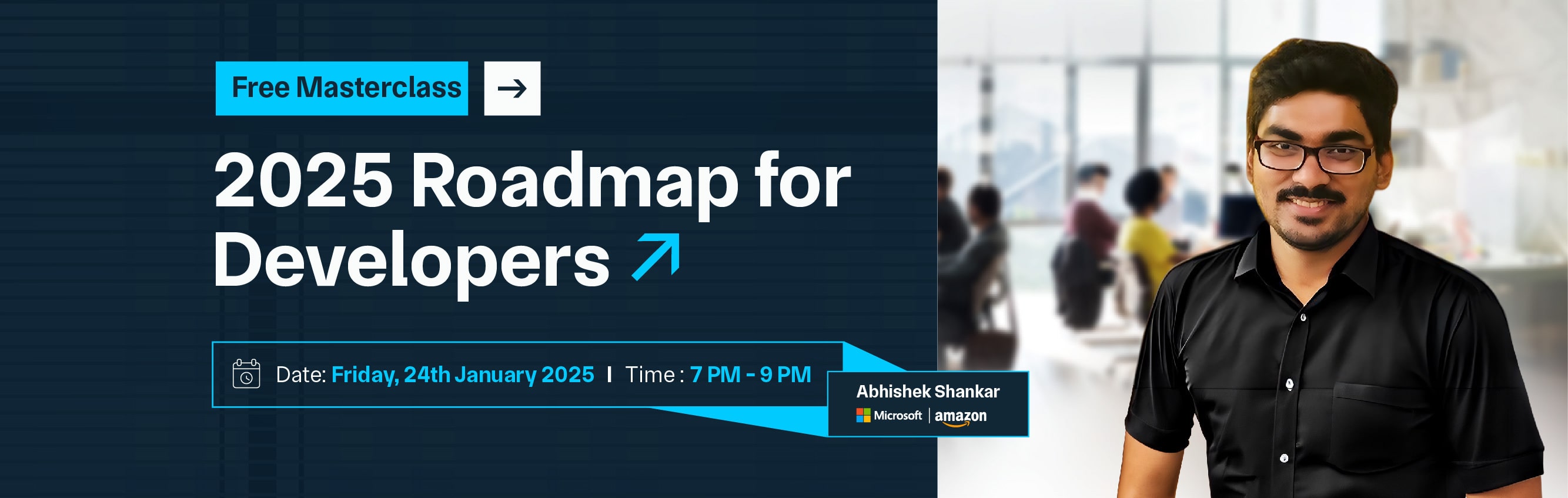

When choosing between Cassandra and MongoDB, it's essential to understand their unique strengths and ideal use cases. Cassandra excels in scenarios requiring high availability and scalability. It’s designed for handling large volumes of data across multiple nodes with no single point of failure. Its distributed architecture and support for multi-datacenter replication make it suitable for applications needing 24/7 uptime and fault tolerance. Cassandra uses a column-family data model, which is beneficial for write-heavy operations and time-series data.
However, it can be more complex to manage and optimize due to its eventual consistency model. MongoDB, on the other hand, is a document-oriented NoSQL database known for its ease of use and flexibility. It stores data in JSON-like BSON format, making it well-suited for applications with dynamic or evolving schemas. MongoDB offers strong consistency and is a good fit for applications requiring complex queries and indexing.
Its user-friendly interface and rich query capabilities make it popular among developers for rapid development and iteration. Chose Cassandra for high write throughput and distributed fault-tolerant systems and MongoDB for flexibility, ease of use, and complex queries. Cassandra excels in high availability and scalability with a distributed model, while MongoDB offers flexibility and ease of use for dynamic schemas.
Cassandra and MongoDB are both popular NoSQL databases but cater to different needs. Cassandra is known for its high scalability and availability, while MongoDB offers flexibility and ease of use for varying data structures.
Apache Cassandra is a highly scalable, distributed NoSQL database designed for handling large volumes of data across many commodity servers with no single point of failure. It offers high availability and fault tolerance through its distributed architecture, ensuring continuous operation and robust performance even in the face of hardware failures.
Cassandra's data model uses column families and supports horizontal scaling, making it ideal for applications with heavy write loads and high throughput requirements. However, it operates with eventual consistency, which may only suit some use cases, particularly those needing immediate data accuracy.
