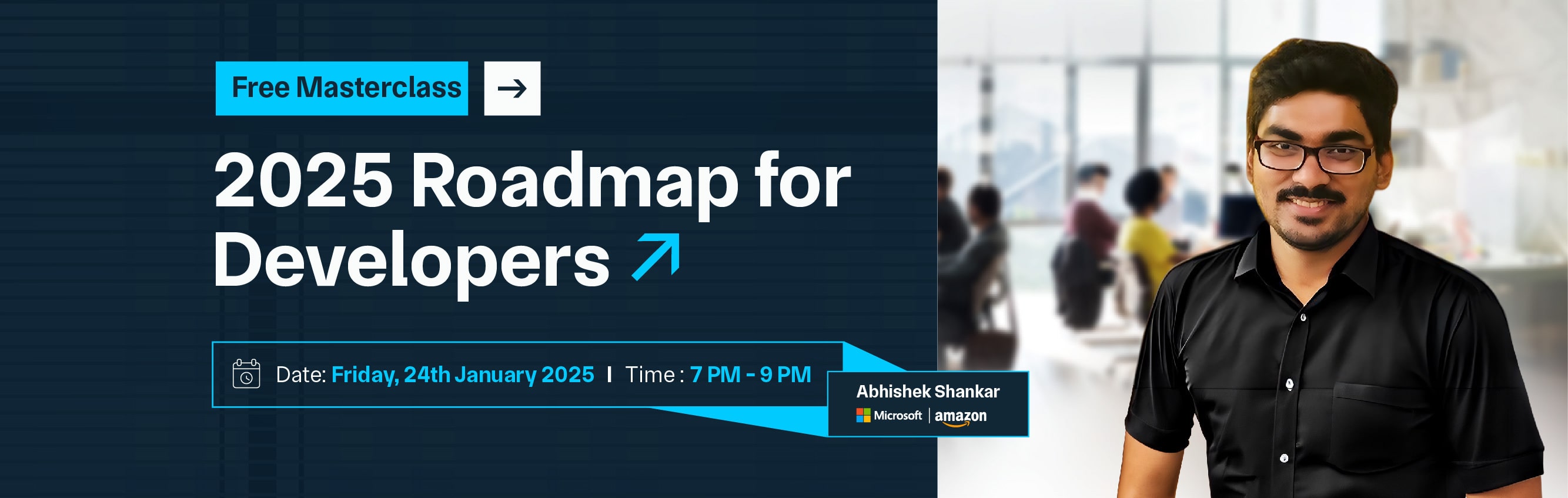

Ant Design's Table component is a robust and versatile tool for displaying tabular data in React applications. It simplifies the process of presenting data in a structured and interactive format, providing built-in support for sorting, filtering, pagination, and row selection. With Ant Design Table, developers can easily define columns, each with custom headers and data properties, and populate rows with data from a source array. The component is highly customizable, allowing for features like expandable rows, fixed headers, and custom cell renderers, making it suitable for complex use cases.
The Table component supports extensive styling options, either through Ant Design’s built-in themes or custom CSS, ensuring that tables can be seamlessly integrated into various design systems. Performance is optimized for large datasets, and accessibility is considered, ensuring the table is usable across different devices and by all users. Whether building data management dashboards, product listings, or user management systems, Ant Design Table provides a comprehensive solution to manage and present data efficiently.
By leveraging these features, developers can create intuitive and responsive tables that enhance the user experience in their React applications. Ant Design's Table component is a robust tool for displaying and managing tabular data in React. It features sorting, filtering, pagination, and row selection and offers extensive customization options, including expandable rows and fixed headers.
Ant Design is a popular open-source design system and React component library developed by Ant Financial, now part of Alibaba Group. It provides a comprehensive set of high-quality, reusable UI components and design guidelines to help developers create elegant and consistent user interfaces for web applications.
Ant Design is widely used in enterprise-level applications and by developers looking for a consistent and high-quality design framework to streamline their development process and enhance user experience.
The Table component in Ant Design is a powerful and flexible tool for displaying and managing tabular data in React applications. It is designed to handle a variety of data presentation needs, from simple lists to complex, interactive data tables.
Here’s a simple example of using the Table component:
import React from 'react';
import { Table } from 'antd';
const dataSource = [
{ key: '1', name: 'John Doe', age: 32, address: 'New York' },
{ key: '2', name: 'Jane Smith', age: 28, address: 'London' },
];
const columns = [
{ title: 'Name', dataIndex: 'name', key: 'name' },
{ title: 'Age', dataIndex: 'age', key: 'age' },
{ title: 'Address', dataIndex: 'address', key: 'address' },
];
const App = () => (
<Table dataSource={dataSource} columns={columns} />
);
export default App;
In this example, the Table component is configured with a data source and column definitions to display a simple table. This basic setup can be expanded with additional features and customizations to fit more complex requirements.
