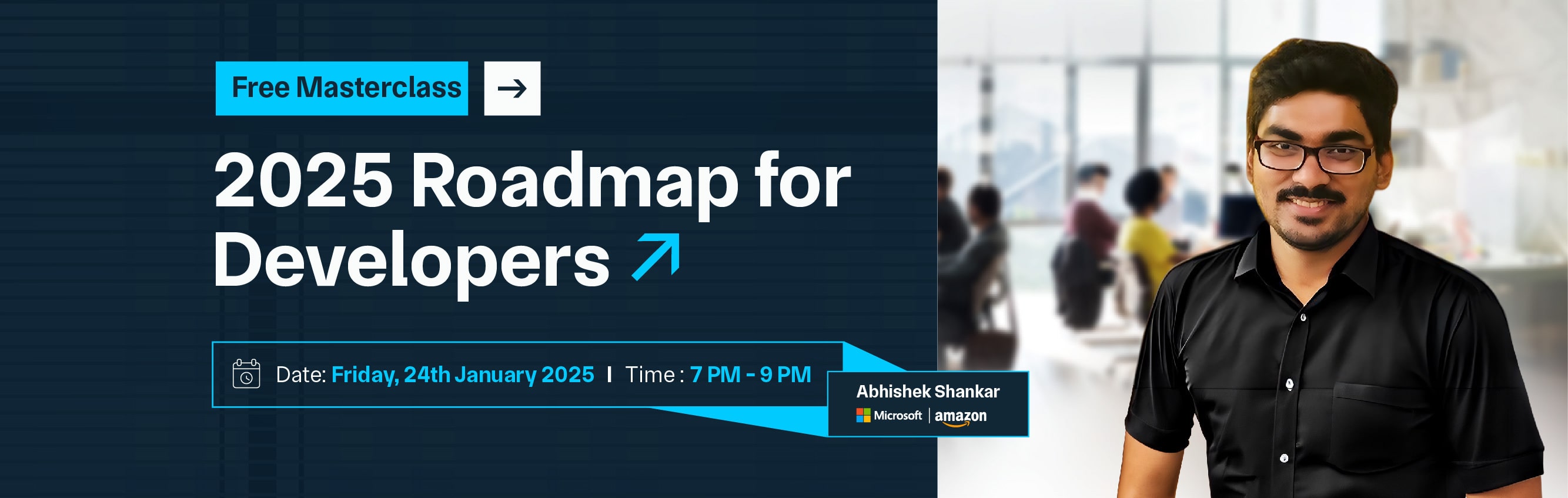

In Node.js, process.env is a powerful global object that provides access to environment variables within your application. These variables are key-value pairs that can store configuration settings, secrets, or any information that may vary between development, testing, and production environments. By using process.env, you can avoid hardcoding sensitive information like API keys, database URLs, or application secrets directly into your code, which enhances security and flexibility.
To use process.env, simply reference the variable you need, such as process.env.DB_URL for a database connection string. This allows you to set different values in different environments without changing your codebase. For instance, you can use a .env file alongside a library like dotenv to load environment variables during development.
Additionally, accessing environment variables through process.env makes your application more portable. When deploying to cloud services or containerized environments, you can configure environment variables directly in the hosting platform, ensuring that your app behaves consistently across various setups. Overall, leveraging process.env is essential for building scalable, secure, and maintainable Node.js applications.
process.env is a global object in Node.js that provides access to environment variables in your application. These variables are key-value pairs that store configuration settings, such as database connection strings, API keys, and other sensitive information.
By using process.env, you can configure your application without hardcoding values directly into your code. This approach enhances security and makes your application more flexible and portable, as you can easily change configurations based on the environment (development, testing, production) without modifying the source code.
For example, you can retrieve an environment variable with process.env.VARIABLE_NAME. To set these variables, you can use a .env file and a library like dotenv to load them during development or configure them directly in your deployment environment. Overall, process.env is essential for managing application settings effectively and securely.
process.env in Node.js is a global object that provides access to the environment variables of the current process. Environment variables are key-value pairs that allow you to configure your application without hardcoding sensitive information, such as API keys, database connection strings, and other settings that may differ between environments (like development, testing, and production).
Here are some key points about process.env:
Overall, process.env is a crucial feature in Node.js for managing application configurations securely and flexibly.
To access process.env in Node.js, follow these steps:
You can access environment variables using the process.env object.
Here's how:
const dbUrl = process.env.DB_URL;
console.log(`Database URL: ${dbUrl}`);
In this example, replace DB_URL with the name of your environment variable.
You can set environment variables directly when starting your application.
