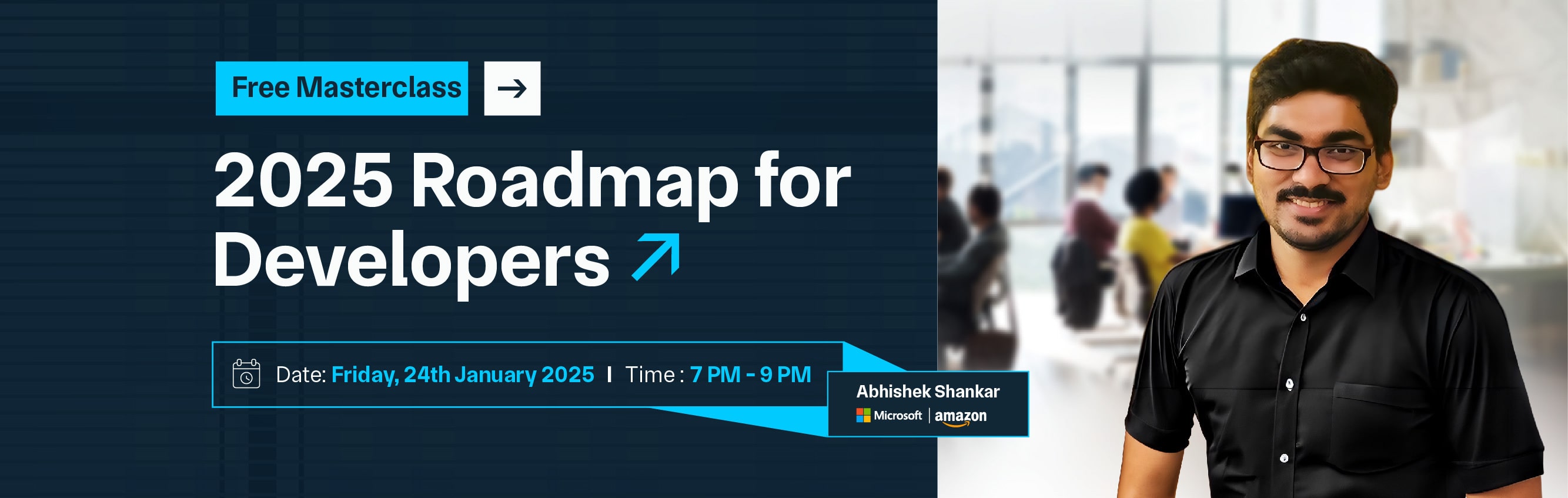

To install React.js on Windows, start by ensuring you have Node.js and npm (Node Package Manager) installed, as they are essential for running and managing React applications. Begin by downloading the Node.js installer from the official website. Run the installer and follow the prompts to complete the installation, which also includes npm.
Once installed, open Command Prompt or PowerShell to verify the installation by running node -v and npm -v to check their versions. Next, install create-react-app, a tool that simplifies the setup of a new React project. Run the command npm install -g create-react-app to install it globally. With create-react-app installed, navigate to your desired project directory and create a new React application by executing npx create-react-app my-app, replacing my-app with your preferred project name.
After the project is created, change to the project directory using cd my-app and start the development server with npm start. This command will open a new browser window displaying your React application at http://localhost:3000. You are now ready to begin developing your React application!
Before diving into React.js development on Windows, it's important to ensure you have a few essential tools in place. Here's a quick guide to the prerequisites you'll need to get started with React.js. With the right setup, you'll be ready to build and manage your React applications efficiently. Before installing React.js on Windows, ensure you have the following:
1. Basic Knowledge:
2. Node.js and npm:
3. Code Editor (Recommended):
4. Internet Connection:
5. Administrative Privileges:
To start working with React.js, you first need to install Node.js and npm, as they are essential for managing your project and its dependencies. Here’s a straightforward guide to downloading and installing Node.js and npm on your Windows system. Once installed, you'll be all set to begin your React development journey.
1. Download Node.js Installer:
2. Run the Installer:
3. Accept the License Agreement:
4. Select Installation Directory:
5. Select Components:
6. Install:
7. Complete the Installation:
8. Verify Installation:
With Node.js and npm installed, you're now ready to proceed with setting up your React environment.
A code editor is essential for developing React applications. Visual Studio Code (VS Code) is highly recommended due to its rich features and extensions tailored for JavaScript and React development. Here's how to install it:
1. Download Visual Studio Code:
2. Run the Installer:
3. Follow the Setup Wizard:
4. Choose Installation Location:
5. Select Additional Tasks:
6. Install:
7. Complete Installation:
8. Open and Configure VS Code:
Visual Studio Code is now set up and ready for React development, providing a powerful environment to write, debug, and manage your code.
