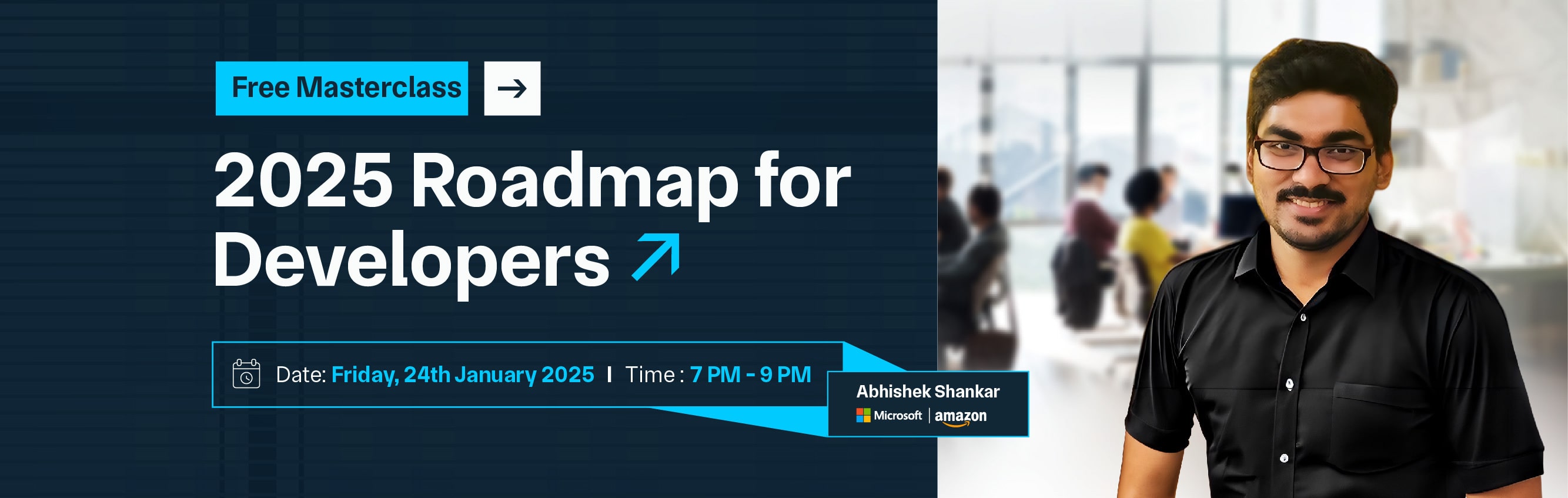

Axios is a popular JavaScript library used for making HTTP requests from browsers or Node.js environments. In the context of React applications, Axios simplifies the process of sending asynchronous HTTP requests to REST endpoints and performing CRUD operations (Create, Read, Update, Delete) with server-side data. Axios offers several key advantages for React developers.
First, it provides a simple and intuitive API for handling HTTP requests, supporting various methods such as GET, POST, PUT, DELETE, etc. Its promise-based approach allows for easy handling of responses using async/await syntax or promise chaining, making code more readable and maintainable. Additionally, Axios provides built-in support for interceptors, allowing you to globally handle request and response errors or modify requests before they are sent.
Integrating Axios with React typically involves installing it via npm or yarn, importing it into your components, and then using it to fetch data from APIs or send data to backend servers. Its ability to handle JSON data seamlessly and its robust error-handling capabilities make it a preferred choice for handling network requests in modern React applications. Axios simplifies the process of integrating backend APIs with React applications, providing a powerful yet straightforward mechanism for managing asynchronous HTTP requests and responses.
Axios is a JavaScript library that simplifies the process of making HTTP requests from both the browser and Node.js. In the context of React, Axios is commonly used to communicate with backend APIs and external servers. It provides an easy-to-use API that supports various HTTP methods, such as GET, POST, PUT, DELETE, etc., making it straightforward to perform CRUD operations and interact with RESTful APIs.
Axios operates asynchronously, leveraging JavaScript promises to handle responses. This allows React developers to manage data fetching and updating without blocking the user interface (UI), ensuring a smooth user experience. Axios also supports interceptors, which are middleware functions that can be used to globally handle errors modify requests or responses before they are sent or received.
To use Axios in a React application, developers typically install it via npm or yarn, import it into their components or utility files, and then use its methods to initiate HTTP requests. Axios seamlessly handles JSON data, which is common in modern web applications. Overall, Axios simplifies the integration of backend APIs with React applications by providing a robust and flexible mechanism for handling network requests and managing data flow between the frontend and backend systems.
