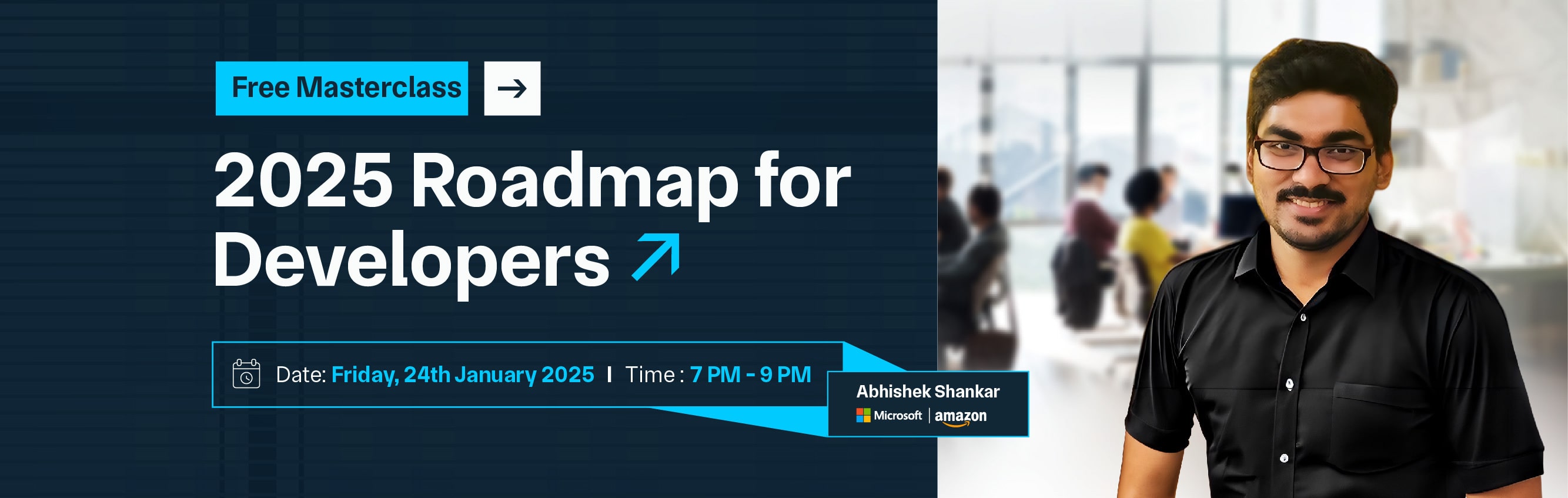

Polymorphism is a fundamental concept in object-oriented programming (OOP) that allows objects to be treated as instances of their parent class rather than their actual class. This capability enables a single function or method to operate differently based on the object it is acting upon. There are two primary types of polymorphism: compile-time (or static) and runtime (or dynamic).
Compile-time polymorphism is achieved through method overloading, where multiple methods with the same name but different parameters exist within a class. Runtime polymorphism is implemented through method overriding, where a subclass provides a specific implementation of a method that is already defined in its superclass. This means that the same method call can invoke different methods depending on the object’s actual type, which enhances flexibility and reusability in code.
For example, a base class Shape might have a method draw(), which is overridden in derived classes Circle and Square to draw different shapes. This approach allows for a more intuitive and scalable design, where new shapes can be added without modifying existing code, adhering to the Open/Closed Principle of software design. Polymorphism thus promotes code modularity and helps manage complex systems more effectively.
Polymorphism is a core concept in object-oriented programming (OOP) that refers to the ability of different objects to be treated as instances of the same class through a common interface.
It allows one function or method to perform different tasks based on the object it is operating on, thereby enabling a single function to be used with different types of objects.
There are two main types of polymorphism:
Polymorphism enhances flexibility and maintainability in code by allowing methods to use objects of different classes interchangeably. For example, a function designed to handle shapes can work with any shape object, such as circles or squares, without needing to know the specific class of the shape in advance. This leads to more modular and extensible code, facilitating easier updates and scalability.
