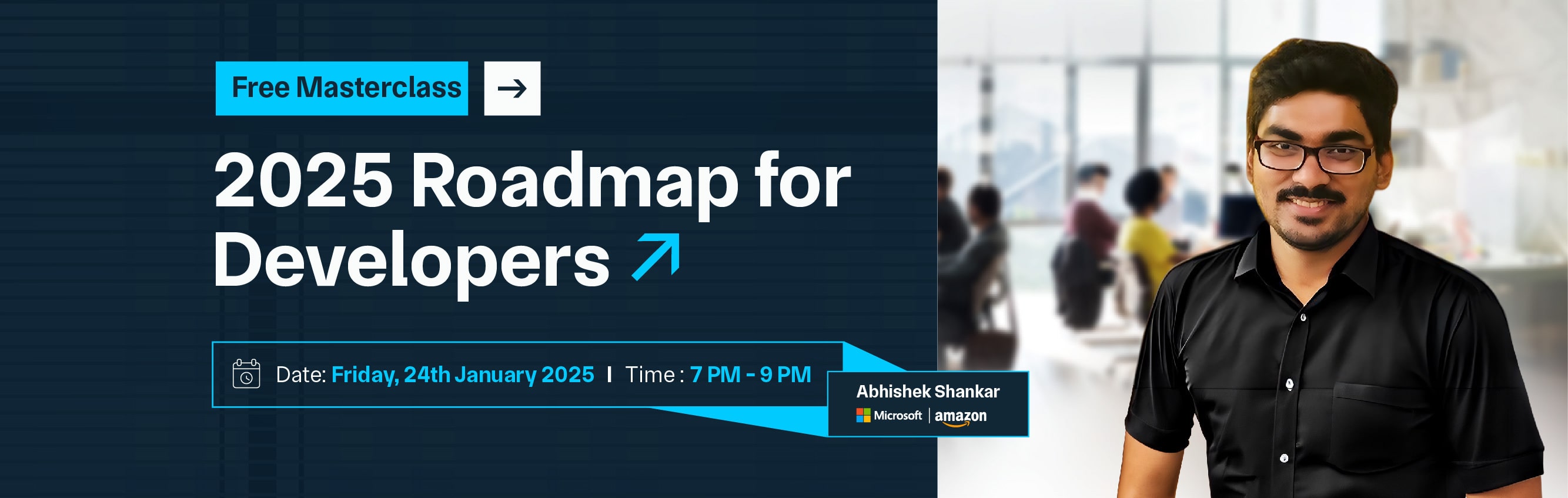

Installing Bootstrap in an Angular project is a straightforward process that enhances your app’s design with pre-built responsive components. First, ensure you have Angular CLI installed. Open your terminal, navigate to your Angular project directory, and install Bootstrap using npm by running npm install bootstrap. Next, you need to include Bootstrap’s CSS in your Angular project. Open angular.json and locate the styles array within the build options. Add the Bootstrap CSS file path: "node_modules/bootstrap/dist/css/bootstrap.min.css".
This integration will make Bootstrap’s styles available throughout your application. For Bootstrap’s JavaScript components, such as modals or tooltips, also install @popperjs/core and bootstrap by running npm install @popperjs/core bootstrap. Ensure you include Bootstrap’s JavaScript in your project by updating the scripts array in angular.json with the path to Bootstrap’s JS file: "node_modules/bootstrap/dist/js/bootstrap.bundle.min.js".
After these steps, you can start using Bootstrap classes in your Angular components to create responsive layouts and stylish components effortlessly. This setup not only simplifies styling but also leverages Bootstrap’s powerful framework to enhance your app’s user interface.To complete the setup, restart your Angular development server to apply changes. Now, Bootstrap's styles and components should be fully integrated and ready for use.
Bootstrap is a popular front-end framework designed to simplify web development by providing pre-designed, responsive components and styles. Created by Twitter, it offers a comprehensive toolkit that includes a grid system, various UI components, and extensive utility classes. The grid system divides the page into a flexible 12-column layout, allowing for easy, responsive design adjustments across different screen sizes.
Bootstrap components such as buttons, navbars, modals, and carousels come with built-in styles and functionalities, reducing the need for custom CSS and JavaScript. Utility classes in Bootstrap help in applying common styles, such as margins, padding, and text alignment, quickly and consistently.