In React applications, Axios is commonly used for several important reasons:
Axios is essential in React applications because it provides a powerful yet easy-to-use toolset for managing HTTP requests, handling asynchronous operations, and integrating with backend APIs effectively, thereby enhancing the functionality and performance of React-based web applications.
As per the documentation and commonly highlighted features of Axios for React applications, the key features include:
These features collectively make Axios a powerful and flexible choice for managing HTTP requests and handling data communication between React components and backend servers or APIs effectively.
React application involves several steps to set up the development environment and start building your project. Here’s a step-by-step guide:
Assuming you've already installed Node.js and npm as per the previous instructions:
npx create-react-app my-react-app
Replace my-react-app with your preferred project name.
cd my-react-app
Navigate into the directory you created in the first step.
Axios is a popular library for making HTTP requests from browsers and Node.js. You can install it using npm:
npm install axios
After installing Axios, your package.json file should include an entry for Axios under dependencies:
"dependencies": {
"axios": "^0.24.0",
// other dependencies
}
The exact version number may vary depending on the latest version available at the time of installation.
Here’s an example of how you might use Axios to make an HTTP GET request in your React application:
import React, { useEffect, useState } from 'react';
import axios from 'axios';
function App() {
const [data, setData] = useState(null);
useEffect(() => {
axios.get('https://jsonplaceholder.typicode.com/posts/1')
.then(response => {
setData(response.data);
})
.catch(error => {
console.error('Error fetching data: ', error);
});
}, []);
return (
<div className="App">
<header className="App-header">
<h1>React App with Axios Example</h1>
{data ? (
<div>
<h2>Title: {data.title}</h2>
<p>Body: {data.body}</p>
</div>
) : (
<p>Loading data...</p>
)}
</header>
</div>
);
}
export default App;
After setting up your React app and installing Axios, you can run the application with the following command:
npm start
This command starts the development server. Open your web browser and navigate to http://localhost:3000/ (or another port if 3000 is already in use) to view your React application running.
Before using Axios in a React application, it's important to set up the environment and dependencies properly. Here are the typical prerequisites:
npm install axios
yarn add axios
Once these prerequisites are in place, you can start using Axios in your React components to fetch data from APIs, send data to servers, handle errors, and manage asynchronous operations effectively. Remember to import Axios into your components or utility files where needed (import axios from 'axios';) and utilize its API methods according to your application's requirements.
Installing Axios in a React project is a straightforward process. Here's how you can do it:
1. Navigate to Your Project Directory: Open your terminal or command prompt and navigate to the root directory of your React project.
Install Axios: Use npm or yarn to install Axios. Choose one of the following commands based on your package manager preference:
npm install axios
Using yarn:
yarn add axios
2. This command will download Axios and add it to your project's dependencies.
3. Verify Installation: Once Axios is installed, you can verify it by checking your package.json file. Axios should appear in the list of dependencies.
Start Using Axios: You're now ready to use Axios in your React components or utility files. Import Axios where you need to make HTTP requests. Typically, you import Axios at the top of your file like this:
import axios from 'axios';
4. Example Usage: Here's a basic example of how you can use Axios to make a GET request to an API endpoint within a React component:
import React, { useEffect, useState } from 'react';
import axios from 'axios';
const MyComponent = () => {
const [data, setData] = useState([]);
useEffect(() => {
const fetchData = async () => {
try {
const response = await axios.get('https://api.example.com/data');
setData(response.data);
} catch (error) {
console.error('Error fetching data: ', error);
}
};
fetchData();
}, []);
return (
<div>
<h1>My Data:</h1>
<ul>
{data.map(item => (
<li key={item.id}>{item.name}</li>
))}
</ul>
</div>
);
};
export default MyComponent;
5. In this example:
6. Run Your React Application: After installing Axios and integrating it into your React components, start or restart your React development server to apply the changes and begin using Axios to fetch data from APIs.
By following these steps, you'll have Axios installed and ready to use in your React project, enabling seamless communication with APIs and backend services. Adjust the API endpoint and HTTP method as per your specific application needs.
Fetching and consuming data with Axios involves using various HTTP methods like GET, POST, and DELETE to interact with APIs. Below, I'll walk you through examples of how to perform each of these operations in a React component using Axios.