To install Ant Design and set it up in a React project, follow these step-by-step instructions:
If you don't already have a React project, you can create one using Create React App. Open your terminal and run:
npx create-react-app my-app
cd my-app
Add Ant Design to your project using npm or yarn:
Using npm:
npm install antd
Using yarn:
yarn add antd
Ant Design uses icons from @ant-design/icons. Install it to ensure all icons are available:
Using npm:
npm install @ant-design/icons
Using yarn:
yarn add @ant-design/icons
To use Ant Design components with the default styles, you need to import the Ant Design CSS into your project. Add the following line to the top of your src/index.js or src/App.js file:
import 'antd/dist/reset.css'; // For Ant Design 5.x
For Ant Design 4.x:
import 'antd/dist/antd.css'; // For Ant Design 4.x
You can now start using Ant Design components in your React project. Import components as needed and use them in your JSX. Here’s a basic example with a Button and Table component:
Example src/App.js:
import React from 'react';
import { Button, Table } from 'antd';
const dataSource = [
{ key: '1', name: 'John Doe', age: 32, address: 'New York' },
{ key: '2', name: 'Jane Smith', age: 28, address: 'London' },
];
const columns = [
{ title: 'Name', dataIndex: 'name', key: 'name' },
{ title: 'Age', dataIndex: 'age', key: 'age' },
{ title: 'Address', dataIndex: 'address', key: 'address' },
];
const App = () => (
<div>
<Button type="primary">Primary Button</Button>
<Table dataSource={dataSource} columns={columns} style={{ marginTop: 20 }} />
</div>
);
export default App;
If you need to customize Ant Design’s default theme, you can use Less for theming. Install Less and the Less loader:
Using npm:
npm install less less-loader
Using yarn:
yarn add less less-loader
Then, configure your project to use Less by modifying your craco.config.js or webpack.config.js (depending on your setup) to support Less variables.
Start your development server to see Ant Design in action:
Using npm:
npm start
Using yarn:
yarn start
This will launch your React app with Ant Design components, and you should see them styled with Ant Design’s default styles.
By following these steps, you’ll have Ant Design successfully integrated into your React project, allowing you to leverage its components and design system in your application.
The Table component from Ant Design is highly customizable and uses several key props to manage and display data. The two most fundamental props are dataSource and columns. Here’s a detailed breakdown of these props:
The dataSource prop provides the data that populates the rows of the table. It should be an array of objects, where each object represents a row in the table. Each object must include a unique key, which helps React identify and manage each row efficiently.
Key Points:
Example:
const dataSource = [
{
key: '1',
name: 'John Doe',
age: 32,
address: 'New York',
},
{
key: '2',
name: 'Jane Smith',
age: 28,
address: 'London',
},
{
key: '3',
name: 'Sam Brown',
age: 45,
address: 'Sydney',
},
];
The columns prop defines the structure of the table, specifying what data to show and how to display it. It is an array of objects where each object describes a column.
Key Points:
Type: Array of objects
Purpose: Defines how each column in the table should be rendered, including headers, data mapping, and optional custom rendering.
Common Properties:
Example:
const columns = [
{
title: 'Name',
dataIndex: 'name',
key: 'name',
},
{
title: 'Age',
dataIndex: 'age',
key: 'age',
},
{
title: 'Address',
dataIndex: 'address',
key: 'address',
},
];
When you use the Table component with these props, it combines the data from dataSource with the layout specified in columns to render the table.
Complete Example:
import React from 'react';
import { Table } from 'antd';
// Sample data
const dataSource = [
{ key: '1', name: 'John Doe', age: 32, address: 'New York' },
{ key: '2', name: 'Jane Smith', age: 28, address: 'London' },
{ key: '3', name: 'Sam Brown', age: 45, address: 'Sydney' },
];
// Column definitions
const columns = [
{ title: 'Name', dataIndex: 'name', key: 'name' },
{ title: 'Age', dataIndex: 'age', key: 'age' },
{ title: 'Address', dataIndex: 'address', key: 'address' },
];
// App component
const App = () => (
<Table dataSource={dataSource} columns={columns} />
);
export default App;
In this example:
By understanding and configuring dataSource and columns, you can effectively display and manage tabular data in your React applications using Ant Design’s Table component.