Polymorphism in object-oriented programming (OOP) is primarily categorized into two types: compile-time polymorphism and runtime polymorphism. Here’s a closer look at each:
Method Overloading: This form of polymorphism occurs when multiple methods within the same class have the same name but different parameter lists (i.e., different types or numbers of parameters). The correct method is determined at compile time based on the method signature used in the call.
Example:
class Printer {
void print(int i) {
System.out.println("Printing integer: " + i);
}
void print(String s) {
System.out.println("Printing string: " + s);
}
}
In this example, the print method is overloaded to handle both integers and strings.
Method Overriding: This occurs when a subclass provides a specific implementation for a method that is already defined in its superclass. At runtime, the JVM determines the appropriate method to call based on the actual object type, not the reference type.
Example:
class Animal {
void makeSound() {
System.out.println("Animal makes a sound");
}
}
class Dog extends Animal {
@Override
void makeSound() {
System.out.println("Dog barks");
}
}
class Cat extends Animal {
@Override
void makeSound() {
System.out.println("Cat meows");
}
}
Here, both Dog and Cat override the makeSound method of the Animal class. At runtime, the method corresponding to the actual object type (e.g., Dog or Cat) is called.
Both types of polymorphism contribute to more flexible and maintainable code by allowing methods to operate on different types of objects or methods to share the same name with different implementations.
Compile-time polymorphism, also known as static polymorphism, is a type of polymorphism resolved during the compilation of a program. It allows a single function or method to have multiple forms based on its parameters. This is primarily achieved through method overloading.
Method Overloading is a feature in object-oriented programming that allows a class to have more than one method with the same name but different parameter lists. The method signature, which includes the method name and parameter types, must be unique for each overloaded method. Method overloading is resolved at compile time; hence it is a type of compile-time polymorphism.
class Display {
void show(int a) {
System.out.println("Integer: " + a);
}
void show(String s) {
System.out.println("String: " + s);
}
void show(double a, double b) {
System.out.println("Double: " + (a + b));
}
}
public class Main {
public static void main(String[] args) {
Display obj = new Display();
obj.show(5); // Calls show(int a)
obj.show("Hello"); // Calls show(String s)
obj.show(3.5, 2.5); // Calls show(double a, double b)
}
}
In this example, the show method is overloaded to handle different types and numbers of parameters.
Operator Overloading allows operators to be redefined and used with user-defined types (classes). This feature provides the ability to define custom behavior for operators such as +, -, *, and / when applied to objects of a class. Operator overloading is a form of runtime polymorphism.
#include <iostream>
using namespace std;
class Complex {
private:
float real;
float imag;
public:
Complex() : real(0), imag(0) {}
Complex(float r, float i) : real(r), imag(i) {}
// Overloading the + operator
Complex operator+(const Complex& c) {
return Complex(real + c.real, imag + c.imag);
}
void display() {
cout << real << " + " << imag << "i" << endl;
}
};
int main() {
Complex c1(1.5, 2.5), c2(3.5, 4.5);
Complex c3 = c1 + c2; // Calls overloaded + operator
c3.display(); // Output: 5.0 + 7.0i
return 0;
}
In this example, the + operator is overloaded for the Complex class to perform the addition of complex numbers. This makes it possible to use the + operator with objects of the Complex class in a way that is natural and intuitive.
Runtime Polymorphism (also known as dynamic polymorphism) is a key concept in object-oriented programming (OOP) that allows a method to perform different actions based on the actual object type that invokes it. Unlike compile-time polymorphism, which is resolved during compilation, runtime polymorphism is resolved during the execution of the program.
class Animal {
void makeSound() {
System.out.println("Animal makes a sound");
}
}
class Dog extends Animal {
@Override
void makeSound() {
System.out.println("Dog barks");
}
}
class Cat extends Animal {
@Override
void makeSound() {
System.out.println("Cat meows");
}
}
public class Main {
public static void main(String[] args) {
Animal myAnimal = new Dog(); // Reference type is Animal, object type is Dog
myAnimal.makeSound(); // Calls the overridden method in Dog
myAnimal = new Cat(); // Reference type is Animal, object type is Cat
myAnimal.makeSound(); // Calls the overridden method in Cat
}
}
In this example:
Runtime polymorphism enhances the capability of object-oriented systems by allowing objects to be treated in a more general way while enabling specific behavior to be executed based on the actual subclass of the object. This contributes to more dynamic and flexible software design.
