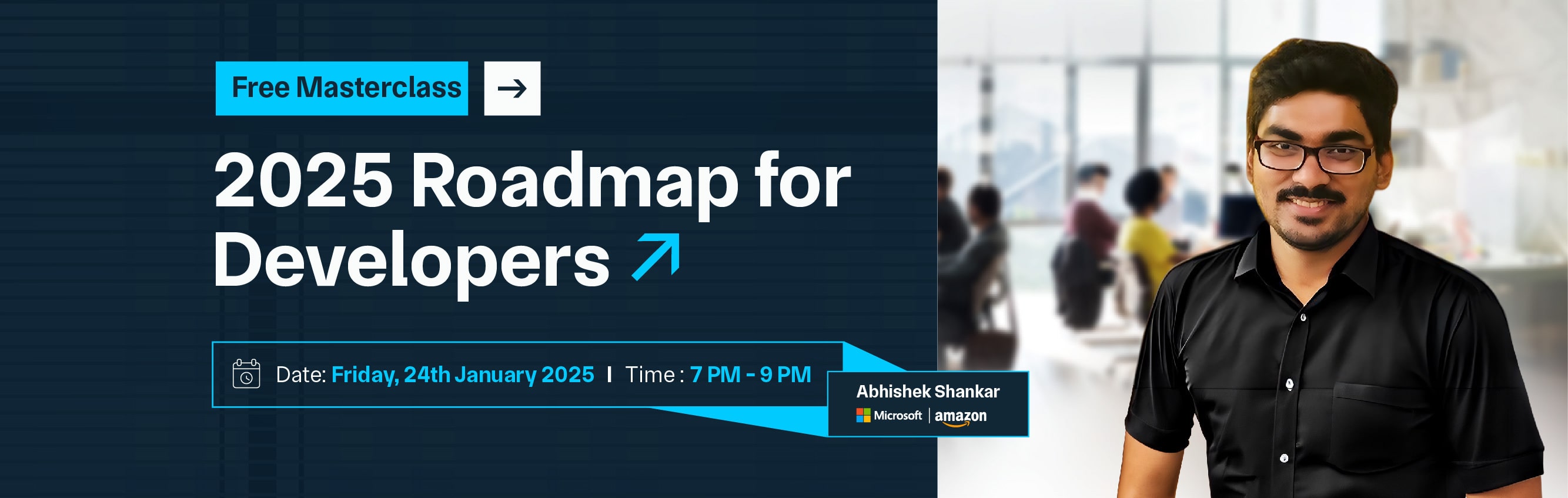

Node.js projects with readily available source code are invaluable resources for developers looking to deepen their understanding and proficiency in server-side JavaScript development. These projects span a wide range of applications and complexities, offering practical examples that showcase Node.js's capabilities in various domains. For instance, building RESTful APIs using Node.js and Express.js demonstrates how to create endpoints for handling CRUD (Create, Read, Update, Delete) operations efficiently. This is essential for developing scalable backend systems that communicate with client applications via HTTP requests.
Real-time applications, such as chat applications utilising WebSocket technology with libraries like Socket.IO, highlight Node.js's event-driven nature and its ability to handle simultaneous connections and real-time data exchange seamlessly. E-commerce websites built with Node.js showcase integration with databases like MongoDB or MySQL for storing product information, managing user sessions, and implementing secure payment gateways (e.g., Stripe or PayPal), thereby demonstrating best practices in transactional web development.
Moreover, projects like blogging platforms or weather applications illustrate fetching data from external APIs, processing it asynchronously, and presenting it dynamically to users through frontend frameworks such as React or Angular. By exploring and dissecting these projects' source code, developers not only gain hands-on experience in Node.js but also acquire insights into building robust, scalable, and efficient applications that meet modern web development standards and user expectations.
Node.js is a runtime environment that allows you to run JavaScript code outside of a web browser. It uses the Chrome V8 JavaScript engine to execute code on the server-side, enabling developers to build scalable network applications and backend services using JavaScript.
Key features of Node.js include:
1. Non-blocking, Asynchronous I/O: Node.js is designed to handle many concurrent connections with minimal overhead by using an event-driven, non-blocking I/O model. This makes it highly efficient for real-time applications.
2. JavaScript Everywhere: With Node.js, JavaScript can be used for both client-side and server-side programming, providing a unified language and ecosystem across the stack.
3. NPM (Node Package Manager): Node.js comes with npm, a package manager that allows developers to share and reuse code easily. npm hosts the largest ecosystem of open-source libraries in the world, making it straightforward to integrate third-party packages into your applications.