Columns define the structure of the table. Each column can have various properties to control its appearance and behavior.
Example Code Snippet:
import React from 'react';
import { Table } from 'antd';
const columns = [
{
title: 'Name',
dataIndex: 'name',
key: 'name',
sorter: (a, b) => a.name.localeCompare(b.name),
render: text => <strong>{text}</strong>,
width: '25%',
align: 'center',
},
{
title: 'Age',
dataIndex: 'age',
key: 'age',
sorter: (a, b) => a.age - b.age,
width: 100,
align: 'right',
},
{
title: 'Address',
dataIndex: 'address',
key: 'address',
filters: [
{ text: 'New York', value: 'New York' },
{ text: 'London', value: 'London' },
],
onFilter: (value, record) => record.address.includes(value),
width: '35%',
},
{
title: 'Description',
dataIndex: 'description',
key: 'description',
ellipsis: true,
width: '40%',
},
];
const App = () => (
<Table dataSource={dataSource} columns={columns} />
);
export default App;
The dataSource prop should be an array of objects. Each object represents a row and must include a unique key property, with other properties matching the dataIndex values defined in columns.
Example Code Snippet:
const dataSource = [
{
key: '1',
name: 'John Doe',
age: 32,
address: 'New York',
description: 'A short description about John Doe that might be very long.',
},
{
key: '2',
name: 'Jane Smith',
age: 28,
address: 'London',
description: 'A brief description about Jane Smith.',
},
{
key: '3',
name: 'Sam Brown',
age: 45,
address: 'Sydney',
description: 'A concise description about Sam Brown.',
},
];
Pagination can be controlled using the pagination prop, which allows you to configure pagination settings or turn it off entirely.
Example Code Snippet:
const App = () => (
<Table
dataSource={dataSource}
columns={columns}
pagination={{
pageSize: 2, // Number of rows per page
showSizeChanger: true, // Option to change page size
pageSizeOptions: ['2', '5', '10'], // Available page sizes
}}
/>
);
Use the sorter property in column definitions to enable sorting. The sorter function defines how to compare rows.
Example Code Snippet:
const columns = [
{
title: 'Age',
dataIndex: 'age',
key: 'age',
sorter: (a, b) => a.age - b.age,
width: 100,
},
];
The filters property allows you to define filter options for a column. The onFilter function specifies how the filtering is applied.
Example Code Snippet:
const columns = [
{
title: 'Address',
dataIndex: 'address',
key: 'address',
filters: [
{ text: 'New York', value: 'New York' },
{ text: 'London', value: 'London' },
],
onFilter: (value, record) => record.address.includes(value),
},
];
Use the expandable prop to add expandable rows, allowing users to view more details when they expand a row.
Example Code Snippet:
const App = () => (
<Table
dataSource={dataSource}
columns={columns}
expandable={{
expandedRowRender: record => <p style={{ margin: 0 }}>{record.description}</p>,
rowExpandable: record => record.name !== 'Not Expandable',
}}
/>
);
Use the rowSelection prop to enable row selection, allowing users to select one or multiple rows.
Example Code Snippet
const rowSelection = {
onChange: (selectedRowKeys, selectedRows) => {
console.log('Selected Row Keys:', selectedRowKeys);
console.log('Selected Rows:', selectedRows);
},
};
const App = () => (
<Table
dataSource={dataSource}
columns={columns}
rowSelection={rowSelection}
/>
);
Here’s an example combining all the features:
import React from 'react';
import { Table } from 'antd';
const dataSource = [
{ key: '1', name: 'John Doe', age: 32, address: 'New York', description: 'A short description about John Doe.' },
{ key: '2', name: 'Jane Smith', age: 28, address: 'London', description: 'A brief description about Jane Smith.' },
{ key: '3', name: 'Sam Brown', age: 45, address: 'Sydney', description: 'A concise description about Sam Brown.' },
];
const columns = [
{
title: 'Name',
dataIndex: 'name',
key: 'name',
sorter: (a, b) => a.name.localeCompare(b.name),
render: text => <strong>{text}</strong>,
width: '25%',
align: 'center',
},
{
title: 'Age',
dataIndex: 'age',
key: 'age',
sorter: (a, b) => a.age - b.age,
width: 100,
align: 'right',
},
{
title: 'Address',
dataIndex: 'address',
key: 'address',
filters: [
{ text: 'New York', value: 'New York' },
{ text: 'London', value: 'London' },
],
onFilter: (value, record) => record.address.includes(value),
width: '35%',
},
{
title: 'Description',
dataIndex: 'description',
key: 'description',
ellipsis: true,
width: '40%',
},
];
const rowSelection = {
onChange: (selectedRowKeys, selectedRows) => {
console.log('Selected Row Keys:', selectedRowKeys);
console.log('Selected Rows:', selectedRows);
},
};
const App = () => (
<Table
dataSource={dataSource}
columns={columns}
pagination={{ pageSize: 2, showSizeChanger: true, pageSizeOptions: ['2', '5', '10'] }}
expandable={{ expandedRowRender: record => <p style={{ margin: 0 }}>{record.description}</p> }}
rowSelection={rowSelection}
/>
);
export default App;
This example demonstrates how to combine column definitions, pagination, sorting, filtering, expandable rows, and row selection in a single Ant Design table. Customize these features to fit your application's needs.