Once you have Node.js, npm, and a code editor installed, you’re ready to create and set up a new React project. Follow these steps:
1. Open Command Prompt or PowerShell:
2. Install Create React App:
create-react-app is a command-line tool that helps set up a new React project with a single command. Install it globally by running:
npm install -g create-react-app
3. Create a New React Application:
Navigate to the directory where you want to create your new React project. You can use the cd command to change directories.
For example:
cd path\to\your\directory
Create a new React application by running:
npx create-react-app my-app
4. Navigate to Your Project Directory:
Change to your project directory with:
cd my-app
5. Start the Development Server:
To see your new React app in action, start the development server by running:
bash
npm start
6. Open the Project in Your Code Editor:
7. Explore and Modify Your Project:
In Visual Studio Code, you can now explore the project structure. Key files include:
Start building your React application by modifying these files and adding new components as needed. Your React project is now set up and ready for development. You can begin creating components, managing state, and integrating with APIs as you build out your application.
While the core setup for React is complete with Node.js, npm, and a code editor, several additional tools and extensions can enhance your development experience. Here are some optional but highly recommended tools:
1. Git:
2. GitHub Desktop:
3. React Developer Tools:
4. Prettier:
5. ESLint:
6. Postman:
7. Storybook:
These tools and extensions can significantly enhance your productivity and streamline your development workflow when working with React. While optional, incorporating them into your setup can lead to a more efficient and organized development process.
Once your React.js setup is complete, it's time to dive into learning and developing with React. Here are some steps and resources to guide your journey:
1. Understand React Basics:
2. Follow Official Documentation:
3. Explore Tutorials and Courses:
4. Practice with Projects:
5. Use Community Resources:
6. Learn Advanced Concepts:
7. Participate in Open Source:
By following these steps, leveraging available resources, and practicing regularly, you'll build a solid understanding of React and enhance your skills as a React developer.
As you work with React.js, you might encounter various issues. Here’s a guide to troubleshooting common problems:
1. Installation Issues
Problem: Node.js or npm commands not recognized.
Problem: create-react-app command fails.
2. Project Creation Issues
Problem: Error during npx create-react-app my-app.
Problem: npm start doesn’t work or shows errors.
3. Code Editor Issues
Problem: Extensions not working in VS Code.
Problem: Code linting or formatting issues.
4. Development Server Issues
Problem: Development server not starting or crashes.
Problem: Application doesn’t update on saving files.
5. Component Issues
Problem: Components not rendering correctly.
Problem: Props or state not updating.
6. General Debugging Tips
By following these troubleshooting steps, you can address and resolve common issues encountered while developing with React.js, ensuring a smoother development experience.
Setting up and working with React.js on Windows involves several key steps, including installing Node.js and npm, choosing a code editor, and creating a new React project. By ensuring that you have the necessary prerequisites and following the installation and setup procedures, you can effectively start building React applications. Once your environment is ready, diving into React’s core concepts such as components, JSX, state, and props will provide a solid foundation for your development. Utilizing additional tools like Git, React Developer Tools, and code formatters can enhance your productivity and streamline your workflow.
Remember to leverage a variety of learning resources such as official documentation, online tutorials, and community forums to deepen your understanding of React and tackle any challenges you encounter. Engaging with open-source projects and contributing to the React community can further enrich your experience and help you stay current with best practices and advancements in the React ecosystem. By following these guidelines and continually learning and practicing, you'll be well-equipped to develop robust, dynamic web applications with React.js, setting the stage for a successful career in modern web development.
Copy and paste below code to page Head section
React.js is a JavaScript library developed by Facebook for building user interfaces, particularly single-page applications. It allows developers to create reusable UI components and manage the state of applications efficiently.
Yes, a basic understanding of JavaScript is essential for working with React. React builds upon JavaScript and requires knowledge of its core concepts to use and integrate React effectively.
Node.js provides the runtime environment for running React applications, while npm (Node Package Manager) is used to manage and install packages and dependencies required for React development.
Yes, you can use any code editor to work with React. However, Visual Studio Code is highly recommended due to its rich set of extensions and features that enhance JavaScript and React development.
Ensure you have a stable internet connection and that create-react-app is installed correctly. Try clearing the npm cache with npm cache clean --force and rerun the command. If the issue persists, check the error message for specific details and search for solutions online.
If the development server fails to start or crashes, check for error messages in the command line. Ensure you are in the correct project directory and no other processes are using port 3000. Restart the server and, if necessary, delete node_modules and package-lock.json before reinstalling dependencies with npm install.