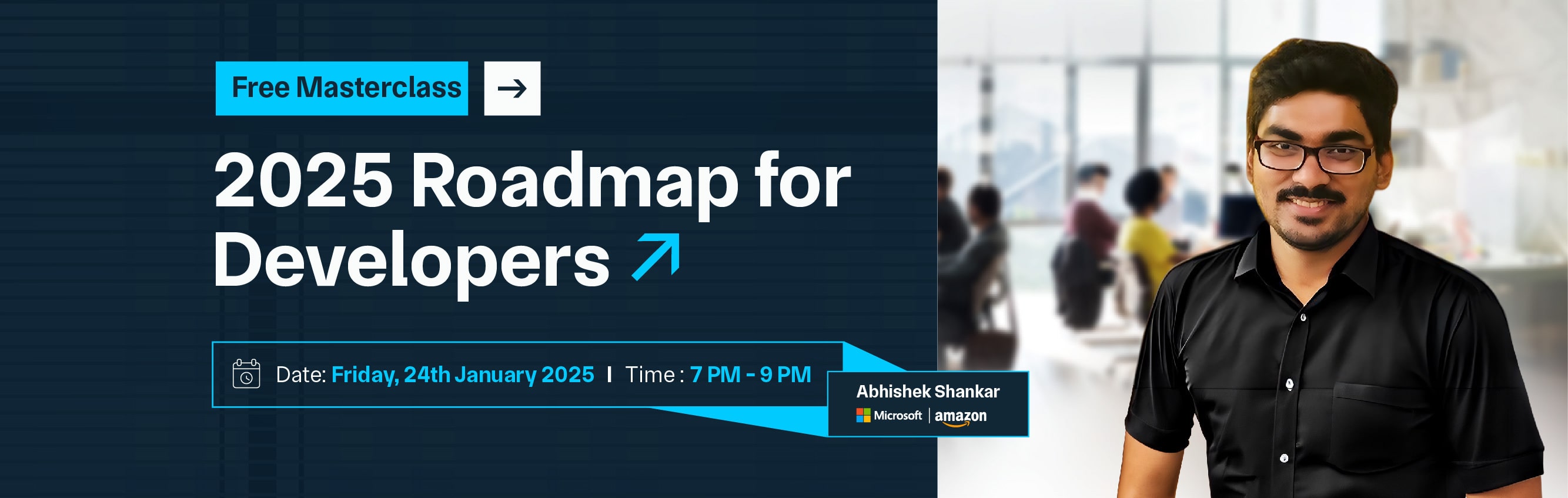

For example, if you have a loop searching for a number and use a break when the number is found, the loop stops immediately, avoiding unnecessary iterations. Conversely, the continue statement skips the remaining code inside the current loop iteration and proceeds directly to the next iteration. This is helpful when you want to bypass certain conditions without stopping the entire loop.
For instance, if you want to print only odd numbers in a range, you can continue to skip over even numbers, thus only executing the print statement for odd values. When used together, break and continue provide fine-grained control over loop execution. For example, you might continue to skip some iterations but use break to exit the loop early when a critical condition is met. This dual approach enhances loop efficiency and readability by handling complex control flows effectively.
Control flow statements in Python are essential for directing the flow of execution in a program. They enable you to make decisions, repeat actions, and control the flow of code execution based on various conditions. Understanding these statements is crucial for writing effective and efficient programs.
1. Conditional Statements:
Purpose: To execute different blocks of code based on certain conditions.
Key Statements:
Example:
x = 10
if x > 0:
print("Positive number")
elif x < 0:
print("Negative number")
else:
print("Zero")
2. Looping Statements:
Purpose: To repeat a block of code multiple times based on a condition or a sequence.
Key Statements:
Example:
for i in range(5):
print(i)
# Output: 0 1 2 3 4
x = 0
while x < 5:
print(x)
x += 1
# Output: 0 1 2 3 4
3. Control Flow Modifiers:
Purpose: To modify the behavior of loops and conditional blocks.
Key Statements:
Example:
for i in range(5):
if i == 2:
continue
print(i)
# Output: 0 1 3 4
for i in range(5):
if i == 3:
break
print(i)
# Output: 0 1 2
Control flow statements are fundamental for implementing logic and handling various scenarios in your code, allowing for more dynamic and flexible programming.
The break statement in Python is used to exit a loop prematurely, regardless of whether the loop’s condition is still true. It can be applied in both for and while loops to terminate the loop’s execution and immediately jump to the code that follows the loop.
break
Exiting for Loops:
Example:
numbers = [1, 2, 3, 4, 5]
for number in numbers:
if number == 3:
break
print(number)
Output:
1
2
Exiting while Loops:
Example:
count = 0
while True:
count += 1
if count > 3:
break
print(count)
Output:
1
2
3
The break statement is a powerful tool for managing loop execution and improving the efficiency of your code by preventing unnecessary iterations.
The continue statement in Python is used within loops to skip the remaining code inside the current iteration and proceed directly to the next iteration of the loop. This statement helps manage the flow of loops by allowing you to bypass specific conditions without terminating the entire loop.
continue
Using continue in for Loops:
Example:
numbers = [1, 2, 3, 4, 5]
for number in numbers:
if number % 2 == 0:
continue
print(number)
Output:
1
3
5
Using continue in while Loops:
Example:
count = 0
while count < 5:
count += 1
if count == 3:
continue
print(count)
Output:
1
2
4
5
The continue statement is a valuable control flow tool in Python that allows for efficient loop management by skipping unnecessary code execution and maintaining smooth loop operations.
In Python, combining break and continue within the same loop allows for more nuanced control over loop execution. While break terminates the loop entirely, continue skips the remainder of the current iteration and proceeds to the next one. This combination can be particularly useful for managing complex looping conditions and optimizing performance.
