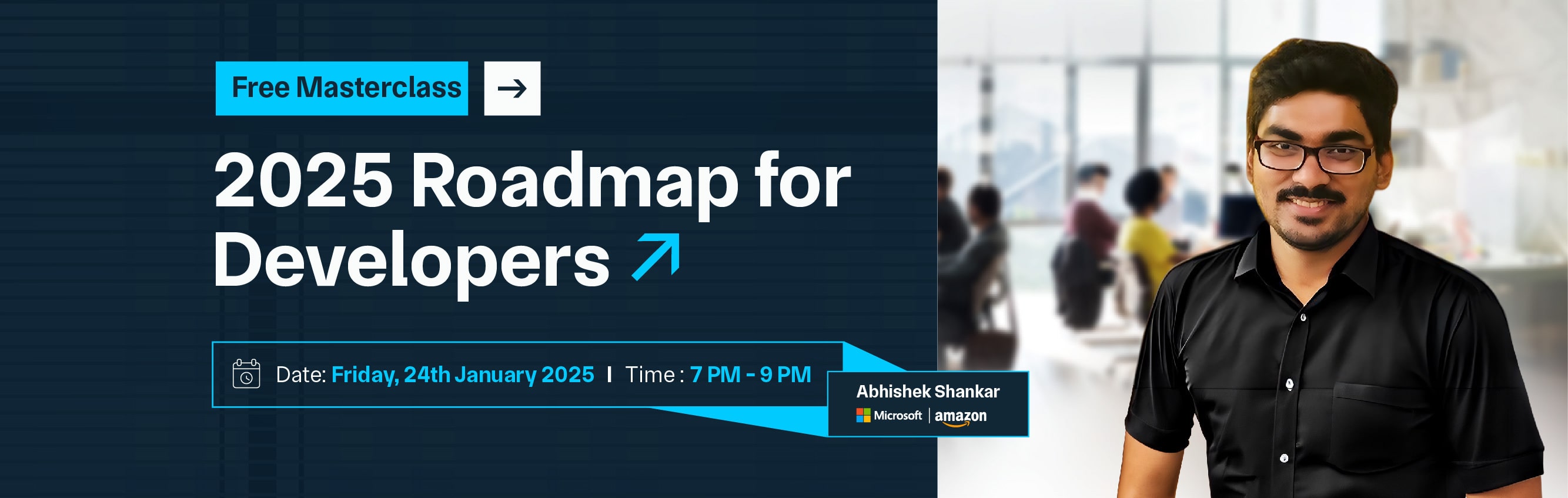

The latest version of React, React 18, introduces several groundbreaking features that enhance performance and improve user experience. One of the most significant advancements is Concurrent Rendering, which allows React to work on multiple tasks simultaneously, leading to smoother and more responsive applications. This is complemented by Automatic Batching, which intelligently groups state updates, reducing the number of re-renders and enhancing performance.
Additionally, the new Transition API helps manage UI state changes more gracefully, providing a seamless experience during interactions. React 18 also introduces powerful hooks such as useTransition and useDeferredValue, allowing developers to manage state transitions and prioritize updates more effectively. Performance improvements extend to server-side rendering (SSR), enabling faster initial loads and better user experiences.
Upgrading to this latest version is straightforward, with clear migration paths provided in the documentation, although developers should be aware of potential challenges. The ecosystem around React has also evolved, ensuring compatibility with popular libraries and tools. With enhanced debugging features in React Developer Tools and robust community support, developers are well-equipped to leverage the full potential of React 18. Overall, the latest version reaffirms React's position as a leading library for building modern web applications.
React 18 introduces a host of innovative features designed to enhance performance, improve user experience, and streamline development processes. These advancements allow developers to create more responsive applications while leveraging new capabilities for managing complex states and rendering scenarios.
With improvements such as Concurrent Rendering and Automatic Batching, React 18 sets the stage for building modern web applications that are both efficient and user-friendly. Below is a list of the key new features that make React 18 a significant upgrade.
Concurrent Rendering is a significant enhancement that allows React to work on multiple tasks simultaneously, improving the responsiveness of applications. This feature enables React to pause and resume rendering work as needed, ensuring that user interactions are prioritized.
