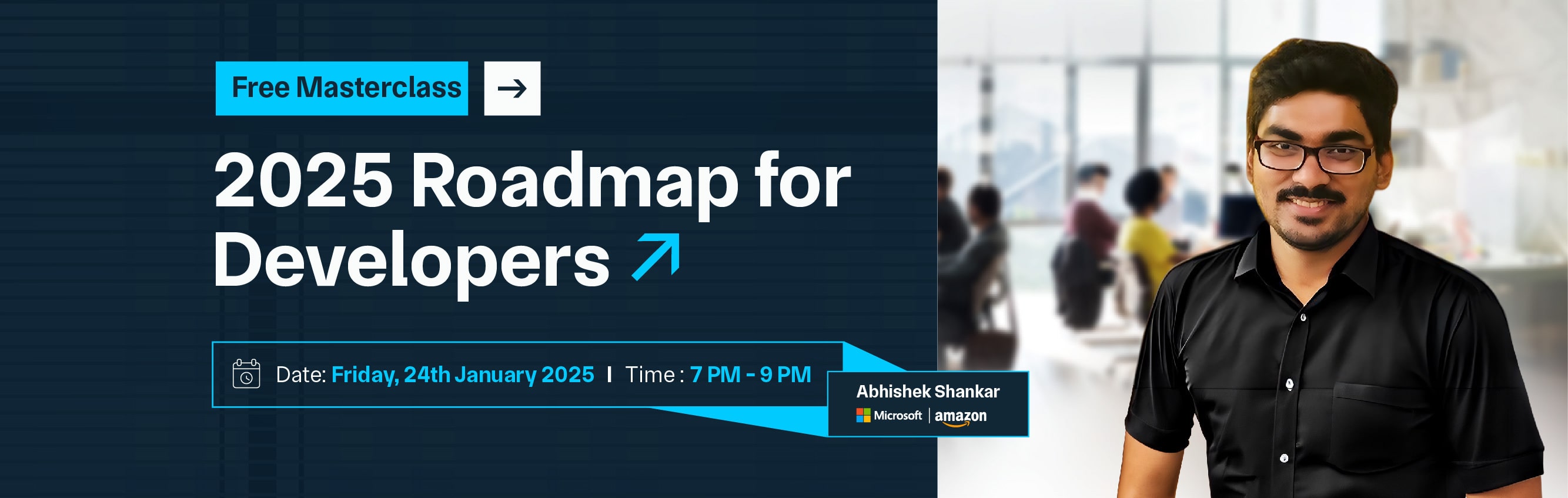

This metadata helps Angular to render components correctly and handle their interactions within the application's structure. Similarly, the @Injectable decorator marks services that can be injected into other components or services, facilitating a modular and reusable code structure. Moreover, decorators like @NgModule define Angular modules, consolidating related components, directives, and services into cohesive units.
They specify how different parts of an application fit together and enable Angular to optimise loading and performance. Additionally, decorators like @Input and @Output facilitate data communication between components, allowing parent components to pass data down to child components (@Input) and child components to emit events and pass data back up to parent components (@Output). Overall, decorators in Angular streamline development by adding necessary metadata and functionality to JavaScript classes, making it easier to build scalable, maintainable, and efficient web applications.
In Angular, decorators are powerful functions prefixed with the @ symbol that modify the behavior or properties of classes, methods, properties, or parameters in TypeScript. They are crucial in defining and configuring various elements within an Angular application, such as components, services, and modules. Decorators provide essential metadata that Angular uses to understand how these elements should behave and interact with each other.
For example, the @Component decorator defines a component by specifying its selector, template, and styles. In contrast, the @Injectable decorator marks a service class as injectable, allowing it to be used as a dependency in other application parts. Similarly, the @NgModule decorator defines Angular modules, which organise and consolidate related components, services, and other features.
Decorators like @Input and @Output facilitate communication between components by enabling data binding and event handling. Overall, decorators in Angular streamline development by adding necessary metadata and functionality to TypeScript classes, contributing to the framework's modular and efficient architecture.
In Angular, decorators are extensively used to enhance classes with metadata that Angular's compiler uses to transform them as needed.
Decorators are typically prefixed with the @ symbol and are applied just before a class, method, property, or parameter declaration. Here are the main types of decorators used in Angular:
Angular supports four types of decorators
Class decorators are applied directly before a class declaration. They are used to configure or transform the class itself. Class decorators in Python are functions that modify the behavior or structure of a class. They are applied using the @decorator_name syntax immediately before the class definition. When a class decorator is used, it replaces or enhances the class definition with the result of calling the decorator function.
