component data. It establishes a communication channel from the component class to the template (HTML file), letting you pass data from the component to the view and update the view when the data changes. To use property binding, you enclose an HTML attribute within square brackets ([]) and assign it to a component property.
For example, <img [src]="imageUrl"> binds the src attribute of the IMG tag to the imageUrl property of the component. Here, imageUrl could be a string property in the component class containing the path to an image. Angular evaluates the expression inside the brackets and assigns it to the corresponding DOM property. If the component property changes, Angular automatically updates the DOM to reflect the new value, ensuring synchronization between the component and the view.
Property binding is particularly useful for tasks like toggling attributes, setting dynamic styles, conditionally rendering elements, or implementing interactive features. It enhances Angular's declarative approach, enabling developers to build dynamic and responsive web applications efficiently by leveraging the power of TypeScript and HTML integration provided by Angular's framework.
Property binding in Angular is a mechanism that allows you to set values for HTML attributes or DOM properties dynamically. It establishes a communication channel from the component class to the template (HTML file), enabling you to pass data from your component to the corresponding view.
In Angular, property binding is denoted by enclosing an HTML attribute within square brackets ([]) and assigning it to a property or expression in the component class. For example, <img [src]="imageUrl"> binds the src attribute of the IMG tag to the imageUrl property of the component class.
The key features of property binding include
Property binding is essential for creating interactive and dynamic Angular applications, as it facilitates the synchronization of data between the component and the view, enabling responsive user interfaces and efficient data management.
a simple example of property binding in Angular.
Suppose we have a component with a property pageTitle defined in its TypeScript file (app.component.ts):
import { Component } from '@angular/core';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
pageTitle: string = 'Welcome to Angular Property Binding Example';
}
In the corresponding HTML template file (app.component.html), we want to bind this pageTitle property to display it dynamically in an < h1 > heading:
<!-- app.component.html -->
<h1>{{ pageTitle }}</h1>
In this example:
This is a straightforward demonstration of how property binding allows you to dynamically display data from the component class in the HTML template, enhancing the flexibility and responsiveness of your Angular application.
In Angular, data binding is a powerful feature that establishes a connection between the component (business logic written in TypeScript) and the view (HTML template). Angular supports four types of data binding:
Interpolation is denoted by double curly braces ({{ }}) and allows you to output component data into the HTML template. For example, <p>Hello, {{ name }}!</p> would display "Hello, [name value]!" where the name is a property in the component class.
Example: html
<h1>{{ pageTitle }}</h1>
1. Here, pageTitle is a property in the component class whose value will be rendered in the < h1 > element.
Property binding allows you to set values for HTML attributes or DOM properties dynamically based on the component data. It uses square brackets ([]) and binds a property of an HTML element to a property in the component class.
Example: html
<img [src]="imageUrl">
2. Here, imageUrl is a property in the component class containing the path to an image, and it dynamically sets the src attribute of the <img> tag.
Event binding allows you to listen to user events such as mouse clicks, keystrokes, or any DOM event, and trigger methods in the component class. It uses parentheses (()) and binds an event of an HTML element to a method in the component class.
Example: html
<button (click)="on button click()">Click me!</button>
3. Here, onButtonClick() is a method defined in the component class that will be called when the < button > is clicked.
Two-way binding allows data to flow both from the component to the view and from the view to the component. It combines property binding and event binding using ngModel directive, typically used with form elements such as < input >, < select >, and < textarea >.
Example: html
<input [(ngModel)]="username">
4. Here, a username is a property in the component class that represents the value of the < input > field. Changes to the username in the component class will update the input field, and changes made by the user in the input field will update the username property in the component class.
These four types of data binding enable Angular developers to create dynamic and interactive web applications, facilitating seamless communication between the component logic and the user interface.
In Angular, when we talk about "binding targets," we're referring to the specific HTML attributes or DOM properties that we can bind to from within our Angular components. Several common binding targets are frequently used:
1. Text Content ({{ }} - Interpolation)
2. HTML Attributes ([attribute]="value" - Property Binding)
3. DOM Properties ([property]="value" - Property Binding)
4. Event Handlers ((event)="handler()" - Event Binding)
5. Two-Way Data Binding ([(ngModel)]="property")
Understanding these binding targets is crucial for effectively building dynamic and responsive Angular applications. They provide powerful tools for managing data flow between the component logic (written in TypeScript) and the user interface (defined in HTML templates). Each binding target serves a specific purpose, enabling developers to create interactive and data-driven web applications efficiently.
In Angular, passing objects between components typically involves using property binding or event binding to transfer data from a parent component to a child component or between components that are not directly related.
Parent Component (Sender):
typescript
import { Component } from '@angular/core';
@Component({
selector: 'app-parent',
template: `
<app-child [childData]="parentData"></app-child>
`
})
export class ParentComponent {
parentData = { id: 1, name: 'John Doe' };
}
html
<app-child [childData]="parentData"></app-child>
Child Component (Receiver):
typescript
import { Component, Input } from '@angular/core';
@Component({
selector: 'app-child',
template: `
<div>
<p>ID: {{ childData.id }}</p>
<p>Name: {{ childData.name }}</p>
</div>
`
})
export class ChildComponent {
@Input() child data: { id: number, name: string };
}
html
<div>
<p>ID: {{ childData.id }}</p>
<p>Name: {{ childData.name }}</p>
</div>
Parent Component:
Child Component:
This approach leverages Angular's property binding mechanism (@Input() decorator) to facilitate the seamless transfer of objects between components, enhancing the modularity and maintainability of your application.