To fetch data from an API using Axios in a React component:
Install Axios (if not already installed):
npm install axios
Import Axios in your React component:
javascript
Copy code
import React, { useEffect, useState } from 'react';
import axios from 'axios';
const FetchDataExample = () => {
const [data, setData] = useState([]);
useEffect(() => {
const fetchData = async () => {
try {
const response = await axios.get('https://api.example.com/data');
setData(response.data);
} catch (error) {
console.error('Error fetching data: ', error);
}
};
fetchData();
}, []);
return (
<div>
<h1>Fetch Data Example</h1>
<ul>
{data.map(item => (
<li key={item.id}>{item.name}</li>
))}
</ul>
</div>
);
};
export default FetchDataExample;
Explanation:
To post data to an API using Axios:
import React, { useState } from 'react';
import axios from 'axios';
const PostDataExample = () => {
const [formData, setFormData] = useState({ title: '', body: '' });
const handleSubmit = async (e) => {
e.preventDefault();
try {
const response = await axios.post('https://api.example.com/posts', formData);
console.log('Post successful! Response:', response.data);
// Optionally, handle success or update state
} catch (error) {
console.error('Error posting data: ', error);
// Optionally, handle error
}
};
const handleChange = (e) => {
setFormData({ ...formData, [e.target.name]: e.target.value });
};
return (
<div>
<h1>Post Data Example</h1>
<form onSubmit={handleSubmit}>
<label>Title:</label>
<input type="text" name="title" value={formData.title} onChange={handleChange} />
<br />
<label>Body:</label>
<textarea name="body" value={formData.body} onChange={handleChange} />
<br />
<button type="submit">Submit</button>
</form>
</div>
);
};
export default PostDataExample;
Explanation:
To delete data from an API using Axios:
import React, { useState, useEffect } from 'react';
import axios from 'axios';
const DeleteDataExample = () => {
const [data, setData] = useState([]);
const [deletedId, setDeletedId] = useState(null);
useEffect(() => {
const fetchData = async () => {
try {
const response = await axios.get('https://api.example.com/data');
setData(response.data);
} catch (error) {
console.error('Error fetching data: ', error);
}
};
fetchData();
}, [deletedId]); // Refresh data when an item is deleted
const handleDelete = async (id) => {
try {
const response = await axios.delete(`https://api.example.com/data/${id}`);
console.log('Delete successful! Response:', response.data);
setDeletedId(id); // Trigger useEffect to refetch data
// Optionally, handle success or update state
} catch (error) {
console.error('Error deleting data: ', error);
// Optionally, handle error
}
};
return (
<div>
<h1>Delete Data Example</h1>
<ul>
{data.map(item => (
<li key={item.id}>
{item.name}
<button onClick={() => handleDelete(item.id)}>Delete</button>
</li>
))}
</ul>
</div>
);
};
export default DeleteDataExample;
Explanation:
These examples demonstrate how to fetch, post, and delete data using Axios within React components. Adjust API endpoints and data handling according to your specific application needs and API structure.
In Axios, when an error occurs during an HTTP request, the catch block will receive an error object that provides detailed information about what went wrong. This error object is an instance of JavaScript's Error object augmented by Axios to include additional properties relevant to HTTP errors. Here's a breakdown of the properties commonly found in the Axios error object:
1. message: A string that describes the error. This is typically the HTTP status message or a network error message.
2. response: This property is only available if an HTTP error response was received from the server (status code outside the range of 2xx). It contains several sub-properties:
3. request: This property contains the Axios request configuration that was used to make the request. It's useful for debugging purposes and includes things like the URL, method, headers, etc.
4. config: The configuration object that was used to make the request. It includes options like url, method, headers, params, etc.
import React, { useState } from 'react';
import axios from 'axios';
const ErrorHandlingExample = () => {
const [errorMessage, setErrorMessage] = useState('');
const fetchData = async () => {
try {
const response = await axios.get('https://api.example.com/data');
console.log('Data received:', response.data);
} catch (error) {
if (error.response) {
// The request was made and the server responded with a status code
// that falls out of the range of 2xx
console.log('Error response:', error.response.data);
console.log('Status code:', error.response.status);
console.log('Status message:', error.response.statusText);
setErrorMessage(`Error: ${error.response.status} ${error.response.statusText}`);
} else if (error.request) {
// The request was made but no response was received
console.log('No response received:', error.request);
setErrorMessage('No response received from server');
} else {
// Something happened in setting up the request that triggered an error
console.error('Error message:', error.message);
setErrorMessage('Error in making request');
}
}
};
return (
<div>
<h1>Error Handling Example</h1>
<button onClick={fetchData}>Fetch Data</button>
{errorMessage && <p>{errorMessage}</p>}
</div>
);
};
export default ErrorHandlingExample;
1. fetchData Function: This function attempts to make a GET request using Axios to https://api.example.com/data. If an error occurs during the request, it's caught in the catch block.
