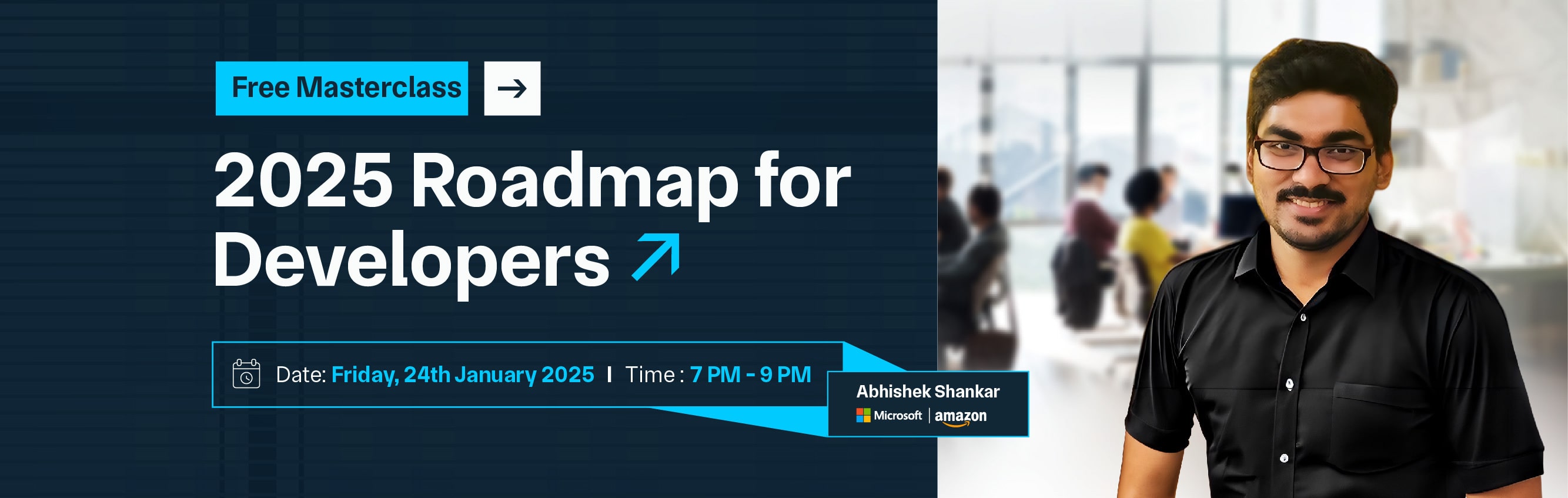

Linear regression is a fundamental technique in machine learning used to model the relationship between a dependent variable and one or more independent variables. It aims to find the best-fitting line through the data points to predict the dependent variable based on the values of the independent variables. In its simplest form, linear regression involves a single feature and a target variable, represented by the equation Y=β0+β1X+ϵY = \beta_0 + \beta_1X + \epsilonY=β0+β1X+ϵ.
Here, YYY is the target variable, β0\beta_0β0 is the intercept, β1\beta_1β1 is the slope (coefficient), XXX is the feature, and ϵ\epsilonϵ represents the error term. The core objective of linear regression is to minimize the difference between the predicted values and the actual data, usually through a method called least squares, which minimizes the Mean Squared Error (MSE). This approach ensures that the line of best fit captures the relationship between variables as accurately as possible.
Linear regression can be extended to multiple features, known as multiple linear regression. Despite its simplicity, it’s widely used for its interpretability and effectiveness in various applications, including finance, marketing, and healthcare. Linear regression is a statistical method used to model and analyze the relationship between a dependent variable and one or more independent variables.
Linear regression is a fundamental statistical and machine-learning technique used to model the relationship between a dependent variable and one or more independent variables. The goal of linear regression is to find the best-fitting line (or hyperplane in higher dimensions) that predicts the dependent variable based on the independent variables.