When using property binding in Angular, it's important to consider security implications to prevent vulnerabilities such as XSS (Cross-Site Scripting) attacks. Angular provides mechanisms to mitigate these risks, but developers must follow best practices to ensure secure property binding:
Angular automatically sanitizes values before binding them to the DOM. This sanitization process ensures that potentially unsafe values (such as JavaScript code) are not executed by the browser. It uses a default policy to sanitize values but allows developers to specify custom sanitization strategies when necessary.
Implementation: Always trust values coming from the server or external sources. Use Angular's built-in sanitization mechanisms (DomSanitizer) to sanitize potentially unsafe values before binding them to the DOM. For example:
typescript
import { DomSanitizer, SafeUrl } from '@angular/platform-browser';
import { Component } from '@angular/core';
@Component({
selector: 'app-example',
template: `
<a [href]="safeUrl">Safe Link</a>
`
})
export class ExampleComponent {
unsafeUrl: string = 'javascript:alert("XSS attack!")';
safeUrl: SafeUrl;
constructor(private sanitizer: DomSanitizer) {
this.safeUrl = this.sanitizer.bypassSecurityTrustUrl(this.unsafeUrl);
}
}
Only bind user-controlled data directly to attributes or properties that can execute code, such as onclick, style, or href attributes, with proper sanitization.
Implement CSP headers to restrict sources from which certain types of content can be loaded. This can mitigate risks associated with injected scripts or malicious content.
Follow secure coding practices to minimize risks:
Property binding is a key feature in Angular that offers several advantages for developing dynamic and interactive web applications. It allows you to establish a connection between your component's TypeScript logic and the HTML template, enabling seamless data flow and UI updates based on changes in your application state.
We'll explore the significant benefits of property binding in Angular. We'll discuss how property binding simplifies development, enhances maintainability, promotes reusability, and supports a responsive user interface. By understanding these advantages, you'll be able to leverage property binding effectively to build robust and scalable Angular applications.
Property binding in Angular offers a range of practical use cases that enhance the dynamic behaviour and responsiveness of web applications. Here are some common scenarios where property binding is particularly useful:
Property binding enables dynamic rendering of content based on application state or user interactions. For example, conditionally displaying elements or changing their attributes based on data in the component.
Example: html
<button [disabled]="isButtonDisabled">Submit</button>
In this example, the disabled attribute of the button element is bound to the isButtonDisabled property in the component. When isButtonDisabled is true, the button becomes disabled; otherwise, it remains enabled.
You can use property binding to apply dynamic styles to elements based on component properties or conditions.
Example: html
<div [style.color]="isError ? 'red' : 'black'">
{{ errorMessage }}
</div>
Here, the text color of the <div> is dynamically set based on the isError property. If isError is true, the text color is red; otherwise, it's black.
Property binding is useful for conditionally showing or hiding elements based on specific conditions in your application logic.
Example: html
<p *ngIf="isLoggedIn">Welcome, {{ username }}!</p>
In this case, the <p> element is displayed only if the isLoggedIn property in the component is true.
Property binding allows you to dynamically set URLs for images, links, or other attributes of HTML elements.
Example: html
<img [src]="imageUrl">
Here, the src attribute of the <img> element is bound to the imageUrl property in the component. Changing imageUrl in the component will update the image displayed on the page.
Property binding can be combined with Angular's *ngFor directive to dynamically render lists of items based on data from the component.
Example: html
<ul>
<li *ngFor="let item of items">{{ item.name }}</li>
</ul>
In this example, the <li> elements are generated dynamically for each item in the items array in the component.
For form elements, property binding is used to bind input values and respond to changes made by users.
Example: html
<input type="text" [(ngModel)]="username">
Here, [(ngModel)] is a two-way data binding syntax that binds the username property in the component to the value of the < input > field. Changes to the username in the component are reflected in the input field, and changes made by the user update the username property in the component.
Property binding in Angular enables dynamic synchronization between a component's TypeScript code and its HTML templates. By using square brackets ([]), developers can bind component properties to HTML attributes or DOM properties, ensuring that changes in the component update the view automatically. This declarative approach simplifies development, reduces boilerplate code, and enhances application responsiveness.
Property binding is crucial for creating interactive user interfaces, supporting features like conditional rendering, dynamic styling, and seamless integration with Angular directives and forms. It leverages Angular's robust security mechanisms to mitigate risks associated with client-side vulnerabilities, making it a powerful tool for building modern and secure web applications.
Copy and paste below code to page Head section
Property binding in Angular is a mechanism that allows you to set values for HTML attributes or DOM properties dynamically based on data in your component. It uses square brackets ([]) to bind a component's property to an attribute or property of an HTML element in the template.
Interpolation ({{ }}) is one-way binding from the component to the view, where data is inserted into the HTML template as text. Property binding ([property]="value") allows you to set properties or attributes of HTML elements based on component data dynamically.
Use property binding when you need to dynamically update the attributes or properties of HTML elements based on changes in your component's data. It's ideal for scenarios such as conditional rendering, dynamic styling, input binding, and integrating with Angular directives and forms.
To bind to an HTML attribute, use the [attribute]="value" syntax. For example, <img [src]="imageUrl"> binds the src attribute of the <img> tag to the imageUrl property in the component.
Yes, property binding can bind to both HTML attributes and DOM properties. Use [property]="value" to bind to DOM properties like value, disabled, checked, etc., of HTML elements.
Angular automatically sanitizes values before binding them to the DOM, preventing XSS (Cross-Site Scripting) attacks by default. Developers can also use Angular's DomSanitizer service to sanitize potentially unsafe values before binding.