For instance, while rendering a large component, React can still respond to user input, providing a smoother experience. This capability is especially beneficial for applications with complex UIs or heavy data processing, as it prevents blocking and keeps the app feeling snappy and interactive.
Automatic Batching simplifies state management by automatically grouping multiple state updates into a single render. Previously, updates made within the same event handler could result in multiple re-renders, which could be inefficient.
With Automatic Batching, React intelligently combines these updates, reducing the number of renders required and improving overall performance. This feature helps optimize rendering performance, especially in applications that frequently update state, leading to faster UI updates and a more seamless user experience.
The Transition API introduces a way to distinguish between urgent and non-urgent updates. By using the startTransition method, developers can mark certain updates as transitions, allowing React to prioritize them accordingly.
This is particularly useful for managing complex state changes in user interfaces, such as loading new data while keeping the current UI responsive. By deferring non-urgent updates, transitions ensure that user interactions remain smooth, enhancing the overall usability of the application.
React 18 introduces new hooks that empower developers to manage states more effectively. The useTransition hook allows developers to mark specific state updates as transitions, providing finer control over rendering priorities.
Similarly, useDeferredValue enables developers to defer updates to non-urgent values, allowing the UI to remain responsive during heavy processing tasks. These hooks offer more flexibility in managing how and when updates occur, leading to smoother user experiences in applications with complex state management.
Suspense for Data Fetching enhances the existing Suspense feature by enabling components to wait for asynchronous data before rendering. This allows developers to build more robust applications that can handle loading states gracefully.
When a component fetches data, it can suspend rendering until the data is available, improving the overall flow of the application. This feature streamlines data handling and enhances the user experience by reducing flickering and providing a more cohesive loading experience.
The streaming Server-Side Rendering (SSR) feature significantly improves the performance of server-rendered applications. By allowing HTML responses to be streamed to the client as they are generated, this capability reduces the time users wait to see content on their screens.
This means that users can interact with parts of the application even while other components are still loading, leading to faster-perceived performance and a better user experience overall.
Selective Hydration optimizes the hydration process for server-rendered applications by allowing React to hydrate components selectively. Instead of hydrating the entire application at once, React can hydrate individual components as they become interactive.
This approach reduces the initial load time and improves the responsiveness of applications, especially for larger projects where full hydration can be resource-intensive. By targeting specific components, selective hydration helps enhance performance without compromising functionality.
React 18 introduces new APIs for server-side rendering, making it easier for developers to build efficient server-rendered applications. These APIs provide more flexibility and control over the rendering process, allowing for better integration with frameworks and libraries.
Developers can take advantage of these enhancements to optimize their SSR setups, leading to improved performance and a smoother experience for users accessing server-rendered content.
The updated React Developer Tools provide improved debugging and performance monitoring capabilities for React applications. With enhanced features, developers can more easily inspect component hierarchies, track state changes, and measure performance metrics.
These tools empower developers to identify bottlenecks and optimize their applications more effectively, contributing to overall better application performance and a smoother development workflow. Each of these features in React 18 contributes to making the library more powerful, efficient, and user-friendly, further solidifying React's position as a leading choice for building modern web applications.
Upgrading to React 18 involves a few key steps to ensure a smooth transition. Here’s a detailed guide on how to upgrade:
Before upgrading, ensure that your project dependencies, including libraries and tools, are compatible with React 18. Check the documentation for any libraries you’re using to confirm their compatibility.
You can upgrade React and React-DOM to the latest version using npm or yarn. Run the following commands in your project directory:
npm install react@latest react-dom@latest
or
yarn add react@latest react-dom@latest
This will install the latest stable version of React and React-DOM.
While React 18 maintains backward compatibility, you can refactor parts of your codebase to take advantage of new features. Here are some recommendations:
If you’re using ReactDOM.render, update it to the new create root API to take advantage of Concurrent Rendering:
Import React from 'react';
import ReactDOM from 'react-dom/client';
import App from './App';
const root = ReactDOM.createRoot(document.getElementById('root'));
root.render(<App />);
After upgrading, thoroughly test your application to ensure that everything works as expected. Pay particular attention to any areas that might be affected by new rendering behaviors or features.
