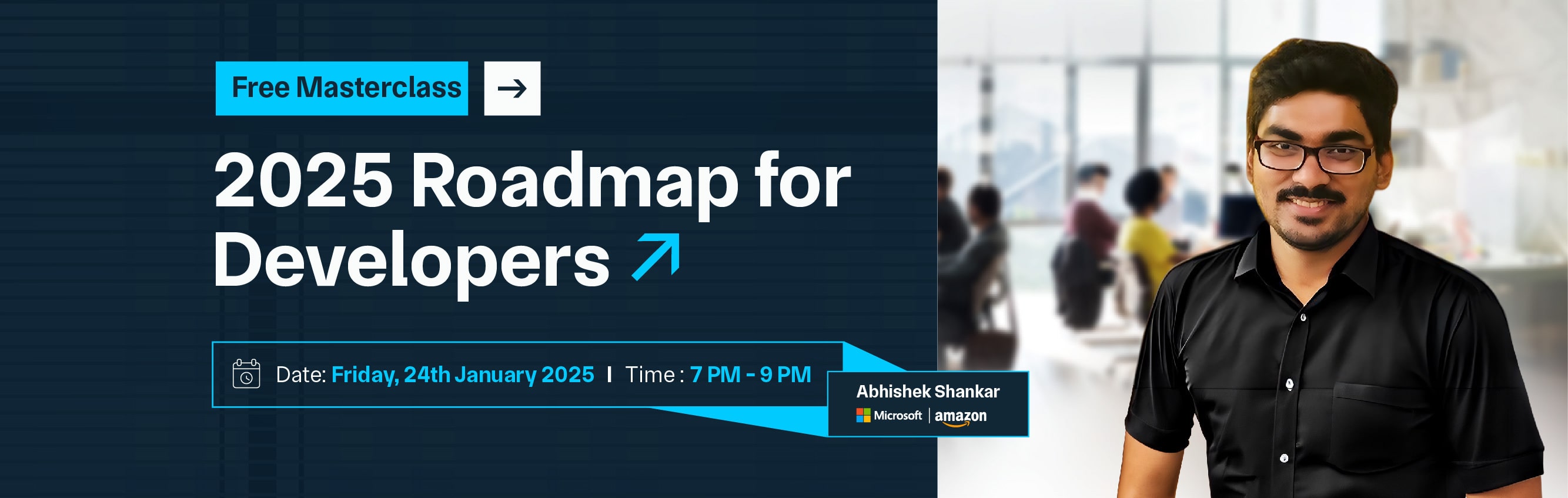

To install Angular, first ensure that Node.js and npm (Node Package Manager) are installed on your system, as Angular relies on them. You can download Node.js, which includes npm, from the Node.js website. After installing, check the versions with node -v, and npm -v to confirm they're set up correctly. Next, install Angular CLI (Command Line Interface) globally by running npm install -g @angular/cli in your terminal. This tool simplifies Angular project creation and management. To create a new Angular project, navigate to your desired directory and execute ng new project-name, replacing project-name with your chosen name.
This command sets up a new Angular application with a default configuration. Once the project is created, enter the project folder using cd project-name, and start the development server by running ng serve. You can then view your Angular application by opening http://localhost:4200 in a web browser. This process provides you with a fully functional Angular development environment. To install Angular, ensure Node.js and npm are installed.
Download them from the Node.js website, then verify the installation with node -v and npm -v. Install Angular CLI globally using npm install -g @angular/cli. Create a new project with ng new project name, replacing the project name with your preferred name. Navigate to your project folder with cd project-name and start the development server using ng serve. Access your Angular app by visiting http://localhost:4200 in your web browser. This setup enables you to begin developing Angular applications with ease.
Node.js is an open-source, cross-platform runtime environment that allows you to execute JavaScript code outside of a web browser. It’s built on Chrome's V8 JavaScript engine and is designed for building scalable network applications. Unlike traditional web servers that handle multiple requests in a single thread, Node.js uses an event-driven, non-blocking I/O model, which makes it highly efficient and suitable for real-time applications like chat services, online gaming, and live streaming.
Node.js also includes a package manager called npm (Node Package Manager), which provides access to a vast repository of libraries and tools that simplify the development of JavaScript applications.
With its robust ecosystem and ability to handle a large number of simultaneous connections, Node.js is widely used for server-side development, building APIs, and automating tasks in various types of applications.
To install Angular on Windows, first ensure you have Node.js and npm installed, as they are required for running Angular CLI. Familiarity with command-line tools and having a text editor or IDE will enhance your development experience.
Make sure you have administrative rights and a stable internet connection for downloading and setting up the necessary software. With these prerequisites in place, you're ready to start developing Angular applications.
Before installing Angular on a Windows system, ensure you have the following prerequisites:
Once these prerequisites are in place, you can proceed to install Angular and start developing Angular applications on your Windows machine.
To install Angular on Windows, start by setting up Node.js and npm, which are essential for Angular development. Next, use npm to install Angular CLI, which simplifies project management. Finally, create and run a new Angular project to begin development. Follow these steps to get your Angular environment up and running quickly.