For example:
DB_URL='your_database_url' node app.js
1. Install the dotenv package:
If you haven't already, install the dotenv package:
npm install dotenv
2. Create a .env file:
Create a file named .env in your project root and define your variables:
DB_URL=your_database_url
API_KEY=your_api_key
3. Load the .env file in your application:
At the beginning of your main file (e.g., app.js), load the variables:
require('dotenv').config();
const dbUrl = process.env.DB_URL;
const apiKey = process.env.API_KEY;
console.log(`Database URL: ${dbUrl}`);
console.log(`API Key: ${apiKey}`);
Once you've set your environment variables, you can access them anywhere in your application using process.env.VARIABLE_NAME.
Default Values: If an environment variable is not set, accessing it will return undefined. You can provide default values like this:
const dbUrl = process.env.DB_URL || 'default_value';
By following these steps, you can effectively manage and access environment variables in your Node.js applications.
Setting environment variables is crucial for managing configuration in your applications. Here are various methods to set environment variables in Node.js:
You can set environment variables directly in your command line when starting your application. The syntax varies slightly between operating systems:
DB_URL='your_database_url' node app.js
set DB_URL=your_database_url && node app.js
$env:DB_URL='your_database_url'; node app.js
Using a .env file is a common and convenient way to manage environment variables, especially in development. Here's how to do it:
First, install the dotenv package:
npm install dotenv
Create a .env file in the root of your project and add your variables in the following format:
DB_URL=your_database_url
API_KEY=your_api_key
At the top of your main JavaScript file (e.g., app.js), load the environment variables:
require('dotenv').config();
// Access the variables
const dbUrl = process.env.DB_URL;
const apiKey = process.env.API_KEY;
console.log(`Database URL: ${dbUrl}`);
console.log(`API Key: ${apiKey}`);
When deploying your application, you often have options to set environment variables through your hosting service. Here are examples of some popular platforms:
Heroku: Use the Heroku CLI:
heroku config:set DB_URL=your_database_url
Docker: You can set environment variables in your Dockerfile or when running a container:
docker run -e DB_URL=your_database_url your_image
After setting the environment variables, you can access them anywhere in your application using process.env.VARIABLE_NAME.
For example:
const dbUrl = process.env.DB_URL || 'default_value';
Installing and managing environment variables in your application involves setting them up so that your application can access configuration values securely. Here’s how to do it effectively, especially in a Node.js environment:
One of the most common ways to manage environment variables in Node.js is by using the dotenv package. Here’s a step-by-step guide on how to install and use it:
You need to install the dotenv package using npm:
npm install dotenv
In the root of your project, create a file named .env. This file will store your environment variables in the key-value format:
DB_URL=your_database_url
API_KEY=your_api_key
PORT=3000
At the top of your main JavaScript file (e.g., app.js or server.js), load the environment variables from the .env file:
require('dotenv').config();
// Access the variables
const dbUrl = process.env.DB_URL;
const apiKey = process.env.API_KEY;
const port = process.env.PORT || 3000;
console.log(`Database URL: ${dbUrl}`);
console.log(`API Key: ${apiKey}`);
console.log(`Server running on port: ${port}`);
You can set environment variables directly in the command line before starting your Node.js application:
On Linux/Mac:
DB_URL='your_database_url' node app.js
On Windows (Command Prompt):
set DB_URL=your_database_url && node app.js
On Windows (PowerShell):
$env:DB_URL='your_database_url'; node app.js
When deploying your application, most cloud platforms allow you to set environment variables directly through their interfaces. Here are some examples:
Heroku:
heroku config:set DB_URL=your_database_url
Docker: Use the -e flag to set environment variables when running a container:
docker run -e DB_URL=your_database_url your_image
Once you've set up your environment variables, you can access them in your code using process.env:
const dbUrl = process.env.DB_URL;
console.log(`Connecting to database at: ${dbUrl}`);
Installing custom environment variables in your application can enhance its configurability and security. Here’s a comprehensive guide on how to set up and use custom environment variables in a Node.js application:
The dotenv package is a popular choice for managing environment variables. Here’s how to install and use it:
Use npm to install the dotenv package:
npm install dotenv
In the root of your project, create a file named .env. You can define your custom environment variables in this file:
# Custom environment variables
CUSTOM_VAR=your_custom_value
ANOTHER_VAR=another_value
In your main JavaScript file (e.g., app.js), load the environment variables at the top:
require('dotenv').config();
// Access your custom variables
const customVar = process.env.CUSTOM_VAR;
const anotherVar = process.env.ANOTHER_VAR;
console.log(`Custom Variable: ${customVar}`);
console.log(`Another Variable: ${anotherVar}`);
You can also set custom environment variables directly in the command line before starting your application:
CUSTOM_VAR='your_custom_value' node app.js
set CUSTOM_VAR=your_custom_value && node app.js
$env:CUSTOM_VAR='your_custom_value'; node app.js
To check if environment variables are installed and accessible in your Node.js application, you can follow these steps:
You can directly access and log the environment variables using process.env.