1. Dependent and Independent Variables:
2. Linear Relationship:
3. Equation of the Line:
4. Objective:
5. Cost Function (Mean Squared Error):
6. Finding the Best Fit:
1. Simple Linear Regression:
2. Multiple Linear Regression:
3. Polynomial Regression:
Linear regression is grounded in a straightforward mathematical equation that models the relationship between variables. The fundamental equation for simple linear regression is: Y=β0+β1X+ϵY = \beta_0 + \beta_1X + \epsilonY=β0+β1X+ϵ
Here's a breakdown of each component in this equation:
1. YYY: Dependent Variable (Target)
2. β0\beta_0β0: Intercept
3. β1\beta_1β1: Slope (Coefficient)
4. XXX: Independent Variable (Feature)
5. ϵ\epsilonϵ: Error Term
The line of best fit, also known as the regression line, is the line that minimizes the sum of the squared differences between the observed values and the predicted values.
Mathematically, this is achieved by finding the values of β0\beta_0β0 and β1\beta_1β1 that minimize the Mean Squared Error (MSE): MSE=1n∑i=1n(Yi−(β0+β1Xi))2\text{MSE} = \frac{1}{n} \sum_{i=1}^{n} (Y_i - (\beta_0 + \beta_1 X_i))^2MSE=n1∑i=1n(Yi−(β0+β1Xi))2
Where:
By minimizing this error, the regression line provides the best linear approximation of the relationship between XXX and YYY, helping to make accurate predictions and understand underlying patterns in the data.
Linear regression is a fundamental algorithm in machine learning used for predicting a continuous target variable based on one or more features. Here's an overview of how it works and how it's optimized:
1. Model Definition:
2. Objective:
1. Cost Function:
The cost function measures the accuracy of the model’s predictions. For linear regression, the most commonly used cost function is the Mean Squared Error (MSE): MSE=1n∑i=1n(Yi−Yi^)2\text{MSE} = \frac{1}{n} \sum_{i=1}^{n} (Y_i - \hat{Y_i})^2MSE=n1∑i=1n(Yi−Yi^)2
where:
2. Minimization:
The goal is to find the parameter values that minimize the MSE. This involves adjusting β0\beta_0β0 and β1\beta_1β1 (or all coefficients in multiple regression) to reduce the cost function.
1. Gradient Descent:
Gradient Descent is an iterative optimization algorithm used to find the minimum of the cost function. It updates the parameters β\betaβ by moving them in the direction of the steepest decrease of the cost function.
Update Rule: βj:=βj−α∂∂βjMSE\beta_j := \beta_j - \alpha \frac{\partial}{\partial \beta_j} \text{MSE}βj:=βj−α∂βj∂MSE where:
2. Learning Rate:
3. Convergence:
In linear regression, the cost function, often called the mean squared error (MSE), measures how well the model's predictions match the actual data. The goal is to minimize this cost function to find the best-fitting line for your data. Here’s a breakdown of the cost function for linear regression:
1. Linear Regression Model:
2. Cost Function:
The cost function, or loss function, is defined as the average squared difference between the actual values yiy_iyi and the predicted values y^i\hat{y}_iy^i: J(θ0,θ1)=12m∑i=1m(y^i−yi)2J(\theta_0, \theta_1) = \frac{1}{2m} \sum_{i=1}^m (\hat{y}_i - y_i)^2J(θ0,θ1)=2m1i=1∑m(y^i−yi)2 where:s
3. Simplified Version (without 12\frac{1}{2}21):
For linear regression to produce valid and reliable results, certain assumptions need to be met. These assumptions ensure that the model's estimates are unbiased, efficient, and consistent. Here’s a brief overview of the key assumptions:
Sure! Implementing linear regression in Python is straightforward. You can use various libraries such as NumPy for basic implementations or scikit-learn for more advanced functionalities. Below are two approaches: a basic implementation using NumPy and a more comprehensive one using scikit-learn.
This example demonstrates how to implement linear regression from scratch using NumPy. It includes calculating the cost function and performing gradient descent.
import numpy as np
import matplotlib.pyplot as plt
# Generate some synthetic data
np.random.seed(42)
X = 2 * np.random.rand(100, 1) # Features
y = 4 + 3 * X + np.random.randn(100, 1) # Target variable
# Add a column of ones to X for the intercept term
X_b = np.c_[np.ones((100, 1)), X]
# Calculate the optimal parameters using the Normal Equation
theta_best = np.linalg.inv(X_b.T.dot(X_b)).dot(X_b.T).dot(y)
# Print the parameters
print("Intercept (theta_0):", theta_best[0])
print("Slope (theta_1):", theta_best[1])
# Predict
X_new = np.array([[0], [2]])
X_new_b = np.c_[np.ones((2, 1)), X_new] # Add intercept term
y_predict = X_new_b.dot(theta_best)
# Plot the results
plt.plot(X, y, "b.")