Custom cell renderers in Ant Design’s Table component allow you to customize the content and appearance of table cells beyond the default display. This is done using the render property within each column definition. The render function provides flexibility to display custom elements, formats, or even complex UI components inside table cells.
The render property in a column definition is a function that receives the cell’s text value, the entire record (row data), and the row index. You can use this function to return any React element or component to be rendered in the cell.
Function Signature
render(text, record, index) => ReactNode
Ant Design’s Table component supports fixed columns and headers, which helps keep specific parts of the table visible while scrolling. This is particularly useful for large datasets where users need to retain context while scrolling through rows or columns.
Fixed headers ensure that the table header remains visible at the top of the viewport while scrolling vertically. To achieve this, you can use the scroll property of the Table component and set the x and y values for scrolling.
Example Code Snippet:
import React from 'react';
import { Table } from 'antd';
const dataSource = [
{ key: '1', name: 'John Doe', age: 32, address: 'New York' },
{ key: '2', name: 'Jane Smith', age: 28, address: 'London' },
// Add more rows as needed
];
const columns = [
{ title: 'Name', dataIndex: 'name', key: 'name' },
{ title: 'Age', dataIndex: 'age', key: 'age' },
{ title: 'Address', dataIndex: 'address', key: 'address' },
// Add more columns as needed
];
const App = () => (
<Table
dataSource={dataSource}
columns={columns}
scroll={{ y: 300 }} // Fixed header with vertical scroll
/>
);
export default App;
In the above example, setting scroll={{ y: 300 }} ensures that the header remains fixed while scrolling through 300 pixels of content vertically.
Fixed columns remain visible while scrolling horizontally. You can fix columns on the left or right side by setting the fixed property of each column.
Example Code Snippet:
import React from 'react';
import { Table } from 'antd';
const dataSource = [
{ key: '1', name: 'John Doe', age: 32, address: 'New York', description: 'A short description about John Doe.' },
{ key: '2', name: 'Jane Smith', age: 28, address: 'London', description: 'A brief description about Jane Smith.' },
// Add more rows as needed
];
const columns = [
{
title: 'Name',
dataIndex: 'name',
key: 'name',
fixed: 'left', // Fix column to the left
},
{
title: 'Age',
dataIndex: 'age',
key: 'age',
},
{
title: 'Address',
dataIndex: 'address',
key: 'address',
},
{
title: 'Description',
dataIndex: 'description',
key: 'description',
fixed: 'right', // Fix column to the right
width: 200, // Set a fixed width for the right-fixed column
},
];
const App = () => (
<Table
dataSource={dataSource}
columns={columns}
scroll={{ x: 800 }} // Fixed columns with horizontal scroll
/>
);
export default App;
In this example:
You can combine fixed headers and columns to create a table where both headers and specific columns remain visible during scrolling.
Example Code Snippet:
import React from 'react';
import { Table } from 'antd';
const dataSource = [
{ key: '1', name: 'John Doe', age: 32, address: 'New York', description: 'A short description about John Doe.' },
{ key: '2', name: 'Jane Smith', age: 28, address: 'London', description: 'A brief description about Jane Smith.' },
// Add more rows as needed
];
const columns = [
{
title: 'Name',
dataIndex: 'name',
key: 'name',
fixed: 'left', // Fix column to the left
},
{
title: 'Age',
dataIndex: 'age',
key: 'age',
},
{
title: 'Address',
dataIndex: 'address',
key: 'address',
},
{
title: 'Description',
dataIndex: 'description',
key: 'description',
fixed: 'right', // Fix column to the right
width: 200, // Set a fixed width for the right-fixed column
},
];
const App = () => (
<Table
dataSource={dataSource}
columns={columns}
scroll={{ x: 1200, y: 400 }} // Fixed columns and header with horizontal and vertical scroll
/>
);
export default App;
In this example:
Customizing the appearance of the Ant Design Table component allows you to tailor its look and feel to match your application's design. Whether you want to adjust the table’s borders, apply custom styles, or implement advanced features like conditional formatting and custom cell renderers, Ant Design provides flexible options.