2. Error Object Handling: Inside the catch block, the error object is examined:
3. State Management: Depending on the type of error encountered, the component sets the errorMessage state accordingly, which can then be displayed in the UI.
By understanding and utilizing the properties of the Axios error object, you can effectively handle different types of errors that may occur during HTTP requests in your React applications. Adjust error handling logic as needed based on your specific application requirements and error scenarios.
To fetch data from an API using Axios in a React component:
Install Axios (if not already installed): Copy code
npm install axios
Import Axios in your React component:
javascript
Copy code
import React, { useEffect, useState } from 'react';
import axios from 'axios';
const FetchDataExample = () => {
const [data, setData] = useState([]);
useEffect(() => {
const fetchData = async () => {
try {
const response = await axios.get('https://jsonplaceholder.typicode.com/posts');
setData(response.data);
} catch (error) {
console.error('Error fetching data: ', error);
}
};
fetchData();
}, []);
return (
<div>
<h1>Fetch Data Example</h1>
<ul>
{data.map(item => (
<li key={item.id}>{item.title}</li>
))}
</ul>
</div>
);
};
export default FetchDataExample;
Explanation:
To post data to an API using Axios in a React component:
import React, { useState } from 'react';
import axios from 'axios';
const PostDataExample = () => {
const [formData, setFormData] = useState({ title: '', body: '' });
const handleSubmit = async (e) => {
e.preventDefault();
try {
const response = await axios.post('https://jsonplaceholder.typicode.com/posts', formData);
console.log('Post successful! Response:', response.data);
// Optionally, handle success or update state
} catch (error) {
console.error('Error posting data: ', error);
// Optionally, handle error
}
};
const handleChange = (e) => {
setFormData({ ...formData, [e.target.name]: e.target.value });
};
return (
<div>
<h1>Post Data Example</h1>
<form onSubmit={handleSubmit}>
<label>Title:</label>
<input type="text" name="title" value={formData.title} onChange={handleChange} />
<br />
<label>Body:</label>
<textarea name="body" value={formData.body} onChange={handleChange} />
<br />
<button type="submit">Submit</button>
</form>
</div>
);
};
export default PostDataExample;
Explanation:
Sending custom headers with Axios allows you to include additional information in your HTTP requests, such as authentication tokens, API keys, or custom metadata. Here's how you can send custom headers using Axios in a React application:
import React, { useEffect, useState } from 'react';
import axios from 'axios';
const CustomHeadersExample = () => {
const [data, setData] = useState([]);
const [error, setError] = useState(null);
useEffect(() => {
const fetchData = async () => {
try {
// Define custom headers
const headers = {
'Content-Type': 'application/json',
Authorization: 'Bearer YOUR_AUTH_TOKEN',
'X-Custom-Header': 'value'
// Add more headers as needed
};
// Make GET request with custom headers
const response = await axios.get('https://api.example.com/data', { headers });
setData(response.data);
} catch (error) {
console.error('Error fetching data: ', error);
setError(error.message);
}
};
fetchData();
}, []);
return (
<div>
<h1>Custom Headers Example</h1>
{error && <p>Error: {error}</p>}
<ul>
{data.map(item => (
<li key={item.id}>{item.name}</li>
))}
</ul>
</div>
);
};
export default CustomHeadersExample;
1. Define Custom Headers:
2. Make GET Request with Custom Headers:
3. Handling Errors:
4. Rendering Data:
Ensure you replace 'Bearer YOUR_AUTH_TOKEN' with your actual authentication token or remove it if your API doesn't require authentication. Adjust the URL (https://api.example.com/data) and data handling logic according to your specific API endpoints and requirements.
By using custom headers with Axios, you can enhance your HTTP requests by including necessary information for authentication, content type, and any other metadata required by your backend API.