plt.plot(X_new, y_predict, "r-")
plt.xlabel("X")
plt.ylabel("y")
plt.title("Linear Regression")
plt.show()
scikit-learn provides a simple and powerful way to perform linear regression. Here’s an example using scikit-learn’s LinearRegression class:
import numpy as np
import matplotlib.pyplot as plt
from sklearn.linear_model import LinearRegression
# Generate some synthetic data
np.random.seed(42)
X = 2 * np.random.rand(100, 1) # Features
y = 4 + 3 * X + np.random.randn(100, 1) # Target variable
# Create and fit the model
model = LinearRegression()
model.fit(X, y)
# Print the parameters
print("Intercept (theta_0):", model.intercept_[0])
print("Slope (theta_1):", model.coef_[0][0])
# Predict
X_new = np.array([[0], [2]])
y_predict = model.predict(X_new)
# Plot the results
plt.plot(X, y, "b.")
plt.plot(X_new, y_predict, "r-")
plt.xlabel("X")
plt.ylabel("y")
plt.title("Linear Regression with scikit-learn")
plt.show()
To assess the performance of a linear regression model, several metrics are commonly used. These metrics help quantify how well the model predicts the target variable and identify areas for improvement. Here’s an overview of the key metrics and their interpretations:
Definition: R-squared measures the proportion of the variance in the dependent variable that is predictable from the independent variables. It is expressed as a value between 0 and 1. R2=1−Sum of Squared Residuals (SSR)Total Sum of Squares (TSS)R^2 = 1 - \frac{\text{Sum of Squared Residuals (SSR)}}{\text{Total Sum of Squares (TSS)}}R2=1−Total Sum of Squares (TSS)Sum of Squared Residuals (SSR)
Interpretation:
Definition: MAE measures the average magnitude of errors in the model’s predictions without considering their direction. It is the average of the absolute differences between predicted and actual values. MAE=1n∑i=1n∣Yi−Yi^∣\text{MAE} = \frac{1}{n} \sum_{i=1}^{n} |Y_i - \hat{Y_i}|MAE=n1∑i=1n∣Yi−Yi^∣
Interpretation:
Definition: MSE measures the average squared difference between predicted and actual values. It penalizes larger errors more than MAE. MSE=1n∑i=1n(Yi−Yi^)2\text{MSE} = \frac{1}{n} \sum_{i=1}^{n} (Y_i - \hat{Y_i})^2MSE=n1∑i=1n(Yi−Yi^)2
Interpretation:
Definition: RMSE is the square root of the MSE. It provides an estimate of the standard deviation of the residuals (prediction errors). RMSE=MSE\text{RMSE} = \sqrt{\text{MSE}}RMSE=MSE
Interpretation:
By analyzing these metrics, you can assess how well your linear regression model is performing and make necessary adjustments to improve its predictive accuracy.
Implementing linear regression involves several key steps: preparing your dataset, splitting it into training and testing sets, training the model, making predictions, and evaluating its performance. Here’s a detailed guide to walk you through each step using Python and the Scikit-learn library:
Before you can train a linear regression model, you need to prepare your data. This involves loading the data, handling missing values, and performing feature engineering if necessary.
import pandas as pd
# Load the dataset
data = pd.read_csv('your_dataset.csv')
# Inspect the dataset
print(data.head())
# Handle missing values (if any)
data = data.dropna() # Or use other imputation techniques
# Feature selection (optional)
features = data[['feature1', 'feature2', 'feature3']] # Replace with actual feature names
target = data['target'] # Replace with the actual target column name
Splitting your data ensures that you can train your model on one subset of the data and evaluate its performance on an unseen subset.
from sklearn.model_selection import train_test_split
# Split the data into training and testing sets
X_train, X_test, y_train, y_test = train_test_split(features, target, test_size=0.2, random_state=42)
Scikit-learn provides an easy-to-use implementation of linear regression. You’ll train the model on your training data.
from sklearn.linear_model import LinearRegression
# Initialize the model
model = LinearRegression()
# Train the model
model.fit(X_train, y_train)
Once the model is trained, you can use it to make predictions on the test data.