By using built-in properties, custom CSS, and theme customization techniques, you can enhance the visual appeal and functionality of your tables to fit your user interface better.
Ant Design’s Table component offers several properties to help with basic styling:
You can use custom CSS to control the appearance of the table further:
The render function in column definitions allows you to customize the content and style of individual cells:
Ant Design supports global theme customization through Less variables, which affects all Ant Design components:
By leveraging these methods, you can achieve a highly customized and visually appealing table that meets the specific design requirements of your application.
In real-world applications, the Ant Design Table component can be tailored to meet various needs, enhancing user experience and improving data presentation. Here are some practical examples of how the Table component is utilized in different scenarios:
1. Dashboard with Data Metrics
Scenario: A business dashboard that displays key performance indicators (KPIs) such as sales figures, user engagement metrics, and financial summaries.
Customization:
2. E-commerce Product Listing
Scenario: An e-commerce site that lists products with details such as name, price, stock status, and actions like "Edit" or "Delete."
Customization:
3. Employee Directory
Scenario: An HR management system showing a list of employees, including their names, job titles, departments, and contact details.
Customization:
4. Financial Reports
Scenario: A financial report showing transaction details, amounts, dates, and categories.
Customization:
5. Customer Support Ticket Management
Scenario: A ticketing system where support agents track and manage customer support tickets, including ticket ID, customer name, issue status, and resolution time.
Customization:
These examples illustrate how the Ant Design Table component can be customized to fit various real-world applications, improving usability and functionality based on specific requirements and user needs.
When working with the Ant Design Table component, you might encounter several common issues. Understanding and troubleshooting these problems effectively can ensure a smooth development experience. Here are some common issues and their solutions:
Issue: The table fails to render or shows an empty state.
Possible Solutions:
Issue: Columns need to be aligned properly or headers need to be aligned.
Possible Solutions:
Issue: Pagination controls do not work or do not reflect the correct number of pages.
Possible Solutions:
Issue: Fixed headers or columns are not sticking as expected during scroll.
Possible Solutions:
Issue: Custom cell content or formatting does not appear as intended.
Possible Solutions:
Issue: The table becomes slow or unresponsive with large datasets.
Possible Solutions:
Issue: Row selection or interactions do not work as expected.
Possible Solutions:
Issue: Custom styles are not applying as intended or are conflicting with Ant Design styles.
Possible Solutions:
By addressing these common issues and applying the suggested solutions, you can effectively troubleshoot and resolve problems with the Ant Design Table component, ensuring a smooth and functional implementation in your application.
The Ant Design Table component offers numerous advantages:
The Ant Design Table component is a powerful and versatile tool for displaying tabular data in React applications. Its rich feature set, including built-in pagination, sorting, filtering, and customizable cell renderers, allows for sophisticated data management with minimal effort. The component’s ease of use, performance optimization, and flexible styling options make it a robust choice for developers looking to enhance user experience and application performance.
Additionally, its accessibility features and seamless integration with Ant Design’s ecosystem ensure a consistent and user-friendly interface. With strong community support and comprehensive documentation, the Ant Design Table component is well-suited to meet a wide range of data presentation needs in modern web applications.
Copy and paste below code to page Head section
To get started, install Ant Design using npm or yarn and import the Table component into your React application. Define the data source and column props to provide the data and structure for your table. Refer to the Ant Design documentation for detailed setup instructions and examples.
Yes, you can customize the Table component using built-in properties like bordered, size, and pagination. For more detailed styling, use custom CSS classes or Fewer variables for theme customization. You can also use custom cell renderers to tailor the appearance of individual cells further.
Pagination can be managed using the pagination prop, where you can specify settings such as pageSize, current, and total. You can also customize pagination controls or use server-side pagination to handle large datasets efficiently.
Column sorting and filtering can be enabled by using the sorter and filter properties in the column definitions. The sorter property allows you to define custom sorting functions, while the filters property lets you specify filter options and handling.
For large datasets, use pagination to limit the number of rows displayed at once. Additionally, consider implementing virtual scrolling to render only visible rows and improve performance. Ant Design’s Table also supports lazy loading techniques.
Yes, you can make rows expandable by using the expandedRowRender prop, which allows you to define a custom rendering function for expanded content. This feature is useful for displaying additional details or nested information within the table.