Here's a simple example:
require('dotenv').config(); // Load .env file if you are using it
// Check specific environment variables
const dbUrl = process.env.DB_URL;
const apiKey = process.env.API_KEY;
if (dbUrl) {
console.log(`Database URL is set: ${dbUrl}`);
} else {
console.log('Database URL is not set.');
}
if (apiKey) {
console.log(`API Key is set: ${apiKey}`);
} else {
console.log('API Key is not set.');
}
You can list all environment variables to see what is currently set. This can be helpful for debugging:
console.log('Environment Variables:');
console.log(process.env);
Initializing your first environment variable in a Node.js application is straightforward. Here’s a step-by-step guide to help you get started:
If you haven't already created a Node.js project, you can do so with the following commands:
mkdir my-node-app
cd my-node-app
npm init -y
To manage environment variables easily, it's common to use the dotenv package.
Install it using npm:
npm install dotenv
In the root directory of your project, create a file named .env. This file will contain your environment variables. For example, you can initialize a database URL like this:
# .env
DB_URL=your_database_url_here
In your main JavaScript file (e.g., app.js), load the environment variables at the top:
require('dotenv').config(); // Load .env variables
// Access the environment variable
const dbUrl = process.env.DB_URL;
// Log the variable to verify it works
console.log(`Database URL: ${dbUrl}`);
Now, you can run your application to see if the environment variable is loaded correctly:
node app.js
You should see the output in your terminal showing the database URL you set in the .env file:
Database URL: your_database_url_here
Using environment variables in Node.js is a best practice for managing configuration settings, especially for sensitive information like API keys, database connection strings, and other settings that may differ across environments (development, testing, production). Here’s how to effectively use environment variables in your Node.js applications:
If you don’t have a Node.js project yet, create one:
mkdir my-node-app
cd my-node-app
npm init -y
To manage environment variables easily, install the dotenv package:
npm install dotenv
In the root of your project, create a file named .env. This file will contain your environment variables:
# .env
DB_URL=your_database_url
API_KEY=your_api_key
PORT=3000
In your main JavaScript file (e.g., app.js), load the environment variables at the top:
require('dotenv').config(); // Load variables from .env
// Access your environment variables
const dbUrl = process.env.DB_URL;
const apiKey = process.env.API_KEY;
const port = process.env.PORT || 3000; // Default to 3000 if not set
console.log(`Database URL: ${dbUrl}`);
console.log(`API Key: ${apiKey}`);
console.log(`Server will run on port: ${port}`);
You can use environment variables throughout your application wherever necessary. For example, when connecting to a database or setting up a server:
const express = require('express');
const mongoose = require('mongoose');
const app = express();
// Connect to the database using the environment variable
mongoose.connect(dbUrl, { useNewUrlParser: true, useUnifiedTopology: true })
.then(() => console.log('Database connected'))
.catch(err => console.error('Database connection error:', err));
// Start the server
app.listen(port, () => {
console.log(`Server running on http://localhost:${port}`);
});
To run your application, use:
node app.js
You can log the environment variables to check if they are being accessed correctly:
console.log('Environment Variables:');
console.log(`DB_URL: ${process.env.DB_URL}`);
console.log(`API_KEY: ${process.env.API_KEY}`);
console.log(`PORT: ${process.env.PORT}`);
When using environment variables in Node.js applications, there are several common pitfalls that developers encounter. Here’s a guide to these pitfalls and how to avoid them:
Pitfall: Forgetting to load the environment variables from the .env file using dotenv.
Solution: Always include the line required ('dotenv').config(); at the top of your main JavaScript file. This ensures that your environment variables are loaded before you attempt to access them.
Pitfall: Hardcoding sensitive information, like API keys or database URLs, directly in your source code.
Solution: Use environment variables to store sensitive information. Always access these variables through process.env.
Pitfall: Accidentally committing your .env file to version control, exposing sensitive information.
Solution: Add the .env file to your .gitignore file to prevent it from being tracked by Git:
# .gitignore
.env
Pitfall: Not providing default values for environment variables can lead to runtime errors if they are not set.
Solution: Use a fallback value when accessing environment variables:
const port = process.env.PORT || 3000; // Default to 3000
Pitfall: Using inconsistent naming conventions for environment variables, leading to confusion and potential errors.
Solution: Establish a clear naming convention (e.g., all uppercase with underscores) and stick to it throughout your application. For example, use DB_URL, API_KEY, etc.