Example:
-- Create a keyspace
CREATE KEYSPACE example_keyspace WITH replication = {'class': 'SimpleStrategy', 'replication_factor': 3};
-- Use the keyspace
USE example_keyspace;
-- Create a table
CREATE TABLE users (user_id UUID PRIMARY KEY, name TEXT, age INT);
-- Insert data
INSERT INTO users (user_id, name, age) VALUES (uuid(), 'Alice', 30);
-- Query data
SELECT * FROM users;
MongoDB is a popular, open-source NoSQL database designed for ease of use and scalability. It stores data in flexible, JSON-like BSON documents, allowing for a dynamic schema that adapts to changing application requirements. MongoDB supports powerful querying, indexing, and aggregation capabilities, making it suitable for a wide range of applications.
It provides high availability through replica sets and scalability through sharding, which distributes data across multiple servers. Its user-friendly design and robust feature set make it a go-to choice for developers working with large, evolving datasets.
Example:
// Create a database
use examples;
// Create a collection
db.users.insertOne({ name: 'Alice', age: 30 });
// Query data
db.users.find({ age: { $gt: 25 } });
Both databases have broad language support, but MongoDB generally has more extensive driver support across a wider array of programming languages.
CQL (Cassandra Query Language): CQL is designed to be similar to SQL, allowing for familiar querying operations like SELECT, INSERT, UPDATE, and DELETE. However, CQL is tailored to Cassandra’s distributed architecture and column-family data model. It supports basic querying and indexing but does not offer the full range of SQL features, such as joins or subqueries.
Example:
-- Create a keyspace
CREATE KEYSPACE example_keyspace WITH replication = {'class': 'SimpleStrategy', 'replication_factor': 3};
-- Use the keyspace
USE example_keyspace;
-- Create a table
CREATE TABLE users (user_id UUID PRIMARY KEY, name TEXT, age INT);
-- Insert data
INSERT INTO users (user_id, name, age) VALUES (uuid(), 'Alice', 30);
-- Query data
SELECT * FROM users WHERE age > 25;
MQL (MongoDB Query Language): MQL is a flexible and powerful query language that operates with MongoDB’s document model. It uses JSON-like syntax for queries, supporting a wide range of operations, including filtering, sorting, and aggregation. MongoDB's aggregation framework allows for more complex data processing and analysis.
Example:
// Create a collection and insert a document
db.users.insertOne({ name: 'Alice', age: 30 });
// Query data
db.users.find({ age: { $gt: 25 } });
// Aggregation example
db.users.aggregate([
{ $match: { age: { $gt: 25 } } },
{ $group: { _id: null, averageAge: { $avg: "$age" } } }
]);
Data Model: Cassandra uses a column-family data model, which is similar to a table in relational databases but more flexible. Data is organized into tables where each row can have a different set of columns. It is designed to handle large volumes of data distributed across many nodes.
Example Schema:
CREATE TABLE user_profiles (
user_id UUID PRIMARY KEY,
name TEXT,
email TEXT,
signup_date TIMESTAMP
);
Data Model: MongoDB uses a document-oriented data model, where data is stored in BSON (Binary JSON) format. Each record is a document and can have a different structure.
Example Schema:
db.user_profiles.insertOne({
user_id: ObjectId("unique_id"),
name: "Alice",
email: "alice@example.com",
signup_date: new Date()
});
1. NoSQL Databases: Both Cassandra and MongoDB are NoSQL databases, meaning they do not use traditional relational database schemas and are designed to handle large-scale, unstructured, or semi-structured data.
2. Horizontal Scalability: Both databases support horizontal scaling, allowing them to distribute data across multiple nodes or servers. This scalability enables them to handle increasing volumes of data and traffic by simply adding more nodes to the cluster.
3. High Availability: Cassandra and MongoDB both offer high availability through replication. Cassandra achieves this through its distributed architecture and multi-datacenter replication, while MongoDB uses replica sets to ensure data redundancy and failover capabilities.
4. Flexible Schema: Both databases provide schema flexibility. Cassandra allows for dynamic column families where columns can be added or removed without affecting existing rows. MongoDB uses a document-oriented model with BSON format, enabling each document in a collection to have its structure.
5. Distributed Architecture: Both Cassandra and MongoDB are built to operate in distributed environments. They distribute data across multiple nodes, which helps in balancing load and improving fault tolerance.
6. Data Modeling: While the models differ (column-family for Cassandra and document-oriented for MongoDB), both support rich data modeling that can handle complex data structures, Cassandra’s wide rows and MongoDB’s nested documents both allow for flexible and varied data representation.