Implementation of Polymorphism in object-oriented programming (OOP) involves using various techniques to enable objects to take on multiple forms. Here’s a detailed look at how polymorphism can be implemented, with examples in Java and C++.
Method Overloading: Method overloading is a common way to implement compile-time polymorphism. It allows multiple methods with the same name but different parameter lists within the same class. The compiler determines which method to call based on the method signature at compile time.
Java Example:
class MathOperations {
// Method to add two integers
int add(int a, int b) {
return a + b;
}
// Method to add three integers
int add(int a, int b, int c) {
return a + b + c;
}
// Method to add two doubles
double add(double a, double b) {
return a + b;
}
}
public class Main {
public static void main(String[] args) {
MathOperations math = new MathOperations();
System.out.println(math.add(5, 10)); // Calls add(int, int)
System.out.println(math.add(1, 2, 3)); // Calls add(int, int, int)
System.out.println(math.add(2.5, 3.5)); // Calls add(double, double)
}
}
C++ Example:
#include <iostream>
using namespace std;
class MathOperations {
public:
// Method to add two integers
int add(int a, int b) {
return a + b;
}
// Method to add three integers
int add(int a, int b, int c) {
return a + b + c;
}
// Method to add two doubles
double add(double a, double b) {
return a + b;
}
};
int main() {
MathOperations math;
cout << math.add(5, 10) << endl; // Calls add(int, int)
cout << math.add(1, 2, 3) << endl; // Calls add(int, int, int)
cout << math.add(2.5, 3.5) << endl; // Calls add(double, double)
return 0;
}
Method Overriding: Method overriding is used to achieve runtime polymorphism. A subclass provides a specific implementation of a method that is already defined in its superclass. The method that gets called is determined at runtime based on the actual object type.
Java Example:
class Animal {
void makeSound() {
System.out.println("Animal makes a sound");
}
}
class Dog extends Animal {
@Override
void makeSound() {
System.out.println("Dog barks");
}
}
class Cat extends Animal {
@Override
void makeSound() {
System.out.println("Cat meows");
}
}
public class Main {
public static void main(String[] args) {
Animal myAnimal = new Dog(); // Reference type is Animal, object type is Dog
myAnimal.makeSound(); // Calls the overridden method in Dog
myAnimal = new Cat(); // Reference type is Animal, object type is Cat
myAnimal.makeSound(); // Calls the overridden method in Cat
}
}
C++ Example:
#include <iostream>
using namespace std;
class Animal {
public:
virtual void makeSound() { // Virtual method
cout << "Animal makes a sound" << endl;
}
};
class Dog : public Animal {
public:
void makeSound() override { // Override the base class method
cout << "Dog barks" << endl;
}
};
class Cat : public Animal {
public:
void makeSound() override { // Override the base class method
cout << "Cat meows" << endl;
}
};
int main() {
Animal* myAnimal = new Dog(); // Reference type is Animal, object type is Dog
myAnimal->makeSound(); // Calls the overridden method in Dog
myAnimal = new Cat(); // Reference type is Animal, object type is Cat
myAnimal->makeSound(); // Calls the overridden method in Cat
delete myAnimal; // Clean up
return 0;
}
Polymorphism provides numerous benefits in object-oriented programming, contributing to more efficient, flexible, and maintainable code. Here’s a breakdown of the key advantages:
Polymorphism allows for writing generic code that can work with different types of objects. This means you can use the same interface or method for different data types or classes, which promotes code reuse. For example, a single method can handle multiple types of objects, reducing the need for redundant code.