4. Scalability: Node.js applications are known for their ability to handle a large number of simultaneous connections efficiently, making it suitable for building highly scalable applications.
5. Single-threaded, Event-driven: Node.js operates on a single-threaded event loop, handling multiple client requests concurrently without creating new threads for every request. This approach reduces memory consumption and increases application performance.
Node.js has become a popular choice for building server-side applications due to its performance, scalability, and the extensive JavaScript ecosystem available through npm.
Node.js is particularly suitable for certain types of applications and scenarios. Here are some common use cases where Node.js shines:
However, Node.js might not be the best choice for CPU-intensive tasks or applications that require extensive computation, as it operates on a single-threaded event loop. For such scenarios, other languages like Python, Java, or C# might be more suitable depending on the specific requirements.
Here are 20 project ideas using Node.js with brief examples.
1. Todo List App: Create a web application to manage tasks.
2. Blog Platform: Build a platform for creating and managing blogs.
3. Chat Application: Develop a real-time chat application using WebSockets.
4. E-commerce Website: Create an online store with shopping cart functionality.
5. Weather App: Fetch weather data from an API and display it.
6. Authentication System: Implement user authentication using JWT or OAuth.
7. URL Shortener: Create a service to shorten URLs.
8. File Uploader: Build a service to upload and manage files.
9. Real-time Analytics Dashboard: Display real-time analytics data using WebSockets or SSE.
10. Payment Gateway Integration: Integrate with a payment gateway like Stripe or PayPal.
11. Social Media Dashboard: Aggregate and display social media posts from different platforms.
12. Job Board: Create a platform for posting and searching job listings.
13. Video Streaming App: Develop a service to stream video content.
14. Collaborative Code Editor: Build a real-time code editor that multiple users can edit simultaneously.
15. Quiz or Polling App: Create an interactive quiz or polling application.
16. Appointment Scheduler: Build a service for scheduling appointments.
17. Content Management System (CMS): Develop a custom CMS for managing website content.
18. Recipe Sharing Platform: Create a platform for sharing and discovering recipes.
19. Online Forum: Build a forum for discussions on various topics.
20. Fitness Tracker: Develop an application to track fitness activities and goals.
These projects cover a range of applications and can be tailored to different levels of complexity based on your skills and interests.
Explore Node.js projects with source code to learn and build real-world applications. Enhance your skills and discover practical examples for development.
A Todo List App built with Node.js could use frameworks like Express.js for server-side logic, MongoDB or MySQL for database storage, and a frontend framework like React or Vue.js for a responsive user interface.
Authentication can be implemented to allow users to log in and access their tasks securely. CRUD operations (Create, Read, Update, Delete) are implemented to manage tasks effectively, providing users with a simple yet powerful tool for task management.
Source Code: Click Here
A web application where users can create, manage, and prioritize their tasks. Users can add new tasks, mark them as complete, set deadlines, and organize tasks into different categories or projects.
Using Node.js and Express.js, you can build a backend that handles CRUD operations for blog posts and user authentication. MongoDB, or a relational database, can store blog data, while frontend frameworks like React or Angular can provide a dynamic user interface.
RESTful APIs can manage interactions between the front end and backend, enabling users to create, edit, and delete blog posts. Authentication and authorisation mechanisms ensure that only authorised users can publish posts or comment on them, making it a comprehensive solution for blogging needs.
Source Code: Click Here
A platform where users can create, publish, and manage blog posts. Features include rich text editing, tagging, commenting, and user profiles.
Built using Node.js with Socket.IO for real-time communication, this chat application supports features like private messaging, typing indicators, and message history. Express.js can handle HTTP routes for authentication and room creation. MongoDB or another database can store user information and chat history.
WebSocket connections enable real-time updates across clients, ensuring a seamless chat experience. With responsive design using CSS frameworks like Bootstrap or Materialize, the application provides an intuitive interface for users to interact and communicate effortlessly in real-time.
Source Code: Click Here
A real-time chat application where users can create chat rooms, send messages, and receive instant updates.
Node.js powers the backend of this e-commerce website, handling product listings, cart management, and order processing. Express.js manages HTTP routes for user authentication, product browsing, and payment processing via integrations with payment gateways like Stripe or PayPal.
MongoDB or a relational database stores product data and user information securely. Frontend frameworks like React or Angular provide a responsive, interactive shopping experience. Features include search functionality, product reviews, and order tracking, ensuring a robust and user-friendly e-commerce platform suitable for various product categories.