The framework supports customization through variables and theming, enabling developers to tailor Bootstrap to match their design needs. By using Bootstrap, developers can speed up the development process, ensure a consistent design, and enhance the responsiveness of their web applications, making it a valuable tool for both beginners and experienced developers.
Bootstrap, originally developed by Twitter engineers Mark Otto and Jacob Thornton, was first released in 2011. It emerged from a need for a unified and standardized toolkit to streamline web development within the company. Initially named "Twitter Blueprint," Bootstrap was designed to address inconsistencies in their internal tools and improve the efficiency of front-end development.
The framework quickly gained popularity for its clean design, responsive grid system, and extensive library of pre-designed components. In 2012, Bootstrap was officially released as an open-source project, broadening its reach to the global web development community. This move catalyzed its adoption across various industries, cementing its role as a fundamental resource in modern web design.
Since then, Bootstrap has undergone several major updates, with significant improvements in functionality, customization options, and modern design practices. Today, it remains one of the most widely used front-end frameworks, continually evolving to meet the demands of contemporary web development and providing developers with the tools to create visually appealing, responsive, and functional websites efficiently.
Integrating Bootstrap into your Angular project can significantly enhance your app's design and functionality. Here are the top ways to include Bootstrap in your Angular project:
1. Using npm Installation: The most common method is to install Bootstrap via npm. Run npm install bootstrap in your project’s root directory. After installation, update the angular.json file to include Bootstrap’s CSS and JavaScript. Add "node_modules/bootstrap/dist/css/bootstrap.min.css" to the styles array and "node_modules/bootstrap/dist/js/bootstrap.bundle.min.js" to the scripts array. This method ensures that Bootstrap’s styles and components are globally available in your project.
2. Using Angular CLI: For a streamlined integration, you can use Angular CLI commands. Install Bootstrap with ng add @ng-bootstrap/ng-bootstrap which adds Bootstrap’s CSS and necessary dependencies for Angular. This method also configures Angular to use Bootstrap components that are compatible with Angular’s framework.
3. Linking via CDN: If you prefer not to add Bootstrap via npm, you can link to Bootstrap’s CDN in your index.html file. Add the following links to the <head> section for CSS and the end of the <body> section for JavaScript:
<link rel="stylesheet" href="https://stackpath.bootstrapcdn.com/bootstrap/4.5.2/css/bootstrap.min.css">
<script src="https://stackpath.bootstrapcdn.com/bootstrap/4.5.2/js/bootstrap.bundle.min.js"></script>
This method is quick but less flexible for customizations.
4. Using Angular Libraries: Integrate specialized libraries like ngx-bootstrap or ng-bootstrap for Angular-specific Bootstrap components. Install with npm install ngx-bootstrap or npm install @ng-bootstrap/ng-bootstrap. These libraries provide Angular wrappers for Bootstrap components, ensuring compatibility and enhanced integration with Angular features.
Each method offers different benefits depending on your project requirements and preferences. Choose the one that best fits your development workflow and project needs.
Pros of Bootstrap
Cons of Bootstrap
Bootstrap is a powerful framework that offers numerous advantages in terms of speed, consistency, and responsiveness. However, it’s important to be aware of potential drawbacks, such as design uniformity and additional overhead, and to customize it according to your project’s needs to maximize its benefits.
Before installing Bootstrap in your Angular project, it's essential to ensure that you have met the following prerequisites:
1. Node.js and npm: Ensure that you have Node.js and npm (Node Package Manager) installed on your development machine. Bootstrap and Angular dependencies are managed via npm, so you need these tools for package installation. You can download Node.js from nodejs.org, which includes npm.
2. Angular CLI: Install Angular CLI if you haven’t already. Angular CLI is a command-line interface tool that helps in creating and managing Angular projects. You can install it globally using npm with the command npm install -g @angular/cli.
3. An Angular Project: Make sure you have an Angular project set up. If you don’t have one, you can create a new Angular project by running ng new your-project-name in your terminal. Navigate into your project directory with cd your-project-name.
4. Basic Understanding of Angular: Familiarize yourself with the basics of Angular, including how to work with components, modules, and the Angular CLI. This understanding will help you integrate Bootstrap effectively.
5. Familiarity with Angular Configuration Files: Understanding how to modify Angular configuration files such as angular.json is crucial for integrating Bootstrap. You’ll need to add Bootstrap’s CSS and possibly JavaScript files to these configuration files.
6. Editor or IDE: Have a code editor, or integrated development environment (IDE) set up, such as Visual Studio Code, WebStorm, or any editor of your choice that supports Angular development.