Class decorators can add new attributes or methods to a class, modify existing methods, or even completely replace the class with a new implementation. They are often used for metaprogramming tasks, adding cross-cutting concerns to classes, or implementing design patterns dynamically based on class definitions.
@Component: Defines an Angular component by specifying metadata such as selector, template, styles, etc.
Example:
import { Component } from '@angular/core';
@Component({
selector: 'app-example',
templateUrl: './example.component.html',
styleUrls: ['./example.component.css']
})
export class ExampleComponent {
// Component logic here
}
@Directive: Declares an Angular directive, which adds behavior to a DOM element.
Example:
import { Directive, ElementRef, HostListener } from '@angular/core';
@Directive({
selector: '[appHighlight]'
})
export class HighlightDirective {
constructor(private el: ElementRef) {}
@HostListener('mouseenter') onMouseEnter() {
this.highlight('yellow');
}
@HostListener('mouseleave') onMouseLeave() {
this.highlight(null);
}
private highlight(color: string | null) {
this.el.nativeElement.style.backgroundColor = color;
}
}
@Pipe: Declares an Angular pipe, which transforms input data in templates.
Example:
import { Pipe, PipeTransform } from '@angular/core';
@Pipe({
name: 'capitalize'
})
export class CapitalizePipe implements PipeTransform {
transform(value: string): string {
if (!value) return '';
return value.charAt(0).toUpperCase() + value.slice(1);
}
}
Property decorators are applied to a property declaration within a class. They are used to configure or transform the property. Property decorators in Python are used to define properties within a class. They allow computed attributes to be defined without explicitly calling methods like getter, setter, and deleter.
The @property decorator is used to define a method as a property getter, which allows accessing an attribute as if it were a regular attribute. Additionally, @property.setter and @property.deleter decorators can be used to define methods that handle setting and deleting property values, respectively. Property decorators facilitate clean and concise attribute management, encapsulating logic for attribute access and modification within a class.
@Input: Declares an input property, allowing data to flow into a component from its parent component.
Example:
import { Component, Input } from '@angular/core';
@Component({
selector: 'app-child',
template: '<p>{{ childTitle }}</p>'
})
export class ChildComponent {
@Input() childTitle: string;
}
@Output: Declares an output property, allowing a component to emit events to its parent component.
Example:
import { Component, Output, EventEmitter } from '@angular/core';
@Component({
selector: 'app-child',
template: '<button (click)="sendMessage()">Send Message</button>'
})
export class ChildComponent {
@Output() message event = new EventEmitter<string>();
sendMessage() {
this.messageEvent.emit('Message from child');
}
}
Method decorators are applied to a method declaration within a class. They are used to configure or transform the method. Method decorators in Python are functions that modify the behavior of methods within a class. They are applied using the @decorator_name syntax before the method definition.
Method decorators receive the method they decorate as the first argument (self, for instance, methods, class for class methods), allowing them to wrap or modify the behavior of the method. Method decorators are commonly used for implementing cross-cutting concerns such as logging, input validation, caching, and access control. They enhance code modularity and reusability by separating method-specific logic from cross-cutting concerns.
@HostListener: Declares a DOM event listener for a host element in a directive.
Example:
import { Directive, HostListener } from '@angular/core';
@Directive({
selector: '[appClick]'
})
export class ClickDirective {
@HostListener('click', ['$event'])
onClick(event: MouseEvent) {
console.log('Element clicked!', event);
}
}
Parameter decorators are applied to a parameter declaration within a method or constructor of a class. They are used to configure or transform the parameter. Parameter decorators in Python are less common and are used to modify the behavior of function or method parameters. They are applied using a special syntax similar to function decorators but are less frequently used compared to class, property, and method decorators.
Parameter decorators receive the parameter they decorate as an argument and can wrap or modify the behavior of the function or method based on the parameter value. Parameter decorators can be used for tasks such as validating input parameters, preprocessing data before passing it to the function or implementing additional behavior specific to function arguments.
@Inject: Specifies a dependency injection token for a parameter in a class constructor.
Example:
import { Injectable, Inject } from '@angular/core';
import { HttpClient } from '@angular/common/http';
@Injectable({
provided: 'root'
})
export class DataService {
constructor(private http: HttpClient, @Inject('API_URL') private apiUrl: string) {}
getData() {
return this.http.get(`${this.apiUrl}/data`);
}
}
In Angular, decorators are functions that provide a way to add metadata to classes, methods, properties, and parameters at design time. They are prefixed with the @ symbol and are widely used throughout Angular applications to enhance and configure various elements.