While testing, keep an eye out for any deprecation warnings or errors in the console. Address these issues promptly to ensure your application remains stable and performs optimally.
Finally, review the official React 18 documentation for any additional guidance or specific details that may apply to your project. By following these steps, you can successfully upgrade to React 18 and take advantage of its new features and performance improvements.
React 18 introduces several significant performance improvements designed to enhance the efficiency and responsiveness of applications. These advancements enable developers to create smoother user experiences, manage complex UIs more effectively, and optimize rendering processes. Below are the key performance enhancements that come with React 18.
Concurrent Rendering is a major enhancement in React 18 that allows the library to work on multiple tasks simultaneously. By enabling React to pause rendering for less urgent updates, it prioritizes user interactions, ensuring that applications remain responsive even during heavy processing.
This feature is particularly beneficial for complex UIs, as it prevents blocking and allows users to interact with the interface. At the same time, background tasks are being processed, resulting in a smoother experience.
Automatic Batching simplifies state management by grouping multiple state updates into a single render automatically. Previously, each state update could trigger a separate render, which could be inefficient.
With Automatic Batching, React intelligently combines these updates, leading to fewer renders and improved performance, especially during user interactions. This optimization enhances the overall fluidity of the application, making it feel more responsive and cohesive.
React 18 enhances server-side rendering capabilities by enabling streaming HTML responses to clients. This improvement allows users to interact with parts of an application even while other components are still loading, significantly reducing perceived load times.
By optimizing the SSR process, React 18 ensures that users experience faster initial loads, making server-rendered applications more efficient and user-friendly.
Selective Hydration allows React to hydrate individual components as they become interactive instead of waiting for the entire application to hydrate. This optimization reduces the initial load time and resource usage, particularly in larger applications. By targeting specific components for hydration, React 18 enhances performance and responsiveness, leading to a better overall user experience.
React 18 introduces improvements in the reconciliation process, making it more efficient in updating the DOM. These optimizations minimize the work required during renders, resulting in quicker updates and a more responsive user interface.
By enhancing the reconciliation algorithm, React 18 ensures that applications can handle dynamic changes seamlessly, improving performance in real-time scenarios.
The updated React Developer Tools now include performance monitoring features that help developers identify bottlenecks and optimize rendering behavior. These tools provide insights into component rendering times and re-renders, allowing developers to pinpoint performance issues and implement necessary optimizations.
With better debugging capabilities, developers can enhance the performance of their applications more effectively. These performance improvements in React 18 collectively contribute to building faster, more responsive, and user-friendly applications, solidifying React's status as a leading choice for modern web development.
Upgrading to the latest version of React is essential for developers looking to leverage new features, performance enhancements, and improvements in stability. Here’s a step-by-step guide to help you successfully upgrade your React application.
Before upgrading, ensure that your project’s dependencies, including libraries and third-party tools, are compatible with the latest version of React. Review the documentation for these libraries to identify any potential breaking changes or required updates.
The first step in the upgrade process is to update your React and React-DOM packages. You can easily do this using npm or yarn. Run the following commands in your project directory:
npm install react@latest react-dom@latest
or
yarn add react@latest react-dom@latest
This will install the latest stable versions of React and React-DOM.
While React 18 maintains backward compatibility, it’s a good idea to refactor your code to take advantage of new features. Consider implementing Concurrent Rendering, using the new hooks (useTransition, useDeferredValue), and adopting the new createRoot API for rendering.
If you’re currently using ReactDOM.render, you’ll need to update it to use the new createRoot method. Here’s an example:
Import React from 'react';
import ReactDOM from 'react-dom/client';
import App from './App';
const root = ReactDOM.createRoot(document.getElementById('root'));
root.render(<App />);
This change allows you to utilize Concurrent Rendering features.
After upgrading, thoroughly test your application to ensure that everything works as expected. Pay special attention to any areas that may be affected by new rendering behaviors or features.