Here’s a step-by-step guide to installing Angular on Windows:
1. Install Node.js and npm:
Verify the installation by opening Command Prompt and running:
node -v
npm -v
2. Install Angular CLI:
Open Command Prompt and install Angular CLI globally using npm:
npm install -g @angular/cli
Verify the installation by checking the Angular CLI version:
ng version
3. Create a New Angular Project:
Run the following command to create a new Angular project:
ng new my-angular-app
4. Navigate to the Project Folder:
Change into your project directory
cd my-angular-app
5. Run the Development Server:
Start the Angular development server by executing the following:
ng serve
By following these steps, you’ll have Angular set up and ready for development on your Windows machine.
To get started with Angular, the first step is to generate a new project using Angular CLI (Command Line Interface). This command sets up a fresh Angular application with a standard directory structure and essential configuration files.
Generate a New Project
ng new my-angular-app
Navigate to the Project Directory
cd my-angular-app
Angular CLI (Command Line Interface) provides a range of commands to simplify Angular development. Here are some commonly used Angular CLI commands:
Create a New Project:
ng new project-name
Serve the Application:
ng serve
Component:
ng generate component component-name
Service:
ng generate service service-name
Directive:
ng generate directive directive-name
Pipe:
ng generate pipe pipe-name
Module:
ng generate module module-name
Build the Project:
ng build
Run Tests:
ng test
Lint the Code:
ng lint
Update Angular CLI and Angular Dependencies:
ng update
Create a New Angular Application from a Template:
ng new project-name --style=scss
These commands streamline development tasks and help manage your Angular projects efficiently.
To verify your Angular installation on Windows, run the ng version in Command Prompt to check if Angular CLI is installed. Next, create and serve a new project with ng new test-angular-app and ng serve. Open http://localhost:4200 in a browser to see the default Angular welcome page, confirming that the installation is successful.
To test if Angular is properly installed on Windows, follow these steps:
Verify Angular CLI Installation:
Open Command Prompt and run:
ng version
Create and Serve a New Project:
Create a new Angular project by running:
ng new test-angular-app
Follow the prompts to set up the project. After the project is created, navigate to the project directory:
cd test-angular-app
Start the development server with the following:
ng serve
Check for Errors:
By successfully running these tests, you can confirm that Angular is correctly installed and configured on your Windows system.
To access the Angular web interface, start the development server with ng serve in your project directory. Then, open a browser and navigate to http://localhost:4200 to view your Angular application.
To access the Angular web interface, follow these steps:
Start the Development Server:
Run:
ng serve
Open Your Browser:
Navigate to the Localhost URL:
Enter the following URL in the browser's address bar:
http://localhost:4200
You should see your Angular application's web interface, confirming that it's accessible and running correctly.
To use Angular, start by installing Node.js and Angular CLI. Create a new project with ng new project name, then serve it locally using ng serve. Develop your app by generating components, modifying code, and building for production with ng build --prod. Run tests using the ng test to ensure everything works correctly.
To use Angular, follow these steps:
Set Up Your Environment:
Install Angular CLI globally with:
npm install -g @angular/cli
Create a New Angular Project:
Generate a new project using Angular CLI:
ng new my-angular-app
Navigate into your project directory:
cd my-angular-app
Serve the Application:
Start the development server:
ng serve
Develop Your Application:
Generate Components: Create new components with:
ng generate component component-name
Build for Production:
Compile the project for production use:
ng build --prod
Run Tests:
Execute unit tests to ensure your application works as expected:
ng test
By following these steps, you can develop, test, and deploy Angular applications efficiently.
To uninstall Angular from Windows, first, remove Angular CLI using npm uninstall -g @angular/cli in Command Prompt. Then, delete any Angular project folders manually. Optionally, clean the npm cache with npm cache clean --force. Verify the uninstallation by running the ng version, which should show an error if successful.
To uninstall Angular from Windows, follow these steps:
1. Uninstall Angular CLI:
Open Command Prompt and run:
npm uninstall -g @angular/cli
2. Remove Angular Project Files:
3. Clean npm Cache (Optional):
To clean up the npm cache and free up space, run the following:
npm cache clean --force
4. Verify Removal:
Check that Angular CLI is removed by running:
ng version
By following these steps, you will have removed Angular CLI and your Angular project files from your Windows system.
Angular is a powerful framework for building dynamic web applications. Installing Angular on Windows involves setting up Node.js, installing Angular CLI, and creating a project to start development. To use Angular effectively, you’ll generate components, serve your application locally, and build it for production.
When no longer needed, you can uninstall Angular by removing Angular CLI, deleting project files, and cleaning the npm cache. Mastering Angular’s tools and commands will streamline your development process, enabling you to create robust, scalable applications with ease.
Copy and paste below code to page Head section
Angular is a TypeScript-based open-source framework developed by Google for building single-page applications (SPAs). It provides a comprehensive set of tools for developing web applications, including modules, components, services, and dependency injection.
Use Angular CLI to create a new project by running ng new project-name in your Command Prompt or terminal. Navigate into your project directory with cd project-name and start the development server using ng serve.
After starting the development server with ng serve, open your web browser and go to http://localhost:4200 to view your Angular application.
Common commands include ng serve (start the development server), ng build (build the application), ng generate component component-name (generate a new component), and ng test (run unit tests).
To update Angular CLI, run npm install -g @angular/cli. To update Angular dependencies in your project, use ng update.
Uninstall Angular CLI with npm uninstall -g @angular/cli, delete Angular project folders manually, and optionally clean npm cache with npm cache clean --force.