# Make predictions on the test set
y_pred = model.predict(X_test)
Evaluate your model’s performance using metrics such as R-squared, Mean Absolute Error (MAE), Mean Squared Error (MSE), and Root Mean Squared Error (RMSE).
from sklearn.metrics import r2_score, mean_absolute_error, mean_squared_error
import numpy as np
# Calculate evaluation metrics
r2 = r2_score(y_test, y_pred)
mae = mean_absolute_error(y_test, y_pred)
mse = mean_squared_error(y_test, y_pred)
rmse = np.sqrt(mse)
# Print the results
print(f'R-squared: {r2:.4f}')
print(f'Mean Absolute Error (MAE): {mae:.4f}')
print(f'Mean Squared Error (MSE): {mse:.4f}')
print(f'Root Mean Squared Error (RMSE): {rmse:.4f}')
When working with linear regression in practice, especially in scenarios involving multiple features, there are several important considerations to ensure that your model performs well and provides reliable insights. Here’s a guide to handling multiple features, addressing multicollinearity, and performing feature scaling and normalization:
Multiple Linear Regression involves predicting the target variable using more than one feature. The model equation is extended to:
Y=β0+β1X1+β2X2+…+βnXn+ϵY = \beta_0 + \beta_1X_1 + \beta_2X_2 + \ldots + \beta_nX_n + \epsilonY=β0+β1X1+β2X2+…+βnXn+ϵ
Steps:
from sklearn.preprocessing import PolynomialFeatures
# Example: Adding polynomial features
poly = PolynomialFeatures(degree=2)
X_poly = poly.fit_transform(features)
Multicollinearity occurs when two or more features are highly correlated, which can lead to instability in the coefficient estimates and make the model’s predictions unreliable.
Detection:
import statsmodels.api as sm
# Compute VIF
def calculate_vif(X):
vif = pd.DataFrame()
vif['features'] = X.columns
vif['VIF'] = [sm.OLS(X.iloc[:, i], sm.add_constant(X.drop(X.columns[i], axis=1))).fit().rsquared for i in range(X.shape[1])]
vif['VIF'] = 1 / (1 - vif['VIF'])
return vif
print(calculate_vif(features))
Mitigation:
from sklearn.decomposition import PCA
# Example: PCA to reduce dimensionality
pca = PCA(n_components=2)
X_pca = pca.fit_transform(features)
Feature Scaling and Normalization are important when features have different units or scales. Scaling ensures that each feature contributes equally to the model.
Standardization (Z-score Normalization): Scales features so they have a mean of 0 and a standard deviation of 1.
from sklearn.preprocessing import StandardScaler
scaler = StandardScaler()
X_scaled = scaler.fit_transform(features)
Min-Max Scaling: Scales feature a fixed range, usually 0 to 1.
from sklearn.preprocessing import MinMaxScaler
scaler = MinMaxScaler()
X_normalized = scaler.fit_transform(features)
Why It's Important:
When implementing linear regression, several challenges can impact the model's performance and interpretability. Addressing these challenges effectively is crucial for building a robust and reliable model. Here’s a guide to common issues and strategies for dealing with them:
Overfitting occurs when the model learns the training data too well, including noise and outliers, which leads to poor generalization to new, unseen data. Underfitting happens when the model is too simple to capture the underlying patterns in the data, resulting in poor performance on both the training and test sets.
How to Address Overfitting:
Regularization: Add a penalty to the model’s complexity to prevent overfitting. Common techniques include:
from sklearn.linear_model import Lasso, Ridge
# Lasso Regression
model_lasso = Lasso(alpha=0.1)
model_lasso.fit(X_train, y_train)
# Ridge Regression
model_ridge = Ridge(alpha=0.1)
model_ridge.fit(X_train, y_train)
Cross-Validation: Use techniques like k-fold cross-validation to evaluate model performance on different subsets of the data to ensure it generalizes well.
from sklearn.model_selection import cross_val_score
scores = cross_val_score(model, X_train, y_train, cv=5)
print(f'Cross-validation scores: {scores}')
How to Address Underfitting:
Increase Model Complexity: Use polynomial features or interactions between features to capture more complex relationships.
from sklearn.preprocessing import PolynomialFeatures
poly = PolynomialFeatures(degree=2)
X_poly = poly.fit_transform(X_train)
model_poly = LinearRegression().fit(X_poly, y_train)
Outliers are data points that deviate significantly from other observations. They can skew the results of linear regression and affect the accuracy of predictions.