Decorators in Angular serve several key purposes.
Decorators play a crucial role in Angular development by allowing developers to specify how classes and their members should behave or interact within the framework. They help reduce boilerplate code, improve code readability, and facilitate the modular design of Angular applications. Understanding and effectively using decorators is essential for building scalable, maintainable, and feature-rich Angular applications.
Decorators in Angular are essential annotations prefixed with the @ symbol that provide metadata to classes, methods, properties, or parameters. They play a crucial role in defining components (@Component), services (@Injectable), and modules (@NgModule), facilitating dependency injection, modularization, and efficient code organization.
Decorators like @Input and @Output enable data binding and event handling between components, while @ViewChild and @ViewChildren allow accessing child components or elements within a parent component's template.
Use @Component to define Angular components with metadata like selectors, templates, and styles. In Angular, the @Component decorator defines components by associating metadata with TypeScript classes. It specifies crucial details such as the component's selector, HTML template, CSS styles, and more.
This metadata enables Angular to render components dynamically and manage their lifecycle effectively. Components encapsulate logic, UI, and behavior into reusable units, promoting modular and maintainable application development.
Mark services with @Injectable for dependency injection across components and services. The @Injectable decorator marks TypeScript classes as injectable services within the Angular dependency injection (DI) framework.
Services annotated with @Injectable can be injected as dependencies into components, directives, or other services. This facilitates code reuse and separation of concerns and promotes scalable architecture by enabling efficient management of application-wide services and business logic.
Utilise @NgModule to define Angular modules, grouping related components, directives, and services. Angular uses the @NgModule decorator to define modules, which are containers for organizing related components, directives, pipes, and services.
@NgModule metadata specifies module dependencies, declarations, imports, and providers. This modular approach promotes code organization separation of concerns and facilitates lazy loading for optimizing application performance. Modules encapsulate functionality, making it easier to manage dependencies and promote reusability across different parts of an application.
Use @Input to pass data into a component and @Output to emit events out of a component. The @Input and @Output decorators facilitate communication between Angular components. @Input allows passing data from parent to child components, enabling dynamic data binding.
@Output emits events from child to parent components, enabling interaction and communication upwards in the component hierarchy. These decorators enhance flexibility and interactivity within Angular applications, enabling seamless data flow and event handling between components.
Employ @ViewChild and @ViewChildren to access child components or DOM elements from a parent component. The @ViewChild and @ViewChildren decorators enable Angular components to access child components or DOM elements within their templates.
@ViewChild provides a reference to a single child component or DOM element, while @ViewChildren provides access to multiple child components or elements. These decorators facilitate interaction and manipulation of child components or elements from within parent components, enabling dynamic and responsive UI behaviour in Angular applications.
Create custom decorators to add specific behaviour or metadata to classes, methods, or properties, enhancing code organization and reusability. Angular allows the creation of custom decorators to extend or modify the behaviour and metadata of TypeScript classes, methods, or properties.
Custom decorators can encapsulate specific functionality, such as logging, caching, or authorization checks, enhancing code organization and reusability. By encapsulating cross-cutting concerns within decorators, Angular promotes cleaner, more maintainable code and facilitates consistent application of business logic across components and services.
Decorators in Angular streamline development by adding essential metadata and functionality to TypeScript classes, promoting modularity, maintainability, and efficiency within applications.
Creating custom decorators in Angular allows you to extend the framework's functionality by adding specific behaviours or metadata to classes, methods, properties, or parameters. Here’s a step-by-step guide on how to create a custom decorator in Angular:
function CustomDecorator(target: any, key: string): void {
// target is the class prototype if applied to a method or property
// key is the name of the decorated method or property
console. log(`Custom decorator called on ${key} of ${target.constructor.name}`);
}
class ExampleClass {
@CustomDecorator
example method() {
// method logic
}
}
Understand the Decorator Function Parameters: Depending on where you apply the decorator, the parameters target and key will vary:
function CustomLog(target: any, key: string): void {
let value = target[key];
const getter = () => {
console.log(`Getting value: ${value}`);
return value;
};
const setter = (val: any) => {
console.log(`Setting value: ${val}`);
value = val;
};
// Replace the property with a new getter/setter
Object.defineProperty(target, key, {
get a getter,
set: setter,
enumerable: true,
configurable: true,
});
}
class ExampleClass {
@CustomLog
private _value: string = '';
getValue(): string {
return this._value;
}
setValue(val: string): void {
this._value = val;
}
}
const instance = new ExampleClass();
instance.setValue('Hello'); // Setting value: Hello
console.log(instance.getValue()); // Getting value: Hello
By following these steps, you can effectively create custom decorators in Angular to encapsulate reusable behaviour or add metadata to your classes, methods, properties, or parameters, enhancing the maintainability and flexibility of your application's architecture.