With these prerequisites in place, you’re ready to install and integrate Bootstrap into your Angular project, enabling you to leverage its responsive and stylish components efficiently.
Using a Content Delivery Network (CDN) is a straightforward method to integrate Bootstrap into your Angular project without adding it to your local dependencies. This approach is useful for quick setup or when you prefer to keep your project's dependency management the same.
Here’s how to do it:
1. Open index.html: In your Angular project, locate the index.html file found in the src folder. This file is where you will add the links to Bootstrap’s CDN.
Add Bootstrap CSS: In the <head> section of your index.html, add the following link to include Bootstrap's CSS:
<link rel="stylesheet" href="https://stackpath.bootstrapcdn.com/bootstrap/5.3.0/css/bootstrap.min.css">
2. This line loads the latest version of Bootstrap’s CSS from a CDN, making its styles available throughout your application.
Add Bootstrap JavaScript (Optional): If you need Bootstrap’s JavaScript components, such as models or tooltips, add the following script tag just before the closing </body> tag:
<script src="https://stackpath.bootstrapcdn.com/bootstrap/5.3.0/js/bootstrap.bundle.min.js"></script>
3. This script includes Bootstrap's JavaScript functionality, along with Popper.js, which is required for some components to function properly.
4. Save Changes: After adding the CDN links, save your index.html file. Your Angular project is now set up to use Bootstrap via CDN.
5. Restart Development Server: If your Angular development server is running, it may automatically reload the changes. If not, restart it using ng serve to apply the updates.
Advantages of Using CDN
Considerations
Using a CDN is an effective way to quickly incorporate Bootstrap into your Angular project, especially for prototype or initial development stages.
Installing Bootstrap via npm (Node Package Manager) is a preferred method for integrating the framework into your Angular project, offering more control and flexibility. This approach allows you to manage Bootstrap and its dependencies within your project’s local environment.
Here’s a step-by-step guide:
1. Open Your Terminal: Navigate to your Angular project directory using your terminal or command prompt.
Install Bootstrap: Run the following command to install Bootstrap and its dependencies:
npm install bootstrap
2. This command downloads Bootstrap and adds it to your project’s node_modules folder.
3. Include Bootstrap CSS:
Locate the styles array within the build options of your project configuration. Add the path to Bootstrap’s CSS file:
"styles": [
"src/styles.css",
"node_modules/bootstrap/dist/css/bootstrap.min.css"
]
4. This line ensures that Bootstrap’s styles are included in your global stylesheet.
5. Include Bootstrap JavaScript (Optional):
Update the scripts array in angular.json with the following:
"scripts": [
"node_modules/@popperjs/core/dist/umd/popper.min.js",
"node_modules/bootstrap/dist/js/bootstrap.bundle.min.js"
]
6. This ensures that Bootstrap’s interactive components work correctly.
7. Restart Your Development Server: If your Angular development server is running, it should automatically pick up these changes. If not, restart it using ng serve to see the effects.
Advantages of Using npm:
Considerations:
Using npm to install Bootstrap provides greater flexibility and control, making it an excellent choice for production applications and projects where maintaining a consistent and manageable codebase is crucial.
Integrating Bootstrap into your Angular project using Angular CLI is a streamlined approach that simplifies the setup process. This method leverages Angular CLI’s built-in capabilities to add Bootstrap and its dependencies efficiently.
Here’s how to do it:
1. Open Your Terminal: Navigate to your Angular project directory using your terminal or command prompt.
Add Bootstrap Using Angular CLI: Run the following command to add Bootstrap and the necessary Angular-specific Bootstrap library:
ng add @ng-bootstrap/ng-bootstrap
2. This command installs the ng-bootstrap library, which provides Angular components built on top of Bootstrap. It also automatically configures your Angular project to include Bootstrap's CSS.
Install Bootstrap and Popper.js: If the Angular CLI setup doesn’t automatically include Bootstrap, manually install Bootstrap and Popper.js using npm. Run:
npm install bootstrap @popperjs/core
3. This command ensures you have the latest versions of Bootstrap and Popper.js, which is required for Bootstrap’s JavaScript components.