Making multiple requests with Axios in a React application often involves scenarios where you need to fetch data from multiple endpoints concurrently or sequentially. Axios provides several ways to handle multiple requests, such as using Promise.all, async/await, or chaining requests. Below are examples of how to make multiple requests using Axios in React:
import React, { useEffect, useState } from 'react';
import axios from 'axios';
const MultipleRequestsExample = () => {
const [userData, setUserData] = useState(null);
const [posts, setPosts] = useState([]);
useEffect(() => {
const fetchData = async () => {
try {
// Array of request promises
const requests = [
axios.get('https://jsonplaceholder.typicode.com/users/1'),
axios.get('https://jsonplaceholder.typicode.com/posts?userId=1')
];
// Execute multiple requests concurrently using Promise.all
const [userResponse, postsResponse] = await Promise.all(requests);
// Extract data from responses
setUserData(userResponse.data);
setPosts(postsResponse.data);
} catch (error) {
console.error('Error fetching data: ', error);
}
};
fetchData();
}, []);
return (
<div>
<h1>Multiple Requests Example</h1>
<h2>User Info</h2>
{userData && (
<div>
<p>Name: {userData.name}</p>
<p>Email: {userData.email}</p>
<p>Website: {userData.website}</p>
</div>
)}
<h2>User Posts</h2>
<ul>
{posts.map(post => (
<li key={post.id}>{post.title}</li>
))}
</ul>
</div>
);
};
export default MultipleRequestsExample;
You can also chain requests sequentially using async/await for scenarios where the second request depends on data from the first request:
import React, { useEffect, useState } from 'react';
import axios from 'axios';
const SequentialRequestsExample = () => {
const [userData, setUserData] = useState(null);
const [userPosts, setUserPosts] = useState([]);
useEffect(() => {
const fetchData = async () => {
try {
// First request to fetch user data
const userResponse = await axios.get('https://jsonplaceholder.typicode.com/users/1');
setUserData(userResponse.data);
// Second request to fetch user's posts based on user data
const postsResponse = await axios.get(`https://jsonplaceholder.typicode.com/posts?userId=${userResponse.data.id}`);
setUserPosts(postsResponse.data);
} catch (error) {
console.error('Error fetching data: ', error);
}
};
fetchData();
}, []);
return (
<div>
<h1>Sequential Requests Example</h1>
<h2>User Info</h2>
{userData && (
<div>
<p>Name: {userData.name}</p>
<p>Email: {userData.email}</p>
<p>Website: {userData.website}</p>
</div>
)}
<h2>User Posts</h2>
<ul>
{userPosts.map(post => (
<li key={post.id}>{post.title}</li>
))}
</ul>
</div>
);
};
export default SequentialRequestsExample;
1. Chaining Requests: Requests are chained sequentially using await:
2. Setting State: Similar to the previous example, data from each request (userResponse.data and postsResponse.data) is stored in component state using setUserData and setUserPosts.
3. Rendering Data: Render the fetched data (userData and userPosts) in the JSX once available.
These examples demonstrate how to effectively make multiple HTTP requests using Axios in React components, handling different scenarios such as concurrent requests and sequential dependencies between requests.
Adjust the URLs (https://jsonplaceholder.typicode.com/...) and data handling logic according to your specific API endpoints and application requirements.
In Axios, shorthand methods provide a more concise syntax for making HTTP requests compared to using the full axios.get, axios.post, etc. These shorthand methods are straightforward and align closely with their respective HTTP methods. Here's how you can use shorthand methods in Axios within a React application:
import React, { useEffect, useState } from 'react';
import axios from 'axios';
const GetRequestExample = () => {
const [data, setData] = useState([]);
useEffect(() => {
const fetchData = async () => {
try {
const response = await axios.get('https://jsonplaceholder.typicode.com/posts');
setData(response.data);
} catch (error) {
console.error('Error fetching data: ', error);
}
};
fetchData();
}, []);
return (
<div>
<h1>GET Request Example</h1>
<ul>
{data.map(item => (
<li key={item.id}>{item.title}</li>
))}
</ul>
</div>
);
};
export default GetRequestExample;
import React, { useState } from 'react';
import axios from 'axios';
const PostRequestExample = () => {
const [formData, setFormData] = useState({ title: '', body: '' });
const handleSubmit = async (e) => {
e.preventDefault();
try {
const response = await axios.post('https://jsonplaceholder.typicode.com/posts', formData);
console.log('Post successful! Response:', response.data);
// Optionally, handle success or update state
} catch (error) {
console.error('Error posting data: ', error);
// Optionally, handle error
}
};
const handleChange = (e) => {
setFormData({ ...formData, [e.target.name]: e.target.value });
};
return (
<div>
<h1>POST Request Example</h1>
<form onSubmit={handleSubmit}>
<label>Title:</label>
<input type="text" name="title" value={formData.title} onChange={handleChange} />
<br />
<label>Body:</label>
<textarea name="body" value={formData.body} onChange={handleChange} />
<br />
<button type="submit">Submit</button>
</form>
</div>
);
};
export default PostRequestExample;
Using Axios shorthand methods in your React components simplifies the syntax for making HTTP requests and integrates well with React's state management and lifecycle methods (useEffect, useState, etc.). Adjust the URLs and data handling logic according to your specific API endpoints and application requirements.