7. APIs and Drivers: Both databases provide official drivers and APIs for various programming languages, making it easier for developers to integrate them into applications. These drivers support essential operations like querying, updating, and managing data.
8. Community and Ecosystem: Both Cassandra and MongoDB have strong community support and a growing ecosystem of tools, libraries, and integrations. They are widely used and supported by extensive documentation and third-party tools.
9. Query Capabilities: Both databases offer querying capabilities, though with different approaches. Cassandra uses CQL (Cassandra Query Language) for SQL-like queries, while MongoDB uses MQL (MongoDB Query Language) with a JSON-like syntax. Both allow for basic operations such as filtering, sorting, and indexing.
10. Real-Time Processing: Both are capable of real-time data processing. They are designed to handle high-throughput scenarios and can be used for applications requiring quick data access and updates.
While Cassandra and MongoDB differ in their data models and specific features, they share key similarities in their NoSQL nature, scalability, high availability, schema flexibility, and distributed architecture.
Choosing between Cassandra and MongoDB depends on several factors related to your application's specific needs and requirements. Here's a guide to help you decide:
1. Cassandra:
2. MongoDB:
1. Cassandra:
2. MongoDB:
1. Cassandra:
2. MongoDB:
1. Cassandra:
2. MongoDB:
1. Cassandra:
2. MongoDB:
Choosing between Cassandra and MongoDB hinges on understanding your application's specific needs and constraints. Cassandra excels in scenarios requiring massive scalability, high write throughput, and fault tolerance, making it ideal for large-scale, distributed applications with high availability demands. Its column-family data model and distributed architecture are designed to handle vast amounts of data across multiple nodes seamlessly. Still, it comes with operational complexity and eventual consistency that may not suit every use case. On the other hand, MongoDB offers flexibility with its document-oriented model, making it suitable for applications with dynamic schemas and complex query requirements.
Its powerful aggregation framework, rich indexing options, and strong consistency support are ideal for use cases that demand sophisticated querying and real-time analytics. MongoDB’s ease of use and operational simplicity make it a strong candidate for applications where schema flexibility and transactional support are crucial. Ultimately, the choice between Cassandra and MongoDB should be guided by factors such as data model preferences, scalability requirements, consistency needs, and operational considerations. By aligning these aspects with the strengths of each database, you can select the one that best meets your application's requirements and goals.
Copy and paste below code to page Head section
Cassandra is a distributed NoSQL database optimized for high write throughput and horizontal scalability with an eventual consistency model. It uses a column-family data model suitable for large-scale applications requiring high availability and fault tolerance. MongoDB is a document-oriented NoSQL database that offers flexible schemas and advanced querying capabilities. It supports strong consistency and complex aggregations, making it ideal for applications needing rich query features and dynamic data structures.
Cassandra is better suited for high write throughput due to its design optimized for handling large volumes of writes across distributed nodes. It is ideal for applications with heavy write loads, such as time-series data or logging systems.
Cassandra provides seamless horizontal scalability by adding more nodes to the cluster, which automatically balances the data. This allows for linear scalability, making it suitable for large-scale deployments. MongoDB also supports horizontal scalability through sharding, where data is distributed across multiple shards or servers. Sharding helps manage large datasets and balances the load, but it requires careful planning to ensure effective distribution and performance.
Cassandra has limited support for complex queries and aggregations. While it supports basic operations like filtering and grouping, it lacks advanced features like joins and complex aggregations. MongoDB excels in complex queries and aggregations with its powerful Aggregation Framework. It allows for sophisticated data processing, including filtering, grouping, and sorting, making it suitable for applications requiring detailed data analysis.
Cassandra uses an eventual consistency model, prioritizing high availability and partition tolerance over immediate consistency. This means that while data will eventually become consistent across nodes, there may be temporary discrepancies. MongoDB offers strong consistency with configurable read and write concerns. In replica sets, it provides consistent reads from the primary node and supports multi-document ACID transactions for applications requiring strict data integrity.
Cassandra can be complex to set up and manage due to its distributed nature and multi-datacenter configurations. It requires expertise in tuning and maintaining the cluster to ensure optimal performance and reliability. MongoDB generally has a simpler setup and management process compared to Cassandra. Its user-friendly tools and extensive documentation make it easier to deploy and operate, though managing sharding and replication still requires attention.