Using process.env in Node.js applications allows you to manage configuration settings flexibly and securely. Here are some common use cases for process.env:
Use Case: Storing database URLs or connection strings.
const mongoose = require('mongoose');
mongoose.connect(process.env.DB_URL, { useNewUrlParser: true, useUnifiedTopology: true })
.then(() => console.log('Database connected'))
.catch(err => console.error('Database connection error:', err));
Use Case: Keeping API keys and other sensitive information secure.
const axios = require('axios');
axios.get(`https://api.example.com/data?api_key=${process.env.API_KEY}`)
.then(response => {
console.log(response.data);
})
.catch(err => {
console.error('Error fetching data:', err);
});
Use Case: Configuring server settings like the port number.
const express = require('express');
const app = express();
const PORT = process.env.PORT || 3000; // Default to 3000 if not set
app.listen(PORT, () => {
console.log(`Server is running on http://localhost:${PORT}`);
});
Use Case: Differentiating behavior between development, testing, and production environments.
if (process.env.NODE_ENV === 'production') {
// Production settings
} else {
// Development settings
}
Use Case: Enabling or disabling features based on environment variables.
if (process.env.FEATURE_X_ENABLED === 'true') {
// Enable feature X
}
Use Case: Setting logging levels (e.g., info, debug, error) based on environment variables.
const logLevel = process.env.LOG_LEVEL || 'info'; // Default to 'info'
if (logLevel === 'debug') {
console.debug('This is a debug message');
}
Debugging environment variables in a Node.js application can be essential for identifying configuration issues and ensuring that your application behaves as expected. Here are some strategies and techniques to effectively debug environment variables:
One of the simplest ways to debug environment variables is to log them to the console. Be cautious not to log sensitive information, especially in production environments.
console.log('Environment Variables:');
console.log('DB_URL:', process.env.DB_URL);
console.log('API_KEY:', process.env.API_KEY);
Before using environment variables, ensure they are set. You can create a function to check for the required variables:
const requiredVars = ['DB_URL', 'API_KEY', 'PORT'];
requiredVars.forEach(varName => {
if (!process.env[varName]) {
console.error(`Error: Environment variable ${varName} is not set.`);
}
});
When accessing environment variables, provide default values to avoid runtime errors if a variable is not set:
const port = process.env.PORT || 3000; // Default to 3000 if not set
Consider using a library like envalid to validate and provide defaults for your environment variables. This helps catch errors early:
npm install envalid
Then use it in your application:
const { cleanEnv, str, num } = require('envalid');
const env = cleanEnv(process.env, {
DB_URL: str(),
API_KEY: str(),
PORT: num({ default: 3000 }),
});
console.log(`Connecting to database at: ${env.DB_URL}`);
Using environment variables in your applications offers several benefits that enhance security, flexibility, and maintainability. Here are some key advantages:
Environment variables protect sensitive information by storing it outside of the codebase. This minimizes the risk of exposing credentials like API keys or database passwords in version control systems.
By keeping such data confidential, you reduce potential vulnerabilities and unauthorized access to your applications.
Environment variables allow developers to configure applications for different environments (development, testing, production) without modifying the source code.
This flexibility enables teams to deploy the same codebase in various settings, ensuring that the application behaves correctly depending on the environment in which it's running.
By separating configuration from application logic, environment variables contribute to cleaner and more maintainable code.
This separation makes it easier to manage changes, as configuration can be adjusted without touching the core logic, reducing the risk of introducing bugs during updates or modifications.
Using environment variables ensures that applications behave consistently across different deployment environments.
This consistency is crucial for avoiding configuration-related issues that can arise when deploying to production. By standardizing configurations, teams can streamline the deployment process and reduce downtime.
Environment variables facilitate dynamic configuration changes without requiring a redeployment of the application.
This capability allows developers to tweak settings in real-time based on operational needs, such as adjusting API endpoints or enabling/disabling features, thereby enhancing the application's responsiveness to changing requirements.
Testing becomes more straightforward with environment variables, as developers can easily simulate different configurations by changing variable values.
This flexibility allows for comprehensive testing scenarios, enabling teams to validate application behavior under various conditions, which ultimately leads to better software quality and fewer bugs.
Understanding process.env in Node.js is crucial for managing configurations securely and efficiently. By utilizing environment variables, developers can enhance application security, maintain flexibility across environments, and streamline deployments. This practice promotes cleaner code and better collaboration, leading to more robust and maintainable applications.
Copy and paste below code to page Head section
process.env is an object in Node.js that provides access to environment variables for the current process. It allows you to manage configuration settings without hardcoding values into your application.
You can access environment variables using process.env.VARIABLE_NAME. For example, const dbUrl = process.env.DB_URL;.
If an environment variable is not set, accessing it via process.env will return undefined. It's good practice to provide default values or check for required variables before using them.
Yes, a .env file is a common way to store sensitive information. However, ensure this file is excluded from version control (by adding it to .gitignore) to protect sensitive data.
Yes, environment variable names are case-sensitive. For example, process.env.DB_URL and process.env.db_url would be treated as different variables.
While you can modify process.env properties at runtime, changes will only persist for the duration of the application’s execution. They won't affect the environmental variables outside of the process.