How to Address Outliers:
Identify Outliers: Use visualization tools like scatter plots or statistical methods like Z-scores or the IQR method to detect outliers.
import numpy as np
from scipy import stats
# Z-score method
z_scores = np.abs(stats.zscore(features))
outliers = (z_scores > 3).all(axis=1)
Transform Data: Apply transformations such as logarithmic or square root transformations to reduce the impact of outliers.
features_transformed = np.log(features + 1) # Log transformation
Robust Regression: Use models that are less sensitive to outliers, such as Robust Regression or Huber Regressor.
from sklearn.linear_model import HuberRegressor
model_huber = HuberRegressor()
model_huber.fit(X_train, y_train)
Feature Selection and Dimensionality Reduction are techniques used to improve model performance by reducing the number of features, thus simplifying the model and improving generalization.
Feature Selection:
Filter Methods: Use statistical tests or correlation metrics to select features that are most relevant to the target variable.
from sklearn.feature_selection import SelectKBest, f_regression
selector = SelectKBest(score_func=f_regression, k='all')
X_new = selector.fit_transform(features, target)
Wrapper Methods: Evaluate feature subsets by training models and assessing their performance (e.g., Recursive Feature Elimination).
from sklearn.feature_selection import RFE
model = LinearRegression()
selector = RFE(model, n_features_to_select=5)
X_rfe = selector.fit_transform(features, target)
Dimensionality Reduction:
Principal Component Analysis (PCA): Reduces the number of features by transforming them into a lower-dimensional space while retaining most of the variance.
from sklearn.decomposition import PCA
pca = PCA(n_components=2)
X_pca = pca.fit_transform(features)
Scenario:
Predicting housing prices is a classic real-world application of linear regression. In this example, we use linear regression to estimate the price of a house based on several features, such as the size of the house, number of bedrooms, location, and age of the property. This can help buyers and sellers make informed decisions and can also be used by real estate professionals to assess property values.
The goal is to develop a model that predicts the selling price of a house based on various features. The target variable is the house price, and the independent variables (features) might include:
We need historical data on house sales, including both the prices and the features of the houses. This data can be sourced from real estate listings, property records, or online datasets.
Example Data:
a. Preparing the Dataset:
Load and clean the data, handle any missing values, and encode categorical variables such as location.
import pandas as pd
from sklearn.model_selection import train_test_split
from sklearn.linear_model import LinearRegression
from sklearn.preprocessing import StandardScaler
# Load the dataset
data = pd.read_csv('housing_data.csv')
# Handle missing values
data = data.dropna()
# Encode categorical variable (Location)
data = pd.get_dummies(data, columns=['Location'], drop_first=True)
# Define features and target
features = data[['Size', 'Bedrooms', 'Bathrooms', 'Age', 'Location_B']]
target = data['Price']
# Split the data into training and testing sets
X_train, X_test, y_train, y_test = train_test_split(features, target, test_size=0.2, random_state=42)
b. Training the Model:
Train the linear regression model on the training data.
# Initialize and train the model
model = LinearRegression()
model.fit(X_train, y_train)
c. Making Predictions:
Use the model to make predictions on the test set.
# Make predictions
y_pred = model.predict(X_test)
d. Evaluating the Model:
Evaluate the model’s performance using metrics like R-squared, Mean Absolute Error (MAE), Mean Squared Error (MSE), and Root Mean Squared Error (RMSE).
from sklearn.metrics import r2_score, mean_absolute_error, mean_squared_error
import numpy as np
# Calculate evaluation metrics
r2 = r2_score(y_test, y_pred)
mae = mean_absolute_error(y_test, y_pred)
mse = mean_squared_error(y_test, y_pred)
rmse = np.sqrt(mse)
# Print the results
print(f'R-squared: {r2:.4f}')
print(f'Mean Absolute Error (MAE): {mae:.4f}')
print(f'Mean Squared Error (MSE): {mse:.4f}')
print(f'Root Mean Squared Error (RMSE): {rmse:.4f}')
Linear regression is a foundational technique in statistics and machine learning with a wide range of applications across various fields. Here are some common applications:
Linear regression is a widely used technique with several advantages and some limitations. Here's a detailed look at both:
1. Simplicity: Linear regression is easy to understand and interpret. It provides a clear, straightforward model of the relationship between the dependent and independent variables.