Source Code: Click Here
An online store allowing users to browse products, add them to a cart, and complete purchases securely.
Using Node.js, the app can make API requests to services like OpenWeatherMap or Weatherstack to retrieve weather information such as temperature, humidity, wind speed, and conditions. Express.js can handle backend logic, including API routing and data processing.
Frontend frameworks like React or Vue.js can render weather data dynamically, presenting it in a visually appealing format. User experience can be enhanced with geolocation features for automatic weather updates based on the user's location. This application provides a practical tool for users to stay informed about weather conditions wherever they are.
Source Code: Click Here
A weather application that fetches current weather data based on user location or search input and displays it along with forecasts.
Node.js can implement robust authentication systems where users can sign up, log in, and manage their accounts securely. With JWT, users receive tokens upon successful authentication, which are used for subsequent authorised requests. OAuth allows users to log in using existing accounts from providers like Google or Facebook, enhancing convenience and security.
Express.js can manage authentication routes and middleware to verify tokens or authenticate users via OAuth protocols. MongoDB or another database stores user credentials and session information securely. This setup ensures that applications handle user authentication effectively while protecting sensitive data.
Source Code: Click Here
An authentication system for securing access to web applications using JWT (JSON Web Tokens) or OAuth (Open Authorization).
Node.js can create a URL shortening service where users input long URLs, and the server generates a unique short URL using algorithms like Base62 encoding. Express.js handles HTTP routes for receiving long URLs, generating short URLs, and redirecting users to the original URLs.
MongoDB or a database stores mappings between short URLs and original URLs, ensuring efficient lookup and redirection. Frontend elements can enhance usability with copy-to-clipboard functionality and statistics on shortened URLs. This service provides a practical solution for sharing and managing long URLs effectively across various platforms.
Source Code: Click Here
A service that generates shortened URLs from long URLs and redirects users to the original URL when accessed.
Node.js facilitates a file uploader application where users can select files from their devices, upload them to the server, and manage uploads with features like progress indicators and file type validation. Express.js handles file upload routes, ensuring secure file storage and access permissions.
MongoDB, or a database, stores metadata about uploaded files, including file names, sizes, and upload timestamps. Frontend frameworks like React or Angular can display upload status and provide options for file management, such as renaming or deleting files. This application caters to diverse needs for securely handling file uploads in web-based environments.
Source Code: Click Here
A file uploading service where users can upload files, manage uploads, and share them securely.
Node.js enables the creation of a real-time analytics dashboard where data from various sources (e.g., databases, APIs) is continuously streamed to clients using WebSockets for bidirectional communication or SSE for server-to-client updates. Express.js manages backend routes for data retrieval and processing, ensuring efficient handling and aggregation of analytics data.
Frontend frameworks like React or Angular can display dynamic charts, graphs, and metrics in real-time, providing users with actionable insights into their data. This application is crucial for businesses to monitor performance metrics and make data-driven decisions promptly.
Source Code: Click Here
A dashboard that visualises real-time analytics data such as website traffic, user interactions, and sales metrics using WebSockets or Server-Sent Events (SSE).
Node.js facilitates seamless integration of payment gateways by handling HTTP requests and responses necessary for processing payments securely. Express.js manages routes for initiating payment requests, handling callbacks, and updating transaction statuses. Integration with Stripe or PayPal SDKs allows for tokenisation of sensitive payment information and ensures PCI DSS compliance.
Frontend elements can include forms for entering payment details and confirmation messages for successful transactions. This setup enables businesses to accept payments online safely, providing customers with a smooth checkout experience and enhancing trust and security.
Source Code: Click Here
Integrating a payment gateway like Stripe or PayPal into an e-commerce website for secure.
Using Node.js, a social media dashboard can gather data via APIs from multiple platforms, such as Twitter's API for tweets and Facebook's API for posts and engagement metrics.
Express.js manages backend routes for fetching and processing social media data, ensuring data synchronisation and real-time updates. Frontend frameworks like React or Vue.js can visualise aggregated posts, engagement statistics, and trends across platforms. Features may include scheduling posts, monitoring hashtags, and analysing audience demographics.
Source Code: Click Here
A dashboard that aggregates and displays social media posts, interactions, and analytics from different platforms (e.g., Twitter, Facebook, Instagram).