Example: A draw method in a graphics application can be used to draw various shapes (e.g., circles, rectangles) without knowing their specific types.
Polymorphism enhances the flexibility of the code by allowing new classes to be added with minimal changes to existing code. You can introduce new subclasses and override methods without modifying the code that uses the base class. This makes it easier to extend and modify software systems.
Example: Adding a new shape class like Triangle to a graphics system that already supports Circle and Rectangles does not require changes to the drawing code.
With polymorphism, changes in the behavior of a subclass do not affect the client code that uses the superclass. This decoupling between interface and implementation simplifies maintenance and testing. You can update or enhance subclasses without altering the code that relies on the base class.
Example: Updating the implementation of Dog or Cat in a pet management system will not affect the code that interacts with the Animal class.
Polymorphism allows for a more organized and structured approach to coding. By using a common interface or base class, you can group related classes and methods, which makes the codebase easier to understand and manage.
Example: A common PaymentMethod interface in an e-commerce system can be implemented by various classes like CreditCard, PayPal, and BankTransfer, making payment processing modular and organized.
Polymorphism can simplify the code by reducing the need for conditional statements or type checks. Instead of writing multiple methods or conditionals to handle different types, polymorphism allows you to handle all cases through a common interface or base class method.
Example: A single method processPayment can handle different payment methods (credit card, PayPal, etc.) through polymorphism, avoiding complex conditionals based on the payment type.
Polymorphism supports encapsulation by hiding the specific implementation details of a class behind a common interface. Clients interact with objects through the interface without needing to know the underlying implementation.
Example: In a simulation system, users can interact with a Vehicle interface without knowing if the vehicle is a Car, Truck, or Motorcycle.
Runtime polymorphism allows for dynamic method binding, where the method that is executed is determined at runtime based on the actual object type. This dynamic behavior provides greater flexibility in managing object interactions.
Example: In a content management system, a Content reference might point to different types of content (e.g., Article, Video), and the appropriate rendering method is determined at runtime.
Polymorphism is a powerful concept in object-oriented programming (OOP), but it can lead to various pitfalls if not implemented carefully. Here are some common pitfalls and best practices to keep in mind:
1. Overuse of Polymorphism:
2. Ignoring the Liskov Substitution Principle:
3. Misuse of Method Overriding:
4. Performance Overheads:
5. Overloading vs. Overriding Confusion:
6. Lack of Documentation:
1. Adhere to SOLID Principles:
2. Use Abstract Classes and Interfaces Wisely:
3. Keep Methods Cohesive:
4. Prefer Composition over Inheritance:
5. Design for Extensibility:
6. Test Polymorphic Behavior:
7. Document Polymorphic Relationships:
Polymorphism is a versatile concept that applies to various real-world scenarios in software development. Here are some practical examples and use cases across different domains:
In graphics systems, polymorphism allows different shapes to be treated uniformly. You can use a common interface or base class for various shapes and then draw each shape using a single method call.
Example:
interface Shape {
void draw();
}
class Circle implements Shape {
@Override
public void draw() {
System.out.println("Drawing a circle");
}
}
class Rectangle implements Shape {
@Override
public void draw() {
System.out.println("Drawing a rectangle");
}
}
public class DrawingApp {
public static void main(String[] args) {
Shape shape1 = new Circle();
Shape shape2 = new Rectangle();
shape1.draw(); // Output: Drawing a circle
shape2.draw(); // Output: Drawing a rectangle
}
}
In payment systems, polymorphism can be used to handle different payment methods (credit cards, PayPal, bank transfers) using a common interface. This allows the system to process payments in a uniform way without needing to know the specifics of each payment method.