Using Axios in React comes with several best practices that help maintain code quality, improve performance, and ensure robust error handling. Here are some best practices for React developers when using Axios:
// apiService.js
import axios from 'axios';
const API_BASE_URL = 'https://api.example.com';
export const fetchData = async (endpoint) => {
try {
const response = await axios.get(`${API_BASE_URL}/${endpoint}`);
return response.data;
} catch (error) {
throw error;
}
};
axios.interceptors.response.use(
response => response,
error => {
// Handle HTTP errors
if (error.response) {
console.error('HTTP error:', error.response.status);
// Optionally handle specific error status codes
// Redirect to login for unauthorized access (401)
} else if (error.request) {
console.error('Request error:', error.request);
// Network error or no response
// Notify user or retry request
} else {
console.error('Error:', error.message);
// Something else happened while setting up the request
}
return Promise.reject(error);
}
);
axios.defaults.headers.common['Authorization'] = 'Bearer YOUR_TOKEN';
axios.defaults.headers.post['Content-Type'] = 'application/json';
const CancelToken = axios.CancelToken;
let cancel;
const fetchData = async () => {
try {
const response = await axios.get('https://api.example.com/data', {
cancelToken: new CancelToken(function executor(c) {
// An executor function receives a cancel function as an argument
cancel = c;
})
});
setData(response.data);
} catch (error) {
if (axios.isCancel(error)) {
console.log('Request canceled', error.message);
} else {
console.error('Error fetching data: ', error);
}
}
};
// Call cancel() when needed
cancel();
const [loading, setLoading] = useState(false);
const fetchData = async () => {
setLoading(true);
try {
const response = await axios.get('https://api.example.com/data');
setData(response.data);
} catch (error) {
console.error('Error fetching data: ', error);
}
setLoading(false);
};
const [data, setData] = useState([]);
const fetchData = async () => {
try {
const response = await axios.get('https://api.example.com/data');
setData(prevData => [...prevData, response.data]);
// Or setData(response.data) depending on your state structure
} catch (error) {
console.error('Error fetching data: ', error);
}
};
// Using Jest with axios-mock-adapter for mocking Axios requests
import axios from 'axios';
import MockAdapter from 'axios-mock-adapter';
const mock = new MockAdapter(axios);
mock.onGet('/data').reply(200, { data: 'mocked data' });
// Test your component or service using the mocked Axios instance
Axios offers several advantages when used in React applications:
Axios is a powerful tool for managing HTTP requests in React applications, offering a promise-based API that simplifies asynchronous data fetching and interaction with APIs. Its concise syntax, support for interceptors and request cancellation, built-in CSRF protection, and community support make it an excellent choice for developers seeking efficient and reliable HTTP communication within their React projects.
By leveraging Axios, developers can enhance the performance, scalability, and maintainability of their applications while ensuring a smooth user experience through optimised data handling and error management.
Copy and paste below code to page Head section
Axios is a popular JavaScript library used for making HTTP requests from browsers or Node.js environments. In React, Axios simplifies the process of sending asynchronous requests to APIs and handling responses.
Axios allows you to handle errors using .catch() or async/await try...catch blocks. You can also use interceptors to handle errors or modify requests/responses globally.
Yes, Axios supports request cancellation using CancelToken. This feature allows you to cancel pending requests, which is useful for scenarios like component unmounting or navigation away from a page.
Axios includes built-in protection against Cross-Site Request Forgery (CSRF) attacks by allowing automatic setting of X-XSRF-TOKEN headers if provided by the server. This helps enhance security when interacting with APIs.
You can refer to the official Axios documentation at axios.github.io/axios/ for detailed API reference, examples, and guides on using Axios in both React and other JavaScript environments.
You can install Axios in a React project using npm or yarn. Here's how: npm install axios # or yarn add axios