Using decorators in Angular is fundamental for adding metadata and functionality to TypeScript classes, methods, properties, and parameters. Decorators are annotations prefixed with the @ symbol that modify or extend the behaviour of these elements, enhancing the organization, reusability, and maintainability of Angular applications.
Whether defining components, services, modules, or custom functionality, decorators in Angular streamline development by providing a declarative way to enrich classes with additional capabilities. They enable features like dependency injection, data binding, event handling, and more, making it easier to build dynamic and scalable applications. Let's explore a practical example to illustrate how decorators are applied in Angular components.
Create a Component
First, let's create an Angular component using the @Component decorator. This decorator defines metadata for the component, such as its selector, template, styles, etc.
import { Component } from '@angular/core';
@Component({
selector: 'app-example',
templateUrl: './example.component.html',
styleUrls: ['./example.component.css']
})
export class ExampleComponent {
// Component logic goes here
}
1. In this example:
Use the Component
After defining the component with the decorator, you can now use it in your Angular application by including its selector (<app-example>) in another component's template or in your routing configuration.
<!-- app.component.html -->
<h1>Welcome to my Angular App</h1>
<app-example></app-example>
Now, let's create a custom decorator in Angular. We'll create a decorator that logs messages when a method is called.
Define the Custom Decorator
Create a TypeScript function that acts as your custom decorator. This function will log messages when a method is called.
function LogMethod(target: any, key: string, descriptor: PropertyDescriptor) {
const originalMethod = descriptor.value;
descriptor.value = function (...args: any[]) {
console.log(`Method ${key} is called with arguments: ${JSON.stringify(args)}`);
return originalMethod.apply(this, args);
};
return descriptor;
}
1. In this example:
Apply the Custom Decorator
Apply the LogMethod decorator to a method within a class.
class ExampleClass {
@LogMethod
greet(name: string) {
console.log(`Hello, ${name}!`);
}
}
const instance = new ExampleClass();
instance.greet('Alice');
Output:
Method greet is called with arguments: ["Alice"]
Hello, Alice!
2. In this example:
Create a Component
Define an Angular component using the @Component decorator to specify its metadata.
import { Component } from '@angular/core';
@Component({
selector: 'app-greeting',
template: `
<h2>Welcome, {{ name }}</h2>
<button (click)="sayHello()">Say Hello</button>
`,
styles: ['button { margin-top: 10px; }']
})
export class GreetingComponent {
name: string = 'John';
sayHello() {
console.log(`Hello, ${this.name}!`);
}
}
Use the Component
Include the GreetingComponent selector (<app-greeting>) in another component's template.
import { Component } from '@angular/core';
@Component({
selector: 'app-root',
template: `
<h1>Welcome to My Angular App</h1>
<app-greeting></app-greeting>
`
})
export class AppComponent {
}
This example showcases how decorators like @Component are used in Angular to define components and encapsulate their behaviour and presentation. It illustrates the use of decorators' metadata (selector, template, styles) to structure and compose Angular applications effectively.