Example:
interface PaymentMethod {
void processPayment(double amount);
}
class CreditCard implements PaymentMethod {
@Override
public void processPayment(double amount) {
System.out.println("Processing credit card payment of $" + amount);
}
}
class PayPal implements PaymentMethod {
@Override
public void processPayment(double amount) {
System.out.println("Processing PayPal payment of $" + amount);
}
}
public class PaymentProcessor {
public static void main(String[] args) {
PaymentMethod payment1 = new CreditCard();
PaymentMethod payment2 = new PayPal();
payment1.processPayment(100.0); // Output: Processing credit card payment of $100.0
payment2.processPayment(200.0); // Output: Processing PayPal payment of $200.0
}
Polymorphism is a foundational concept in object-oriented programming, and its advanced topics delve deeper into how it interacts with other design principles and techniques. Here’s a look at some advanced topics related to polymorphism:
Covariance and contravariance are concepts related to type relationships in polymorphic scenarios, particularly when dealing with generic types and method parameters.
Covariance: Allows a method to return a type that is more derived than the type specified in the base class. For example, if Cat is a subclass of Animal, a method returning Animal can return Cat.
Java Example:
class Animal {}
class Cat extends Animal {}
class AnimalShelter {
Animal getAnimal() {
return new Cat(); // Covariant return type
}
}
Contravariance: Allows a method to accept parameters that are less derived than those specified in the base class. This is useful in scenarios where you are dealing with consumers of different types.
Java Example:
interface Consumer<T> {
void consume(T item);
}
class CatConsumer implements Consumer<Animal> {
@Override
public void consume(Animal item) {
// Implementation
}
}
Generic polymorphism allows classes and methods to operate on objects of various types while providing compile-time type safety. Generics enable polymorphic behavior without sacrificing type safety.
Java Example:
class Box<T> {
private T item;
void setItem(T item) {
this.item = item;
}
T getItem() {
return item;
}
}
public class Main {
public static void main(String[] args) {
Box<String> stringBox = new Box<>();
stringBox.setItem("Hello");
System.out.println(stringBox.getItem()); // Output: Hello
Box<Integer> intBox = new Box<>();
intBox.setItem(123);
System.out.println(intBox.getItem()); // Output: 123
}
}
Polymorphism is a key concept in many design patterns, which provide reusable solutions to common problems. Some notable design patterns that leverage polymorphism include:
Strategy Pattern: Defines a family of algorithms, encapsulates each one, and makes them interchangeable. The algorithm can vary independently from clients that use it.
Example:
interface SortingStrategy {
void sort(int[] array);
}
class QuickSort implements SortingStrategy {
@Override
public void sort(int[] array) {
// QuickSort implementation
}
}
class MergeSort implements SortingStrategy {
@Override
public void sort(int[] array) {
// MergeSort implementation
}
}
class Sorter {
private SortingStrategy strategy;
void setStrategy(SortingStrategy strategy) {
this.strategy = strategy;
}
void sortArray(int[] array) {
strategy.sort(array);
}
}
Factory Pattern: Defines an interface for creating objects but allows subclasses to alter the type of objects that will be created. Polymorphism is used to create different types of objects based on the input.
Example:
interface Product {
void use();
}
class ConcreteProductA implements Product {
@Override
public void use() {
// Use product A
}
}
class ConcreteProductB implements Product {
@Override
public void use() {
// Use product B
}
}
abstract class ProductFactory {
abstract Product createProduct();
}
class ProductAFactory extends ProductFactory {
@Override
Product createProduct() {
return new ConcreteProductA();
}
}
class ProductBFactory extends ProductFactory {
@Override
Product createProduct() {
return new ConcreteProductB();
}
}
In dynamically typed languages, polymorphism can be achieved through duck typing, where the type of an object is determined by its behavior rather than its explicit class. This is prevalent in languages like Python and Ruby.
Python Example:
class Dog:
def speak(self):
return "Woof"
class Cat:
def speak(self):
return "Meow"
def make_animal_speak(animal):
print(animal.speak())
dog = Dog()
cat = Cat()
make_animal_speak(dog) # Output: Woof
make_animal_speak(cat) # Output: Meow
**5. Polymorphism in Functional Programming
While traditionally associated with object-oriented programming, polymorphism also appears in functional programming through concepts like higher-order functions and type classes.
