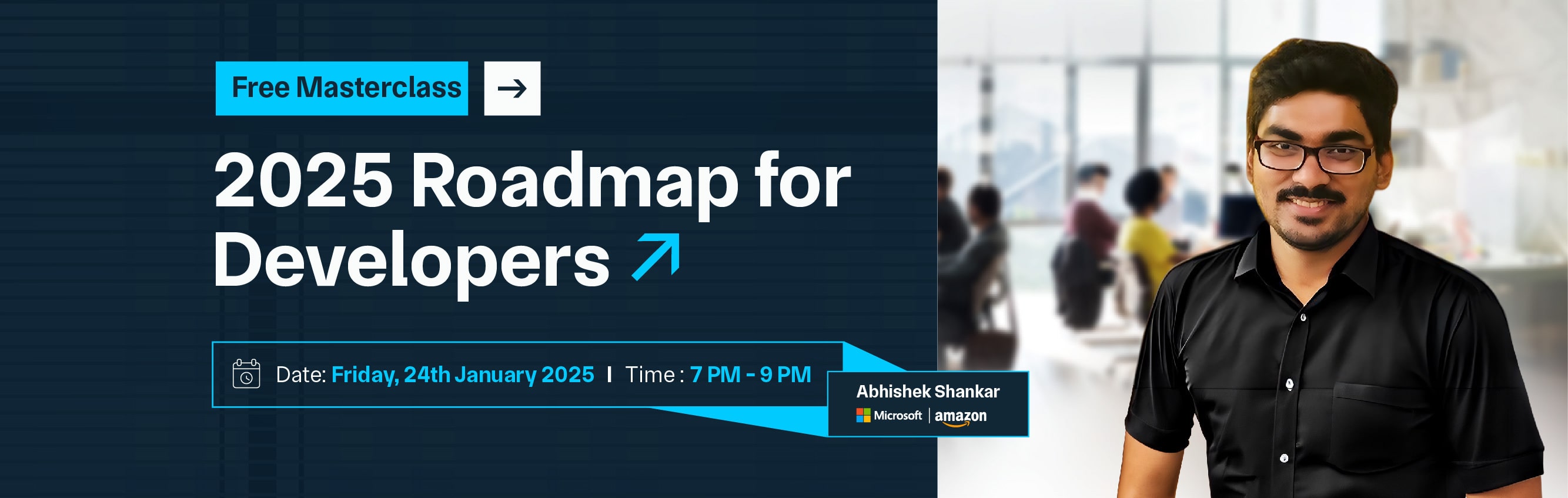

ngModel is a crucial directive in Angular that enables two-way data binding, allowing seamless interaction between the model and the view in forms. By utilizing ngModel, developers can easily synchronize user input with the underlying application data, enhancing the user experience. To implement ngModel, the FormsModule must be imported into the Angular module.
This directive is typically used in template-driven forms, where it simplifies the management of form controls. For instance, an input field can be bound to a component property, so any changes in the input reflect immediately in the component's state and vice versa. This real-time binding facilitates dynamic UI updates and efficient handling of user input. Additionally, ngModel supports validation, making it easier to ensure data integrity.
While using ngModel, it's essential to assign a name attribute to each input to enable proper form control tracking. However, developers should be mindful of naming conflicts and ensure that properties do not overlap with form controls. Overall, ngModel streamlines form handling in Angular applications, contributing to cleaner code and improved responsiveness in user interfaces.
ngModel is a directive in Angular that enables two-way data binding between the model and the view, primarily used in forms. It allows you to synchronize the data between an input field (or any form of control) and a property in the component class. This means that when the user enters data into an input field, the corresponding property in the component is automatically updated, and any changes to that property will also reflect in the input field. To use ngModel, you need to import the FormsModule into your Angular module.
The syntax for ngModel involves using square brackets and parentheses: [(ngModel)]. This notation indicates two-way binding, allowing for real-time updates. ngModel is particularly beneficial in template-driven forms, simplifying tasks such as form validation and data submission. It also facilitates dynamic UI changes, making applications more responsive to user input. Overall, ngModel plays a vital role in enhancing the user experience by ensuring that the data flow between the UI and the application logic is smooth and efficient.
To use ngModel in Angular, follow these steps:
First, ensure that you import the FormsModule in your Angular module. This is essential for using ngModel.
import { FormsModule } from '@angular/forms';
@NgModule({
imports: [FormsModule],
declarations: [/* your components */],
bootstrap: [/* your main component */]
})
export class AppModule {}
In your component, define a property that will be bound to the input field. For example:
import { Component } from '@angular/core';
@Component({
selector: 'app-root,'
templateUrl: './app.component.html'
})
export class AppComponent {
userName: string = '';
}
In the template (HTML file), use ngModel to bind the input field to the component property. The syntax for two-way data binding involves square brackets and parentheses: [(ngModel)].