2. Efficiency: It requires relatively low computational resources compared to more complex models, making it fast and efficient for training and prediction.
3. Less Prone to Overfitting: With fewer parameters to tune (just the coefficients of the linear equation), linear regression is less likely to overfit the data, especially when compared to more complex models.
4. Ease of Implementation: Many statistical software packages and libraries (e.g., scikit-learn in Python) offer built-in functions for linear regression, making it easy to implement.
5. Good for Linearly Separable Data: Linear regression performs well when the relationship between variables is approximately linear. It can effectively capture this linear relationship.
6. Interpretable Coefficients: The coefficients in a linear regression model are directly interpretable, providing insights into the magnitude and direction of the relationships between features and the target variable.
7. Foundation for More Complex Models: Understanding linear regression provides a foundation for learning more complex models and techniques, such as polynomial regression and regularization methods.
1. Assumption of Linearity: Linear regression assumes a linear relationship between the independent and dependent variables. It may perform poorly if the true relationship is non-linear or if important interactions between variables are not captured.
2. Sensitivity to Outliers: Linear regression can be sensitive to outliers, which can significantly affect the model’s performance and coefficients.
3. Multicollinearity: If the independent variables are highly correlated with each other, it can lead to multicollinearity, making it difficult to estimate the coefficients accurately and interpret their effect.
4. Assumption of Homoscedasticity: Linear regression assumes that the variance of the errors (residuals) is constant across all levels of the independent variables. If this assumption is violated, it can lead to inefficient estimates and misleading conclusions.
5. No Handling of Complex Relationships: Linear regression cannot capture complex relationships or interactions between variables without transforming the data or adding polynomial terms.
6. Assumption of Normality: For hypothesis testing and confidence intervals, linear regression assumes that the residuals are normally distributed. Violations of this assumption can affect the reliability of statistical inferences.
7. Over-simplification: Because linear regression is simple, it may oversimplify the model and fail to capture the nuances and complexities of the real-world data.
Linear regression is a fundamental and powerful statistical technique used to model and analyze the relationship between a dependent variable and one or more independent variables.
Its simplicity, interpretability, and effectiveness make it a popular choice for a wide range of applications, from predicting housing prices to evaluating financial trends and beyond.
Copy and paste below code to page Head section
Linear regression is a statistical technique used to model the relationship between a dependent variable and one or more independent variables. It aims to find the best-fitting linear relationship that predicts the dependent variable based on the independent variables.
Simple Linear Regression: Involves a single independent variable and a dependent variable. The model predicts the dependent variable based on the value of the independent variable. Multiple Linear Regression: Involves two or more independent variables. The model predicts the dependent variable based on multiple predictors.
The main assumptions of linear regression are: Linearity: The relationship between the independent and dependent variables is linear. Independence: Observations are independent of each other. Homoscedasticity: The variance of residuals is constant across all levels of the independent variables. Normality: The residuals (errors) of the model are normally distributed.
Multicollinearity occurs when independent variables are highly correlated with each other, which can make it difficult to estimate the relationship between each predictor and the target variable. It can be addressed by: Removing Highly Correlated Features: Dropping one of the correlated variables. Using Dimensionality Reduction: Techniques like Principal Component Analysis (PCA) to transform features into uncorrelated components.
Performance is evaluated using metrics such as: R-squared: Measures the proportion of the variance in the dependent variable explained by the model. Mean Absolute Error (MAE): Average of the absolute errors between predicted and actual values. Mean Squared Error (MSE): Average of the squared errors. Root Mean Squared Error (RMSE): Square root of the MSE, providing error in the same units as the target variable.
The line of best fit is the line that minimizes the sum of the squared differences between the observed values and the values predicted by the model. It represents the best linear approximation of the relationship between the independent and dependent variables.