4. Include Bootstrap CSS:
Find the styles array within the build options. Add Bootstrap’s CSS file path:
"styles": [
"src/styles.css",
"node_modules/bootstrap/dist/css/bootstrap.min.css"
]
5. This step includes Bootstrap’s styles globally in your Angular application.
6. Configure Bootstrap JavaScript (Optional):
For components requiring Bootstrap’s JavaScript, ensure you have Popper.js included. Update the scripts array in angular.json if necessary:
"scripts":
[
"node_modules/@popperjs/core/dist/umd/popper.min.js,"
"node_modules/bootstrap/dist/js/bootstrap.bundle.min.js"
]
7. Adding these scripts ensures that interactive Bootstrap components function correctly.
8. Restart Your Development Server: If your Angular development server is running, it should automatically detect these changes. If not, restart it with ng serve to apply the updates.
Advantages of Using Angular CLI:
Considerations:
Using Angular CLI to install Bootstrap is an effective method for integrating Bootstrap with Angular, particularly if you’re already using Angular CLI and seek a streamlined setup with Angular-specific Bootstrap components.
Bower is a package manager that was widely used for front-end libraries before the rise of npm and Yarn. While Bower is no longer actively maintained and npm is generally preferred, you might encounter projects that use Bower or prefer it for legacy reasons. Here’s how you can install Bootstrap using Bower:
Install Bower (If Not Installed): If Bower is not installed on your system, you can install it globally via npm with the following command:
npm install -g bower
1. This command ensures that Bower is available on your system to manage front-end packages.
Initialize Bower in Your Project: Navigate to your Angular project directory using your terminal or command prompt, and initialize Bower if it hasn’t been set up already:
bower init
2. Follow the prompts to create a bower.json file in your project’s root directory, which will manage your Bower dependencies.
Install Bootstrap: Run the following command to install Bootstrap via Bower:
Bower install bootstrap --save
3. The --save flag adds Bootstrap to the bower.json file’s dependencies, ensuring Bower tracks it.
4. Include Bootstrap CSS:
Add a link to the Bootstrap CSS file provided by Bower in the <head> section:
<link rel="stylesheet" href="bower_components/bootstrap/dist/css/bootstrap.min.css">
5. This line ensures that Bootstrap’s styles are applied globally.
6. Include Bootstrap JavaScript (Optional):
For Bootstrap’s JavaScript components, you also need to include the JavaScript files. Add the following script tags just before the closing </body> tag in index.html:
<script src="bower_components/bootstrap/dist/js/bootstrap.bundle.min.js"></script>
7. This step ensures that Bootstrap’s interactive components are functional.
8. Restart Your Development Server: If your Angular development server is running, it should pick up these changes automatically. Otherwise, restart it using ng serve to apply the updates.
Advantages of Using Bower:
Considerations:
Using Bower to install Bootstrap can be appropriate for maintaining older projects or specific environments where Bower is still in use. However, for new projects, it’s recommended to use npm or other modern package managers to take advantage of more current tools and practices.
Using a custom build of Bootstrap allows you to tailor the framework to your specific needs, optimizing both performance and design. This method involves customizing Bootstrap’s source files and creating a build that includes only the components and styles you need. Here’s a step-by-step guide to integrating Bootstrap into your Angular project with a custom build:
Set Up Your Project: Ensure you have an Angular project set up with Angular CLI. If not, you can create one using:
ng new your-project-name
Navigate to your project directory:
cd your-project-name
1. Install Bootstrap and Dependencies: First, install Bootstrap and its dependencies using npm. Run:
npm install bootstrap @popperjs/core
2. This installs Bootstrap along with Popper.js, which is necessary for Bootstrap’s JavaScript components.
Install Bootstrap’s Source Files: To create a custom build, you need the source files for Bootstrap. These are typically SASS files that allow you to customize Bootstrap. Install the bootstrap and bootstrap-sass packages if not already included:
npm install bootstrap-sass
3. Configure SASS for Customization: If your Angular project uses SASS (SCSS) for styling, configure it to use Bootstrap’s SASS files:
Import Bootstrap’s SASS files into your styles.scss:
// Import Bootstrap's SASS files
@import "~bootstrap/scss/bootstrap";
Customise Bootstrap by overriding default variables before importing Bootstrap’s SASS. For example, to change the primary color:
$primary: #ff5733; // Your custom color
@import "~bootstrap/scss/bootstrap";
4. Build Your Custom CSS:
Ensure your angular.json file is set up to include the custom styles. scss. Update the styles array:
"styles": [
"src/styles.scss"
]
5. Include JavaScript (Optional): If you need Bootstrap’s JavaScript components, make sure to include them in angular.json:
"scripts": [
"node_modules/@popperjs/core/dist/umd/popper.min.js",
"node_modules/bootstrap/dist/js/bootstrap.bundle.min.js"
]
6. Restart Your Development Server: Run or restart your Angular development server with the following:
ng serve
Advantages of a Custom Build:
Considerations:
Using a custom build of Bootstrap provides significant flexibility and optimization benefits, making it a great choice for projects with specific design needs and performance considerations.