<input [(ngModel)]="userName" placeholder="Enter your name">
<p>Hello, {{ userName }}!</p>
If you're using ngModel in a form, you can easily handle form submission:
<form (submit)="onSubmit()">
<input [(ngModel)]="userName" name="userName" required>
<button type="submit">Submit</button>
</form>
In the component:
onSubmit() {
console.log('User Name:', this.userName);
}
You can add validation directly in the template:
<form #form="form" (ngSubmit)="onSubmit()" novalidate>
<input [(ngModel)]="userName" name="userName" required>
<div *ngIf="form.submitted && !userName">Name is required!</div>
<button type="submit">Submit</button>
</form>
Setting up forms in Angular using ngModel is a straightforward process that facilitates two-way data binding between the user interface and the component. Here's a comprehensive guide to help you get started.
To use ngModel, you need to import the FormsModule in your Angular application. This module enables template-driven forms, which utilize ngModel for data binding.
import { NgModule } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
import { FormsModule } from '@angular/forms';
import { AppComponent } from './app.component';
@NgModule({
declarations: [AppComponent],
imports: [BrowserModule, FormsModule],
bootstrap: [AppComponent]
})
export class AppModule {}
Define properties in your component that will hold the form data. For instance:
import { Component } from '@angular/core';
@Component({
selector: 'app-root,'
templateUrl: './app.component.html'
})
export class AppComponent {
userName: string = '';
userEmail: string = '';
onSubmit() {
console.log('User Name:', this.userName);
console.log('User Email:', this.userEmail);
}
}
In your HTML template, create a form and use ngModel to bind input fields to the component properties. Make sure to include the name attribute for each input to associate it with the form.
<form (ngSubmit)="onSubmit()" #userForm="ngForm">
<div>
<label for="name">Name:</label>
<input type="text" id="name" [(ngModel)]="userName" name="userName" required>
</div>
<div>
<label for="email">Email:</label>
<input type="email" id="email" [(ngModel)]="userEmail" name="userEmail" required>
</div>
<button type="submit" [disabled]="!userForm.form.valid">Submit</button>
</form>
You can easily incorporate validation messages in your form. For example, display an error message if the name or email fields are left empty upon submission:
<div *ngIf="userForm.submitted && !userName">
A name is required!
</div>
<div *ngIf="userForm.submitted && !userEmail">
Email is required!
</div>
In the component, implement the onSubmit method to process the submitted form data:
onSubmit() {
if (this.userName && this.userEmail) {
console.log('Form submitted successfully:', {
Name: this.Username,
Email: this.user email
});
}
}
ngModel is a versatile directive in Angular that simplifies data binding and form management. Here are some common use cases where ngModel shines:
ngModel is primarily used for capturing user input in forms. It allows developers to bind input fields directly to component properties, making it easy to reflect changes in real-time. For instance, a simple input for a user’s name can be bound using ngModel, enabling instant updates to the displayed value.
When creating dynamic forms based on user selections or application state, ngModel can help manage form controls efficiently. For example, in a multi-step form, different fields can appear or disappear based on previous inputs, all while maintaining accurate data binding.
With ngModel, you can easily implement validation for forms. By using built-in validation features, such as required fields or email formats, you can provide immediate feedback to users. This enhances user experience by preventing errors before form submission.
In applications where data needs to be displayed or processed in real-time (e.g., live search or filtering), ngModel allows for seamless synchronization between the input fields and the underlying data model. Changes made by the user instantly reflect in the application’s state.
ngModel can bind to complex objects, enabling the creation of nested forms. For example, in a user profile form, you can have inputs for first name, last name, and address, all bound to a single user object. This makes it easier to manage and submit structured data.
ngModel works well with various third-party libraries for UI components, such as Angular Material or Bootstrap. You can easily integrate custom components with ngModel, ensuring that data binding remains consistent across your application.
ngModel is not limited to forms; it can be used in custom components to create reusable input controls. By implementing ngModel in a custom component, you can achieve two-way data binding, allowing the parent component to control and respond to changes.
While ngModel is primarily associated with template-driven forms in Angular, it can also be integrated into reactive forms under specific scenarios. However, it's important to understand that reactive forms are generally designed to use reactive programming principles, focusing on form control and state management. Here’s how you can effectively integrate ngModel with reactive forms:
Reactive forms in Angular provide a more programmatic approach to managing form controls. They use FormGroup and FormControl to track the state and validity of form inputs. While using reactive forms, ngModel is not the primary method, but it can still be utilized for specific needs.