During testing, watch for any deprecation warnings or errors in the console. React may provide messages about outdated practices or features. Addressing these promptly will help maintain application stability and performance.
Finally, consult the official React 18 documentation for additional guidance and details about new features. The documentation includes valuable information on best practices, migration paths, and usage examples.
By following these steps, you can smoothly upgrade to the latest version of React, ensuring your application benefits from the latest features and performance improvements. Embracing the updates not only enhances your application’s functionality but also keeps your skills and knowledge current in the ever-evolving world of web development.
The ecosystem and tooling surrounding React 18 have evolved to support its new features and improve the overall development experience. This includes updates to libraries, tools, and community resources that enhance productivity and streamline the development process.
Many popular libraries and frameworks in the React ecosystem have been updated to support React 18. Libraries like React Router, Redux, and Styled Components are now compatible with the latest version, allowing developers to integrate them seamlessly into their applications.
This compatibility ensures that developers can leverage existing tools while utilizing new features in React 18.
The React Developer Tools have received enhancements to improve debugging and performance monitoring. The new version offers features that help developers visualize component hierarchies, track state changes, and analyze rendering behavior.
These tools are essential for identifying performance bottlenecks and optimizing applications effectively, making them even more valuable with the introduction of Concurrent Rendering and other new features.
Testing libraries such as React Testing Library and Jest have also adapted to support the latest React features. This includes updates for testing new hooks, concurrent features, and the createRoot method.
With these enhancements, developers can write more effective and efficient tests, ensuring that their applications remain robust as they upgrade to the latest version.
React 18 has improved integration capabilities with popular frameworks like Next.js and Gatsby. These frameworks have adopted the latest React features, such as automatic static optimization and improved server-side rendering.
This integration allows developers to build performant, modern web applications that take full advantage of React 18's capabilities while maintaining the best practices offered by these frameworks.
The React community continues to provide a wealth of resources, tutorials, and documentation focused on the latest version. Online forums, GitHub repositories, and educational platforms offer valuable insights into best practices and use cases for new features.
Engaging with the community can provide support and inspiration for developers looking to enhance their skills and projects.
New tools and plugins designed for performance monitoring and analysis have emerged alongside React 18. These tools allow developers to gain insights into rendering performance, user interactions, and application efficiency. By integrating these tools into their workflows, developers can proactively identify areas for optimization and ensure that their applications run smoothly.
The ecosystem and tooling around React 18 have matured significantly, providing developers with the resources they need to build efficient, high-quality applications. By leveraging these updates and community resources, developers can enhance their productivity and stay at the forefront of modern web development.
React 18 marks a significant evolution in the React library, introducing a range of features and improvements that enhance performance, usability, and developer experience. With advancements such as Concurrent Rendering, Automatic Batching, and new hooks, developers are empowered to create more responsive and efficient applications. The improved server-side rendering capabilities and selective hydration further optimize load times and user interactions, making React an even more robust choice for modern web development.
The ecosystem surrounding React 18, including updated libraries, enhanced developer tools, and rich community resources, ensures that developers have the support they need to maximize the benefits of the latest version. By upgrading to React 18 and leveraging these new features, developers can build high-quality applications that meet user expectations for speed and responsiveness.
Copy and paste below code to page Head section
React 18 is the latest major version of the React library, introducing new features and improvements aimed at enhancing performance, user experience, and developer productivity.
Key features of React 18 include Concurrent Rendering, Automatic Batching, new hooks (useTransition, useDeferredValue), streaming server-side rendering, and the createRoot API for rendering.
To upgrade, you can use npm or yarn to install the latest versions of React and React-DOM. Additionally, you'll need to update your rendering code to use the createRoot API and refactor your components to take advantage of new features.
Yes, React 18 maintains backward compatibility. Most existing React code will work without modification, although you may want to refactor parts of your application to leverage new features and improvements.
Concurrent Rendering is a feature that allows React to work on multiple tasks simultaneously, improving responsiveness by prioritizing user interactions over less urgent updates.
Automatic Batching automatically groups multiple state updates into a single render, reducing the number of re-renders needed and improving overall performance during state changes.