Using Node.js, you can build a backend that manages job listings, user accounts, and application processes. Express.js handles HTTP routes for creating, updating, and deleting job postings, as well as managing user authentication and authorization. MongoDB or a relational database stores job data, user profiles, and application details securely.
Frontend frameworks like React or Angular can provide a user-friendly interface for job seekers to search for jobs based on criteria like location and industry, submit applications, and receive notifications. Employers can manage job postings, review applications, and communicate with candidates through the platform.
Source Code: Click Here
A job board platform where employers can post job openings and job seekers can search and apply for jobs.
Node.js facilitates backend development for a video streaming app, handling functionalities such as video storage, transcoding, and streaming. Express.js manages routes for video uploads, playback requests, and user interactions.
Cloud storage services like AWS S3 or Firebase Storage store video files securely, while services like FFmpeg can be used for video transcoding to ensure compatibility across devices and bandwidths. Frontend frameworks like React Native for mobile or React/Vue.js for the web can deliver a seamless user experience with features like video playback controls, playlists, and social sharing capabilities.
Source Code: Click Here
A video streaming service that allows users to upload, watch, and share video content.
Node.js powers a collaborative code editor with WebSocket or Socket.IO for real-time communication. Express.js manages backend routes for user authentication, code synchronization, and version control. MongoDB or another database stores user sessions, code snippets, and collaboration history.
Frontend frameworks like React or Vue.js can render a code editor with syntax highlighting, code completion, and collaborative features such as cursor tracking and chat. This setup enables developers to work together remotely on coding projects, enhancing productivity and enabling seamless code reviews and pair programming sessions.
Source Code: Click Here
A real-time code editor where multiple users can write and edit code simultaneously, with instant updates.
Node.js can develop a backend for a quiz or polling app, handling quiz creation, participant responses, and result aggregation. Express.js manages HTTP routes for quiz creation, submission handling, and real-time result updates using technologies like WebSocket or Server-Sent Events (SSE).
MongoDB or a database stores quiz questions, participant responses, and analytics data securely. Frontend frameworks like React or Angular can render dynamic quiz interfaces with features such as multiple-choice questions, timers, and instant feedback on answers. This application caters to educational, marketing, or engagement purposes, offering interactive experiences for users.
Source Code: Click Here
An interactive application for creating and conducting quizzes or polls, with real-time results.
Node.js facilitates backend development for an appointment scheduling service, managing user accounts, appointment calendars, and notifications. Express.js handles routes for appointment creation, scheduling conflicts, and user authentication. MongoDB or a database stores appointment details, user preferences, and availability data securely.
Frontend frameworks like React or Vue.js can provide a calendar interface for users to select available time slots, confirm appointments, and receive reminders via email or SMS. Admin panels can manage schedules, handle cancellations or rescheduling requests, and generate reports. This service streamlines appointment booking processes for various industries, improving customer convenience and operational efficiency.
Source Code: Click Here
A service that allows users to schedule appointments with businesses or professionals.
Node.js can power the backend of a CMS, managing content storage, user roles, and permissions. Express.js handles HTTP routes for content creation, editing, and publishing. MongoDB, or a relational database, stores structured content data, user profiles, and settings.
Frontend frameworks like React or Angular can provide a responsive dashboard for content management featuring WYSIWYG editors, media libraries, and SEO tools. Authentication mechanisms ensure secure access to content management functionalities. This CMS empowers businesses and individuals to maintain dynamic and scalable websites with ease.
Source Code: Click Here
A custom CMS that allows users to create, edit, and manage website content such as articles, pages, and media.
Node.js facilitates backend development for a recipe-sharing platform, handling user accounts, recipe creation, and search functionalities. Express.js manages API routes for recipe CRUD operations, user authentication, and search queries. MongoDB or another database stores recipe data, user profiles, and interaction metrics securely.
Frontend frameworks like React or Vue.js can render recipe cards, search filters, and social features such as liking and sharing recipes. Integration with external APIs for nutritional information or meal planning enhances user experience. This platform serves culinary enthusiasts and professionals seeking inspiration and community engagement around cooking.
Source Code: Click Here
A platform where users can discover, share, and save recipes, with features for ratings, reviews, and ingredient lists.
Node.js can develop a backend for an online forum, managing user accounts, threads, posts, and notifications. Express.js handles HTTP routes for forum interactions, including thread creation, post replies, and user authentication. MongoDB or a relational database stores forum data, user profiles, and thread metadata securely.