First, you need to import the ReactiveFormsModule in your Angular module:
import { ReactiveFormsModule } from '@angular/forms';
@NgModule({
imports: [ReactiveFormsModule],
declarations: [/* your components */],
})
export class AppModule {}
In your component, create a FormGroup to manage your form controls:
import { Component } from '@angular/core';
import { FormGroup, FormControl } from '@angular/forms';
@Component({
selector: 'app-root,'
templateUrl: './app.component.html'
})
export class AppComponent {
user form: FormGroup;
constructor() {
this.userForm = new FormGroup({
userName: new FormControl(''),
userEmail: new FormControl('')
});
}
onSubmit() {
console.log(this.userForm.value);
}
}
While it's not standard practice, you can still use ngModel within reactive forms if necessary. For example, you might want to use ngModel for specific form elements while the rest of the form is managed reactively:
<form [formGroup]="userForm" (ngSubmit)="onSubmit()">
<div>
<label for="name">Name:</label>
<input id="name" formControlName="userName" [(ngModel)]="userForm.controls['userName'].value">
</div>
<div>
<label for="email">Email:</label>
<input id="email" formControlName="userEmail" [(ngModel)]="userForm.controls['userEmail'].value">
</div>
<button type="submit">Submit</button>
</form>
While integrating ngModel with reactive forms can be useful for specific cases, it’s crucial to maintain consistency in form management. Mixing template-driven and reactive forms can lead to confusion, so it’s generally recommended to choose one approach for each form.
ngModel is a powerful directive in Angular that provides several benefits when managing forms and user input. Here are the key advantages of using ngModel:
1. Two-Way Data Binding: One of the most significant advantages of ngModel is its ability to facilitate two-way data binding. This means that changes in the input fields are automatically reflected in the component's properties and vice versa. This real-time synchronization simplifies data management in applications.
2. Simplified Form Handling: ngModel simplifies the process of handling forms by reducing boilerplate code. With minimal setup, you can bind form controls to model properties, making it easy to create and manage complex forms.
3. Built-in Validation: Using ngModel, you can easily implement form validation. Angular provides built-in validation attributes, such as required, minlength, and pattern, allowing you to enforce rules on user input and display error messages accordingly.
4. Dynamic UI Updates: With ngModel, any changes made by the user are immediately reflected in the UI. This is particularly useful for creating dynamic applications where the interface needs to respond instantly to user actions, enhancing user experience.
5. Ease of Use: ngModel is straightforward to use, making it accessible even for beginners. The syntax is intuitive, and it allows for quick setup and implementation of forms, enabling faster development cycles.
While ngModel offers many advantages in Angular applications, there are potential pitfalls that developers should be aware of. Here are some common challenges and best practices to ensure effective use of ngModel.
ngModel is a powerful directive in Angular that significantly enhances the process of managing forms and user input. Its ability to facilitate two-way data binding simplifies data synchronization between the model and the view, making it easier for developers to create dynamic and responsive applications. The directive also supports built-in validation, allowing for immediate feedback to users, which is crucial for ensuring data integrity.
However, while ngModel provides many advantages, it is essential to be mindful of potential pitfalls, such as mixing forms approaches and naming conflicts. Adhering to best practices like using unique names for inputs, implementing robust validation, and keeping forms modular—can help you maximize the benefits of ngModel while maintaining code clarity and organization.
Copy and paste below code to page Head section
ngModel is a directive in Angular that enables two-way data binding between the model and the view, primarily used in forms. It allows developers to synchronize user input with the underlying application data seamlessly.
To use ngModel, you need to import the FormsModule in your Angular module. Then, you can bind it to an input element using the syntax [(ngModel)]="propertyName" within your template.
Some key advantages include two-way data binding, simplified form handling, built-in validation, dynamic UI updates, and ease of use for developers. It allows for quick and efficient management of user input.
While ngModel is primarily designed for template-driven forms, it can be used with reactive forms in certain scenarios. However, it’s generally advisable to stick to one approach to avoid confusion.
Common pitfalls include mixing template-driven and reactive forms, naming conflicts between component properties and form controls, and inadequate validation feedback. It’s essential to be aware of these issues to maintain clean and effective code.
You can use Angular's built-in validation directives, such as required, minlength, and pattern, with ngModel. Additionally, you can display error messages in the template based on the form's validation state.