Higher-order Functions: Functions that take other functions as arguments or return them as results.
Example in Haskell:
haskell
Copy code
add :: Int -> Int -> Int
add x y = x + y
applyFunction :: (Int -> Int -> Int) -> Int -> Int -> Int
applyFunction f x y = f x y
result = applyFunction add 5 10 -- Output: 15
Type Classes (in Haskell): Define a set of functions that can be implemented by various types.
Example in Haskell:
haskell
Copy code
class Eq a where
(==) :: a -> a -> Bool
instance Eq Int where
x == y = x `Prelude.eq` y
instance Eq String where
x == y = x `Prelude.eq` y
**6. Virtual Functions and Method Tables
In languages like C++, polymorphism is implemented using virtual functions and method tables (vtables). Each class has a vtable that holds pointers to its virtual functions, allowing dynamic method dispatch.
C++ Example:
cpp
Copy code
#include <iostream>
using namespace std;
class Base {
public:
virtual void show() {
cout << "Base class show" << endl;
}
};
class Derived : public Base {
public:
void show() override {
cout << "Derived class show" << endl;
}
};
int main() {
Base* obj = new Derived();
obj->show(); // Output: Derived class show
delete obj;
return 0;
}
Polymorphism is a fundamental concept in object-oriented programming that significantly enhances flexibility, code reusability, and maintainability. By allowing objects to be treated as instances of their base class rather than their actual class, polymorphism enables developers to design systems that can adapt to changes with minimal impact on existing code. This flexibility is achieved through compile-time polymorphism, such as method and operator overloading, and runtime polymorphism via method overriding.
While polymorphism brings numerous benefits, including improved code organization, reduced complexity, and the ability to extend functionalities easily, it also presents challenges like potential performance overheads and the risk of complex code structures. Adhering to best practices, such as following SOLID principles and ensuring proper documentation, helps mitigate these risks. Advanced topics, including covariant and contravariant types, generic polymorphism, and the application of polymorphism in design patterns, further extend its utility. Overall, a deep understanding of polymorphism and its nuances allows developers to create adaptable, scalable, and robust software systems, making it an essential aspect of modern software engineering.
Copy and paste below code to page Head section
Polymorphism is a concept in object-oriented programming that allows objects to be treated as instances of their base class rather than their actual class. This enables a single interface to be used for different underlying data types or classes. Polymorphism allows methods to do different things based on the object they are acting upon, which is crucial for writing flexible and reusable code.
There are two main types of polymorphism: Compile-Time Polymorphism (Static Polymorphism): Achieved through method overloading and operator overloading. The method or operator to be invoked is determined at compile time. Runtime Polymorphism (Dynamic Polymorphism): Achieved through method overriding. The method to be executed is determined at runtime based on the actual object type.
Method Overloading occurs when multiple methods have the same name but different parameter lists (different number or types of parameters) within the same class. This is a form of compile-time polymorphism. Method Overriding occurs when a subclass provides a specific implementation of a method that is already defined in its superclass. This is a form of runtime polymorphism.
The Liskov Substitution Principle (LSP) is one of the SOLID principles and states that objects of a superclass should be replaceable with objects of a subclass without affecting the correctness of the program. It is important for polymorphism because it ensures that subclasses can stand in for their base classes without causing unexpected behavior or errors.
Runtime polymorphism, particularly through virtual function calls, can introduce performance overhead due to dynamic method dispatch. While this overhead is generally small, it is important to be aware of it in performance-critical applications. Compile-time polymorphism, like method overloading, does not incur this runtime cost.
In dynamically typed languages, polymorphism often relies on duck typing, where the type of an object is determined by its behavior (methods and properties) rather than its explicit class. This allows objects to be used interchangeably if they implement the required methods or properties.