Combining break and continue in a for Loop:
Example:
numbers = [1, 2, 3, 4, 5, 6, 7]
for number in numbers:
if number % 2 == 0:
continue # Skip even numbers
if number == 5:
break # Exit loop when number equals 5
print(number)
Output:
1
3
Combining break and continue in a while Loop:
Example:
count = 0
while count < 10:
count += 1
if count % 3 == 0:
continue # Skip printing multiples of 3
if count == 7:
break # Exit loop when count equals 7
print(count)
Output:
1
2
4
5
In Python, both break and continue are control flow statements used within loops to modify their behavior, but they serve different purposes and impact the loop execution in distinct ways.
Using break and continue statements effectively can enhance the clarity and performance of your code. Here are some best practices to consider:
Comment Your Code: Add comments to explain why break or continue is used. This helps others (or your future self) understand the purpose of these statements.
for i in range(10):
if i % 2 == 0:
continue # Skip even numbers
if i == 7:
break # Stop the loop if i is 7
print(i)
Prevent Infinite Loops: Be cautious with continue statements inside while loops to avoid creating infinite loops. Ensure the loop’s condition will eventually become False.
count = 0
while count < 10:
count += 1
if count % 2 == 0:
continue
print(count)
Reduce Unnecessary Iterations: Use break to exit loops early when a result is found or a condition is met, thus avoiding unnecessary iterations.
for item in collection:
if condition(item):
process(item)
break # Exit loop as soon as the condition is met
Simplify Nested Loops: If you find yourself using break and continue extensively in nested loops, consider refactoring your code to simplify the logic or break it into smaller functions.
def process_item(item):
if item meets_condition:
return True
return False
for item in collection:
if process_item(item):
break
By following these best practices, you can use break and continue statements effectively to manage loop control in a way that is both efficient and easy to understand.
Using break and continue statements in Python can streamline loop control, but improper use can lead to several issues. Here are common pitfalls to watch out for:
Problem: Misuse of continue in while loops can result in infinite loops if the loop condition never changes to False.
Example:
count = 0
while count < 10:
count += 1
if count % 2 == 0:
continue
# Missing code to update count or exit loop
Solution: Ensure the loop condition is eventually met and that all necessary updates occur to avoid endless iterations.
Problem: Overusing break and continue can make loops harder to understand, especially when nested or used in complex conditions.
Example:
for i in range(10):
if i % 2 == 0:
continue
if i > 5:
break
print(i)
Solution: Use break and continue sparingly and add comments to clarify their purpose.
Problem: Using continue can skip necessary code within the loop, potentially leading to incorrect program behavior.
Example:
for i in range(10):
if i % 2 == 0:
continue
print("Processing:", i)
# Some important code that could be skipped
Solution: Ensure that the skipped code is not essential for the correct execution of the loop.
Problem: Incorrect placement of break or continue can lead to unintended behavior, such as exiting or skipping more code than intended.
Example:
for i in range(5):
if i % 2 == 0:
for j in range(5):
if j == 2:
break
print(j)
Solution: Carefully place break and continue to ensure they affect only the intended loop level and scope.
Problem: Incorrect conditions for break and continue can lead to logical errors, such as prematurely stopping a loop or skipping necessary iterations.
Example:
for i in range(5):
if i == 2:
break
if i == 3:
continue
print(i)
Solution: Review and test loop conditions thoroughly to ensure they align with the desired logic.
Problem: Excessive use of break and continue can sometimes impact performance, especially if the conditions are complex or the loop iterates over large datasets.
Example:
for i in large_dataset:
if complex_condition(i):
continue
# More complex processing
Solution: Optimize conditions and processing logic to minimize performance impact and avoid unnecessary computations.
By being aware of these common pitfalls, you can use break and continue more effectively and avoid issues that could lead to bugs or reduced code clarity.
In Python, break and continue statements are powerful tools for controlling the flow of loops, allowing for more precise and efficient code execution. The break statement is used to exit a loop prematurely when a specific condition is met, making it ideal for stopping unnecessary iterations and optimizing performance. On the other hand, the continue statement allows you to skip the remainder of the current loop iteration and proceed to the next one, which is useful for bypassing certain conditions without terminating the loop entirely. Effective use of these statements can greatly enhance the clarity and efficiency of your code, but it's important to use them judiciously to avoid common pitfalls such as creating infinite loops, reducing readability, or skipping critical code.
By adhering to best practices—such as maintaining code readability, ensuring loop termination, and avoiding overuse—you can leverage break and continue to streamline loop management and improve program performance. Ultimately, understanding the distinct roles of break and continue, along with recognizing their potential pitfalls, will help you write cleaner, more effective Python code. By carefully integrating these control flow statements into your loops, you can achieve more robust and maintainable programming solutions.
Copy and paste below code to page Head section
The break statement is used to exit a loop immediately, regardless of whether the loop condition is still true. In contrast, the continue statement skips the remaining code inside the current loop iteration and proceeds to the next iteration of the loop.
Yes, you can use both break and continue together within the same loop. break will exit the loop entirely when its condition is met, while continue will skip the current iteration and move to the next one based on its condition.
When the break is used inside nested loops, it only exits the innermost loop that contains the break statement. The outer loops will continue to execute unless they also contain break statements.
Yes, the continue statement can be used in both for and while loops. It will skip to the next iteration of the loop and is useful for skipping specific iterations based on a condition.
To avoid infinite loops with continue, ensure that the loop’s condition will eventually be met and that the code will modify variables or conditions in a way that allows the loop to terminate.
Overusing break and continue can sometimes impact performance, especially if conditions are complex or if they result in excessive looping. To optimize performance, use these statements judiciously and ensure that they improve the loop’s efficiency.