In Angular, several commonly used decorators streamline development and enhance functionality across various aspects of applications. Here are some of the most frequently used decorators in Angular:
Example:
import { Component } from '@angular/core';
@Component({
selector: 'app-example',
templateUrl: './example.component.html',
styleUrls: ['./example.component.css']
})
export class ExampleComponent {
// Component logic here
}
Example:
import { Injectable } from '@angular/core';
@Injectable({
provided: 'root'
})
export class DataService {
// Service logic here
}
Example:
import { NgModule } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
@NgModule({
declarations: [AppComponent, ExampleComponent],
imports: [BrowserModule],
providers: [DataService],
bootstrap: [AppComponent]
})
export class AppModule {
// Module logic here
}
Example:
import { Component, Input } from '@angular/core';
@Component({
selector: 'app-child',
template: `<p>{{ inputData }}</p>`
})
export class ChildComponent {
@Input() inputData: string;
}
Example:
import { Component, Output, EventEmitter } from '@angular/core';
@Component({
selector: 'app-child',
template: `<button (click)="sendMessage()">Send Message</button>`
})
export class ChildComponent {
@Output() message event = new EventEmitter<string>();
sendMessage() {
this.messageEvent.emit('Hello from child!');
}
}
Example:
import { Component, ViewChild, ElementRef } from '@angular/core';
@Component({
selector: 'app-parent',
template: `
<app-child #childComponent></app-child>
<button (click)="callChildMethod()">Call Child Method</button>
`
})
export class ParentComponent {
@ViewChild('childComponent') childComponent: ElementRef;
callChildMethod() {
this.childComponent.someMethod();
}
}
These decorators are fundamental in Angular development for defining components, managing dependencies, handling data flow between components, accessing child components, and organizing application structures efficiently.
They leverage TypeScript's decorators to provide rich metadata and facilitate Angular's powerful features, such as dependency injection, data binding, and component composition.
In Angular, passing data between components is facilitated primarily through decorators like @Input and @Output. These decorators play a crucial role in establishing communication between parent and child components in a structured and efficient manner.
The @Input decorator allows a parent component to pass data into a child component. Here’s how you can use it:
Define an input property using the @Input() decorator in the child component class.
import { Component, Input } from '@angular/core';
@Component({
selector: 'app-child',
template: `<p>{{ inputData }}</p>`
})
export class ChildComponent {
@Input() inputData: string;
}
Bind to the child component's input property using property binding ([]).
import { Component } from '@angular/core';
@Component({
selector: 'app-parent',
template: `
<app-child [inputData]="parent data"></app-child>
`
})
export class ParentComponent {
parent data: string = 'Data from parent';
}
The @Output decorator allows a child component to emit events (pass data) to its parent component. Here’s how you can use it:
Define an output property using @Output() decorator of type EventEmitter.
import { Component, Output, EventEmitter } from '@angular/core';
@Component({
selector: 'app-child',
template: `<button (click)="sendData()">Send Data</button>`
})
export class ChildComponent {
@Output() data event = new EventEmitter<string>();
sendData() {
this.dataEvent.emit('Data from child');
}
}
Bind to the child component's output event using event binding (()).
import { Component } from '@angular/core';
@Component({
selector: 'app-parent',
template: `
<app-child (data event)="receive data($event)"></app-child>
<p>Received Data: {{ received data }}</p>
`
})
export class ParentComponent {
received data: string;
receiveData(data: string) {
this.receivedData = data;
}
}
These decorators are essential in Angular for building dynamic and interactive applications by enabling seamless data flow between components, supporting component composition, and enhancing reusability and maintainability in Angular applications.
Decorators in Angular have several practical applications in real-world scenarios, contributing significantly to the structure, functionality, and maintainability of applications. Here are some key real-time applications of decorators in Angular.
1. Component Definition and Configuration:
2. Service Injection and Dependency Management:
3. Module Definition and Organization:
4. Custom Decorators for Cross-Cutting Concerns:
5. Data Binding and Interaction Between Components:
6. Accessing DOM Elements or Child Components:
7. Metadata-driven Development and Configuration:
Overall, decorators in Angular are pivotal in modern web development, offering a declarative way to enhance and extend application functionality, structure code effectively, promote best practices, and deliver robust, scalable applications suited for real-time demands and complex business requirements. Their versatility and efficiency make them indispensable tools in building maintainable and performant Angular applications.
Decorators in Angular serve several crucial purposes that make them essential in modern web development.
In conclusion, Angular decorators enhance code readability, maintainability, and functionality. They provide a powerful way to add metadata and behaviour to classes, methods, and properties, thereby optimizing application architecture and performance. Embracing decorators empowers developers to write cleaner, more efficient Angular applications.
Copy and paste below code to page Head section
Decorators are functions that modify classes, methods, or properties in Angular, adding metadata or behaviour.
Decorators use the @ symbol followed by the decorator name to apply functionality to classes, methods, or properties.
They are used for dependency injection, component, and directive metadata, method and property enhancement, and more.
No, they are not mandatory but highly recommended for efficiently structuring and enhancing Angular applications.
Decorators can be applied to classes (e.g., @Component, @Injectable) and methods (e.g., @ViewChild, @HostListener).
Yes, Angular provides several built-in decorators like @Component, @Directive, @Injectable, @Input, @Output, etc.