Frontend frameworks like React or Angular can provide a responsive forum interface with features like threaded discussions, voting, and moderation tools. Real-time updates using WebSocket or SSE enhance user engagement by notifying users of new posts and replies. This platform fosters community-driven discussions and knowledge sharing across diverse topics.
Source Code: Click Here
A forum platform where users can discuss various topics, post questions, and engage in conversations.
Node.js facilitates backend development for a fitness-tracking application, managing user accounts, activity logs, and goal tracking. Express.js handles API routes for recording workouts, setting goals, and user authentication. MongoDB or another database stores fitness data, user profiles, and progress metrics securely.
Frontend frameworks like React Native for mobile or React/Vue.js for the web can render activity logs, goal progress charts, and personalised fitness plans. Integration with wearable devices or fitness APIs allows automatic data syncing, enhancing accuracy and usability. This application motivates users to achieve fitness goals through data-driven insights and personalised recommendations.
Source Code: Click Here
An application that tracks fitness activities sets goals, and monitors progress over time.
Working on Node. js-based projects offers several compelling reasons for developers and organisations alike:
Overall, working on Node.js based projects not only offers technical advantages but also aligns with modern software development trends and enhances career prospects in the software industry.
Learning development with Node.js effectively involves several key strategies to maximise your understanding and proficiency. Here’s a structured approach to learning Node.js:
By following these steps, you can effectively learn Node.js in a structured manner, gradually building your skills and confidence to develop robust and scalable applications using Node.js.
Node.js provides a powerful and versatile platform for building modern, scalable, and high-performance applications, making it a popular choice among developers and organizations alike.
Welcome to Fynd. academy, your premier destination for professional online courses designed to enhance your career prospects and skills. At Fynd.academy, we believe in empowering students and professionals alike with the knowledge they need to succeed in today's competitive world.
Our mission at Fynd.academy is to provide accessible, high-quality education that is flexible and fits into your busy lifestyle. Whether you're looking to advance in your current career or explore a new field altogether, our curated courses cover a wide range of industries and disciplines.
At Fynd.academy, we are committed to your success. Our interactive learning platform, combined with personalised support from instructors, ensures that you receive a rewarding educational experience.
Join the Fynd.academy community today and take the next step towards achieving your career aspirations. Explore our courses, enroll with confidence, and embark on a journey of professional growth with us.
Node.js stands out as a powerful and versatile platform for modern web and server-side development. Its advantages include using JavaScript across the full stack, scalability for handling concurrent connections efficiently, a rich ecosystem of libraries and packages through npm, and robust support for real-time applications and microservices architectures. Node.js's asynchronous, non-blocking I/O model enables developers to build fast and responsive applications, suitable for today's demanding web and mobile environments.
Its cross-platform compatibility, strong community support, and extensive tooling further enhance its appeal. By learning Node.js, developers gain proficiency in a technology that is widely adopted by tech giants and startups alike, offering ample career opportunities and the ability to build scalable, high-performance applications. Whether you're building APIs, real-time applications, microservices, or full-stack web applications, Node.js provides the tools and ecosystem to support your development needs effectively.
Copy and paste below code to page Head section
Node.js is a runtime environment that allows you to run JavaScript code outside of a web browser. It uses the Chrome V8 JavaScript engine and is designed to be lightweight and efficient for building scalable network applications and backend services.
Node.js offers several advantages: JavaScript Everywhere: Allows you to use JavaScript for both frontend and backend development. Scalability: Handles a large number of simultaneous connections efficiently. Rich Ecosystem: Extensive library of open-source packages through npm. Asynchronous and Non-blocking: Supports handling concurrent operations without blocking the event loop. Real-time Applications: Ideal for building real-time applications like chat apps and streaming platforms.
You can build a variety of applications with Node.js, including: APIs and microservices Real-time web applications (chat apps, live tracking) Single-page applications (SPAs) Command-line tools IoT (Internet of Things) applications Data streaming applications
While Node.js is primarily used for backend development, it can also be used for frontend development (e.g., with frameworks like Electron for building desktop applications) and full-stack development. Its versatility allows developers to use JavaScript across different layers of the application.
Node.js uses an event-driven, non-blocking I/O model to handle asynchronous operations. It uses callbacks, promises, and async/await to manage asynchronous tasks efficiently without blocking the execution thread, which is crucial for handling multiple concurrent requests.
Yes, Node.js is suitable for building large-scale applications due to its scalability, asynchronous nature, and robust ecosystem. Many large companies use Node.js for their backend services, demonstrating its ability to handle complex and high-traffic applications effectively.