When installing Bootstrap in your project, following best practices ensures that you leverage the framework's benefits effectively while maintaining performance, flexibility, and manageability. Here are some key best practices:
1. Choose the Right Installation Method: Select an installation method that aligns with your project’s needs:
2. Optimize Bootstrap Build: If using npm or custom builds, include only the Bootstrap components and utilities you need. This reduces the file size and improves performance:
3. Update Regularly: Keep Bootstrap and its dependencies up to date to benefit from the latest features, improvements, and security patches. Regular updates help prevent compatibility issues and security vulnerabilities:
4. Use Bootstrap Responsibly: Avoid over-relying on Bootstrap’s default styles to maintain uniqueness in your design:
5. Leverage the Grid System Wisely: Utilize Bootstrap’s grid system to create responsive layouts, but be mindful of its limitations:
6. Incorporate JavaScript Components Judiciously: Include Bootstrap’s JavaScript components only if necessary:
7. Test Across Browsers and Devices: Ensure that your application functions correctly across various browsers and devices:
8. Maintain Accessibility: Bootstrap provides accessibility features, but it’s crucial to ensure that your implementation adheres to accessibility best practices:
9. Document Customizations: If you customize Bootstrap or integrate it with other tools, document your changes:
By adhering to these best practices, you can maximize the benefits of Bootstrap while ensuring your project remains performant, maintainable, and aligned with your design and accessibility goals.
When considering the integration of Bootstrap into your project, understanding its advantages helps in leveraging the framework effectively. Here are some key advantages of using Bootstrap:
Overall, Bootstrap’s advantages lie in its ability to streamline development, ensure design consistency, and provide responsive, customizable, and accessible components. These benefits make it a popular choice for building high-quality web applications efficiently.
Bootstrap stands out as a powerful and versatile framework that significantly enhances the development process for web applications. Its comprehensive library of pre-designed components, responsive grid system, and extensive customization options streamline the creation of modern, visually appealing, and functional web interfaces. By facilitating rapid development, ensuring consistent design, and providing robust cross-browser compatibility, Bootstrap helps developers deliver high-quality user experiences efficiently.
The framework’s strong community support, extensive documentation, and modular components further contribute to its effectiveness, making it a valuable tool for both novice and experienced developers. Whether using a CDN for quick setups, npm for detailed dependency management, or custom builds for tailored performance; Bootstrap offers flexibility to meet various project needs. However, while Bootstrap provides numerous advantages, it’s essential to integrate it thoughtfully to avoid potential drawbacks such as design uniformity or unnecessary overhead. By adhering to best practices—such as optimizing builds, customizing styles, and maintaining accessibility—developers can fully leverage Bootstrap's capabilities while ensuring their projects remain unique and performant.
Copy and paste below code to page Head section
Bootstrap is an open-source front-end framework developed by Twitter, designed to streamline web development. It provides a collection of CSS and JavaScript components, including a responsive grid system, typography, forms, buttons, navigation bars, and various UI elements, helping developers create visually appealing and responsive websites quickly and consistently.
Yes, Bootstrap is highly customizable. You can override default styles using SASS variables or custom CSS to tailor Bootstrap’s components to match your design requirements. You can also create a custom build by including only the components you need, which helps optimize performance and reduces file size.
Yes, Bootstrap is designed with mobile-first principles in mind. Its responsive grid system and utility classes ensure that websites and applications built with Bootstrap work seamlessly across different devices and screen sizes, from mobile phones to desktops.
Bootstrap’s JavaScript components, such as modals, tooltips, and dropdowns, enhance interactivity and functionality. You only need to include these if your project requires them. For a lighter build, you can include only the JavaScript components you use.
Bootstrap includes built-in accessibility features and follows best practices to improve accessibility. Components are designed to be accessible out of the box, with support for ARIA attributes and keyboard navigation. However, it’s important to test your application with assistive technologies and follow web accessibility guidelines.
Yes, Bootstrap can be used alongside other frameworks and libraries, such as Angular, React, and Vue.js. Many of these frameworks have specific integrations or wrappers for Bootstrap to ensure compatibility and enhance functionality.